Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / MS / Internal / Ink / StrokeIntersection.cs / 1305600 / StrokeIntersection.cs
//------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using MS.Internal; using MS.Internal.Ink; using MS.Utility; using System; using System.Windows; using System.Collections.Generic; using System.Globalization; namespace System.Windows.Ink { ////// A helper struct that represents a fragment of a stroke spine. /// internal struct StrokeIntersection { #region Private statics private static StrokeIntersection s_empty = new StrokeIntersection(AfterLast, AfterLast, BeforeFirst, BeforeFirst); private static StrokeIntersection s_full = new StrokeIntersection(BeforeFirst, BeforeFirst, AfterLast, AfterLast); #endregion #region Public API ////// BeforeFirst /// ///internal static double BeforeFirst { get { return StrokeFIndices.BeforeFirst; } } /// /// AfterLast /// ///internal static double AfterLast { get { return StrokeFIndices.AfterLast; } } /// /// Constructor /// /// /// /// /// internal StrokeIntersection(double hitBegin, double inBegin, double inEnd, double hitEnd) { //ISSUE-2004/12/06-XiaoTu: should we validate the input? _hitSegment = new StrokeFIndices(hitBegin, hitEnd); _inSegment = new StrokeFIndices(inBegin, inEnd); } ////// hitBeginFIndex /// ///internal double HitBegin { set { _hitSegment.BeginFIndex = value; } } /// /// hitEndFIndex /// ///internal double HitEnd { get { return _hitSegment.EndFIndex; } set { _hitSegment.EndFIndex = value; } } /// /// InBegin /// ///internal double InBegin { get { return _inSegment.BeginFIndex; } set { _inSegment.BeginFIndex = value; } } /// /// InEnd /// ///internal double InEnd { get { return _inSegment.EndFIndex; } set { _inSegment.EndFIndex = value; } } /// /// ToString /// public override string ToString() { return "{" + StrokeFIndices.GetStringRepresentation(_hitSegment.BeginFIndex) + "," + StrokeFIndices.GetStringRepresentation(_inSegment.BeginFIndex) + "," + StrokeFIndices.GetStringRepresentation(_inSegment.EndFIndex) + "," + StrokeFIndices.GetStringRepresentation(_hitSegment.EndFIndex) + "}"; } ////// Equals /// /// ///public override bool Equals(Object obj) { // Check for null and compare run-time types if (obj == null || GetType() != obj.GetType()) return false; return ((StrokeIntersection)obj == this); } /// /// GetHashCode /// ///public override int GetHashCode() { return _hitSegment.GetHashCode() ^ _inSegment.GetHashCode(); } /// /// operator == /// /// /// ///public static bool operator ==(StrokeIntersection left, StrokeIntersection right) { return (left._hitSegment == right._hitSegment && left._inSegment == right._inSegment); } /// /// operator != /// /// /// ///public static bool operator !=(StrokeIntersection left, StrokeIntersection right) { return !(left == right); } #endregion #region Internal API /// /// /// internal static StrokeIntersection Full { get { return s_full; } } ////// /// internal bool IsEmpty { get { return _hitSegment.IsEmpty; } } ////// /// internal StrokeFIndices HitSegment { get { return _hitSegment; } } ////// /// internal StrokeFIndices InSegment { get { return _inSegment; } } #endregion #region Internal static methods ////// Get the "in-segments" of the intersections. /// internal static StrokeFIndices[] GetInSegments(StrokeIntersection[] intersections) { System.Diagnostics.Debug.Assert(intersections != null); System.Diagnostics.Debug.Assert(intersections.Length > 0); ListinFIndices = new List (intersections.Length); for (int j = 0; j < intersections.Length; j++) { System.Diagnostics.Debug.Assert(!intersections[j].IsEmpty); if (!intersections[j].InSegment.IsEmpty) { if (inFIndices.Count > 0 && inFIndices[inFIndices.Count - 1].EndFIndex >= intersections[j].InSegment.BeginFIndex) { //merge StrokeFIndices sfiPrevious = inFIndices[inFIndices.Count - 1]; sfiPrevious.EndFIndex = intersections[j].InSegment.EndFIndex; inFIndices[inFIndices.Count - 1] = sfiPrevious; } else { inFIndices.Add(intersections[j].InSegment); } } } return inFIndices.ToArray(); } /// /// Get the "hit-segments" /// internal static StrokeFIndices[] GetHitSegments(StrokeIntersection[] intersections) { System.Diagnostics.Debug.Assert(intersections != null); System.Diagnostics.Debug.Assert(intersections.Length > 0); ListhitFIndices = new List (intersections.Length); for (int j = 0; j < intersections.Length; j++) { System.Diagnostics.Debug.Assert(!intersections[j].IsEmpty); if (!intersections[j].HitSegment.IsEmpty) { if (hitFIndices.Count > 0 && hitFIndices[hitFIndices.Count - 1].EndFIndex >= intersections[j].HitSegment.BeginFIndex) { //merge StrokeFIndices sfiPrevious = hitFIndices[hitFIndices.Count - 1]; sfiPrevious.EndFIndex = intersections[j].HitSegment.EndFIndex; hitFIndices[hitFIndices.Count - 1] = sfiPrevious; } else { hitFIndices.Add(intersections[j].HitSegment); } } } return hitFIndices.ToArray(); } #endregion #region Fields private StrokeFIndices _hitSegment; private StrokeFIndices _inSegment; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using MS.Internal; using MS.Internal.Ink; using MS.Utility; using System; using System.Windows; using System.Collections.Generic; using System.Globalization; namespace System.Windows.Ink { ////// A helper struct that represents a fragment of a stroke spine. /// internal struct StrokeIntersection { #region Private statics private static StrokeIntersection s_empty = new StrokeIntersection(AfterLast, AfterLast, BeforeFirst, BeforeFirst); private static StrokeIntersection s_full = new StrokeIntersection(BeforeFirst, BeforeFirst, AfterLast, AfterLast); #endregion #region Public API ////// BeforeFirst /// ///internal static double BeforeFirst { get { return StrokeFIndices.BeforeFirst; } } /// /// AfterLast /// ///internal static double AfterLast { get { return StrokeFIndices.AfterLast; } } /// /// Constructor /// /// /// /// /// internal StrokeIntersection(double hitBegin, double inBegin, double inEnd, double hitEnd) { //ISSUE-2004/12/06-XiaoTu: should we validate the input? _hitSegment = new StrokeFIndices(hitBegin, hitEnd); _inSegment = new StrokeFIndices(inBegin, inEnd); } ////// hitBeginFIndex /// ///internal double HitBegin { set { _hitSegment.BeginFIndex = value; } } /// /// hitEndFIndex /// ///internal double HitEnd { get { return _hitSegment.EndFIndex; } set { _hitSegment.EndFIndex = value; } } /// /// InBegin /// ///internal double InBegin { get { return _inSegment.BeginFIndex; } set { _inSegment.BeginFIndex = value; } } /// /// InEnd /// ///internal double InEnd { get { return _inSegment.EndFIndex; } set { _inSegment.EndFIndex = value; } } /// /// ToString /// public override string ToString() { return "{" + StrokeFIndices.GetStringRepresentation(_hitSegment.BeginFIndex) + "," + StrokeFIndices.GetStringRepresentation(_inSegment.BeginFIndex) + "," + StrokeFIndices.GetStringRepresentation(_inSegment.EndFIndex) + "," + StrokeFIndices.GetStringRepresentation(_hitSegment.EndFIndex) + "}"; } ////// Equals /// /// ///public override bool Equals(Object obj) { // Check for null and compare run-time types if (obj == null || GetType() != obj.GetType()) return false; return ((StrokeIntersection)obj == this); } /// /// GetHashCode /// ///public override int GetHashCode() { return _hitSegment.GetHashCode() ^ _inSegment.GetHashCode(); } /// /// operator == /// /// /// ///public static bool operator ==(StrokeIntersection left, StrokeIntersection right) { return (left._hitSegment == right._hitSegment && left._inSegment == right._inSegment); } /// /// operator != /// /// /// ///public static bool operator !=(StrokeIntersection left, StrokeIntersection right) { return !(left == right); } #endregion #region Internal API /// /// /// internal static StrokeIntersection Full { get { return s_full; } } ////// /// internal bool IsEmpty { get { return _hitSegment.IsEmpty; } } ////// /// internal StrokeFIndices HitSegment { get { return _hitSegment; } } ////// /// internal StrokeFIndices InSegment { get { return _inSegment; } } #endregion #region Internal static methods ////// Get the "in-segments" of the intersections. /// internal static StrokeFIndices[] GetInSegments(StrokeIntersection[] intersections) { System.Diagnostics.Debug.Assert(intersections != null); System.Diagnostics.Debug.Assert(intersections.Length > 0); ListinFIndices = new List (intersections.Length); for (int j = 0; j < intersections.Length; j++) { System.Diagnostics.Debug.Assert(!intersections[j].IsEmpty); if (!intersections[j].InSegment.IsEmpty) { if (inFIndices.Count > 0 && inFIndices[inFIndices.Count - 1].EndFIndex >= intersections[j].InSegment.BeginFIndex) { //merge StrokeFIndices sfiPrevious = inFIndices[inFIndices.Count - 1]; sfiPrevious.EndFIndex = intersections[j].InSegment.EndFIndex; inFIndices[inFIndices.Count - 1] = sfiPrevious; } else { inFIndices.Add(intersections[j].InSegment); } } } return inFIndices.ToArray(); } /// /// Get the "hit-segments" /// internal static StrokeFIndices[] GetHitSegments(StrokeIntersection[] intersections) { System.Diagnostics.Debug.Assert(intersections != null); System.Diagnostics.Debug.Assert(intersections.Length > 0); ListhitFIndices = new List (intersections.Length); for (int j = 0; j < intersections.Length; j++) { System.Diagnostics.Debug.Assert(!intersections[j].IsEmpty); if (!intersections[j].HitSegment.IsEmpty) { if (hitFIndices.Count > 0 && hitFIndices[hitFIndices.Count - 1].EndFIndex >= intersections[j].HitSegment.BeginFIndex) { //merge StrokeFIndices sfiPrevious = hitFIndices[hitFIndices.Count - 1]; sfiPrevious.EndFIndex = intersections[j].HitSegment.EndFIndex; hitFIndices[hitFIndices.Count - 1] = sfiPrevious; } else { hitFIndices.Add(intersections[j].HitSegment); } } } return hitFIndices.ToArray(); } #endregion #region Fields private StrokeFIndices _hitSegment; private StrokeFIndices _inSegment; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
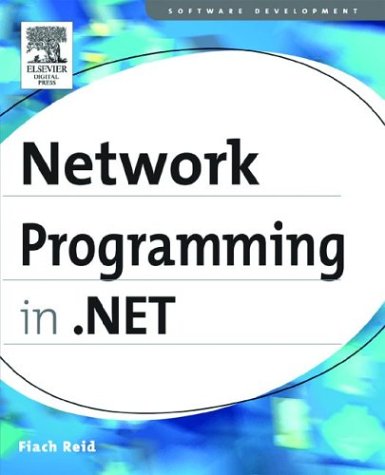
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EventItfInfo.cs
- AdornedElementPlaceholder.cs
- URI.cs
- WebPartAddingEventArgs.cs
- ReferenceService.cs
- OracleDataReader.cs
- ParallelLoopState.cs
- BaseUriHelper.cs
- recordstatefactory.cs
- StringCollectionEditor.cs
- SeekableReadStream.cs
- ToolboxCategoryItems.cs
- StaticSiteMapProvider.cs
- ViewGenResults.cs
- ZipIOLocalFileBlock.cs
- Codec.cs
- RuntimeConfig.cs
- TriggerActionCollection.cs
- EntityDataSourceColumn.cs
- NullableDoubleSumAggregationOperator.cs
- XamlVector3DCollectionSerializer.cs
- ConvertBinder.cs
- DragDeltaEventArgs.cs
- cookie.cs
- ExceptionHandler.cs
- FileEnumerator.cs
- GlyphsSerializer.cs
- SafeHandles.cs
- CSharpCodeProvider.cs
- ToolTipService.cs
- ipaddressinformationcollection.cs
- RelationshipEndCollection.cs
- EventListenerClientSide.cs
- SettingsPropertyValueCollection.cs
- DbParameterHelper.cs
- cache.cs
- CodeAttributeDeclarationCollection.cs
- DynamicQueryableWrapper.cs
- NetworkStream.cs
- RunClient.cs
- GridEntryCollection.cs
- StorageTypeMapping.cs
- DbSetClause.cs
- ReferenceTypeElement.cs
- FormatConvertedBitmap.cs
- CompilerLocalReference.cs
- SaveFileDialog.cs
- FontCollection.cs
- WebConfigurationHost.cs
- SQLResource.cs
- DataGridItemCollection.cs
- ImageFormat.cs
- ResourceProviderFactory.cs
- TypeUsageBuilder.cs
- WorkflowTimerService.cs
- HtmlElementErrorEventArgs.cs
- Normalization.cs
- ToolboxComponentsCreatedEventArgs.cs
- XPathSingletonIterator.cs
- SecurityTokenInclusionMode.cs
- XamlVector3DCollectionSerializer.cs
- XmlSchemaValidationException.cs
- SelectingProviderEventArgs.cs
- InfiniteTimeSpanConverter.cs
- RelatedCurrencyManager.cs
- XmlDataSourceView.cs
- DataGridItemEventArgs.cs
- DataGridViewRowCollection.cs
- IgnorePropertiesAttribute.cs
- DesignerUtils.cs
- Propagator.ExtentPlaceholderCreator.cs
- ClientSettingsStore.cs
- _ListenerResponseStream.cs
- TransferRequestHandler.cs
- CommandHelpers.cs
- CodeDomExtensionMethods.cs
- ScriptingScriptResourceHandlerSection.cs
- DBConnectionString.cs
- GradientStop.cs
- FixedDSBuilder.cs
- DateTimeConverter.cs
- ImportCatalogPart.cs
- KeyGestureValueSerializer.cs
- GregorianCalendar.cs
- ThicknessAnimation.cs
- COM2TypeInfoProcessor.cs
- ConstraintConverter.cs
- PropertyOverridesTypeEditor.cs
- FontSizeConverter.cs
- Size.cs
- Grant.cs
- ReferencedCategoriesDocument.cs
- RemoteCryptoRsaServiceProvider.cs
- IntPtr.cs
- RecordConverter.cs
- FormViewPageEventArgs.cs
- XPathDescendantIterator.cs
- DesignTimeTemplateParser.cs
- _ConnectionGroup.cs
- XmlSortKey.cs