Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Net / System / Net / Mail / smtppermission.cs / 1305376 / smtppermission.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net.Mail { using System.Collections; using System.Security; using System.Security.Permissions; using System.Globalization; using System.Threading; ///public enum SmtpAccess { None = 0, Connect = 1, ConnectToUnrestrictedPort = 2}; /// [ AttributeUsage( AttributeTargets.Method | AttributeTargets.Constructor | AttributeTargets.Class | AttributeTargets.Struct | AttributeTargets.Assembly, AllowMultiple = true, Inherited = false )] [Serializable] public sealed class SmtpPermissionAttribute: CodeAccessSecurityAttribute { private const string strAccess = "Access"; private string access = null; /// public SmtpPermissionAttribute(SecurityAction action): base( action ) { } public string Access { get{ return access; } set{ access = value; } } /// public override IPermission CreatePermission() { SmtpPermission perm = null; if (Unrestricted) { perm = new SmtpPermission(PermissionState.Unrestricted); } else { perm = new SmtpPermission(PermissionState.None); if (access != null) { if (0 == string.Compare(access, "Connect", StringComparison.OrdinalIgnoreCase)) { perm.AddPermission(SmtpAccess.Connect); } else if (0 == string.Compare(access, "ConnectToUnrestrictedPort", StringComparison.OrdinalIgnoreCase)) { perm.AddPermission(SmtpAccess.ConnectToUnrestrictedPort); } else if (0 == string.Compare(access, "None", StringComparison.OrdinalIgnoreCase)) { perm.AddPermission(SmtpAccess.None); } else { throw new ArgumentException(SR.GetString(SR.net_perm_invalid_val, strAccess, access)); } } } return perm; } } /// [Serializable] public sealed class SmtpPermission: CodeAccessPermission, IUnrestrictedPermission { SmtpAccess access; private bool unrestricted; /// public SmtpPermission(PermissionState state) { if (state == PermissionState.Unrestricted){ access = SmtpAccess.ConnectToUnrestrictedPort; unrestricted = true; } else{ access = SmtpAccess.None; } } public SmtpPermission(bool unrestricted) { if (unrestricted){ access = SmtpAccess.ConnectToUnrestrictedPort; this.unrestricted = true; } else{ access = SmtpAccess.None; } } /// public SmtpPermission(SmtpAccess access) { this.access = access; } public SmtpAccess Access { get{ return access; } } /// public void AddPermission(SmtpAccess access) { if (access > this.access) this.access = access; } /// public bool IsUnrestricted() { return unrestricted; } /// public override IPermission Copy() { if(unrestricted){ return new SmtpPermission(true); } return new SmtpPermission(access); } /// public override IPermission Union(IPermission target) { if (target==null) { return this.Copy(); } SmtpPermission other = target as SmtpPermission; if(other == null) { throw new ArgumentException(SR.GetString(SR.net_perm_target), "target"); } if(unrestricted || other.IsUnrestricted()){ return new SmtpPermission(true); } return new SmtpPermission(this.access > other.access ? this.access : other.access); } /// public override IPermission Intersect(IPermission target) { if (target == null) { return null; } SmtpPermission other = target as SmtpPermission; if(other == null) { throw new ArgumentException(SR.GetString(SR.net_perm_target), "target"); } if(IsUnrestricted() && other.IsUnrestricted()){ return new SmtpPermission(true); } return new SmtpPermission(this.access < other.access ? this.access : other.access); } /// public override bool IsSubsetOf(IPermission target) { // Pattern suggested by security engine if (target == null) { return (access == SmtpAccess.None); } SmtpPermission other = target as SmtpPermission; if (other == null) { throw new ArgumentException(SR.GetString(SR.net_perm_target), "target"); } if(unrestricted && !other.IsUnrestricted()){ return false; } return (other.access >= access); } /// public override void FromXml(SecurityElement securityElement) { if (securityElement == null) { throw new ArgumentNullException("securityElement"); } if (!securityElement.Tag.Equals("IPermission")) { throw new ArgumentException(SR.GetString(SR.net_not_ipermission), "securityElement"); } string className = securityElement.Attribute("class"); if (className == null) { throw new ArgumentException(SR.GetString(SR.net_no_classname), "securityElement"); } if (className.IndexOf(this.GetType().FullName) < 0) { throw new ArgumentException(SR.GetString(SR.net_no_typename), "securityElement"); } String str = securityElement.Attribute("Unrestricted"); if (str != null) { if (0 == string.Compare( str, "true", StringComparison.OrdinalIgnoreCase)){ access = SmtpAccess.ConnectToUnrestrictedPort; unrestricted = true; return; } } str = securityElement.Attribute("Access"); if (str != null) { if(0 == string.Compare(str, "Connect", StringComparison.OrdinalIgnoreCase)){ access = SmtpAccess.Connect; } else if(0 == string.Compare(str, "ConnectToUnrestrictedPort", StringComparison.OrdinalIgnoreCase)){ access = SmtpAccess.ConnectToUnrestrictedPort; } else if(0 == string.Compare(str, "None", StringComparison.OrdinalIgnoreCase)){ access = SmtpAccess.None; } else{ throw new ArgumentException(SR.GetString(SR.net_perm_invalid_val_in_element), "Access"); } } } /// public override SecurityElement ToXml() { SecurityElement securityElement = new SecurityElement( "IPermission" ); securityElement.AddAttribute("class", this.GetType().FullName + ", " + this.GetType().Module.Assembly.FullName.Replace( '\"', '\'' )); securityElement.AddAttribute("version", "1"); if(unrestricted){ securityElement.AddAttribute("Unrestricted", "true"); return securityElement; } if (access == SmtpAccess.Connect) { securityElement.AddAttribute("Access", "Connect"); } else if (access == SmtpAccess.ConnectToUnrestrictedPort) { securityElement.AddAttribute("Access", "ConnectToUnrestrictedPort"); } return securityElement; } }// class SmtpPermission } // namespace System.Net // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net.Mail { using System.Collections; using System.Security; using System.Security.Permissions; using System.Globalization; using System.Threading; ///public enum SmtpAccess { None = 0, Connect = 1, ConnectToUnrestrictedPort = 2}; /// [ AttributeUsage( AttributeTargets.Method | AttributeTargets.Constructor | AttributeTargets.Class | AttributeTargets.Struct | AttributeTargets.Assembly, AllowMultiple = true, Inherited = false )] [Serializable] public sealed class SmtpPermissionAttribute: CodeAccessSecurityAttribute { private const string strAccess = "Access"; private string access = null; /// public SmtpPermissionAttribute(SecurityAction action): base( action ) { } public string Access { get{ return access; } set{ access = value; } } /// public override IPermission CreatePermission() { SmtpPermission perm = null; if (Unrestricted) { perm = new SmtpPermission(PermissionState.Unrestricted); } else { perm = new SmtpPermission(PermissionState.None); if (access != null) { if (0 == string.Compare(access, "Connect", StringComparison.OrdinalIgnoreCase)) { perm.AddPermission(SmtpAccess.Connect); } else if (0 == string.Compare(access, "ConnectToUnrestrictedPort", StringComparison.OrdinalIgnoreCase)) { perm.AddPermission(SmtpAccess.ConnectToUnrestrictedPort); } else if (0 == string.Compare(access, "None", StringComparison.OrdinalIgnoreCase)) { perm.AddPermission(SmtpAccess.None); } else { throw new ArgumentException(SR.GetString(SR.net_perm_invalid_val, strAccess, access)); } } } return perm; } } /// [Serializable] public sealed class SmtpPermission: CodeAccessPermission, IUnrestrictedPermission { SmtpAccess access; private bool unrestricted; /// public SmtpPermission(PermissionState state) { if (state == PermissionState.Unrestricted){ access = SmtpAccess.ConnectToUnrestrictedPort; unrestricted = true; } else{ access = SmtpAccess.None; } } public SmtpPermission(bool unrestricted) { if (unrestricted){ access = SmtpAccess.ConnectToUnrestrictedPort; this.unrestricted = true; } else{ access = SmtpAccess.None; } } /// public SmtpPermission(SmtpAccess access) { this.access = access; } public SmtpAccess Access { get{ return access; } } /// public void AddPermission(SmtpAccess access) { if (access > this.access) this.access = access; } /// public bool IsUnrestricted() { return unrestricted; } /// public override IPermission Copy() { if(unrestricted){ return new SmtpPermission(true); } return new SmtpPermission(access); } /// public override IPermission Union(IPermission target) { if (target==null) { return this.Copy(); } SmtpPermission other = target as SmtpPermission; if(other == null) { throw new ArgumentException(SR.GetString(SR.net_perm_target), "target"); } if(unrestricted || other.IsUnrestricted()){ return new SmtpPermission(true); } return new SmtpPermission(this.access > other.access ? this.access : other.access); } /// public override IPermission Intersect(IPermission target) { if (target == null) { return null; } SmtpPermission other = target as SmtpPermission; if(other == null) { throw new ArgumentException(SR.GetString(SR.net_perm_target), "target"); } if(IsUnrestricted() && other.IsUnrestricted()){ return new SmtpPermission(true); } return new SmtpPermission(this.access < other.access ? this.access : other.access); } /// public override bool IsSubsetOf(IPermission target) { // Pattern suggested by security engine if (target == null) { return (access == SmtpAccess.None); } SmtpPermission other = target as SmtpPermission; if (other == null) { throw new ArgumentException(SR.GetString(SR.net_perm_target), "target"); } if(unrestricted && !other.IsUnrestricted()){ return false; } return (other.access >= access); } /// public override void FromXml(SecurityElement securityElement) { if (securityElement == null) { throw new ArgumentNullException("securityElement"); } if (!securityElement.Tag.Equals("IPermission")) { throw new ArgumentException(SR.GetString(SR.net_not_ipermission), "securityElement"); } string className = securityElement.Attribute("class"); if (className == null) { throw new ArgumentException(SR.GetString(SR.net_no_classname), "securityElement"); } if (className.IndexOf(this.GetType().FullName) < 0) { throw new ArgumentException(SR.GetString(SR.net_no_typename), "securityElement"); } String str = securityElement.Attribute("Unrestricted"); if (str != null) { if (0 == string.Compare( str, "true", StringComparison.OrdinalIgnoreCase)){ access = SmtpAccess.ConnectToUnrestrictedPort; unrestricted = true; return; } } str = securityElement.Attribute("Access"); if (str != null) { if(0 == string.Compare(str, "Connect", StringComparison.OrdinalIgnoreCase)){ access = SmtpAccess.Connect; } else if(0 == string.Compare(str, "ConnectToUnrestrictedPort", StringComparison.OrdinalIgnoreCase)){ access = SmtpAccess.ConnectToUnrestrictedPort; } else if(0 == string.Compare(str, "None", StringComparison.OrdinalIgnoreCase)){ access = SmtpAccess.None; } else{ throw new ArgumentException(SR.GetString(SR.net_perm_invalid_val_in_element), "Access"); } } } /// public override SecurityElement ToXml() { SecurityElement securityElement = new SecurityElement( "IPermission" ); securityElement.AddAttribute("class", this.GetType().FullName + ", " + this.GetType().Module.Assembly.FullName.Replace( '\"', '\'' )); securityElement.AddAttribute("version", "1"); if(unrestricted){ securityElement.AddAttribute("Unrestricted", "true"); return securityElement; } if (access == SmtpAccess.Connect) { securityElement.AddAttribute("Access", "Connect"); } else if (access == SmtpAccess.ConnectToUnrestrictedPort) { securityElement.AddAttribute("Access", "ConnectToUnrestrictedPort"); } return securityElement; } }// class SmtpPermission } // namespace System.Net // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
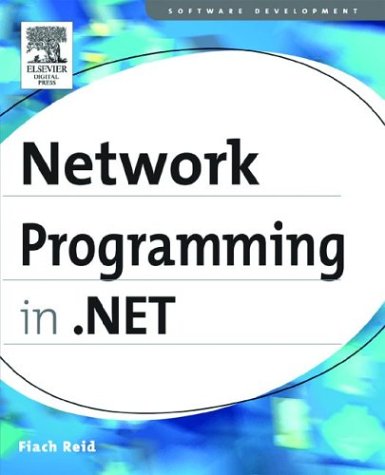
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SymDocumentType.cs
- SplashScreenNativeMethods.cs
- PropertyPathWorker.cs
- NavigationCommands.cs
- Property.cs
- DataTemplateKey.cs
- SubqueryRules.cs
- PartitionResolver.cs
- ContextInformation.cs
- EntityDataSourceWrapper.cs
- XmlUtil.cs
- DataBoundControl.cs
- WhitespaceSignificantCollectionAttribute.cs
- StrongNameKeyPair.cs
- GeneralTransform2DTo3DTo2D.cs
- XPathDocument.cs
- StatusBarPanelClickEvent.cs
- DispatcherHooks.cs
- HttpContext.cs
- ConditionCollection.cs
- DeploymentSection.cs
- Array.cs
- DataBoundControlHelper.cs
- _BufferOffsetSize.cs
- ListViewHitTestInfo.cs
- XamlReaderConstants.cs
- NumericUpDownAccelerationCollection.cs
- ScriptMethodAttribute.cs
- SymmetricSecurityProtocol.cs
- SByteStorage.cs
- DesignerCommandAdapter.cs
- MenuItem.cs
- UiaCoreTypesApi.cs
- SettingsBase.cs
- PinnedBufferMemoryStream.cs
- datacache.cs
- WebPart.cs
- HttpCookiesSection.cs
- SHA1.cs
- ThrowOnMultipleAssignment.cs
- DataGridLinkButton.cs
- LayoutEditorPart.cs
- PrePostDescendentsWalker.cs
- NativeRecognizer.cs
- RemoveStoryboard.cs
- glyphs.cs
- SymLanguageVendor.cs
- QuaternionConverter.cs
- NameValueSectionHandler.cs
- DisplayMemberTemplateSelector.cs
- DefaultPropertiesToSend.cs
- QuaternionRotation3D.cs
- NonSerializedAttribute.cs
- AsyncDataRequest.cs
- DataGridViewRowCancelEventArgs.cs
- SyndicationItemFormatter.cs
- DataView.cs
- AssemblyAttributes.cs
- ExceptionUtil.cs
- IODescriptionAttribute.cs
- BulletDecorator.cs
- AsyncSerializedWorker.cs
- DataGridViewAddColumnDialog.cs
- PeerApplication.cs
- ReadOnlyHierarchicalDataSource.cs
- XPathNodeInfoAtom.cs
- ToolboxDataAttribute.cs
- LOSFormatter.cs
- CodeParameterDeclarationExpression.cs
- PathGradientBrush.cs
- RelationshipManager.cs
- ToolStripDropTargetManager.cs
- OpenTypeMethods.cs
- QilLiteral.cs
- TableLayoutStyleCollection.cs
- MultiBindingExpression.cs
- XsltLoader.cs
- RelationshipDetailsRow.cs
- DataGridViewTextBoxColumn.cs
- BezierSegment.cs
- LinqTreeNodeEvaluator.cs
- Point.cs
- DocumentCollection.cs
- PointLightBase.cs
- ImplicitInputBrush.cs
- TreeNode.cs
- XmlTextEncoder.cs
- FrameworkElementAutomationPeer.cs
- AppearanceEditorPart.cs
- Debugger.cs
- XmlWellformedWriter.cs
- Color.cs
- CachingParameterInspector.cs
- CodeCompileUnit.cs
- XmlNavigatorStack.cs
- MutexSecurity.cs
- DesignerView.Commands.cs
- IISUnsafeMethods.cs
- QueryCursorEventArgs.cs
- FixedBufferAttribute.cs