Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Data / System / Data / SqlClient / SqlConnectionStringBuilder.cs / 1305376 / SqlConnectionStringBuilder.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Data.Common; using System.Diagnostics; using System.Globalization; using System.Runtime.Serialization; using System.Security.Permissions; using System.Text; namespace System.Data.SqlClient { [DefaultProperty("DataSource")] [System.ComponentModel.TypeConverterAttribute(typeof(SqlConnectionStringBuilder.SqlConnectionStringBuilderConverter))] public sealed class SqlConnectionStringBuilder : DbConnectionStringBuilder { private enum Keywords { // specific ordering for ConnectionString output construction // NamedConnection, DataSource, FailoverPartner, AttachDBFilename, InitialCatalog, IntegratedSecurity, PersistSecurityInfo, UserID, Password, Enlist, Pooling, MinPoolSize, MaxPoolSize, AsynchronousProcessing, ConnectionReset, MultipleActiveResultSets, Replication, ConnectTimeout, Encrypt, TrustServerCertificate, LoadBalanceTimeout, NetworkLibrary, PacketSize, TypeSystemVersion, ApplicationName, CurrentLanguage, WorkstationID, UserInstance, ContextConnection, TransactionBinding, // keep the count value last KeywordsCount } internal const int KeywordsCount = (int)Keywords.KeywordsCount; private static readonly string[] _validKeywords; private static readonly Dictionary_keywords; private string _applicationName = DbConnectionStringDefaults.ApplicationName; private string _attachDBFilename = DbConnectionStringDefaults.AttachDBFilename; private string _currentLanguage = DbConnectionStringDefaults.CurrentLanguage; private string _dataSource = DbConnectionStringDefaults.DataSource; private string _failoverPartner = DbConnectionStringDefaults.FailoverPartner; private string _initialCatalog = DbConnectionStringDefaults.InitialCatalog; // private string _namedConnection = DbConnectionStringDefaults.NamedConnection; private string _networkLibrary = DbConnectionStringDefaults.NetworkLibrary; private string _password = DbConnectionStringDefaults.Password; private string _transactionBinding = DbConnectionStringDefaults.TransactionBinding; private string _typeSystemVersion = DbConnectionStringDefaults.TypeSystemVersion; private string _userID = DbConnectionStringDefaults.UserID; private string _workstationID = DbConnectionStringDefaults.WorkstationID; private int _connectTimeout = DbConnectionStringDefaults.ConnectTimeout; private int _loadBalanceTimeout = DbConnectionStringDefaults.LoadBalanceTimeout; private int _maxPoolSize = DbConnectionStringDefaults.MaxPoolSize; private int _minPoolSize = DbConnectionStringDefaults.MinPoolSize; private int _packetSize = DbConnectionStringDefaults.PacketSize; private bool _asynchronousProcessing = DbConnectionStringDefaults.AsynchronousProcessing; private bool _connectionReset = DbConnectionStringDefaults.ConnectionReset; private bool _contextConnection = DbConnectionStringDefaults.ContextConnection; private bool _encrypt = DbConnectionStringDefaults.Encrypt; private bool _trustServerCertificate = DbConnectionStringDefaults.TrustServerCertificate; private bool _enlist = DbConnectionStringDefaults.Enlist; private bool _integratedSecurity = DbConnectionStringDefaults.IntegratedSecurity; private bool _multipleActiveResultSets = DbConnectionStringDefaults.MultipleActiveResultSets; private bool _persistSecurityInfo = DbConnectionStringDefaults.PersistSecurityInfo; private bool _pooling = DbConnectionStringDefaults.Pooling; private bool _replication = DbConnectionStringDefaults.Replication; private bool _userInstance = DbConnectionStringDefaults.UserInstance; static SqlConnectionStringBuilder() { string[] validKeywords = new string[KeywordsCount]; validKeywords[(int)Keywords.ApplicationName] = DbConnectionStringKeywords.ApplicationName; validKeywords[(int)Keywords.AsynchronousProcessing] = DbConnectionStringKeywords.AsynchronousProcessing; validKeywords[(int)Keywords.AttachDBFilename] = DbConnectionStringKeywords.AttachDBFilename; validKeywords[(int)Keywords.ConnectionReset] = DbConnectionStringKeywords.ConnectionReset; validKeywords[(int)Keywords.ContextConnection] = DbConnectionStringKeywords.ContextConnection; validKeywords[(int)Keywords.ConnectTimeout] = DbConnectionStringKeywords.ConnectTimeout; validKeywords[(int)Keywords.CurrentLanguage] = DbConnectionStringKeywords.CurrentLanguage; validKeywords[(int)Keywords.DataSource] = DbConnectionStringKeywords.DataSource; validKeywords[(int)Keywords.Encrypt] = DbConnectionStringKeywords.Encrypt; validKeywords[(int)Keywords.Enlist] = DbConnectionStringKeywords.Enlist; validKeywords[(int)Keywords.FailoverPartner] = DbConnectionStringKeywords.FailoverPartner; validKeywords[(int)Keywords.InitialCatalog] = DbConnectionStringKeywords.InitialCatalog; validKeywords[(int)Keywords.IntegratedSecurity] = DbConnectionStringKeywords.IntegratedSecurity; validKeywords[(int)Keywords.LoadBalanceTimeout] = DbConnectionStringKeywords.LoadBalanceTimeout; validKeywords[(int)Keywords.MaxPoolSize] = DbConnectionStringKeywords.MaxPoolSize; validKeywords[(int)Keywords.MinPoolSize] = DbConnectionStringKeywords.MinPoolSize; validKeywords[(int)Keywords.MultipleActiveResultSets] = DbConnectionStringKeywords.MultipleActiveResultSets; // validKeywords[(int)Keywords.NamedConnection] = DbConnectionStringKeywords.NamedConnection; validKeywords[(int)Keywords.NetworkLibrary] = DbConnectionStringKeywords.NetworkLibrary; validKeywords[(int)Keywords.PacketSize] = DbConnectionStringKeywords.PacketSize; validKeywords[(int)Keywords.Password] = DbConnectionStringKeywords.Password; validKeywords[(int)Keywords.PersistSecurityInfo] = DbConnectionStringKeywords.PersistSecurityInfo; validKeywords[(int)Keywords.Pooling] = DbConnectionStringKeywords.Pooling; validKeywords[(int)Keywords.Replication] = DbConnectionStringKeywords.Replication; validKeywords[(int)Keywords.TransactionBinding] = DbConnectionStringKeywords.TransactionBinding; validKeywords[(int)Keywords.TrustServerCertificate] = DbConnectionStringKeywords.TrustServerCertificate; validKeywords[(int)Keywords.TypeSystemVersion] = DbConnectionStringKeywords.TypeSystemVersion; validKeywords[(int)Keywords.UserID] = DbConnectionStringKeywords.UserID; validKeywords[(int)Keywords.UserInstance] = DbConnectionStringKeywords.UserInstance; validKeywords[(int)Keywords.WorkstationID] = DbConnectionStringKeywords.WorkstationID; _validKeywords = validKeywords; Dictionary hash = new Dictionary (50, StringComparer.OrdinalIgnoreCase); hash.Add(DbConnectionStringKeywords.ApplicationName, Keywords.ApplicationName); hash.Add(DbConnectionStringKeywords.AsynchronousProcessing, Keywords.AsynchronousProcessing); hash.Add(DbConnectionStringKeywords.AttachDBFilename, Keywords.AttachDBFilename); hash.Add(DbConnectionStringKeywords.ConnectTimeout, Keywords.ConnectTimeout); hash.Add(DbConnectionStringKeywords.ConnectionReset, Keywords.ConnectionReset); hash.Add(DbConnectionStringKeywords.ContextConnection, Keywords.ContextConnection); hash.Add(DbConnectionStringKeywords.CurrentLanguage, Keywords.CurrentLanguage); hash.Add(DbConnectionStringKeywords.DataSource, Keywords.DataSource); hash.Add(DbConnectionStringKeywords.Encrypt, Keywords.Encrypt); hash.Add(DbConnectionStringKeywords.Enlist, Keywords.Enlist); hash.Add(DbConnectionStringKeywords.FailoverPartner, Keywords.FailoverPartner); hash.Add(DbConnectionStringKeywords.InitialCatalog, Keywords.InitialCatalog); hash.Add(DbConnectionStringKeywords.IntegratedSecurity, Keywords.IntegratedSecurity); hash.Add(DbConnectionStringKeywords.LoadBalanceTimeout, Keywords.LoadBalanceTimeout); hash.Add(DbConnectionStringKeywords.MultipleActiveResultSets, Keywords.MultipleActiveResultSets); hash.Add(DbConnectionStringKeywords.MaxPoolSize, Keywords.MaxPoolSize); hash.Add(DbConnectionStringKeywords.MinPoolSize, Keywords.MinPoolSize); // hash.Add(DbConnectionStringKeywords.NamedConnection, Keywords.NamedConnection); hash.Add(DbConnectionStringKeywords.NetworkLibrary, Keywords.NetworkLibrary); hash.Add(DbConnectionStringKeywords.PacketSize, Keywords.PacketSize); hash.Add(DbConnectionStringKeywords.Password, Keywords.Password); hash.Add(DbConnectionStringKeywords.PersistSecurityInfo, Keywords.PersistSecurityInfo); hash.Add(DbConnectionStringKeywords.Pooling, Keywords.Pooling); hash.Add(DbConnectionStringKeywords.Replication, Keywords.Replication); hash.Add(DbConnectionStringKeywords.TransactionBinding, Keywords.TransactionBinding); hash.Add(DbConnectionStringKeywords.TrustServerCertificate, Keywords.TrustServerCertificate); hash.Add(DbConnectionStringKeywords.TypeSystemVersion, Keywords.TypeSystemVersion); hash.Add(DbConnectionStringKeywords.UserID, Keywords.UserID); hash.Add(DbConnectionStringKeywords.UserInstance, Keywords.UserInstance); hash.Add(DbConnectionStringKeywords.WorkstationID, Keywords.WorkstationID); hash.Add(DbConnectionStringSynonyms.APP, Keywords.ApplicationName); hash.Add(DbConnectionStringSynonyms.Async, Keywords.AsynchronousProcessing); hash.Add(DbConnectionStringSynonyms.EXTENDEDPROPERTIES, Keywords.AttachDBFilename); hash.Add(DbConnectionStringSynonyms.INITIALFILENAME, Keywords.AttachDBFilename); hash.Add(DbConnectionStringSynonyms.CONNECTIONTIMEOUT, Keywords.ConnectTimeout); hash.Add(DbConnectionStringSynonyms.TIMEOUT, Keywords.ConnectTimeout); hash.Add(DbConnectionStringSynonyms.LANGUAGE, Keywords.CurrentLanguage); hash.Add(DbConnectionStringSynonyms.ADDR, Keywords.DataSource); hash.Add(DbConnectionStringSynonyms.ADDRESS, Keywords.DataSource); hash.Add(DbConnectionStringSynonyms.NETWORKADDRESS, Keywords.DataSource); hash.Add(DbConnectionStringSynonyms.SERVER, Keywords.DataSource); hash.Add(DbConnectionStringSynonyms.DATABASE, Keywords.InitialCatalog); hash.Add(DbConnectionStringSynonyms.TRUSTEDCONNECTION, Keywords.IntegratedSecurity); hash.Add(DbConnectionStringSynonyms.ConnectionLifetime, Keywords.LoadBalanceTimeout); hash.Add(DbConnectionStringSynonyms.NET, Keywords.NetworkLibrary); hash.Add(DbConnectionStringSynonyms.NETWORK, Keywords.NetworkLibrary); hash.Add(DbConnectionStringSynonyms.Pwd, Keywords.Password); hash.Add(DbConnectionStringSynonyms.PERSISTSECURITYINFO, Keywords.PersistSecurityInfo); hash.Add(DbConnectionStringSynonyms.UID, Keywords.UserID); hash.Add(DbConnectionStringSynonyms.User, Keywords.UserID); hash.Add(DbConnectionStringSynonyms.WSID, Keywords.WorkstationID); Debug.Assert((KeywordsCount + SqlConnectionString.SynonymCount) == hash.Count, "initial expected size is incorrect"); _keywords = hash; } public SqlConnectionStringBuilder() : this((string)null) { } public