Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / BufferAllocator.cs / 1 / BufferAllocator.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Buffer Allocators with recycling * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web { using System.Collections; using System.IO; using System.Globalization; using System.Web.Util; ////////////////////////////////////////////////////////////////////////////// // Generic buffer recycling /* * Base class for allocator doing buffer recycling */ internal abstract class BufferAllocator { private int _maxFree; private int _numFree; private Stack _buffers; private static int s_ProcsFudgeFactor; static BufferAllocator() { s_ProcsFudgeFactor = SystemInfo.GetNumProcessCPUs(); if (s_ProcsFudgeFactor < 1) s_ProcsFudgeFactor = 1; if (s_ProcsFudgeFactor > 4) s_ProcsFudgeFactor = 4; } internal BufferAllocator(int maxFree) { _buffers = new Stack(); _numFree = 0; _maxFree = maxFree * s_ProcsFudgeFactor; } internal /*public*/ Object GetBuffer() { Object buffer = null; if (_numFree > 0) { lock(this) { if (_numFree > 0) { buffer = _buffers.Pop(); _numFree--; } } } if (buffer == null) buffer = AllocBuffer(); return buffer; } internal void ReuseBuffer(Object buffer) { if (_numFree < _maxFree) { lock(this) { if (_numFree < _maxFree) { _buffers.Push(buffer); _numFree++; } } } } /* * To be implemented by a derived class */ abstract protected Object AllocBuffer(); } /* * Concrete allocator class for ubyte[] buffer recycling */ internal class UbyteBufferAllocator : BufferAllocator { private int _bufferSize; internal UbyteBufferAllocator(int bufferSize, int maxFree) : base(maxFree) { _bufferSize = bufferSize; } protected override Object AllocBuffer() { return new byte[_bufferSize]; } } /* * Concrete allocator class for char[] buffer recycling */ internal class CharBufferAllocator : BufferAllocator { private int _bufferSize; internal CharBufferAllocator(int bufferSize, int maxFree) : base(maxFree) { _bufferSize = bufferSize; } protected override Object AllocBuffer() { return new char[_bufferSize]; } } /* * Concrete allocator class for int[] buffer recycling */ internal class IntegerArrayAllocator : BufferAllocator { private int _arraySize; internal IntegerArrayAllocator(int arraySize, int maxFree) : base(maxFree) { _arraySize = arraySize; } protected override Object AllocBuffer() { return new int[_arraySize]; } } /* * Concrete allocator class for IntPtr[] buffer recycling */ internal class IntPtrArrayAllocator : BufferAllocator { private int _arraySize; internal IntPtrArrayAllocator(int arraySize, int maxFree) : base(maxFree) { _arraySize = arraySize; } protected override Object AllocBuffer() { return new IntPtr[_arraySize]; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Buffer Allocators with recycling * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web { using System.Collections; using System.IO; using System.Globalization; using System.Web.Util; ////////////////////////////////////////////////////////////////////////////// // Generic buffer recycling /* * Base class for allocator doing buffer recycling */ internal abstract class BufferAllocator { private int _maxFree; private int _numFree; private Stack _buffers; private static int s_ProcsFudgeFactor; static BufferAllocator() { s_ProcsFudgeFactor = SystemInfo.GetNumProcessCPUs(); if (s_ProcsFudgeFactor < 1) s_ProcsFudgeFactor = 1; if (s_ProcsFudgeFactor > 4) s_ProcsFudgeFactor = 4; } internal BufferAllocator(int maxFree) { _buffers = new Stack(); _numFree = 0; _maxFree = maxFree * s_ProcsFudgeFactor; } internal /*public*/ Object GetBuffer() { Object buffer = null; if (_numFree > 0) { lock(this) { if (_numFree > 0) { buffer = _buffers.Pop(); _numFree--; } } } if (buffer == null) buffer = AllocBuffer(); return buffer; } internal void ReuseBuffer(Object buffer) { if (_numFree < _maxFree) { lock(this) { if (_numFree < _maxFree) { _buffers.Push(buffer); _numFree++; } } } } /* * To