Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Automation / Peers / ComboBoxAutomationPeer.cs / 1 / ComboBoxAutomationPeer.cs
using System; using System.Collections; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Windows; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Interop; using System.Windows.Media; using MS.Internal; using MS.Win32; namespace System.Windows.Automation.Peers { /// public class ComboBoxAutomationPeer: SelectorAutomationPeer, IValueProvider, IExpandCollapseProvider { /// public ComboBoxAutomationPeer(ComboBox owner): base(owner) {} /// override protected ItemAutomationPeer CreateItemAutomationPeer(object item) { // Use the same peer as ListBox return new ListBoxItemAutomationPeer(item, this); } /// override protected AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.ComboBox; } /// override protected string GetClassNameCore() { return "ComboBox"; } /// override public object GetPattern(PatternInterface pattern) { object iface = null; if (pattern == PatternInterface.Value) { ComboBox owner = (ComboBox)Owner; if (owner.IsEditable) iface = this; } else if(pattern == PatternInterface.ExpandCollapse) { iface = this; } else { iface = base.GetPattern(pattern); } return iface; } /// protected override ListGetChildrenCore() { List children = base.GetChildrenCore(); // include text box part into children collection ComboBox owner = (ComboBox)Owner; TextBox textBox = owner.EditableTextBoxSite; if (textBox != null) { AutomationPeer peer = UIElementAutomationPeer.CreatePeerForElement(textBox); if (peer != null) { if (children == null) children = new List (); children.Insert(0, peer); } } return children; } #region Value /// /// Request to set the value that this UI element is representing /// /// Value to set the UI to, as an object ///true if the UI element was successfully set to the specified value //[CodeAnalysis("AptcaMethodsShouldOnlyCallAptcaMethods")] //Tracking Bug: 29647 void IValueProvider.SetValue(string val) { if (val == null) throw new ArgumentNullException("val"); ComboBox owner = (ComboBox)Owner; if (!owner.IsEnabled) throw new ElementNotEnabledException(); owner.Text = val; } ///Value of a value control, as a a string. string IValueProvider.Value { get { return ((ComboBox)(((ComboBoxAutomationPeer)this).Owner)).Text; } } ///Indicates that the value can only be read, not modified. ///returns True if the control is read-only bool IValueProvider.IsReadOnly { get { ComboBox owner = (ComboBox)Owner; return !owner.IsEnabled || owner.IsReadOnly; } } #endregion Value #region ExpandCollapse ////// Blocking method that returns after the element has been expanded. /// ///true if the node was successfully expanded void IExpandCollapseProvider.Expand() { if(!IsEnabled()) throw new ElementNotEnabledException(); ComboBox owner = (ComboBox)((ComboBoxAutomationPeer)this).Owner; owner.IsDropDownOpen = true; } ////// Blocking method that returns after the element has been collapsed. /// ///true if the node was successfully collapsed void IExpandCollapseProvider.Collapse() { if(!IsEnabled()) throw new ElementNotEnabledException(); ComboBox owner = (ComboBox)((ComboBoxAutomationPeer)this).Owner; owner.IsDropDownOpen = false; } ///indicates an element's current Collapsed or Expanded state ExpandCollapseState IExpandCollapseProvider.ExpandCollapseState { get { ComboBox owner = (ComboBox)((ComboBoxAutomationPeer)this).Owner; return owner.IsDropDownOpen ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed; } } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseExpandCollapseAutomationEvent(bool oldValue, bool newValue) { RaisePropertyChangedEvent( ExpandCollapsePatternIdentifiers.ExpandCollapseStateProperty, oldValue ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed, newValue ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed); } #endregion ExpandCollapse } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Windows; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Interop; using System.Windows.Media; using MS.Internal; using MS.Win32; namespace System.Windows.Automation.Peers { /// public class ComboBoxAutomationPeer: SelectorAutomationPeer, IValueProvider, IExpandCollapseProvider { /// public ComboBoxAutomationPeer(ComboBox owner): base(owner) {} /// override protected ItemAutomationPeer CreateItemAutomationPeer(object item) { // Use the same peer as ListBox return new ListBoxItemAutomationPeer(item, this); } /// override protected AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.ComboBox; } /// override protected string GetClassNameCore() { return "ComboBox"; } /// override public object GetPattern(PatternInterface pattern) { object iface = null; if (pattern == PatternInterface.Value) { ComboBox owner = (ComboBox)Owner; if (owner.IsEditable) iface = this; } else if(pattern == PatternInterface.ExpandCollapse) { iface = this; } else { iface = base.GetPattern(pattern); } return iface; } /// protected override ListGetChildrenCore() { List children = base.GetChildrenCore(); // include text box part into children