SqlConnectionStringBuilder(string connectionString) : base() { if (!ADP.IsEmpty(connectionString)) { ConnectionString = connectionString; } } public override object this[string keyword] { get { Keywords index = GetIndex(keyword); return GetAt(index); } set { Bid.Trace(" keyword='%ls'\n", keyword); if (null != value) { Keywords index = GetIndex(keyword); switch(index) { case Keywords.ApplicationName: ApplicationName = ConvertToString(value); break; case Keywords.AttachDBFilename: AttachDBFilename = ConvertToString(value); break; case Keywords.CurrentLanguage: CurrentLanguage = ConvertToString(value); break; case Keywords.DataSource: DataSource = ConvertToString(value); break; case Keywords.FailoverPartner: FailoverPartner = ConvertToString(value); break; case Keywords.InitialCatalog: InitialCatalog = ConvertToString(value); break; // case Keywords.NamedConnection: NamedConnection = ConvertToString(value); break; case Keywords.NetworkLibrary: NetworkLibrary = ConvertToString(value); break; case Keywords.Password: Password = ConvertToString(value); break; case Keywords.UserID: UserID = ConvertToString(value); break; case Keywords.TransactionBinding: TransactionBinding = ConvertToString(value); break; case Keywords.TypeSystemVersion: TypeSystemVersion = ConvertToString(value); break; case Keywords.WorkstationID: WorkstationID = ConvertToString(value); break; case Keywords.ConnectTimeout: ConnectTimeout = ConvertToInt32(value); break; case Keywords.LoadBalanceTimeout: LoadBalanceTimeout = ConvertToInt32(value); break; case Keywords.MaxPoolSize: MaxPoolSize = ConvertToInt32(value); break; case Keywords.MinPoolSize: MinPoolSize = ConvertToInt32(value); break; case Keywords.PacketSize: PacketSize = ConvertToInt32(value); break; case Keywords.IntegratedSecurity: IntegratedSecurity = ConvertToIntegratedSecurity(value); break; case Keywords.AsynchronousProcessing: AsynchronousProcessing = ConvertToBoolean(value); break; #pragma warning disable 618 // Obsolete ConnectionReset case Keywords.ConnectionReset: ConnectionReset = ConvertToBoolean(value); break; #pragma warning restore 618 case Keywords.ContextConnection: ContextConnection = ConvertToBoolean(value); break; case Keywords.Encrypt: Encrypt = ConvertToBoolean(value); break; case Keywords.TrustServerCertificate: TrustServerCertificate = ConvertToBoolean(value); break; case Keywords.Enlist: Enlist = ConvertToBoolean(value); break; case Keywords.MultipleActiveResultSets: MultipleActiveResultSets = ConvertToBoolean(value); break; case Keywords.PersistSecurityInfo: PersistSecurityInfo = ConvertToBoolean(value); break; case Keywords.Pooling: Pooling = ConvertToBoolean(value); break; case Keywords.Replication: Replication = ConvertToBoolean(value); break; case Keywords.UserInstance: UserInstance = ConvertToBoolean(value); break; default: Debug.Assert(false, "unexpected keyword"); throw ADP.KeywordNotSupported(keyword); } } else { Remove(keyword); } } } [DisplayName(DbConnectionStringKeywords.ApplicationName)] [ResCategoryAttribute(Res.DataCategory_Context)] [ResDescriptionAttribute(Res.DbConnectionString_ApplicationName)] [RefreshPropertiesAttribute(RefreshProperties.All)] public string ApplicationName { get { return _applicationName; } set { SetValue(DbConnectionStringKeywords.ApplicationName, value); _applicationName = value; } } [DisplayName(DbConnectionStringKeywords.AsynchronousProcessing)] [ResCategoryAttribute(Res.DataCategory_Initialization)] [ResDescriptionAttribute(Res.DbConnectionString_AsynchronousProcessing)] [RefreshPropertiesAttribute(RefreshProperties.All)] public