Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / Configuration / BuildProvider.cs / 1 / BuildProvider.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.Globalization; using System.IO; using System.Text; using System.Web.Compilation; using System.Reflection; using System.Web.Hosting; using System.Web.UI; using System.CodeDom.Compiler; using System.Web.Util; using System.ComponentModel; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class BuildProvider : ConfigurationElement { private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propExtension = new ConfigurationProperty("extension", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired | ConfigurationPropertyOptions.IsKey); private static readonly ConfigurationProperty _propType = new ConfigurationProperty("type", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired); private Type _type; // AppliesTo value from the BuildProviderAppliesToAttribute private BuildProviderAppliesTo _appliesToInternal; static BuildProvider() { _properties = new ConfigurationPropertyCollection(); _properties.Add(_propExtension); _properties.Add(_propType); } public BuildProvider(String extension, String type) : this() { Extension = extension; Type = type; } internal BuildProvider() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } // this override is required because AppliesTo may be in any order in the // property string but it still and the default equals operator would consider // them different depending on order in the persisted string. public override bool Equals(object provider) { BuildProvider o = provider as BuildProvider; return (o != null && StringUtil.EqualsIgnoreCase(Extension, o.Extension) && Type == o.Type); } public override int GetHashCode() { return HashCodeCombiner.CombineHashCodes(Extension.ToLower(CultureInfo.InvariantCulture).GetHashCode(), Type.GetHashCode()); } [ConfigurationProperty("extension", IsRequired = true, IsKey = true, DefaultValue = "")] [StringValidator(MinLength = 1)] public string Extension { get { return (string)base[_propExtension]; } set { base[_propExtension] = value; } } [ConfigurationProperty("type", IsRequired = true, DefaultValue = "")] [StringValidator(MinLength = 1)] public string Type { get { return (string)base[_propType]; } set { base[_propType] = value; } } internal Type TypeInternal { get { if (_type == null) { lock (this) { if (_type == null) { _type = CompilationUtil.LoadTypeWithChecks(Type, typeof(System.Web.Compilation.BuildProvider), null, this, "type"); } } } return _type; } } internal BuildProviderAppliesTo AppliesToInternal { get { if (_appliesToInternal != 0) return _appliesToInternal; // Check whether the control builder's class exposes an AppliesTo attribute object[] attrs = TypeInternal.GetCustomAttributes( typeof(BuildProviderAppliesToAttribute), /*inherit*/ true); if ((attrs != null) && (attrs.Length > 0)) { Debug.Assert(attrs[0] is BuildProviderAppliesToAttribute); _appliesToInternal = ((BuildProviderAppliesToAttribute)attrs[0]).AppliesTo; } else { // Default to applying to All _appliesToInternal = BuildProviderAppliesTo.All; } return _appliesToInternal; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.Globalization; using System.IO; using System.Text; using System.Web.Compilation; using System.Reflection; using System.Web.Hosting; using System.Web.UI; using System.CodeDom.Compiler; using System.Web.Util; using System.ComponentModel; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class BuildProvider : ConfigurationElement { private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propExtension = new ConfigurationProperty("extension", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired | ConfigurationPropertyOptions.IsKey); private static readonly ConfigurationProperty _propType = new ConfigurationProperty("type", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired); private Type _type; // AppliesTo value from the BuildProviderAppliesToAttribute private BuildProviderAppliesTo _appliesToInternal; static BuildProvider() { _properties = new ConfigurationPropertyCollection(); _properties.Add(_propExtension); _properties.Add(_propType); } public BuildProvider(String extension, String type) : this() { Extension = extension; Type = type; } internal BuildProvider() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } // this override