Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / IO / FileLoadException.cs / 1 / FileLoadException.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: FileLoadException ** ** ** Purpose: Exception for failure to load a file that was successfully found. ** ** ===========================================================*/ using System; using System.Globalization; using System.Runtime.Serialization; using System.Runtime.CompilerServices; using System.Security.Permissions; using SecurityException = System.Security.SecurityException; namespace System.IO { [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public class FileLoadException : IOException { private String _fileName; // the name of the file we could not load. private String _fusionLog; // fusion log (when applicable) public FileLoadException() : base(Environment.GetResourceString("IO.FileLoad")) { SetErrorCode(__HResults.COR_E_FILELOAD); } public FileLoadException(String message) : base(message) { SetErrorCode(__HResults.COR_E_FILELOAD); } public FileLoadException(String message, Exception inner) : base(message, inner) { SetErrorCode(__HResults.COR_E_FILELOAD); } public FileLoadException(String message, String fileName) : base(message) { SetErrorCode(__HResults.COR_E_FILELOAD); _fileName = fileName; } public FileLoadException(String message, String fileName, Exception inner) : base(message, inner) { SetErrorCode(__HResults.COR_E_FILELOAD); _fileName = fileName; } public override String Message { get { SetMessageField(); return _message; } } private void SetMessageField() { if (_message == null) _message = FormatFileLoadExceptionMessage(_fileName, HResult); } public String FileName { get { return _fileName; } } public override String ToString() { String s = GetType().FullName + ": " + Message; if (_fileName != null && _fileName.Length != 0) s += Environment.NewLine + String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("IO.FileName_Name"), _fileName); if (InnerException != null) s = s + " ---> " + InnerException.ToString(); if (StackTrace != null) s += Environment.NewLine + StackTrace; try { if(FusionLog!=null) { if (s==null) s=" "; s+=Environment.NewLine; s+=Environment.NewLine; s+=FusionLog; } } catch(SecurityException) { } return s; } protected FileLoadException(SerializationInfo info, StreamingContext context) : base (info, context) { // Base class constructor will check info != null. _fileName = info.GetString("FileLoad_FileName"); try { _fusionLog = info.GetString("FileLoad_FusionLog"); } catch { _fusionLog = null; } } private FileLoadException(String fileName, String fusionLog,int hResult) : base(null) { SetErrorCode(hResult); _fileName = fileName; _fusionLog=fusionLog; SetMessageField(); } public String FusionLog { [SecurityPermissionAttribute( SecurityAction.Demand, Flags = SecurityPermissionFlag.ControlEvidence | SecurityPermissionFlag.ControlPolicy)] get { return _fusionLog; } } [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { // Serialize data for our base classes. base will verify info != null. base.GetObjectData(info, context); // Serialize data for this class info.AddValue("FileLoad_FileName", _fileName, typeof(String)); try { info.AddValue("FileLoad_FusionLog", FusionLog, typeof(String)); } catch (SecurityException) { } } internal static String FormatFileLoadExceptionMessage(String fileName, int hResult) { return String.Format(CultureInfo.CurrentCulture, GetFileLoadExceptionMessage(hResult), fileName, GetMessageForHR(hResult)); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private static extern String GetFileLoadExceptionMessage(int hResult); [MethodImplAttribute(MethodImplOptions.InternalCall)] private static extern String GetMessageForHR(int hresult); } }
Link Menu
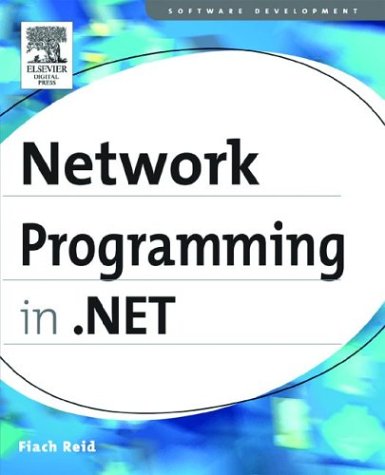
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TdsEnums.cs
- COM2Properties.cs
- PrePrepareMethodAttribute.cs
- SchemaImporterExtensionElementCollection.cs
- selecteditemcollection.cs
- TransformerInfoCollection.cs
- Odbc32.cs
- LinqDataSourceDisposeEventArgs.cs
- CodeObject.cs
- RegisteredHiddenField.cs
- Opcode.cs
- GridViewRowPresenterBase.cs
- BamlTreeMap.cs
- SafeLocalAllocation.cs
- CheckPair.cs
- SQLDouble.cs
- OpenCollectionAsyncResult.cs
- AppSettingsSection.cs
- NoneExcludedImageIndexConverter.cs
- SystemSounds.cs
- StateMachine.cs
- SemanticResultValue.cs
- AsymmetricSecurityProtocolFactory.cs
- CodeValidator.cs
- mda.cs
- SqlGenericUtil.cs
- WebPartManagerInternals.cs
- handlecollector.cs
- CodeLinePragma.cs
- XmlNodeReader.cs
- HttpModulesSection.cs
- ChooseAction.cs
- panel.cs
- ReturnType.cs
- SelfIssuedTokenFactoryCredential.cs
- StreamGeometry.cs
- FamilyTypeface.cs
- Material.cs
- DrawingContext.cs
- DbConnectionClosed.cs
- ErrorView.xaml.cs
- SchemaNames.cs
- ImageCollectionEditor.cs
- ConfigurationLockCollection.cs
- AlphabetConverter.cs
- StyleCollection.cs
- DataObject.cs
- SqlClientWrapperSmiStreamChars.cs
- RegionIterator.cs
- PropertyTabAttribute.cs
- ZipPackagePart.cs
- ByteFacetDescriptionElement.cs
- HotSpot.cs
- RegexStringValidatorAttribute.cs
- ComponentEditorForm.cs
- MembershipPasswordException.cs
- SafeSecurityHandles.cs
- TreeNodeEventArgs.cs
- XmlAnyElementAttributes.cs
- DesignRelationCollection.cs
- httpserverutility.cs
- FactoryGenerator.cs
- ListDictionary.cs
- WebPartAddingEventArgs.cs
- DropShadowBitmapEffect.cs
- ProfileManager.cs
- ClientOptions.cs
- ResourceExpressionBuilder.cs
- InstanceLockedException.cs
- SimpleLine.cs
- DictionarySectionHandler.cs
- FontWeightConverter.cs
- Int64AnimationBase.cs
- ExtendedProperty.cs
- TransformPattern.cs
- StatusBarPanelClickEvent.cs
- SupportsEventValidationAttribute.cs
- CompilerError.cs
- HttpResponseWrapper.cs
- RelationshipFixer.cs
- Int64AnimationBase.cs
- SamlAuthorizationDecisionClaimResource.cs
- TranslateTransform3D.cs
- ResXResourceReader.cs
- ControlBindingsCollection.cs
- IfAction.cs
- RectangleHotSpot.cs
- BaseValidatorDesigner.cs
- ControlSerializer.cs
- PolyBezierSegment.cs
- ResourcePropertyMemberCodeDomSerializer.cs
- ExpressionValueEditor.cs
- Int64Animation.cs
- GZipDecoder.cs
- coordinatorfactory.cs
- DeclarationUpdate.cs
- ThicknessKeyFrameCollection.cs
- TCPClient.cs
- ProcessInputEventArgs.cs
- BitmapVisualManager.cs