collection ComboBox owner = (ComboBox)Owner; TextBox textBox = owner.EditableTextBoxSite; if (textBox != null) { AutomationPeer peer = UIElementAutomationPeer.CreatePeerForElement(textBox); if (peer != null) { if (children == null) children = new List (); children.Insert(0, peer); } } return children; } #region Value /// /// Request to set the value that this UI element is representing /// /// Value to set the UI to, as an object ///true if the UI element was successfully set to the specified value //[CodeAnalysis("AptcaMethodsShouldOnlyCallAptcaMethods")] //Tracking Bug: 29647 void IValueProvider.SetValue(string val) { if (val == null) throw new ArgumentNullException("val"); ComboBox owner = (ComboBox)Owner; if (!owner.IsEnabled) throw new ElementNotEnabledException(); owner.Text = val; } ///Value of a value control, as a a string. string IValueProvider.Value { get { return ((ComboBox)(((ComboBoxAutomationPeer)this).Owner)).Text; } } ///Indicates that the value can only be read, not modified. ///returns True if the control is read-only bool IValueProvider.IsReadOnly { get { ComboBox owner = (ComboBox)Owner; return !owner.IsEnabled || owner.IsReadOnly; } } #endregion Value #region ExpandCollapse ////// Blocking method that returns after the element has been expanded. /// ///true if the node was successfully expanded void IExpandCollapseProvider.Expand() { if(!IsEnabled()) throw new ElementNotEnabledException(); ComboBox owner = (ComboBox)((ComboBoxAutomationPeer)this).Owner; owner.IsDropDownOpen = true; } ////// Blocking method that returns after the element has been collapsed. /// ///true if the node was successfully collapsed void IExpandCollapseProvider.Collapse() { if(!IsEnabled()) throw new ElementNotEnabledException(); ComboBox owner = (ComboBox)((ComboBoxAutomationPeer)this).Owner; owner.IsDropDownOpen = false; } ///indicates an element's current Collapsed or Expanded state ExpandCollapseState IExpandCollapseProvider.ExpandCollapseState { get { ComboBox owner = (ComboBox)((ComboBoxAutomationPeer)this).Owner; return owner.IsDropDownOpen ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed; } } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseExpandCollapseAutomationEvent(bool oldValue, bool newValue) { RaisePropertyChangedEvent( ExpandCollapsePatternIdentifiers.ExpandCollapseStateProperty, oldValue ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed, newValue ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed); } #endregion ExpandCollapse } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
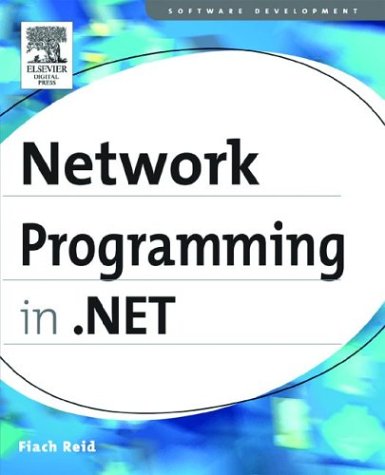
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- isolationinterop.cs
- SizeChangedEventArgs.cs
- Image.cs
- AppearanceEditorPart.cs
- CodeTypeConstructor.cs
- WebServiceMethodData.cs
- DataObjectFieldAttribute.cs
- _NativeSSPI.cs
- EntityDataSourceDataSelectionPanel.cs
- ProjectionCamera.cs
- OLEDB_Enum.cs
- ComponentEditorPage.cs
- SaveFileDialog.cs
- sqlmetadatafactory.cs
- ObservableDictionary.cs
- ManipulationVelocities.cs
- EndpointDispatcher.cs
- XamlTemplateSerializer.cs
- RegistryDataKey.cs
- ModelItemCollection.cs
- ResourceSetExpression.cs
- GiveFeedbackEvent.cs
- BaseValidator.cs
- TextEvent.cs
- XmlComment.cs
- RichTextBox.cs
- ProcessManager.cs
- TimeSpanSecondsConverter.cs
- SimpleColumnProvider.cs
- XslTransform.cs
- DiagnosticTrace.cs
- ApplicationFileCodeDomTreeGenerator.cs
- InternalConfigHost.cs
- GuidTagList.cs
- FileCodeGroup.cs
- SessionIDManager.cs
- DataKeyPropertyAttribute.cs
- CleanUpVirtualizedItemEventArgs.cs
- SQLByte.cs
- EpmTargetPathSegment.cs
- GradientStopCollection.cs
- MouseGestureConverter.cs
- ToolStripItemTextRenderEventArgs.cs
- ComponentChangedEvent.cs
- CombinedHttpChannel.cs
- BuildProviderAppliesToAttribute.cs
- SafeBitVector32.cs
- PathFigure.cs
- objectquery_tresulttype.cs
- ComNativeDescriptor.cs
- ManifestSignedXml.cs
- ItemsControlAutomationPeer.cs
- FormViewAutoFormat.cs
- ColorBuilder.cs
- ResXResourceSet.cs
- _FixedSizeReader.cs
- PtsPage.cs
- ListControl.cs
- RsaEndpointIdentity.cs
- Button.cs
- TextSelectionHighlightLayer.cs
- loginstatus.cs
- WebPartTracker.cs
- WebPartDisplayMode.cs
- IDispatchConstantAttribute.cs
- ScriptBehaviorDescriptor.cs
- UpdatePanelControlTrigger.cs
- InvokeGenerator.cs
- CodeTryCatchFinallyStatement.cs
- TimeSpanStorage.cs
- HyperLinkColumn.cs
- ImagingCache.cs
- SQLConvert.cs
- SelectionProviderWrapper.cs
- regiisutil.cs
- FamilyMapCollection.cs
- ObjectDataSourceFilteringEventArgs.cs
- ResponseBodyWriter.cs
- CacheDependency.cs
- CommandManager.cs
- List.cs
- XmlIlTypeHelper.cs
- CodeObjectCreateExpression.cs
- TraceData.cs
- SafeRegistryHandle.cs
- UriTemplateDispatchFormatter.cs
- DataProtectionSecurityStateEncoder.cs
- DNS.cs
- CopyOnWriteList.cs
- PrimitiveXmlSerializers.cs
- ControlPager.cs
- Rectangle.cs
- PropertyDescriptorComparer.cs
- Configuration.cs
- PolyLineSegment.cs
- Rect3D.cs
- ProvidePropertyAttribute.cs
- ValidatingReaderNodeData.cs
- AsyncPostBackErrorEventArgs.cs
- PrivilegedConfigurationManager.cs