Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Model / ModelServiceImpl.cs / 1305376 / ModelServiceImpl.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.Presentation.Model { using System.Collections.Generic; using System.Diagnostics; using System.Activities.Presentation.Services; using System.Runtime; // This is the implementaion of the ModelService, this is published by the ModelTreeManager // on the editingContext. This is just a facade to the modelTreemanager methods. class ModelServiceImpl : ModelService { ModelTreeManager modelTreeManager; public ModelServiceImpl(ModelTreeManager modelTreeManager) { if (modelTreeManager == null) { throw FxTrace.Exception.AsError( new ArgumentNullException("modelTreeManager")); } this.modelTreeManager = modelTreeManager; } public override event EventHandlerModelChanged; public override ModelItem Root { get { return modelTreeManager.Root; } } public override IEnumerable Find(ModelItem startingItem, Predicate match) { return modelTreeManager.Find(startingItem, match, false); } public override IEnumerable Find(ModelItem startingItem, Type type) { if (startingItem == null) { throw FxTrace.Exception.AsError( new ArgumentNullException("startingItem")); } if (type == null) { throw FxTrace.Exception.AsError( new ArgumentNullException("type")); } Fx.Assert(!type.IsValueType, "hmm why would some one search for modelitems for value types?"); return modelTreeManager.Find(startingItem, delegate(Type modelItemType) { return type.IsAssignableFrom(modelItemType); }, false); } public override ModelItem FromName(ModelItem scope, string name, StringComparison comparison) { // The workflow component model does not implement a unique named activity object right now // so we cannot support this feature. throw FxTrace.Exception.AsError( new NotSupportedException()); } internal void OnModelItemAdded(ModelItem modelItem) { Fx.Assert(modelItem != null, "modelItem should not be null"); if (ModelChanged != null) { Fx.Assert(modelItem != null, "trying to add empty model item"); List modelItemsAdded = new List (1); modelItemsAdded.Add(modelItem); ModelChanged.Invoke(this, new ModelChangedEventArgsImpl(modelItemsAdded, null, null)); modelTreeManager.SyncModelAndText(); } } internal void OnModelItemRemoved(ModelItem modelItem) { Fx.Assert(modelItem != null, "modelItem should not be null"); if (ModelChanged != null) { List modelItemsRemoved = new List (1); modelItemsRemoved.Add(modelItem); ModelChanged.Invoke(this, new ModelChangedEventArgsImpl(null, modelItemsRemoved, null)); modelTreeManager.SyncModelAndText(); } } internal void OnModelItemsRemoved(IEnumerable modelItems) { Fx.Assert(modelItems != null, "modelItem should not be null"); if (ModelChanged != null) { List modelItemsRemoved = new List (); modelItemsRemoved.AddRange(modelItems); ModelChanged.Invoke(this, new ModelChangedEventArgsImpl(null, modelItemsRemoved, null)); modelTreeManager.SyncModelAndText(); } } internal void OnModelPropertyChanged(ModelProperty property) { Fx.Assert(property != null,"property cannot be null"); if (ModelChanged != null) { List propertiesChanged = new List (1); propertiesChanged.Add(property); ModelChanged.Invoke(this, new ModelChangedEventArgsImpl(null, null, propertiesChanged)); modelTreeManager.SyncModelAndText(); } } protected override ModelItem CreateItem(object instance) { return modelTreeManager.CreateModelItem(null, instance); } protected override ModelItem CreateItem(Type itemType, CreateOptions options, params object[] arguments) { Object instance = Activator.CreateInstance(itemType, arguments); return modelTreeManager.CreateModelItem(null, instance); } protected override ModelItem CreateStaticMemberItem(Type type, string memberName) { throw FxTrace.Exception.AsError( new NotSupportedException()); } internal ModelItem WrapAsModelItem(object instance) { return CreateItem(instance); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
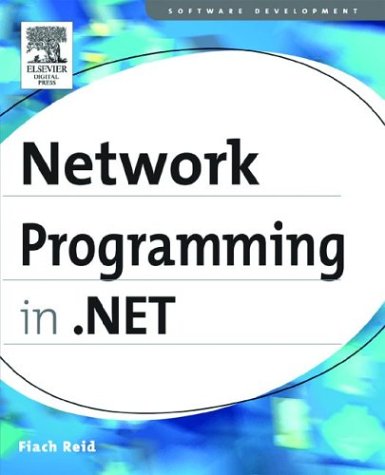
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GorillaCodec.cs
- XamlLoadErrorInfo.cs
- ReadOnlyAttribute.cs
- TemplateBindingExtensionConverter.cs
- DropDownButton.cs
- Border.cs
- BinaryFormatterWriter.cs
- ConfigurationLockCollection.cs
- XmlSequenceWriter.cs
- FunctionDescription.cs
- StringInfo.cs
- ClaimTypeElementCollection.cs
- EntityProxyFactory.cs
- XPathItem.cs
- _HelperAsyncResults.cs
- InstanceCreationEditor.cs
- CryptoStream.cs
- ToolStripMenuItem.cs
- Function.cs
- SmtpTransport.cs
- ToolStripOverflow.cs
- Lease.cs
- SystemTcpStatistics.cs
- SerializationUtilities.cs
- MatrixAnimationUsingKeyFrames.cs
- MemberAssignment.cs
- HtmlEmptyTagControlBuilder.cs
- ContextQuery.cs
- PauseStoryboard.cs
- CipherData.cs
- PackageRelationship.cs
- XmlChildEnumerator.cs
- userdatakeys.cs
- IssuedTokenServiceCredential.cs
- bindurihelper.cs
- DragDrop.cs
- PackWebResponse.cs
- SmtpReplyReaderFactory.cs
- RetriableClipboard.cs
- WSFederationHttpBinding.cs
- ObjectHelper.cs
- AddInAdapter.cs
- Exception.cs
- UnmanagedMemoryStreamWrapper.cs
- SettingsAttributes.cs
- MediaScriptCommandRoutedEventArgs.cs
- Message.cs
- PerformanceCounterPermission.cs
- DrawingContextWalker.cs
- TargetException.cs
- CompositeFontParser.cs
- LinkLabelLinkClickedEvent.cs
- XmlDictionaryString.cs
- CodeGenerator.cs
- Pen.cs
- TextServicesManager.cs
- PropertyChangedEventArgs.cs
- MetabaseReader.cs
- RegisterResponseInfo.cs
- OuterGlowBitmapEffect.cs
- XamlBuildTaskServices.cs
- HtmlWindow.cs
- OrCondition.cs
- AttributeData.cs
- ProcessRequestArgs.cs
- DecimalStorage.cs
- WeakHashtable.cs
- SystemBrushes.cs
- _CookieModule.cs
- QueryStringHandler.cs
- XmlTypeMapping.cs
- ValidationPropertyAttribute.cs
- QuaternionRotation3D.cs
- SqlDuplicator.cs
- ExpandSegment.cs
- HwndSourceParameters.cs
- InfoCardSymmetricCrypto.cs
- GeneralTransformCollection.cs
- TextEndOfSegment.cs
- XmlIlGenerator.cs
- PerfService.cs
- TextViewBase.cs
- RenderDataDrawingContext.cs
- XmlQualifiedName.cs
- SpellCheck.cs
- Roles.cs
- ValueExpressions.cs
- OperationInfo.cs
- TextDocumentView.cs
- CommandDevice.cs
- ConfigXmlReader.cs
- FtpWebResponse.cs
- ColorBlend.cs
- InvalidDataContractException.cs
- ThemeableAttribute.cs
- System.Data_BID.cs
- State.cs
- CompilerWrapper.cs
- WizardDesigner.cs
- ProcessManager.cs