Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridViewElement.cs / 1305376 / DataGridViewElement.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Diagnostics; using System.ComponentModel; using System.Diagnostics.CodeAnalysis; ////// /// public class DataGridViewElement { private DataGridViewElementStates state; // enabled frozen readOnly resizable selected visible private DataGridView dataGridView; ///Identifies an element in the dataGridView (base class for TCell, TBand, TRow, TColumn. ////// /// public DataGridViewElement() { this.state = DataGridViewElementStates.Visible; } internal DataGridViewElement(DataGridViewElement dgveTemplate) { // Selected and Displayed states are not inherited this.state = dgveTemplate.State & (DataGridViewElementStates.Frozen | DataGridViewElementStates.ReadOnly | DataGridViewElementStates.Resizable | DataGridViewElementStates.ResizableSet | DataGridViewElementStates.Visible); } ////// Initializes a new instance of the ///class. /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Advanced) ] public virtual DataGridViewElementStates State { get { return this.state; } } internal DataGridViewElementStates StateInternal { set { this.state = value; } } internal bool StateIncludes(DataGridViewElementStates elementState) { return (this.State & elementState) == elementState; } internal bool StateExcludes(DataGridViewElementStates elementState) { return (this.State & elementState) == 0; } /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public DataGridView DataGridView { get { return this.dataGridView; } } internal DataGridView DataGridViewInternal { set { if (this.DataGridView != value) { this.dataGridView = value; OnDataGridViewChanged(); } } } /// protected virtual void OnDataGridViewChanged() { } /// [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] // Method raises an event for the grid control protected void RaiseCellClick(DataGridViewCellEventArgs e) { if (this.dataGridView != null) { this.dataGridView.OnCellClickInternal(e); } } /// [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] // Method raises an event for the grid control protected void RaiseCellContentClick(DataGridViewCellEventArgs e) { if (this.dataGridView != null) { this.dataGridView.OnCellContentClickInternal(e); } } /// [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] // Method raises an event for the grid control protected void RaiseCellContentDoubleClick(DataGridViewCellEventArgs e) { if (this.dataGridView != null) { this.dataGridView.OnCellContentDoubleClickInternal(e); } } /// [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] // Method raises an event for the grid control protected void RaiseCellValueChanged(DataGridViewCellEventArgs e) { if (this.dataGridView != null) { this.dataGridView.OnCellValueChangedInternal(e); } } /// [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] // Method raises an event for the grid control protected void RaiseDataError(DataGridViewDataErrorEventArgs e) { if (this.dataGridView != null) { this.dataGridView.OnDataErrorInternal(e); } } /// [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] // Method raises an event for the grid control protected void RaiseMouseWheel(MouseEventArgs e) { if (this.dataGridView != null) { this.dataGridView.OnMouseWheelInternal(e); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Diagnostics; using System.ComponentModel; using System.Diagnostics.CodeAnalysis; ////// /// public class DataGridViewElement { private DataGridViewElementStates state; // enabled frozen readOnly resizable selected visible private DataGridView dataGridView; ///Identifies an element in the dataGridView (base class for TCell, TBand, TRow, TColumn. ////// /// public DataGridViewElement() { this.state = DataGridViewElementStates.Visible; } internal DataGridViewElement(DataGridViewElement dgveTemplate) { // Selected and Displayed states are not inherited this.state = dgveTemplate.State & (DataGridViewElementStates.Frozen | DataGridViewElementStates.ReadOnly | DataGridViewElementStates.Resizable | DataGridViewElementStates.ResizableSet | DataGridViewElementStates.Visible); } ////// Initializes a new instance of the ///class. /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Advanced) ] public virtual DataGridViewElementStates State { get { return this.state; } } internal DataGridViewElementStates StateInternal { set { this.state = value; } } internal bool StateIncludes(DataGridViewElementStates elementState) { return (this.State & elementState) == elementState; } internal bool