Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / Utils / GrowingArray.cs / 1305376 / GrowingArray.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // GrowingArray.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Diagnostics.Contracts; namespace System.Linq.Parallel { ////// A growing array. Unlike List{T}, it makes the internal array available to its user. /// ///internal class GrowingArray { T[] m_array; int m_count; const int DEFAULT_ARRAY_SIZE = 1024; internal GrowingArray() { m_array = new T[DEFAULT_ARRAY_SIZE]; m_count = 0; } //---------------------------------------------------------------------------------------- // Returns the internal array representing the list. Note that the array may be larger // than necessary to hold all elements in the list. // internal T[] InternalArray { get { return m_array; } } internal int Count { get { return m_count; } } internal void Add(T element) { if (m_count >= m_array.Length) { GrowArray(2 * m_array.Length); } m_array[m_count++] = element; } private void GrowArray(int newSize) { Contract.Assert(newSize > m_array.Length); T[] array2 = new T[newSize]; m_array.CopyTo(array2, 0); m_array = array2; } internal void CopyFrom(T[] otherArray, int otherCount) { // Ensure there is just enough room for both. if (m_count + otherCount > m_array.Length) { GrowArray(m_count + otherCount); } // And now just blit the keys directly. Array.Copy(otherArray, 0, m_array, m_count, otherCount); m_count += otherCount; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
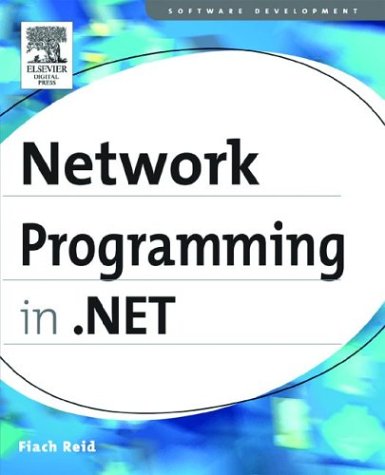
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StorageModelBuildProvider.cs
- SqlCommandBuilder.cs
- XmlnsCache.cs
- X500Name.cs
- DataSourceControlBuilder.cs
- GridToolTip.cs
- SerializerWriterEventHandlers.cs
- DataGridViewRowHeaderCell.cs
- HttpModulesSection.cs
- ZipFileInfoCollection.cs
- PointAnimationClockResource.cs
- ContainerUtilities.cs
- CustomWebEventKey.cs
- DBConnectionString.cs
- StrongTypingException.cs
- ReceiveReply.cs
- TextStore.cs
- DispatcherHookEventArgs.cs
- BrowserCapabilitiesCodeGenerator.cs
- EntityDescriptor.cs
- Workspace.cs
- HostProtectionException.cs
- StylusDevice.cs
- RegexGroup.cs
- FilteredDataSetHelper.cs
- LogManagementAsyncResult.cs
- ResourceExpression.cs
- ResourceDescriptionAttribute.cs
- DocumentPaginator.cs
- DoubleAnimationUsingKeyFrames.cs
- Window.cs
- DataGridViewColumnDesigner.cs
- EventLogPermissionEntry.cs
- X509ClientCertificateAuthenticationElement.cs
- ZipIOBlockManager.cs
- AssemblyBuilder.cs
- BaseParser.cs
- ProfilePropertyMetadata.cs
- SchemaDeclBase.cs
- Profiler.cs
- odbcmetadatacolumnnames.cs
- TemplateControl.cs
- RequiredAttributeAttribute.cs
- ImmutableAssemblyCacheEntry.cs
- RevocationPoint.cs
- PopupRoot.cs
- ProcessThread.cs
- OleDbFactory.cs
- VirtualPath.cs
- AssemblyCache.cs
- SamlDelegatingWriter.cs
- ToggleButton.cs
- SqlStream.cs
- XpsDocument.cs
- TextBoxView.cs
- SerialStream.cs
- TraceHwndHost.cs
- NetSectionGroup.cs
- DrawToolTipEventArgs.cs
- XmlUTF8TextWriter.cs
- Bold.cs
- CheckedPointers.cs
- OdbcConnectionHandle.cs
- InnerItemCollectionView.cs
- StylusOverProperty.cs
- TerminatorSinks.cs
- ResourceDictionary.cs
- _Win32.cs
- StrongNamePublicKeyBlob.cs
- ConfigurationPropertyCollection.cs
- TemplateContent.cs
- CookielessHelper.cs
- SelectorItemAutomationPeer.cs
- DataControlField.cs
- VirtualDirectoryMappingCollection.cs
- ObjectManager.cs
- StandardOleMarshalObject.cs
- WebResourceAttribute.cs
- RedistVersionInfo.cs
- MetadataSource.cs
- ParserHooks.cs
- MergePropertyDescriptor.cs
- XmlReaderDelegator.cs
- TemplatedEditableDesignerRegion.cs
- AccessKeyManager.cs
- InlineUIContainer.cs
- ConfigXmlAttribute.cs
- InfoCardRSAOAEPKeyExchangeDeformatter.cs
- PrivilegedConfigurationManager.cs
- UserCancellationException.cs
- SortableBindingList.cs
- StrongNamePublicKeyBlob.cs
- ItemDragEvent.cs
- Wildcard.cs
- CroppedBitmap.cs
- DataBindingExpressionBuilder.cs
- XamlWriter.cs
- AssemblyInfo.cs
- SettingsAttributeDictionary.cs
- HttpModuleAction.cs