Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / OutputCacheSettings.cs / 3 / OutputCacheSettings.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Web.Util; using System.Security.Permissions; [FlagsAttribute()] internal enum OutputCacheParameter : int { // Flags to determine if a particular parameter has been set CacheProfile = 0x00000001, Duration = 0x00000002, Enabled = 0x00000004, Location = 0x00000008, NoStore = 0x00000010, SqlDependency = 0x00000020, VaryByControl = 0x00000040, VaryByCustom = 0x00000080, VaryByHeader = 0x00000100, VaryByParam = 0x00000200, VaryByContentEncoding = 0x00000400 } [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class OutputCacheParameters { #pragma warning disable 0649 private SimpleBitVector32 _flags; #pragma warning restore 0649 private bool _enabled = true; private int _duration; private OutputCacheLocation _location; private string _varyByCustom; private string _varyByParam; private string _varyByContentEncoding; private string _varyByHeader; private bool _noStore; private string _sqlDependency; private string _varyByControl; private string _cacheProfile; public OutputCacheParameters() { } internal bool IsParameterSet(OutputCacheParameter value) { return _flags[(int) value]; } public bool Enabled { get { return _enabled; } set { _flags[(int) OutputCacheParameter.Enabled] = true; _enabled = value; } } public int Duration { get { return _duration; } set { _flags[(int) OutputCacheParameter.Duration] = true; _duration = value; } } public OutputCacheLocation Location { get { return _location; } set { _flags[(int) OutputCacheParameter.Location] = true; _location = value; } } public string VaryByCustom { get { return _varyByCustom; } set { _flags[(int) OutputCacheParameter.VaryByCustom] = true; _varyByCustom = value; } } public string VaryByParam { get { return _varyByParam; } set { _flags[(int) OutputCacheParameter.VaryByParam] = true; _varyByParam = value; } } public string VaryByContentEncoding { get { return _varyByContentEncoding; } set { _flags[(int) OutputCacheParameter.VaryByContentEncoding] = true; _varyByContentEncoding = value; } } public string VaryByHeader { get { return _varyByHeader; } set { _flags[(int) OutputCacheParameter.VaryByHeader] = true; _varyByHeader = value; } } public bool NoStore { get { return _noStore; } set { _flags[(int) OutputCacheParameter.NoStore] = true; _noStore = value; } } public string SqlDependency { get { return _sqlDependency; } set { _flags[(int) OutputCacheParameter.SqlDependency] = true; _sqlDependency = value; } } public string VaryByControl { get { return _varyByControl; } set { _flags[(int) OutputCacheParameter.VaryByControl] = true; _varyByControl = value; } } public string CacheProfile { get { return _cacheProfile; } set { _flags[(int) OutputCacheParameter.CacheProfile] = true; _cacheProfile = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Web.Util; using System.Security.Permissions; [FlagsAttribute()] internal enum OutputCacheParameter : int { // Flags to determine if a particular parameter has been set CacheProfile = 0x00000001, Duration = 0x00000002, Enabled = 0x00000004, Location = 0x00000008, NoStore = 0x00000010, SqlDependency = 0x00000020, VaryByControl = 0x00000040, VaryByCustom = 0x00000080, VaryByHeader = 0x00000100, VaryByParam = 0x00000200, VaryByContentEncoding = 0x00000400 } [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class OutputCacheParameters { #pragma warning disable 0649 private SimpleBitVector32 _flags; #pragma warning restore 0649 private bool _enabled = true; private int _duration; private OutputCacheLocation _location; private string _varyByCustom; private string _varyByParam; private string _varyByContentEncoding; private string _varyByHeader; private bool _noStore; private string _sqlDependency; private string _varyByControl; private string _cacheProfile; public OutputCacheParameters() { } internal bool IsParameterSet(OutputCacheParameter value) { return _flags[(int) value]; } public bool Enabled { get { return _enabled; } set { _flags[(int) OutputCacheParameter.Enabled] = true; _enabled = value; } } public int Duration { get { return _duration; } set { _flags[(int) OutputCacheParameter.Duration] = true; _duration = value; } } public OutputCacheLocation Location { get { return _location; } set { _flags[(int) OutputCacheParameter.Location] = true; _location = value; } } public string VaryByCustom { get { return _varyByCustom; } set { _flags[(int) OutputCacheParameter.VaryByCustom] = true; _varyByCustom = value; } } public string VaryByParam { get { return _varyByParam; } set { _flags[(int) OutputCacheParameter.VaryByParam] = true; _varyByParam = value; } } public string VaryByContentEncoding { get { return _varyByContentEncoding; } set { _flags[(int) OutputCacheParameter.VaryByContentEncoding] = true; _varyByContentEncoding = value; } } public string VaryByHeader { get { return _varyByHeader; } set { _flags[(int) OutputCacheParameter.VaryByHeader] = true; _varyByHeader = value; } } public bool NoStore { get { return _noStore; } set { _flags[(int) OutputCacheParameter.NoStore] = true; _noStore = value; } } public string SqlDependency { get { return _sqlDependency; } set { _flags[(int) OutputCacheParameter.SqlDependency] = true; _sqlDependency = value; } } public string VaryByControl { get { return _varyByControl; } set { _flags[(int) OutputCacheParameter.VaryByControl] = true; _varyByControl = value; } } public string CacheProfile { get { return _cacheProfile; } set { _flags[(int) OutputCacheParameter.CacheProfile] = true; _cacheProfile = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
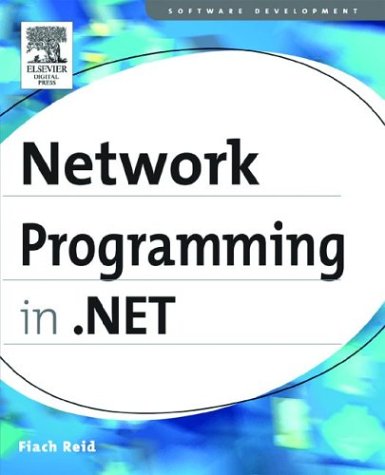
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WindowsScrollBar.cs
- SqlDataSourceConfigureSortForm.cs
- RotationValidation.cs
- StateChangeEvent.cs
- DynamicValidatorEventArgs.cs
- InfoCardProofToken.cs
- odbcmetadatacollectionnames.cs
- Zone.cs
- EntityDataSourceWrapperPropertyDescriptor.cs
- DescendentsWalker.cs
- SchemaTableOptionalColumn.cs
- BindingExpressionBase.cs
- TagMapCollection.cs
- DbCommandTree.cs
- GenericUriParser.cs
- URLMembershipCondition.cs
- RegexCompilationInfo.cs
- TableAutomationPeer.cs
- EventWaitHandle.cs
- OdbcCommand.cs
- CompilerGeneratedAttribute.cs
- UniqueIdentifierService.cs
- objectquery_tresulttype.cs
- GeneralTransform3D.cs
- SqlBooleanizer.cs
- Array.cs
- DbInsertCommandTree.cs
- BaseCodePageEncoding.cs
- DES.cs
- ForeignKeyConstraint.cs
- URLMembershipCondition.cs
- ChangeConflicts.cs
- QilGeneratorEnv.cs
- CqlIdentifiers.cs
- CachedResourceDictionaryExtension.cs
- WindowsFormsSectionHandler.cs
- AssociatedControlConverter.cs
- DataAdapter.cs
- ContentFilePart.cs
- CommonGetThemePartSize.cs
- _SSPISessionCache.cs
- ServerValidateEventArgs.cs
- XmlStreamedByteStreamReader.cs
- XmlIgnoreAttribute.cs
- XmlUTF8TextReader.cs
- PngBitmapEncoder.cs
- SiteMapDesignerDataSourceView.cs
- HandlerBase.cs
- WindowsRegion.cs
- SerializationEventsCache.cs
- BamlResourceContent.cs
- ChildChangedEventArgs.cs
- DecimalAnimationBase.cs
- ObjectHandle.cs
- DataGridParentRows.cs
- Itemizer.cs
- TextAction.cs
- lengthconverter.cs
- RetrieveVirtualItemEventArgs.cs
- DSASignatureFormatter.cs
- DefaultParameterValueAttribute.cs
- HttpStreamFormatter.cs
- DbProviderServices.cs
- ObjectItemCollectionAssemblyCacheEntry.cs
- TableLayoutStyleCollection.cs
- DataChangedEventManager.cs
- SQLByteStorage.cs
- BaseTemplatedMobileComponentEditor.cs
- OrderablePartitioner.cs
- RenderDataDrawingContext.cs
- WorkflowMessageEventArgs.cs
- TextPenaltyModule.cs
- CellTreeSimplifier.cs
- ObjectDataProvider.cs
- LocalizableResourceBuilder.cs
- CodeThrowExceptionStatement.cs
- AssemblyInfo.cs
- UpDownBaseDesigner.cs
- SubclassTypeValidatorAttribute.cs
- SystemResourceHost.cs
- TargetConverter.cs
- KnownBoxes.cs
- SqlErrorCollection.cs
- InternalEnumValidator.cs
- SqlWorkflowPersistenceService.cs
- RC2CryptoServiceProvider.cs
- _FtpControlStream.cs
- DocumentViewerAutomationPeer.cs
- NameValuePair.cs
- WindowsRebar.cs
- DependencyPropertyKey.cs
- ScrollPattern.cs
- FileDialogCustomPlace.cs
- CollectionBuilder.cs
- MailDefinition.cs
- GregorianCalendarHelper.cs
- CheckBox.cs
- PropertyChangingEventArgs.cs
- PrintDialogException.cs
- OutputScopeManager.cs