bool AsynchronousProcessing { get { return _asynchronousProcessing; } set { SetValue(DbConnectionStringKeywords.AsynchronousProcessing, value); _asynchronousProcessing = value; } } [DisplayName(DbConnectionStringKeywords.AttachDBFilename)] [ResCategoryAttribute(Res.DataCategory_Source)] [ResDescriptionAttribute(Res.DbConnectionString_AttachDBFilename)] [RefreshPropertiesAttribute(RefreshProperties.All)] // [Editor("System.Windows.Forms.Design.FileNameEditor, " + AssemblyRef.SystemDesign, "System.Drawing.Design.UITypeEditor, " + AssemblyRef.SystemDrawing)] public string AttachDBFilename { get { return _attachDBFilename; } set { SetValue(DbConnectionStringKeywords.AttachDBFilename, value); _attachDBFilename = value; } } [Browsable(false)] [DisplayName(DbConnectionStringKeywords.ConnectionReset)] [Obsolete("ConnectionReset has been deprecated. SqlConnection will ignore the 'connection reset' keyword and always reset the connection")] // SQLPT 41700 [ResCategoryAttribute(Res.DataCategory_Pooling)] [ResDescriptionAttribute(Res.DbConnectionString_ConnectionReset)] [RefreshPropertiesAttribute(RefreshProperties.All)] public bool ConnectionReset { get { return _connectionReset; } set { SetValue(DbConnectionStringKeywords.ConnectionReset, value); _connectionReset = value; } } [DisplayName(DbConnectionStringKeywords.ContextConnection)] [ResCategoryAttribute(Res.DataCategory_Source)] [ResDescriptionAttribute(Res.DbConnectionString_ContextConnection)] [RefreshPropertiesAttribute(RefreshProperties.All)] public bool ContextConnection { get { return _contextConnection; } set { SetValue(DbConnectionStringKeywords.ContextConnection, value); _contextConnection = value; } } [DisplayName(DbConnectionStringKeywords.ConnectTimeout)] [ResCategoryAttribute(Res.DataCategory_Initialization)] [ResDescriptionAttribute(Res.DbConnectionString_ConnectTimeout)] [RefreshPropertiesAttribute(RefreshProperties.All)] public int ConnectTimeout { get { return _connectTimeout; } set { if (value < 0) { throw ADP.InvalidConnectionOptionValue(DbConnectionStringKeywords.ConnectTimeout); } SetValue(DbConnectionStringKeywords.ConnectTimeout, value); _connectTimeout = value; } } [DisplayName(DbConnectionStringKeywords.CurrentLanguage)] [ResCategoryAttribute(Res.DataCategory_Initialization)] [ResDescriptionAttribute(Res.DbConnectionString_CurrentLanguage)] [RefreshPropertiesAttribute(RefreshProperties.All)] public string CurrentLanguage { get { return _currentLanguage; } set { SetValue(DbConnectionStringKeywords.CurrentLanguage, value); _currentLanguage = value; } } [DisplayName(DbConnectionStringKeywords.DataSource)] [ResCategoryAttribute(Res.DataCategory_Source)] [ResDescriptionAttribute(Res.DbConnectionString_DataSource)] [RefreshProperties(RefreshProperties.All)] [TypeConverter(typeof(SqlDataSourceConverter))] public string DataSource { get { return _dataSource; } set { SetValue(DbConnectionStringKeywords.DataSource, value); _dataSource = value; } } [DisplayName(DbConnectionStringKeywords.Encrypt)] [ResCategoryAttribute(Res.DataCategory_Security)] [ResDescriptionAttribute(Res.DbConnectionString_Encrypt)] [RefreshPropertiesAttribute(RefreshProperties.All)] public bool Encrypt { get { return _encrypt; } set { SetValue(DbConnectionStringKeywords.Encrypt, value); _encrypt = value; } } [DisplayName(DbConnectionStringKeywords.TrustServerCertificate)] [ResCategoryAttribute(Res.DataCategory_Security)] [ResDescriptionAttribute(Res.DbConnectionString_TrustServerCertificate)] [RefreshPropertiesAttribute(RefreshProperties.All)] public