Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Metadata / ObjectHelper.cs / 1305376 / ObjectHelper.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Mapping; using System.Diagnostics; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Text; using System.Data.Common.Utils; using System.IO; namespace System.Data.Metadata.Edm { ////// Helper Class for EDM Metadata - this class contains all the helper methods /// which needs access to internal methods. The other partial class contains all /// helper methods which just uses public methods/properties. The reason why we /// did this for allowing view gen to happen at compile time - all the helper /// methods that view gen or mapping uses are in the other helper class. Rest of the /// methods are in this class /// internal static partial class Helper { #region Fields // List of all the static empty list used all over the code internal static readonly ReadOnlyCollection> EmptyKeyValueStringObjectList = new ReadOnlyCollection >(new KeyValuePair [0]); internal static readonly ReadOnlyCollection EmptyStringList = new ReadOnlyCollection (new string[0]); internal static readonly ReadOnlyCollection EmptyFacetDescriptionEnumerable = new ReadOnlyCollection (new FacetDescription[0]); internal static readonly ReadOnlyCollection EmptyEdmFunctionReadOnlyCollection = new ReadOnlyCollection (new EdmFunction[0]); internal static readonly ReadOnlyCollection EmptyPrimitiveTypeReadOnlyCollection = new ReadOnlyCollection (new PrimitiveType[0]); internal static readonly KeyValuePair [] EmptyKeyValueStringObjectArray = new KeyValuePair [0]; internal const char PeriodSymbol = '.'; internal const char CommaSymbol = ','; #endregion #region Methods /// /// Returns the single error message from the list of errors /// /// ///static internal string CombineErrorMessage(IEnumerable errors) { Debug.Assert(errors != null); StringBuilder sb = new StringBuilder(System.Environment.NewLine); int count = 0; foreach (System.Data.Metadata.Edm.EdmSchemaError error in errors) { //Don't append a new line at the beginning of the messages if ((count++) != 0) { sb.Append(System.Environment.NewLine); } sb.Append(error.ToString()); } Debug.Assert(count!=0, "Empty Error List"); return sb.ToString(); } /// /// Returns the single error message from the list of errors /// /// ///static internal string CombineErrorMessage(IEnumerable errors) { StringBuilder sb = new StringBuilder(System.Environment.NewLine); int count = 0; foreach (EdmItemError error in errors) { // Only add the new line if this is not the first error if ((count++) != 0) { sb.Append(System.Environment.NewLine); } sb.Append(error.Message); } return sb.ToString(); } // requires: enumerations must have the same number of members // effects: returns paired enumeration values internal static IEnumerable > PairEnumerations (IBaseList left, IEnumerable right) { IEnumerator leftEnumerator = left.GetEnumerator(); IEnumeratorrightEnumerator = right.GetEnumerator(); while (leftEnumerator.MoveNext() && rightEnumerator.MoveNext()) { yield return new KeyValuePair((T)leftEnumerator.Current, rightEnumerator.Current); } yield break; } /// /// Returns a model (C-Space) typeusage for the given typeusage. if the type is already in c-space, it returns /// the given typeusage. The typeUsage returned is created by invoking the provider service to map from provider /// specific type to model type. /// /// typeusage ///the respective Model (C-Space) typeusage static internal TypeUsage GetModelTypeUsage(TypeUsage typeUsage) { return typeUsage.GetModelTypeUsage(); } ////// Returns a model (C-Space) typeusage for the given member typeusage. if the type is already in c-space, it returns /// the given typeusage. The typeUsage returned is created by invoking the provider service to map from provider /// specific type to model type. /// /// EdmMember ///the respective Model (C-Space) typeusage static internal TypeUsage GetModelTypeUsage(EdmMember member) { return GetModelTypeUsage(member.TypeUsage); } ////// Checks if the edm type in the cspace type usage maps to some sspace type (called it S1). If S1 is equivalent or /// promotable to the store type in sspace type usage, then it creates a new type usage with S1 and copies all facets /// if necessary /// /// Edm property containing the cspace member type information /// edm property containing the sspace member