is required because AppliesTo may be in any order in the // property string but it still and the default equals operator would consider // them different depending on order in the persisted string. public override bool Equals(object provider) { BuildProvider o = provider as BuildProvider; return (o != null && StringUtil.EqualsIgnoreCase(Extension, o.Extension) && Type == o.Type); } public override int GetHashCode() { return HashCodeCombiner.CombineHashCodes(Extension.ToLower(CultureInfo.InvariantCulture).GetHashCode(), Type.GetHashCode()); } [ConfigurationProperty("extension", IsRequired = true, IsKey = true, DefaultValue = "")] [StringValidator(MinLength = 1)] public string Extension { get { return (string)base[_propExtension]; } set { base[_propExtension] = value; } } [ConfigurationProperty("type", IsRequired = true, DefaultValue = "")] [StringValidator(MinLength = 1)] public string Type { get { return (string)base[_propType]; } set { base[_propType] = value; } } internal Type TypeInternal { get { if (_type == null) { lock (this) { if (_type == null) { _type = CompilationUtil.LoadTypeWithChecks(Type, typeof(System.Web.Compilation.BuildProvider), null, this, "type"); } } } return _type; } } internal BuildProviderAppliesTo AppliesToInternal { get { if (_appliesToInternal != 0) return _appliesToInternal; // Check whether the control builder's class exposes an AppliesTo attribute object[] attrs = TypeInternal.GetCustomAttributes( typeof(BuildProviderAppliesToAttribute), /*inherit*/ true); if ((attrs != null) && (attrs.Length > 0)) { Debug.Assert(attrs[0] is BuildProviderAppliesToAttribute); _appliesToInternal = ((BuildProviderAppliesToAttribute)attrs[0]).AppliesTo; } else { // Default to applying to All _appliesToInternal = BuildProviderAppliesTo.All; } return _appliesToInternal; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
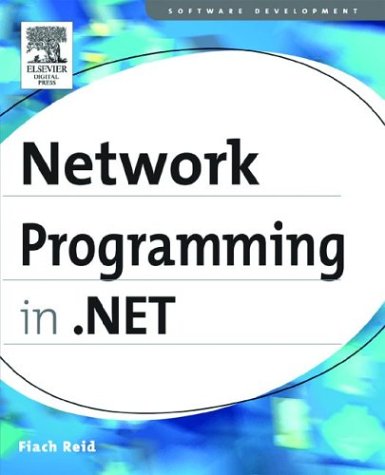
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CriticalFinalizerObject.cs
- WindowsHyperlink.cs
- PersonalizableTypeEntry.cs
- SafeArrayTypeMismatchException.cs
- CacheOutputQuery.cs
- PopOutPanel.cs
- ToolStripButton.cs
- StackSpiller.Generated.cs
- FigureParaClient.cs
- NewItemsContextMenuStrip.cs
- ToggleButton.cs
- CustomValidator.cs
- WebControlAdapter.cs
- FormsAuthenticationUserCollection.cs
- DynamicResourceExtension.cs
- SplashScreen.cs
- FactoryGenerator.cs
- KernelTypeValidation.cs
- WebPartMenu.cs
- PackWebRequestFactory.cs
- MatrixTransform3D.cs
- EndpointInstanceProvider.cs
- assemblycache.cs
- ColumnMap.cs
- Expander.cs
- OperationAbortedException.cs
- PackageStore.cs
- ConfigurationSectionCollection.cs
- CodeThrowExceptionStatement.cs
- Quaternion.cs
- StrokeIntersection.cs
- RequestCachingSection.cs
- MapPathBasedVirtualPathProvider.cs
- PathFigure.cs
- XmlNullResolver.cs
- LinqDataSourceHelper.cs
- EdmValidator.cs
- ListBase.cs
- InvokeProviderWrapper.cs
- DtdParser.cs
- ClientWindowsAuthenticationMembershipProvider.cs
- WebPartConnectionsCloseVerb.cs
- SpecularMaterial.cs
- CatalogZoneBase.cs
- ReadOnlyDataSource.cs
- TextTreeRootNode.cs
- PrefixQName.cs
- HMACMD5.cs
- JpegBitmapDecoder.cs
- SqlDataReaderSmi.cs
- TextViewSelectionProcessor.cs
- LinkConverter.cs
- CodeMethodReturnStatement.cs
- SymLanguageVendor.cs
- ListViewInsertionMark.cs
- ResourcesChangeInfo.cs
- IndexOutOfRangeException.cs
- PropertyCollection.cs
- SiteMapNodeItem.cs
- DispatcherExceptionEventArgs.cs
- SspiSafeHandles.cs
- TrackingProfileCache.cs
- ToolStripControlHost.cs
- EntityDataSourceQueryBuilder.cs
- DesigntimeLicenseContext.cs
- SqlInternalConnectionSmi.cs
- ColumnReorderedEventArgs.cs
- TableRow.cs
- KerberosTicketHashIdentifierClause.cs
- InkSerializer.cs
- ArrayMergeHelper.cs
- QueryOpcode.cs
- AutoResetEvent.cs
- ArgumentException.cs
- ArgumentNullException.cs
- PieceDirectory.cs
- CompilerState.cs
- Panel.cs
- BindingElementCollection.cs
- EmptyStringExpandableObjectConverter.cs
- HatchBrush.cs
- OracleColumn.cs
- TextShapeableCharacters.cs
- TextEndOfSegment.cs
- BinaryObjectWriter.cs
- RsaSecurityToken.cs
- PostBackTrigger.cs
- GcHandle.cs
- UnmanagedBitmapWrapper.cs
- Context.cs
- IndexedEnumerable.cs
- TextElementCollectionHelper.cs
- Int32Storage.cs
- PropertyEntry.cs
- Utils.cs
- FamilyTypefaceCollection.cs
- ColorAnimation.cs
- XMLDiffLoader.cs
- DataRowView.cs
- MatrixTransform3D.cs