bool TrustServerCertificate { get { return _trustServerCertificate; } set { SetValue(DbConnectionStringKeywords.TrustServerCertificate, value); _trustServerCertificate = value; } } [DisplayName(DbConnectionStringKeywords.Enlist)] [ResCategoryAttribute(Res.DataCategory_Pooling)] [ResDescriptionAttribute(Res.DbConnectionString_Enlist)] [RefreshPropertiesAttribute(RefreshProperties.All)] public bool Enlist { get { return _enlist; } set { SetValue(DbConnectionStringKeywords.Enlist, value); _enlist = value; } } [DisplayName(DbConnectionStringKeywords.FailoverPartner)] [ResCategoryAttribute(Res.DataCategory_Source)] [ResDescriptionAttribute(Res.DbConnectionString_FailoverPartner)] [RefreshPropertiesAttribute(RefreshProperties.All)] [TypeConverter(typeof(SqlDataSourceConverter))] public string FailoverPartner { get { return _failoverPartner; } set { SetValue(DbConnectionStringKeywords.FailoverPartner, value); _failoverPartner= value; } } [DisplayName(DbConnectionStringKeywords.InitialCatalog)] [ResCategoryAttribute(Res.DataCategory_Source)] [ResDescriptionAttribute(Res.DbConnectionString_InitialCatalog)] [RefreshPropertiesAttribute(RefreshProperties.All)] [TypeConverter(typeof(SqlInitialCatalogConverter))] public string InitialCatalog { get { return _initialCatalog; } set { SetValue(DbConnectionStringKeywords.InitialCatalog, value); _initialCatalog = value; } } [DisplayName(DbConnectionStringKeywords.IntegratedSecurity)] [ResCategoryAttribute(Res.DataCategory_Security)] [ResDescriptionAttribute(Res.DbConnectionString_IntegratedSecurity)] [RefreshPropertiesAttribute(RefreshProperties.All)] public bool IntegratedSecurity { get { return _integratedSecurity; } set { SetValue(DbConnectionStringKeywords.IntegratedSecurity, value); _integratedSecurity = value; } } [DisplayName(DbConnectionStringKeywords.LoadBalanceTimeout)] [ResCategoryAttribute(Res.DataCategory_Pooling)] [ResDescriptionAttribute(Res.DbConnectionString_LoadBalanceTimeout)] [RefreshPropertiesAttribute(RefreshProperties.All)] public int LoadBalanceTimeout { get { return _loadBalanceTimeout; } set { if (value < 0) { throw ADP.InvalidConnectionOptionValue(DbConnectionStringKeywords.LoadBalanceTimeout); } SetValue(DbConnectionStringKeywords.LoadBalanceTimeout, value); _loadBalanceTimeout = value; } } [DisplayName(DbConnectionStringKeywords.MaxPoolSize)] [ResCategoryAttribute(Res.DataCategory_Pooling)] [ResDescriptionAttribute(Res.DbConnectionString_MaxPoolSize)] [RefreshPropertiesAttribute(RefreshProperties.All)] public int MaxPoolSize { get { return _maxPoolSize; } set { if (value < 1) { throw ADP.InvalidConnectionOptionValue(DbConnectionStringKeywords.MaxPoolSize); } SetValue(DbConnectionStringKeywords.MaxPoolSize, value); _maxPoolSize = value; } } [DisplayName(DbConnectionStringKeywords.MinPoolSize)] [ResCategoryAttribute(Res.DataCategory_Pooling)] [ResDescriptionAttribute(Res.DbConnectionString_MinPoolSize)] [RefreshPropertiesAttribute(RefreshProperties.All)] public int MinPoolSize { get { return _minPoolSize; } set { if (value < 0) { throw ADP.InvalidConnectionOptionValue(DbConnectionStringKeywords.MinPoolSize); } SetValue(DbConnectionStringKeywords.MinPoolSize, value); _minPoolSize = value; } } [DisplayName(DbConnectionStringKeywords.MultipleActiveResultSets)] [ResCategoryAttribute(Res.DataCategory_Advanced)] [ResDescriptionAttribute(Res.DbConnectionString_MultipleActiveResultSets)] [RefreshPropertiesAttribute(RefreshProperties.All)] public