Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / TrustUi / MS / Internal / documents / Application / SuppressedPackageProperties.cs / 1 / SuppressedPackageProperties.cs
//------------------------------------------------------------------------------ //// Copyright (C) Microsoft Corporation. All rights reserved. // //// Responsible for suppressing the Assert for unmanaged code permission and // replacing it with SecurityCritical attribute. // // // History: // 12/15/2005: [....]: Initial implementation. //----------------------------------------------------------------------------- using System; using System.IO.Packaging; using System.Security; using System.Security.Permissions; using MS.Internal.Permissions; namespace MS.Internal.Documents.Application { ////// Responsible for suppressing the Assert for unmanaged code permission and // replacing it with SecurityCritical attribute. /// ////// This is implemented as a decorating proxy where all calls are passed to /// a target PackageProperties object. The primary mitigation for the /// asserts is that the class sets the target from EncryptedPackageEnvelope /// the known good source for the target; as well the entire class is /// SecurityCritical. /// ////// Critical: /// 1) all methods supress a demand for compound file IO code by asserting the /// the CompountFileIOPermission /// 2) members fields are also critical; target because it's expected only to /// come from EncryptedPackageEnvelope and the permission because it's what /// we assert for /// [SecurityCritical(SecurityCriticalScope.Everything)] internal class SuppressedProperties : PackageProperties { #region Constructors //------------------------------------------------------------------------- // Constructors //------------------------------------------------------------------------- internal SuppressedProperties(EncryptedPackageEnvelope envelope) { _cfioPermission.Assert(); // BlessedAssert try { _target = envelope.PackageProperties; } finally { CompoundFileIOPermission.RevertAssert(); } } #endregion Constructors #region Public Properties //-------------------------------------------------------------------------- // Public Properties //------------------------------------------------------------------------- #region SummaryInformation properties ////// The title. /// public override string Title { get { _cfioPermission.Assert(); // BlessedAssert try { return _target.Title; } finally { CompoundFileIOPermission.RevertAssert(); } } set { _cfioPermission.Assert(); // BlessedAssert try { _target.Title = value; } finally { CompoundFileIOPermission.RevertAssert(); } } } ////// The topic of the contents. /// public override string Subject { get { _cfioPermission.Assert(); // BlessedAssert try { return _target.Subject; } finally { CompoundFileIOPermission.RevertAssert(); } } set { _cfioPermission.Assert(); // BlessedAssert try { _target.Subject = value; } finally { CompoundFileIOPermission.RevertAssert(); } } } ////// The primary creator. The identification is environment-specific and /// can consist of a name, email address, employee ID, etc. It is /// recommended that this value be only as verbose as necessary to /// identify the individual. /// public override string Creator { get { _cfioPermission.Assert(); // BlessedAssert try { return _target.Creator; } finally { CompoundFileIOPermission.RevertAssert(); } } set { _cfioPermission.Assert(); // BlessedAssert try { _target.Creator = value; } finally { CompoundFileIOPermission.RevertAssert(); } } } ////// A delimited set of keywords to support searching and indexing. This /// is typically a list of terms that are not available elsewhere in the /// properties. /// public override string Keywords { get { _cfioPermission.Assert(); // BlessedAssert try { return _target.Keywords; } finally { CompoundFileIOPermission.RevertAssert(); } } set { _cfioPermission.Assert(); // BlessedAssert try { _target.Keywords = value; } finally { CompoundFileIOPermission.RevertAssert(); } } } ////// The description or abstract of the contents. /// public override string Description { get { _cfioPermission.Assert(); // BlessedAssert try { return _target.Description; } finally { CompoundFileIOPermission.RevertAssert(); } } set { _cfioPermission.Assert(); // BlessedAssert try { _target.Description = value; } finally { CompoundFileIOPermission.RevertAssert(); } } } ////// The user who performed the last modification. The identification is /// environment-specific