Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DLinq / Dlinq / SqlClient / Query / SqlMethodTransformer.cs / 1305376 / SqlMethodTransformer.cs
using System; using System.Collections.Generic; using System.Text; using System.Data.Linq; namespace System.Data.Linq.SqlClient { ////// After retyping and conversions take place, some functions need to be changed into more suitable calls. /// Example: LEN -> DATALENGTH for long text types. /// internal class SqlMethodTransformer : SqlVisitor { protected SqlFactory sql; internal SqlMethodTransformer(SqlFactory sql) { this.sql = sql; } internal override SqlExpression VisitFunctionCall(SqlFunctionCall fc) { // process the arguments SqlExpression result = base.VisitFunctionCall(fc); if (result is SqlFunctionCall) { SqlFunctionCall resultFunctionCall = (SqlFunctionCall)result; if (resultFunctionCall.Name == "LEN") { SqlExpression expr = resultFunctionCall.Arguments[0]; if (expr.SqlType.IsLargeType && !expr.SqlType.SupportsLength) { result = sql.DATALENGTH(expr); if (expr.SqlType.IsUnicodeType) { result = sql.ConvertToInt(sql.Divide(result, sql.ValueFromObject(2, expr.SourceExpression))); } } } // If the return type of the sql function is not compatible with // the expected CLR type of the function, inject a conversion. This // step must be performed AFTER SqlRetyper has run. Type clrType = resultFunctionCall.SqlType.GetClosestRuntimeType(); bool skipConversion = SqlMethodTransformer.SkipConversionForDateAdd(resultFunctionCall.Name, resultFunctionCall.ClrType, clrType); if ((resultFunctionCall.ClrType != clrType) && !skipConversion) { result = sql.ConvertTo(resultFunctionCall.ClrType, resultFunctionCall); } } return result; } internal override SqlExpression VisitUnaryOperator(SqlUnary fc) { // process the arguments SqlExpression result = base.VisitUnaryOperator(fc); if (result is SqlUnary) { SqlUnary unary = (SqlUnary)result; switch (unary.NodeType) { case SqlNodeType.ClrLength: SqlExpression expr = unary.Operand; result = sql.DATALENGTH(expr); if (expr.SqlType.IsUnicodeType) { result = sql.Divide(result, sql.ValueFromObject(2, expr.SourceExpression)); } result = sql.ConvertToInt(result); break; default: break; } } return result; } // We don't inject a conversion for DATEADD if doing so will downgrade the result to // a less precise type. // private static bool SkipConversionForDateAdd(string functionName, Type expected, Type actual) { if (string.Compare(functionName, "DATEADD", StringComparison.OrdinalIgnoreCase) != 0) return false; return (expected == typeof(DateTime) && actual == typeof(DateTimeOffset)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
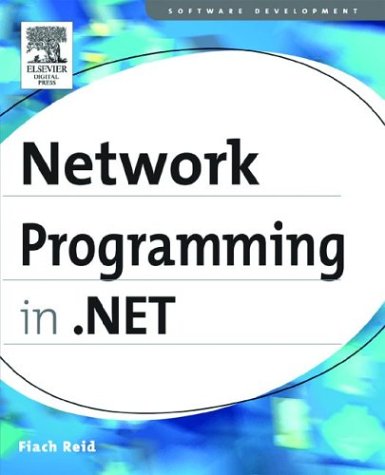
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ScrollViewer.cs
- PropertyBuilder.cs
- DbDataReader.cs
- NamespaceEmitter.cs
- ManipulationLogic.cs
- SizeAnimationClockResource.cs
- ArrangedElementCollection.cs
- BooleanProjectedSlot.cs
- WebPartsSection.cs
- QilIterator.cs
- UriScheme.cs
- CodeTypeMember.cs
- XmlSchemaSubstitutionGroup.cs
- FileDialogCustomPlaces.cs
- XmlQueryContext.cs
- RoleService.cs
- OpacityConverter.cs
- CFStream.cs
- BamlRecordWriter.cs
- CollectionViewGroupInternal.cs
- MemberAccessException.cs
- CdpEqualityComparer.cs
- XslAst.cs
- XmlNodeChangedEventArgs.cs
- XmlSchemaInfo.cs
- SettingsAttributeDictionary.cs
- PtsHelper.cs
- MetadataSerializer.cs
- DataGridViewSelectedRowCollection.cs
- Button.cs
- PipeStream.cs
- DrawingAttributeSerializer.cs
- mediaclock.cs
- ComboBoxAutomationPeer.cs
- DocumentViewerBaseAutomationPeer.cs
- IconEditor.cs
- DependentTransaction.cs
- SqlCharStream.cs
- SecurityTokenValidationException.cs
- Message.cs
- BufferAllocator.cs
- StringBuilder.cs
- XmlHelper.cs
- EventLogPermissionEntryCollection.cs
- SchemaCollectionCompiler.cs
- ObjectConverter.cs
- ReflectionPermission.cs
- AccessorTable.cs
- Stylesheet.cs
- DelegatedStream.cs
- FileSystemWatcher.cs
- MenuItemCollection.cs
- RoleManagerSection.cs
- ProfessionalColors.cs
- GridLengthConverter.cs
- CheckedListBox.cs
- OdbcConnectionStringbuilder.cs
- ItemsControl.cs
- ComboBoxRenderer.cs
- WebHttpSecurityElement.cs
- SystemWebCachingSectionGroup.cs
- HttpCachePolicy.cs
- Renderer.cs
- ChtmlTextWriter.cs
- BufferedStream.cs
- SignatureDescription.cs
- ExpressionContext.cs
- VectorValueSerializer.cs
- ProfileGroupSettings.cs
- DbProviderFactory.cs
- BasePattern.cs
- NameSpaceExtractor.cs
- Application.cs
- TemplateKey.cs
- ValuePatternIdentifiers.cs
- WebEventTraceProvider.cs
- XXXInfos.cs
- SmiEventSink_Default.cs
- DataObject.cs
- SqlWebEventProvider.cs
- PerformanceCounterCategory.cs
- WebPartTransformerCollection.cs
- MachineKeyValidationConverter.cs
- Cursors.cs
- ProfileModule.cs
- SafeBitVector32.cs
- XamlWrappingReader.cs
- QilInvoke.cs
- ThemeableAttribute.cs
- Cloud.cs
- InfoCardKeyedHashAlgorithm.cs
- CommonXSendMessage.cs
- HTMLTextWriter.cs
- ServiceElementCollection.cs
- SwitchElementsCollection.cs
- ZipIOExtraFieldZip64Element.cs
- Adorner.cs
- DiscoveryVersionConverter.cs
- ContentFilePart.cs
- ToolStripContentPanelDesigner.cs