Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / ControlCachePolicy.cs / 1305376 / ControlCachePolicy.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.IO; using System.Text; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design; using System.Globalization; using System.Web; using System.Web.Util; using System.Web.UI.WebControls; using System.Web.Caching; using System.Security.Permissions; public sealed class ControlCachePolicy { private static ControlCachePolicy _cachePolicyStub = new ControlCachePolicy(); private BasePartialCachingControl _pcc; internal ControlCachePolicy() { } internal ControlCachePolicy(BasePartialCachingControl pcc) { _pcc = pcc; } internal static ControlCachePolicy GetCachePolicyStub() { // Return a stub, which returns SupportsCaching==false and throws on everything else. return _cachePolicyStub; } // Check whether it is valid to access properties on this object private void CheckValidCallingContext() { // If it's not being cached, the CachePolicy can't be used if (_pcc == null) { throw new HttpException( SR.GetString(SR.UC_not_cached)); } // Make sure it's not being used too late if (_pcc.ControlState >= ControlState.PreRendered) { throw new HttpException( SR.GetString(SR.UCCachePolicy_unavailable)); } } public bool SupportsCaching { get { // Caching is supported if we have a PartialCachingControl return (_pcc != null); } } public bool Cached { get { CheckValidCallingContext(); return !_pcc._cachingDisabled; } set { CheckValidCallingContext(); _pcc._cachingDisabled = !value; } } public TimeSpan Duration { get { CheckValidCallingContext(); return _pcc.Duration; } set { CheckValidCallingContext(); _pcc.Duration = value; } } public HttpCacheVaryByParams VaryByParams { get { CheckValidCallingContext(); return _pcc.VaryByParams; } } public string VaryByControl { get { CheckValidCallingContext(); return _pcc.VaryByControl; } set { CheckValidCallingContext(); _pcc.VaryByControl = value; } } public CacheDependency Dependency { get { CheckValidCallingContext(); return _pcc.Dependency; } set { CheckValidCallingContext(); _pcc.Dependency = value; } } public void SetVaryByCustom(string varyByCustom) { CheckValidCallingContext(); _pcc._varyByCustom = varyByCustom; } public void SetSlidingExpiration(bool useSlidingExpiration) { CheckValidCallingContext(); _pcc._useSlidingExpiration = useSlidingExpiration; } public void SetExpires(DateTime expirationTime) { CheckValidCallingContext(); _pcc._utcExpirationTime = DateTimeUtil.ConvertToUniversalTime(expirationTime); } public String ProviderName { get { CheckValidCallingContext(); if (_pcc._provider == null) { return OutputCache.ASPNET_INTERNAL_PROVIDER_NAME; } else { return _pcc._provider; } } set { CheckValidCallingContext(); if (value == OutputCache.ASPNET_INTERNAL_PROVIDER_NAME) { value = null; } OutputCache.ThrowIfProviderNotFound(value); _pcc._provider = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.IO; using System.Text; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design; using System.Globalization; using System.Web; using System.Web.Util; using System.Web.UI.WebControls; using System.Web.Caching; using System.Security.Permissions; public sealed class ControlCachePolicy { private static ControlCachePolicy _cachePolicyStub = new ControlCachePolicy(); private BasePartialCachingControl _pcc; internal ControlCachePolicy() { } internal ControlCachePolicy(BasePartialCachingControl pcc) { _pcc = pcc; } internal static ControlCachePolicy GetCachePolicyStub() { // Return a stub, which returns SupportsCaching==false and throws on everything else. return _cachePolicyStub; } // Check whether it is valid to access properties on this object private void CheckValidCallingContext() { // If it's not being cached, the CachePolicy can't be used if (_pcc == null) { throw new HttpException( SR.GetString(SR.UC_not_cached)); } // Make sure it's not being used too late if (_pcc.ControlState >= ControlState.PreRendered) { throw new HttpException( SR.GetString(SR.UCCachePolicy_unavailable)); } } public bool SupportsCaching { get { // Caching is supported if we have a PartialCachingControl return (_pcc != null); } } public bool Cached { get { CheckValidCallingContext(); return !