Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / Generated / BezierSegment.cs / 1 / BezierSegment.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media { sealed partial class BezierSegment : PathSegment { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new BezierSegment Clone() { return (BezierSegment)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new BezierSegment CloneCurrentValue() { return (BezierSegment)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// Point1 - Point. Default value is new Point(). /// public Point Point1 { get { return (Point) GetValue(Point1Property); } set { SetValueInternal(Point1Property, value); } } ////// Point2 - Point. Default value is new Point(). /// public Point Point2 { get { return (Point) GetValue(Point2Property); } set { SetValueInternal(Point2Property, value); } } ////// Point3 - Point. Default value is new Point(). /// public Point Point3 { get { return (Point) GetValue(Point3Property); } set { SetValueInternal(Point3Property, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new BezierSegment(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties // // This property finds the correct initial size for the _effectiveValues store on the // current DependencyObject as a performance optimization // // This includes: // Point1 // Point2 // Point3 // internal override int EffectiveValuesInitialSize { get { return 3; } } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the BezierSegment.Point1 property. /// public static readonly DependencyProperty Point1Property; ////// The DependencyProperty for the BezierSegment.Point2 property. /// public static readonly DependencyProperty Point2Property; ////// The DependencyProperty for the BezierSegment.Point3 property. /// public static readonly DependencyProperty Point3Property; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal static Point s_Point1 = new Point(); internal static Point s_Point2 = new Point(); internal static Point s_Point3 = new Point(); #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static BezierSegment() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(BezierSegment); Point1Property = RegisterProperty("Point1", typeof(Point), typeofThis, new Point(), null, null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); Point2Property = RegisterProperty("Point2", typeof(Point), typeofThis, new Point(), null, null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); Point3Property = RegisterProperty("Point3", typeof(Point), typeofThis, new Point(), null, null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media { sealed partial class BezierSegment : PathSegment { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new BezierSegment Clone() { return (BezierSegment)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new BezierSegment CloneCurrentValue() { return (BezierSegment)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// Point1 - Point. Default value is new Point(). /// public Point Point1 { get { return (Point) GetValue(Point1Property); } set { SetValueInternal(Point1Property, value); } } ////// Point2 - Point. Default value is new Point(). /// public Point Point2 { get { return (Point) GetValue(Point2Property); } set { SetValueInternal(Point2Property, value); } } ////// Point3 - Point. Default value is new Point(). /// public Point Point3 { get { return (Point) GetValue(Point3Property); } set { SetValueInternal(Point3Property, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new BezierSegment(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties // // This property finds the correct initial size for the _effectiveValues store on the // current DependencyObject as a performance optimization // // This includes: // Point1 // Point2 // Point3 // internal override int EffectiveValuesInitialSize { get { return 3; } } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the BezierSegment.Point1 property. /// public static readonly DependencyProperty Point1Property; ////// The DependencyProperty for the BezierSegment.Point2 property. /// public static readonly DependencyProperty Point2Property; ////// The DependencyProperty for the BezierSegment.Point3 property. /// public static readonly DependencyProperty Point3Property; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal static Point s_Point1 = new Point(); internal static Point s_Point2 = new Point(); internal static Point s_Point3 = new Point(); #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static BezierSegment() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(BezierSegment); Point1Property = RegisterProperty("Point1", typeof(Point), typeofThis, new Point(), null, null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); Point2Property = RegisterProperty("Point2", typeof(Point), typeofThis, new Point(), null, null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); Point3Property = RegisterProperty("Point3", typeof(Point), typeofThis, new Point(), null, null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
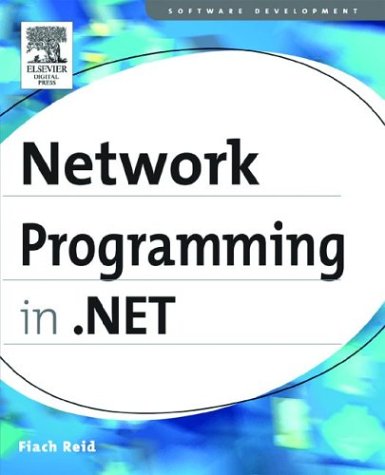
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TimeSpanHelper.cs
- RecognizedPhrase.cs
- RemotingConfigParser.cs
- InvalidCommandTreeException.cs
- TextEndOfParagraph.cs
- DelegateTypeInfo.cs
- MetadataPropertyvalue.cs
- HMACSHA384.cs
- DataBoundControl.cs
- BorderSidesEditor.cs
- OperandQuery.cs
- TracePayload.cs
- LinkedList.cs
- TextElementEnumerator.cs
- LicenseException.cs
- ObjectHelper.cs
- NegatedCellConstant.cs
- FixedSOMLineRanges.cs
- QuaternionKeyFrameCollection.cs
- EdmItemCollection.cs
- MissingSatelliteAssemblyException.cs
- EventData.cs
- CheckBox.cs
- _ListenerRequestStream.cs
- WebPartTransformerCollection.cs
- FormViewDeletedEventArgs.cs
- ResolveMatchesApril2005.cs
- Regex.cs
- InternalUserCancelledException.cs
- TreeNodeConverter.cs
- ContextStaticAttribute.cs
- DataSourceHelper.cs
- Pen.cs
- ArraySegment.cs
- TargetConverter.cs
- PointAnimation.cs
- SafeLibraryHandle.cs
- EmptyElement.cs
- XmlElement.cs
- ObfuscationAttribute.cs
- DataFormats.cs
- DelegatedStream.cs
- SqlWriter.cs
- PageCatalogPartDesigner.cs
- PropertyRecord.cs
- ValidationResult.cs
- PermissionAttributes.cs
- Utils.cs
- AnimatedTypeHelpers.cs
- xsdvalidator.cs
- _SecureChannel.cs
- TypeDelegator.cs
- GifBitmapEncoder.cs
- TemplatedMailWebEventProvider.cs
- URI.cs
- XmlRawWriter.cs
- ToolStripGrip.cs
- ReferencedAssembly.cs
- LeafCellTreeNode.cs
- EntitySetBaseCollection.cs
- MailDefinition.cs
- SchemaMapping.cs
- MissingMethodException.cs
- BitmapSizeOptions.cs
- ByteConverter.cs
- UserControlParser.cs
- MemberMaps.cs
- GridViewUpdateEventArgs.cs
- FlagsAttribute.cs
- ResXResourceReader.cs
- KernelTypeValidation.cs
- DataGridViewTopLeftHeaderCell.cs
- FlowSwitchLink.cs
- ViewKeyConstraint.cs
- ChangeProcessor.cs
- DataPagerFieldCollection.cs
- XPathNodeList.cs
- Event.cs
- TreeNodeStyleCollection.cs
- objectquery_tresulttype.cs
- RecordConverter.cs
- CompilerErrorCollection.cs
- EmbeddedObject.cs
- CurrencyWrapper.cs
- PagesSection.cs
- ObjectDataSourceChooseMethodsPanel.cs
- ScopelessEnumAttribute.cs
- RegexRunnerFactory.cs
- XmlTypeMapping.cs
- BindingsSection.cs
- SAPICategories.cs
- LifetimeServices.cs
- MobileComponentEditorPage.cs
- InputBindingCollection.cs
- DataContractSet.cs
- UpdateTracker.cs
- validationstate.cs
- TableLayoutStyleCollection.cs
- ActivationArguments.cs
- MultipleViewPattern.cs