type information /// name of the mapping file from which this information was loaded from /// lineInfo containing the line information about the cspace and sspace property mapping /// List of parsing errors - we need to add any new error to this list /// store item collection ///internal static TypeUsage ValidateAndConvertTypeUsage(EdmProperty edmProperty, EdmProperty columnProperty, Xml.IXmlLineInfo lineInfo, string sourceLocation, List parsingErrors, StoreItemCollection storeItemCollection) { Debug.Assert(edmProperty.TypeUsage.EdmType.DataSpace == DataSpace.CSpace, "cspace property must have a cspace type"); Debug.Assert(columnProperty.TypeUsage.EdmType.DataSpace == DataSpace.SSpace, "sspace type usage must have a sspace type"); Debug.Assert(Helper.IsPrimitiveType(edmProperty.TypeUsage.EdmType), "cspace property must contain a primitive type"); Debug.Assert(Helper.IsPrimitiveType(columnProperty.TypeUsage.EdmType), "sspace property must contain a primitive type"); TypeUsage mappedStoreType = ValidateAndConvertTypeUsage(edmProperty, lineInfo, sourceLocation, edmProperty.TypeUsage, columnProperty.TypeUsage, parsingErrors, storeItemCollection); return mappedStoreType; } internal static TypeUsage ValidateAndConvertTypeUsage(EdmMember edmMember, Xml.IXmlLineInfo lineInfo, string sourceLocation, TypeUsage cspaceType, TypeUsage sspaceType, List parsingErrors, StoreItemCollection storeItemCollection) { // if we are already C-Space, dont call the provider. this can happen for functions. TypeUsage modelEquivalentSspace = sspaceType; if (sspaceType.EdmType.DataSpace == DataSpace.SSpace) { modelEquivalentSspace = sspaceType.GetModelTypeUsage(); } // check that cspace type is subtype of c-space equivalent type from the ssdl definition if (TypeSemantics.IsSubTypeOf(cspaceType, modelEquivalentSspace)) { return modelEquivalentSspace; } return null; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
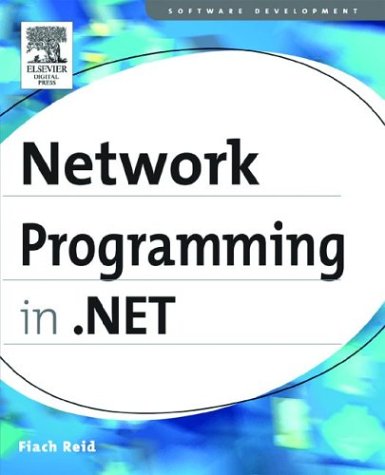
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Sql8ConformanceChecker.cs
- PlatformNotSupportedException.cs
- Solver.cs
- StyleTypedPropertyAttribute.cs
- SqlGenerator.cs
- CatalogPartChrome.cs
- Models.cs
- DataGridViewImageColumn.cs
- ChannelManagerBase.cs
- HtmlElementEventArgs.cs
- PassportAuthenticationModule.cs
- EncoderFallback.cs
- XmlElementAttribute.cs
- regiisutil.cs
- LocalizableAttribute.cs
- ContainerVisual.cs
- EncodingStreamWrapper.cs
- NodeInfo.cs
- ActiveXContainer.cs
- EntityContainerRelationshipSet.cs
- CrossSiteScriptingValidation.cs
- WindowsContainer.cs
- SimpleType.cs
- AsyncResult.cs
- Util.cs
- DataGridViewButtonCell.cs
- PathFigureCollectionConverter.cs
- CleanUpVirtualizedItemEventArgs.cs
- CustomAttributeBuilder.cs
- CustomValidator.cs
- AssociationSet.cs
- JournalNavigationScope.cs
- ProxySimple.cs
- SmiMetaData.cs
- ExportFileRequest.cs
- LinearGradientBrush.cs
- ClipboardProcessor.cs
- mediapermission.cs
- SQLInt64Storage.cs
- DynamicValidatorEventArgs.cs
- SymbolType.cs
- KnownTypesProvider.cs
- MenuCommand.cs
- RoleService.cs
- ListViewInsertionMark.cs
- FileFormatException.cs
- HttpGetClientProtocol.cs
- RenderContext.cs
- CreatingCookieEventArgs.cs
- ServiceBuildProvider.cs
- LinkedResourceCollection.cs
- HashLookup.cs
- ToolboxItemFilterAttribute.cs
- TextSelectionProcessor.cs
- CollectionsUtil.cs
- SmtpClient.cs
- BooleanFunctions.cs
- updateconfighost.cs
- TemplatedControlDesigner.cs
- LassoHelper.cs
- PointConverter.cs
- CalculatedColumn.cs
- PagerSettings.cs
- FixedDSBuilder.cs
- ByteStreamMessageEncoderFactory.cs
- CorrelationManager.cs
- ContourSegment.cs
- DataGridItem.cs
- ResetableIterator.cs
- FixedTextBuilder.cs
- ComNativeDescriptor.cs
- EdmComplexTypeAttribute.cs
- InkCanvas.cs
- BrowserCapabilitiesFactoryBase.cs
- HandleExceptionArgs.cs
- LineInfo.cs
- ClientTargetSection.cs
- TextRangeSerialization.cs
- PropertyInformation.cs
- UITypeEditors.cs
- SafeMILHandle.cs
- PageCatalogPart.cs
- xmlformatgeneratorstatics.cs
- LoginView.cs
- XmlUrlResolver.cs
- FaultConverter.cs
- entityreference_tresulttype.cs
- Semaphore.cs
- HtmlSelect.cs
- WbmpConverter.cs
- CapiNative.cs
- MediaPlayerState.cs
- QueryStringParameter.cs
- InputProcessorProfiles.cs
- WebPartAddingEventArgs.cs
- CodeArrayCreateExpression.cs
- XAMLParseException.cs
- ConfigurationValidatorBase.cs
- DataGridViewCellMouseEventArgs.cs
- GeneralTransformCollection.cs