_pcc._cachingDisabled; } set { CheckValidCallingContext(); _pcc._cachingDisabled = !value; } } public TimeSpan Duration { get { CheckValidCallingContext(); return _pcc.Duration; } set { CheckValidCallingContext(); _pcc.Duration = value; } } public HttpCacheVaryByParams VaryByParams { get { CheckValidCallingContext(); return _pcc.VaryByParams; } } public string VaryByControl { get { CheckValidCallingContext(); return _pcc.VaryByControl; } set { CheckValidCallingContext(); _pcc.VaryByControl = value; } } public CacheDependency Dependency { get { CheckValidCallingContext(); return _pcc.Dependency; } set { CheckValidCallingContext(); _pcc.Dependency = value; } } public void SetVaryByCustom(string varyByCustom) { CheckValidCallingContext(); _pcc._varyByCustom = varyByCustom; } public void SetSlidingExpiration(bool useSlidingExpiration) { CheckValidCallingContext(); _pcc._useSlidingExpiration = useSlidingExpiration; } public void SetExpires(DateTime expirationTime) { CheckValidCallingContext(); _pcc._utcExpirationTime = DateTimeUtil.ConvertToUniversalTime(expirationTime); } public String ProviderName { get { CheckValidCallingContext(); if (_pcc._provider == null) { return OutputCache.ASPNET_INTERNAL_PROVIDER_NAME; } else { return _pcc._provider; } } set { CheckValidCallingContext(); if (value == OutputCache.ASPNET_INTERNAL_PROVIDER_NAME) { value = null; } OutputCache.ThrowIfProviderNotFound(value); _pcc._provider = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
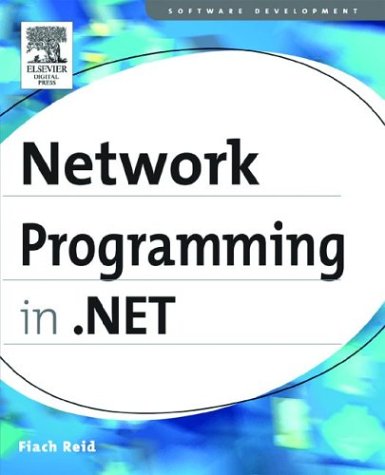
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UrlMappingCollection.cs
- DatagridviewDisplayedBandsData.cs
- BindingMemberInfo.cs
- AnnotationHelper.cs
- GZipDecoder.cs
- CustomActivityDesigner.cs
- CompModHelpers.cs
- Point3DAnimation.cs
- ContainsSearchOperator.cs
- GlobalEventManager.cs
- RightsManagementEncryptionTransform.cs
- AppDomainProtocolHandler.cs
- RawAppCommandInputReport.cs
- ExportOptions.cs
- CustomCredentialPolicy.cs
- DispatcherObject.cs
- Section.cs
- TableLayoutStyleCollection.cs
- PageCanvasSize.cs
- SchemaTableColumn.cs
- CacheSection.cs
- ControlEvent.cs
- LinqDataSourceUpdateEventArgs.cs
- SQLMembershipProvider.cs
- AxisAngleRotation3D.cs
- CompilerHelpers.cs
- NetworkAddressChange.cs
- HtmlForm.cs
- InsufficientMemoryException.cs
- CompiledELinqQueryState.cs
- OleDbSchemaGuid.cs
- MarkerProperties.cs
- AssemblyBuilder.cs
- ContentType.cs
- IdnMapping.cs
- DataServiceRequestException.cs
- OdbcConnectionString.cs
- SystemIcons.cs
- SqlIdentifier.cs
- ButtonChrome.cs
- PersonalizationStateQuery.cs
- TextDpi.cs
- CallbackTimeoutsBehavior.cs
- Brush.cs
- ShutDownListener.cs
- MsmqException.cs
- ScriptManagerProxy.cs
- HtmlInputPassword.cs
- ToolStripRendererSwitcher.cs
- XmlEntity.cs
- Rect.cs
- PersonalizableAttribute.cs
- XamlStackWriter.cs
- Encoder.cs
- BitmapSourceSafeMILHandle.cs
- TransportDefaults.cs
- PropertyItem.cs
- RegexBoyerMoore.cs
- PackagePart.cs
- UnauthorizedWebPart.cs
- MethodCallConverter.cs
- UdpChannelListener.cs
- TypeGenericEnumerableViewSchema.cs
- Rule.cs
- FormatterConverter.cs
- Button.cs
- BooleanConverter.cs
- DataViewManagerListItemTypeDescriptor.cs
- TypeListConverter.cs
- ConnectionStringsExpressionBuilder.cs
- LambdaCompiler.ControlFlow.cs
- _OverlappedAsyncResult.cs
- CustomPopupPlacement.cs
- HostingEnvironmentException.cs
- RemotingClientProxy.cs
- GenericTextProperties.cs
- Selection.cs
- SpecularMaterial.cs
- TrustManager.cs
- NamespaceEmitter.cs
- FormViewModeEventArgs.cs
- XmlNotation.cs
- PropertyMetadata.cs
- SendSecurityHeader.cs
- ClientTarget.cs
- XmlTextReader.cs
- WebConfigurationHostFileChange.cs
- ImplicitInputBrush.cs
- ApplicationFileCodeDomTreeGenerator.cs
- BooleanToVisibilityConverter.cs
- ResourceFallbackManager.cs
- PeerNameRecordCollection.cs
- Terminate.cs
- QilStrConcatenator.cs
- InfoCardRSAPKCS1SignatureFormatter.cs
- PageSettings.cs
- StreamHelper.cs
- OleDbParameter.cs
- AmbientProperties.cs
- GroupBoxAutomationPeer.cs