and can consist of a name, email address, /// employee ID, etc. It is recommended that this value be only as /// verbose as necessary to identify the individual. /// public override string LastModifiedBy { get { _cfioPermission.Assert(); // BlessedAssert try { return _target.LastModifiedBy; } finally { CompoundFileIOPermission.RevertAssert(); } } set { _cfioPermission.Assert(); // BlessedAssert try { _target.LastModifiedBy = value; } finally { CompoundFileIOPermission.RevertAssert(); } } } ////// The revision number. This value indicates the number of saves or /// revisions. The application is responsible for updating this value /// after each revision. /// public override string Revision { get { _cfioPermission.Assert(); // BlessedAssert try { return _target.Revision; } finally { CompoundFileIOPermission.RevertAssert(); } } set { _cfioPermission.Assert(); // BlessedAssert try { _target.Revision = value; } finally { CompoundFileIOPermission.RevertAssert(); } } } ////// The date and time of the last printing. /// public override NullableLastPrinted { get { _cfioPermission.Assert(); // BlessedAssert try { return _target.LastPrinted; } finally { CompoundFileIOPermission.RevertAssert(); } } set { _cfioPermission.Assert(); // BlessedAssert try { _target.LastPrinted = value; } finally { CompoundFileIOPermission.RevertAssert(); } } } /// /// The creation date and time. /// public override NullableCreated { get { _cfioPermission.Assert(); // BlessedAssert try { return _target.Created; } finally { CompoundFileIOPermission.RevertAssert(); } } set { _cfioPermission.Assert(); // BlessedAssert try { _target.Created = value; } finally { CompoundFileIOPermission.RevertAssert(); } } } /// /// The date and time of the last modification. /// public override NullableModified { get { _cfioPermission.Assert(); // BlessedAssert try { return _target.Modified; } finally { CompoundFileIOPermission.RevertAssert(); } } set { _cfioPermission.Assert(); // BlessedAssert try { _target.Modified = value; } finally { CompoundFileIOPermission.RevertAssert(); } } } #endregion SummaryInformation properties #region DocumentSummaryInformation properties /// /// The category. This value is typically used by UI applications to create /// navigation controls. /// public override string Category { get { _cfioPermission.Assert(); // BlessedAssert try { return _target.Category; } finally { CompoundFileIOPermission.RevertAssert(); } } set { _cfioPermission.Assert(); // BlessedAssert try { _target.Category = value; } finally { CompoundFileIOPermission.RevertAssert(); } } } ////// A unique identifier. /// public override string Identifier { get { _cfioPermission.Assert(); // BlessedAssert try { return _target.Identifier; } finally { CompoundFileIOPermission.RevertAssert(); } } set { _cfioPermission.Assert(); // BlessedAssert try { _target.Identifier = value; } finally { CompoundFileIOPermission.RevertAssert(); } } } ////// The type of content represented, generally defined by a specific /// use and intended audience. Example values include "Whitepaper", /// "Security Bulletin", and "Exam". (This property is distinct from /// MIME content types as defined in RFC 2045.) /// public override string ContentType { get { _cfioPermission.Assert(); // BlessedAssert try { return _target.ContentType; } finally { CompoundFileIOPermission.RevertAssert(); } } set { _cfioPermission.Assert(); // BlessedAssert try { _target.ContentType = value; } finally { CompoundFileIOPermission.RevertAssert(); } } } ////// The primary language of the package content. The language tag is /// composed of one or more parts: A primary language subtag and a /// (possibly empty) series of subsequent subtags, for example, "EN-US". /// These values MUST follow the convention specified in RFC 3066. /// public override string Language { get { _cfioPermission.Assert(); // BlessedAssert try { return _target.Language; } finally { CompoundFileIOPermission.RevertAssert(); } } set { _cfioPermission.Assert(); // BlessedAssert try { _target.Language = value; } finally { CompoundFileIOPermission.RevertAssert(); } } } ////// The version number. This value is set by the user or by the application. /// public override string Version { get { _cfioPermission.Assert(); // BlessedAssert try { return _target.Version; } finally { CompoundFileIOPermission.RevertAssert(); } } set { _cfioPermission.Assert(); // BlessedAssert try { _target.Version = value; } finally { CompoundFileIOPermission.RevertAssert(); } } } ////// The