be implemented by a derived class */ abstract protected Object AllocBuffer(); } /* * Concrete allocator class for ubyte[] buffer recycling */ internal class UbyteBufferAllocator : BufferAllocator { private int _bufferSize; internal UbyteBufferAllocator(int bufferSize, int maxFree) : base(maxFree) { _bufferSize = bufferSize; } protected override Object AllocBuffer() { return new byte[_bufferSize]; } } /* * Concrete allocator class for char[] buffer recycling */ internal class CharBufferAllocator : BufferAllocator { private int _bufferSize; internal CharBufferAllocator(int bufferSize, int maxFree) : base(maxFree) { _bufferSize = bufferSize; } protected override Object AllocBuffer() { return new char[_bufferSize]; } } /* * Concrete allocator class for int[] buffer recycling */ internal class IntegerArrayAllocator : BufferAllocator { private int _arraySize; internal IntegerArrayAllocator(int arraySize, int maxFree) : base(maxFree) { _arraySize = arraySize; } protected override Object AllocBuffer() { return new int[_arraySize]; } } /* * Concrete allocator class for IntPtr[] buffer recycling */ internal class IntPtrArrayAllocator : BufferAllocator { private int _arraySize; internal IntPtrArrayAllocator(int arraySize, int maxFree) : base(maxFree) { _arraySize = arraySize; } protected override Object AllocBuffer() { return new IntPtr[_arraySize]; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
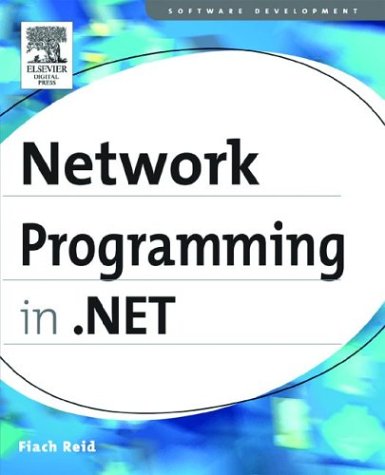
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Assign.cs
- UpdateManifestForBrowserApplication.cs
- TransportConfigurationTypeElementCollection.cs
- GifBitmapDecoder.cs
- ListItemDetailViewAttribute.cs
- FontStretchConverter.cs
- RuntimeHelpers.cs
- InternalBufferOverflowException.cs
- TrackingRecordPreFilter.cs
- BridgeDataRecord.cs
- OrderedEnumerableRowCollection.cs
- WindowsTooltip.cs
- DocumentSchemaValidator.cs
- ControlParameter.cs
- AppliedDeviceFiltersDialog.cs
- PropertyReferenceSerializer.cs
- AppDomain.cs
- ArrayExtension.cs
- SqlParameter.cs
- RemotingHelper.cs
- DataControlReferenceCollection.cs
- ExpressionBinding.cs
- DataDesignUtil.cs
- ReaderWriterLockWrapper.cs
- PenLineCapValidation.cs
- SchemaTableColumn.cs
- PixelFormat.cs
- DataGridViewCell.cs
- TranslateTransform.cs
- ErrorFormatterPage.cs
- RequestResizeEvent.cs
- StateManagedCollection.cs
- HitTestWithPointDrawingContextWalker.cs
- CaseExpr.cs
- BamlRecordReader.cs
- RangeExpression.cs
- SoapMessage.cs
- TextDecorationUnitValidation.cs
- DigestComparer.cs
- EarlyBoundInfo.cs
- SqlParameterizer.cs
- DockingAttribute.cs
- XmlDataImplementation.cs
- UnsafeNetInfoNativeMethods.cs
- StylusCollection.cs
- SchemaObjectWriter.cs
- ZipIOModeEnforcingStream.cs
- ClientRoleProvider.cs
- Expressions.cs
- ContentFilePart.cs
- Span.cs
- PrintingPermissionAttribute.cs
- RC2.cs
- PolicyStatement.cs
- AlternateViewCollection.cs
- MarshalByRefObject.cs
- QueuePropertyVariants.cs
- CallbackHandler.cs
- MenuItemBinding.cs
- DataGridRelationshipRow.cs
- WindowsScrollBarBits.cs
- HelpProvider.cs
- StorageConditionPropertyMapping.cs
- X509Utils.cs
- ParallelQuery.cs
- DynamicFilter.cs
- KnownTypesHelper.cs
- TraceEventCache.cs
- Transform.cs
- HandlerFactoryWrapper.cs
- Token.cs
- DataGrid.cs
- ResetableIterator.cs
- ConfigXmlAttribute.cs
- XsltSettings.cs
- RightsManagementEncryptedStream.cs
- ListCollectionView.cs
- UTF8Encoding.cs
- ExecutionEngineException.cs
- Parallel.cs
- dataprotectionpermissionattribute.cs
- ServiceModelSectionGroup.cs
- PeerTransportListenAddressValidator.cs
- ConfigurationException.cs
- EntitySqlException.cs
- WebServiceResponse.cs
- AmbientEnvironment.cs
- FileResponseElement.cs
- DataRowCollection.cs
- TemplateBindingExtension.cs
- ReceiveSecurityHeader.cs
- Part.cs
- ImportOptions.cs
- NameValueCollection.cs
- WinOEToolBoxItem.cs
- GridViewAutomationPeer.cs
- Span.cs
- InkCanvasAutomationPeer.cs
- Rule.cs
- SqlConnectionManager.cs