Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Description / ServiceDescription.cs / 1 / ServiceDescription.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Description { using System.Collections.Generic; using System.ServiceModel; using System.ServiceModel.Activation; using System.Collections.ObjectModel; using System.Runtime.Serialization; using System.Reflection; using System.Diagnostics; using System.Security; using System.Security.Permissions; [DebuggerDisplay("ServiceType={serviceType}")] public class ServiceDescription { KeyedByTypeCollectionbehaviors = new KeyedByTypeCollection (); string configurationName; ServiceEndpointCollection endpoints = new ServiceEndpointCollection(); Type serviceType; XmlName serviceName; string serviceNamespace = NamingHelper.DefaultNamespace; public ServiceDescription() { } internal ServiceDescription(String serviceName) { if (String.IsNullOrEmpty(serviceName)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("serviceName"); this.Name = serviceName; } public ServiceDescription(IEnumerable endpoints) : this() { if (endpoints == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("endpoints"); foreach (ServiceEndpoint endpoint in endpoints) this.endpoints.Add(endpoint); } public string Name { get { if (serviceName != null) return serviceName.EncodedName; else if (ServiceType != null) return NamingHelper.XmlName(ServiceType.Name); else return NamingHelper.DefaultServiceName; } set { if (string.IsNullOrEmpty(value)) { serviceName = null; } else { // the XmlName ctor validate the value serviceName = new XmlName(value, true /*isEncoded*/); } } } public string Namespace { get { return serviceNamespace; } set { serviceNamespace = value; } } public KeyedByTypeCollection Behaviors { get { return this.behaviors; } } public string ConfigurationName { get { return this.configurationName; } set { if (value == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("value"); } this.configurationName = value; } } public ServiceEndpointCollection Endpoints { get { return this.endpoints; } } public Type ServiceType { get { return this.serviceType; } set { this.serviceType = value; } } static void AddBehaviors(ServiceDescription serviceDescription) { Type type = serviceDescription.ServiceType; System.ServiceModel.Description.TypeLoader.ApplyServiceInheritance >( type, serviceDescription.Behaviors, ServiceDescription.GetIServiceBehaviorAttributes); ServiceBehaviorAttribute serviceBehavior = EnsureBehaviorAttribute(serviceDescription); if (serviceBehavior.Name != null) serviceDescription.Name = new XmlName(serviceBehavior.Name).EncodedName; if (serviceBehavior.Namespace != null) serviceDescription.Namespace = serviceBehavior.Namespace; if (String.IsNullOrEmpty(serviceBehavior.ConfigurationName)) { serviceDescription.ConfigurationName = type.FullName; } else { serviceDescription.ConfigurationName = serviceBehavior.ConfigurationName; } if (ServiceHostingEnvironment.IsHosted) { AspNetCompatibilityRequirementsAttribute aspNetCompatibilityRequirements = serviceDescription.Behaviors.Find (); if (aspNetCompatibilityRequirements == null) { aspNetCompatibilityRequirements = new AspNetCompatibilityRequirementsAttribute(); serviceDescription.Behaviors.Add(aspNetCompatibilityRequirements); } } } internal static object CreateImplementation(Type serviceType) { ConstructorInfo constructor = serviceType.GetConstructor( TypeLoader.DefaultBindingFlags, null, Type.EmptyTypes, null); if (constructor == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException( SR.GetString(SR.SFxNoDefaultConstructor))); } try { object implementation = constructor.Invoke( TypeLoader.DefaultBindingFlags, null, null, System.Globalization.CultureInfo.InvariantCulture); return implementation; } catch (MethodAccessException methodAccessException) { SecurityException securityException = methodAccessException.InnerException as SecurityException; if (securityException != null && securityException.PermissionType.Equals(typeof(ReflectionPermission))) { if (DiagnosticUtility.ShouldTraceWarning) { DiagnosticUtility.ExceptionUtility.TraceHandledException(methodAccessException, TraceEventType.Warning); } throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new SecurityException(SR.GetString( SR.PartialTrustServiceCtorNotVisible, serviceType.FullName))); } else { throw; } } } static ServiceBehaviorAttribute EnsureBehaviorAttribute(ServiceDescription description) { ServiceBehaviorAttribute attr = description.Behaviors.Find (); if (attr == null) { attr = new ServiceBehaviorAttribute(); description.Behaviors.Insert(0, attr); } return attr; } // This method ensures that the description object graph is structurally sound and that none // of the fundamental SFx framework assumptions have been