status of the content. Example values include "Draft", /// "Reviewed", and "Final". /// public override string ContentStatus { get { _cfioPermission.Assert(); // BlessedAssert try { return _target.ContentStatus; } finally { CompoundFileIOPermission.RevertAssert(); } } set { _cfioPermission.Assert(); // BlessedAssert try { _target.ContentStatus = value; } finally { CompoundFileIOPermission.RevertAssert(); } } } #endregion DocumentSummaryInformation properties #endregion Public Properties #region IDisposable //-------------------------------------------------------------------------- // IDisposable Methods //-------------------------------------------------------------------------- ////// Dispose(bool disposing) executes in two distinct scenarios. /// If disposing equals true, the method has been called directly /// or indirectly by a user's code. Managed and unmanaged resources /// can be disposed. /// /// If disposing equals false, the method has been called by the /// runtime from inside the finalizer and you should not reference /// other objects. Only unmanaged resources can be disposed. /// /// This class does have unmanaged resources, namely the OLE property /// storage interface pointers. /// /// /// true if called from Dispose(); false if called from the finalizer. /// ////// Critical: /// - critical because it asserts for UMC /// TreatAsSafe: /// - target is critical data and desposing of the object is a safe /// operation /// [SecurityTreatAsSafe] protected override void Dispose( bool disposing ) { try { base.Dispose(true); } finally { _cfioPermission.Assert(); // BlessedAssert try { _target.Dispose(); } finally { CompoundFileIOPermission.RevertAssert(); } } } #endregion IDisposable private PackageProperties _target; private readonly CompoundFileIOPermission _cfioPermission = new CompoundFileIOPermission(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
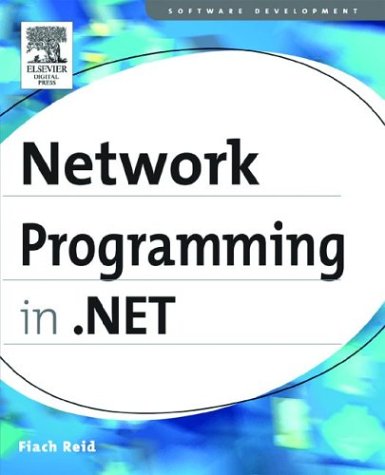
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ValidationSummary.cs
- DelimitedListTraceListener.cs
- MessageBox.cs
- DataGridViewTopLeftHeaderCell.cs
- StylusLogic.cs
- ValidatedControlConverter.cs
- ResourceExpressionBuilder.cs
- LowerCaseStringConverter.cs
- ClientFormsAuthenticationCredentials.cs
- XmlSchemaParticle.cs
- ConfigPathUtility.cs
- GridErrorDlg.cs
- ServiceReference.cs
- SHA1CryptoServiceProvider.cs
- NamedPipeAppDomainProtocolHandler.cs
- MemoryPressure.cs
- SqlParameter.cs
- NamespaceCollection.cs
- ToolStripLabel.cs
- StrongTypingException.cs
- DBDataPermission.cs
- _NestedSingleAsyncResult.cs
- SafeHandle.cs
- DataTableClearEvent.cs
- DataGridTable.cs
- ProcessInputEventArgs.cs
- ReferenceSchema.cs
- DataKeyCollection.cs
- ZoneButton.cs
- StringTraceRecord.cs
- CellTreeNode.cs
- NativeCppClassAttribute.cs
- IdentityModelStringsVersion1.cs
- HttpClientCertificate.cs
- WebConfigurationHostFileChange.cs
- MetadataSource.cs
- TableLayoutPanelDesigner.cs
- ImplicitInputBrush.cs
- SafeCancelMibChangeNotify.cs
- ProfileGroupSettings.cs
- FrameworkElementFactoryMarkupObject.cs
- ListMarkerSourceInfo.cs
- WSFederationHttpSecurity.cs
- UriTemplateVariableQueryValue.cs
- SecUtil.cs
- TypeDescriptionProvider.cs
- WaitHandle.cs
- CheckBox.cs
- ValuePatternIdentifiers.cs
- RoleManagerSection.cs
- NativeRightsManagementAPIsStructures.cs
- SemanticBasicElement.cs
- EventEntry.cs
- DBConnectionString.cs
- AsyncOperationContext.cs
- ProxySimple.cs
- TypeInitializationException.cs
- ToolZoneDesigner.cs
- DataGridItem.cs
- TileBrush.cs
- DrawingState.cs
- TreeNodeEventArgs.cs
- DescriptionCreator.cs
- SiteMembershipCondition.cs
- Point3DAnimation.cs
- ListenDesigner.cs
- DbProviderSpecificTypePropertyAttribute.cs
- ListenerElementsCollection.cs
- COM2ExtendedBrowsingHandler.cs
- isolationinterop.cs
- SqlProvider.cs
- XmlNode.cs
- NamedPipeProcessProtocolHandler.cs
- ControlAdapter.cs
- DesigntimeLicenseContextSerializer.cs
- WebRequestModuleElement.cs
- FileIOPermission.cs
- Object.cs
- Triplet.cs
- TextDecoration.cs
- CategoryGridEntry.cs
- WindowsSysHeader.cs
- Point4D.cs
- BinaryMessageFormatter.cs
- Sentence.cs
- SerializationSectionGroup.cs
- ScrollBar.cs
- HttpServerVarsCollection.cs
- ReadContentAsBinaryHelper.cs
- SoapMessage.cs
- XmlMemberMapping.cs
- X509CertificateStore.cs
- BmpBitmapDecoder.cs
- AudioFormatConverter.cs
- BulletedList.cs
- SiteMapPath.cs
- CurrentChangingEventArgs.cs
- DirectionalLight.cs
- AnimationLayer.cs
- CategoryValueConverter.cs