StateExcludes(DataGridViewElementStates elementState) { return (this.State & elementState) == 0; } /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public DataGridView DataGridView { get { return this.dataGridView; } } internal DataGridView DataGridViewInternal { set { if (this.DataGridView != value) { this.dataGridView = value; OnDataGridViewChanged(); } } } /// protected virtual void OnDataGridViewChanged() { } /// [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] // Method raises an event for the grid control protected void RaiseCellClick(DataGridViewCellEventArgs e) { if (this.dataGridView != null) { this.dataGridView.OnCellClickInternal(e); } } /// [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] // Method raises an event for the grid control protected void RaiseCellContentClick(DataGridViewCellEventArgs e) { if (this.dataGridView != null) { this.dataGridView.OnCellContentClickInternal(e); } } /// [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] // Method raises an event for the grid control protected void RaiseCellContentDoubleClick(DataGridViewCellEventArgs e) { if (this.dataGridView != null) { this.dataGridView.OnCellContentDoubleClickInternal(e); } } /// [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] // Method raises an event for the grid control protected void RaiseCellValueChanged(DataGridViewCellEventArgs e) { if (this.dataGridView != null) { this.dataGridView.OnCellValueChangedInternal(e); } } /// [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] // Method raises an event for the grid control protected void RaiseDataError(DataGridViewDataErrorEventArgs e) { if (this.dataGridView != null) { this.dataGridView.OnDataErrorInternal(e); } } /// [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] // Method raises an event for the grid control protected void RaiseMouseWheel(MouseEventArgs e) { if (this.dataGridView != null) { this.dataGridView.OnMouseWheelInternal(e); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
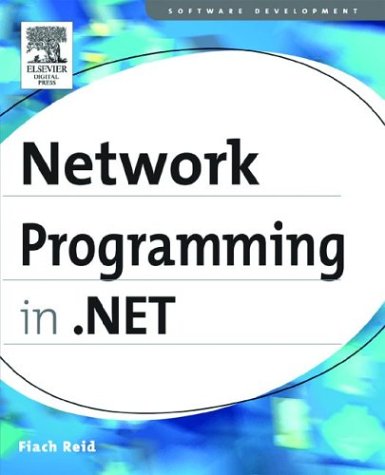
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FloaterParaClient.cs
- XmlStringTable.cs
- _SslSessionsCache.cs
- PageStatePersister.cs
- SQLString.cs
- DataAdapter.cs
- BaseEntityWrapper.cs
- XmlWriterTraceListener.cs
- SafeProcessHandle.cs
- FormsAuthenticationUserCollection.cs
- TransformerTypeCollection.cs
- DataControlField.cs
- EtwProvider.cs
- CompiledScopeCriteria.cs
- ListViewInsertedEventArgs.cs
- JsonFormatGeneratorStatics.cs
- DuplexChannelBinder.cs
- XmlArrayItemAttributes.cs
- WindowsMenu.cs
- AutoScrollHelper.cs
- ObjectAnimationBase.cs
- SourceElementsCollection.cs
- HostProtectionException.cs
- TileModeValidation.cs
- WindowsFormsHost.cs
- Trace.cs
- RoleManagerSection.cs
- SignerInfo.cs
- MenuRendererStandards.cs
- DocumentPage.cs
- NotFiniteNumberException.cs
- XmlWellformedWriter.cs
- BezierSegment.cs
- HandleInitializationContext.cs
- TextSelectionHelper.cs
- TiffBitmapDecoder.cs
- Object.cs
- RectAnimationUsingKeyFrames.cs
- ResourceReferenceExpression.cs
- Pkcs7Recipient.cs
- ToggleProviderWrapper.cs
- ConsoleEntryPoint.cs
- ColumnTypeConverter.cs
- TextServicesContext.cs
- ContentFileHelper.cs
- CodeCompileUnit.cs
- XmlPreloadedResolver.cs
- FontStretchConverter.cs
- AttachedPropertyBrowsableWhenAttributePresentAttribute.cs
- TextRenderer.cs
- CodeThrowExceptionStatement.cs
- SplineKeyFrames.cs
- GeneralTransform2DTo3DTo2D.cs
- Style.cs
- OneOfTypeConst.cs
- Oid.cs
- EntityDesignerBuildProvider.cs
- ExpressionBindingCollection.cs
- ConfigsHelper.cs
- VirtualizedContainerService.cs
- IsolationInterop.cs
- PartialCachingAttribute.cs
- ExpressionBinding.cs
- XmlObjectSerializerWriteContextComplexJson.cs
- DbModificationCommandTree.cs
- TextBox.cs
- MulticastIPAddressInformationCollection.cs
- ConnectionModeReader.cs
- ItemContainerGenerator.cs
- TimerTable.cs
- RuntimeArgumentHandle.cs
- ColumnPropertiesGroup.cs
- RegistryConfigurationProvider.cs
- LongValidator.cs
- TemplatedWizardStep.cs
- X509Certificate2Collection.cs
- GroupBox.cs
- FormConverter.cs
- ACL.cs
- StringCollectionMarkupSerializer.cs
- CodeSubDirectoriesCollection.cs
- AuthenticodeSignatureInformation.cs
- FixedTextSelectionProcessor.cs
- ListMarkerLine.cs
- InternalConfigRoot.cs
- HtmlGenericControl.cs
- QueryParameter.cs
- XmlSerializationReader.cs
- RuntimeConfigLKG.cs
- LicenseManager.cs
- _NegoState.cs
- Adorner.cs
- PathTooLongException.cs
- Wildcard.cs
- AddDataControlFieldDialog.cs
- ProcessModelInfo.cs
- SerialPort.cs
- GeneralTransformGroup.cs
- SByte.cs
- SchemaImporter.cs