violated. internal void EnsureInvariants() { for (int i = 0; i < this.Endpoints.Count; i++) { ServiceEndpoint endpoint = this.Endpoints[i]; if (endpoint == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.AChannelServiceEndpointIsNull0))); } endpoint.EnsureInvariants(); } } static void GetIServiceBehaviorAttributes(Type currentServiceType, KeyedByTypeCollection behaviors) { foreach (IServiceBehavior behaviorAttribute in ServiceReflector.GetCustomAttributes(currentServiceType, typeof(IServiceBehavior))) { behaviors.Add(behaviorAttribute); } } public static ServiceDescription GetService(Type serviceType) { if (serviceType == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("serviceType"); } if (!serviceType.IsClass) { throw new ArgumentException(SR.GetString(SR.SFxServiceHostNeedsClass)); } ServiceDescription description = new ServiceDescription(); description.ServiceType = serviceType; AddBehaviors(description); SetupSingleton(description, null, false); return description; } public static ServiceDescription GetService(object serviceImplementation) { if (serviceImplementation == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("serviceImplementation"); } Type serviceType = serviceImplementation.GetType(); ServiceDescription description = new ServiceDescription(); description.ServiceType = serviceType; if (serviceImplementation is IServiceBehavior) { description.Behaviors.Add((IServiceBehavior)serviceImplementation); } AddBehaviors(description); SetupSingleton(description, serviceImplementation, true); return description; } static void SetupSingleton(ServiceDescription serviceDescription, object implementation, bool isWellKnown) { ServiceBehaviorAttribute serviceBehavior = EnsureBehaviorAttribute(serviceDescription); Type type = serviceDescription.ServiceType; if ((implementation == null) && (serviceBehavior.InstanceContextMode == InstanceContextMode.Single)) { implementation = CreateImplementation(type); } if (isWellKnown) { serviceBehavior.SetWellKnownSingleton(implementation); } else if ((implementation != null) && (serviceBehavior.InstanceContextMode == InstanceContextMode.Single)) { serviceBehavior.SetHiddenSingleton(implementation); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
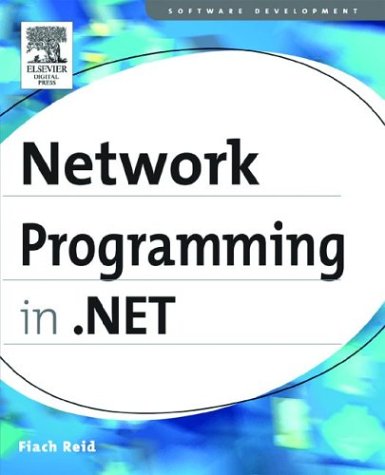
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CompositeDataBoundControl.cs
- RetrieveVirtualItemEventArgs.cs
- AppDomainUnloadedException.cs
- ElementAtQueryOperator.cs
- AnnotationHelper.cs
- Single.cs
- HtmlInputRadioButton.cs
- LocationUpdates.cs
- ShutDownListener.cs
- SchemaElement.cs
- FunctionOverloadResolver.cs
- ListViewTableCell.cs
- sitestring.cs
- SqlDuplicator.cs
- MultiSelectRootGridEntry.cs
- UInt16Storage.cs
- TextShapeableCharacters.cs
- SHA384.cs
- OraclePermissionAttribute.cs
- FormsAuthenticationUserCollection.cs
- ImmutableCommunicationTimeouts.cs
- Console.cs
- GeometryHitTestResult.cs
- ErrorHandlerFaultInfo.cs
- IPAddressCollection.cs
- webeventbuffer.cs
- UnsignedPublishLicense.cs
- ColorIndependentAnimationStorage.cs
- PromptStyle.cs
- Rect3D.cs
- ApplicationFileParser.cs
- BookmarkOptionsHelper.cs
- GeometryHitTestParameters.cs
- DependencyObjectValidator.cs
- TrackingRecord.cs
- ServiceSecurityAuditBehavior.cs
- TargetControlTypeCache.cs
- SessionStateItemCollection.cs
- _ProxyRegBlob.cs
- FontDialog.cs
- NumberSubstitution.cs
- PackUriHelper.cs
- AdPostCacheSubstitution.cs
- ApplicationException.cs
- PrintPageEvent.cs
- TextSpanModifier.cs
- WebService.cs
- ITreeGenerator.cs
- ToolStripPanelRenderEventArgs.cs
- QilScopedVisitor.cs
- WinEventWrap.cs
- ExpressionPrefixAttribute.cs
- OverflowException.cs
- LocatorPartList.cs
- CellLabel.cs
- IdentityHolder.cs
- DateTimeStorage.cs
- DifferencingCollection.cs
- IndependentAnimationStorage.cs
- LogExtent.cs
- MouseButtonEventArgs.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- log.cs
- HelpEvent.cs
- SqlMethodCallConverter.cs
- ThrowHelper.cs
- IndexedEnumerable.cs
- GeometryGroup.cs
- NameNode.cs
- MessageDesigner.cs
- SqlTriggerContext.cs
- ComPlusThreadInitializer.cs
- DataServiceProviderWrapper.cs
- AnonymousIdentificationModule.cs
- ImportContext.cs
- SpeechDetectedEventArgs.cs
- SettingsPropertyCollection.cs
- RootCodeDomSerializer.cs
- ImportCatalogPart.cs
- AudioStateChangedEventArgs.cs
- ProfilePropertySettingsCollection.cs
- GeneratedContractType.cs
- DataMember.cs
- SplashScreenNativeMethods.cs
- XmlDownloadManager.cs
- SqlDataSourceTableQuery.cs
- ProfilePropertyMetadata.cs
- EventLogEntryCollection.cs
- HtmlElement.cs
- EditingCoordinator.cs
- BigInt.cs
- xmlsaver.cs
- DataTransferEventArgs.cs
- ScrollViewerAutomationPeer.cs
- DummyDataSource.cs
- ExceptionList.cs
- TypedReference.cs
- Int32Collection.cs
- TagNameToTypeMapper.cs
- PropertyMapper.cs