bool MultipleActiveResultSets { get { return _multipleActiveResultSets; } set { SetValue(DbConnectionStringKeywords.MultipleActiveResultSets, value); _multipleActiveResultSets = value; } } /* [DisplayName(DbConnectionStringKeywords.NamedConnection)] [ResCategoryAttribute(Res.DataCategory_NamedConnectionString)] [ResDescriptionAttribute(Res.DbConnectionString_NamedConnection)] [RefreshPropertiesAttribute(RefreshProperties.All)] [TypeConverter(typeof(NamedConnectionStringConverter))] public string NamedConnection { get { return _namedConnection; } set { SetValue(DbConnectionStringKeywords.NamedConnection, value); _namedConnection = value; } } */ [DisplayName(DbConnectionStringKeywords.NetworkLibrary)] [ResCategoryAttribute(Res.DataCategory_Advanced)] [ResDescriptionAttribute(Res.DbConnectionString_NetworkLibrary)] [RefreshPropertiesAttribute(RefreshProperties.All)] [TypeConverter(typeof(NetworkLibraryConverter))] public string NetworkLibrary { get { return _networkLibrary; } set { if (null != value) { switch(value.Trim().ToLower(CultureInfo.InvariantCulture)) { case SqlConnectionString.NETLIB.AppleTalk: value = SqlConnectionString.NETLIB.AppleTalk; break; case SqlConnectionString.NETLIB.BanyanVines: value = SqlConnectionString.NETLIB.BanyanVines; break; case SqlConnectionString.NETLIB.IPXSPX: value = SqlConnectionString.NETLIB.IPXSPX; break; case SqlConnectionString.NETLIB.Multiprotocol: value = SqlConnectionString.NETLIB.Multiprotocol; break; case SqlConnectionString.NETLIB.NamedPipes: value = SqlConnectionString.NETLIB.NamedPipes; break; case SqlConnectionString.NETLIB.SharedMemory: value = SqlConnectionString.NETLIB.SharedMemory; break; case SqlConnectionString.NETLIB.TCPIP: value = SqlConnectionString.NETLIB.TCPIP; break; case SqlConnectionString.NETLIB.VIA: value = SqlConnectionString.NETLIB.VIA; break; default: throw ADP.InvalidConnectionOptionValue(DbConnectionStringKeywords.NetworkLibrary); } } SetValue(DbConnectionStringKeywords.NetworkLibrary, value); _networkLibrary = value; } } [DisplayName(DbConnectionStringKeywords.PacketSize)] [ResCategoryAttribute(Res.DataCategory_Advanced)] [ResDescriptionAttribute(Res.DbConnectionString_PacketSize)] [RefreshPropertiesAttribute(RefreshProperties.All)] public int PacketSize { get { return _packetSize; } set { if ((value < TdsEnums.MIN_PACKET_SIZE) || (TdsEnums.MAX_PACKET_SIZE < value)) { throw SQL.InvalidPacketSizeValue(); } SetValue(DbConnectionStringKeywords.PacketSize, value); _packetSize = value; } } [DisplayName(DbConnectionStringKeywords.Password)] [PasswordPropertyTextAttribute(true)] [ResCategoryAttribute(Res.DataCategory_Security)] [ResDescriptionAttribute(Res.DbConnectionString_Password)] [RefreshPropertiesAttribute(RefreshProperties.All)] public string Password { get { return _password; } set { SetValue(DbConnectionStringKeywords.Password, value); _password = value; } } [DisplayName(DbConnectionStringKeywords.PersistSecurityInfo)] [ResCategoryAttribute(Res.DataCategory_Security)] [ResDescriptionAttribute(Res.DbConnectionString_PersistSecurityInfo)] [RefreshPropertiesAttribute(RefreshProperties.All)] public bool PersistSecurityInfo { get { return _persistSecurityInfo; } set { SetValue(DbConnectionStringKeywords.PersistSecurityInfo, value); _persistSecurityInfo = value; } } [DisplayName(DbConnectionStringKeywords.Pooling)] [ResCategoryAttribute(Res.DataCategory_Pooling)] [ResDescriptionAttribute(Res.DbConnectionString_Pooling)] [RefreshPropertiesAttribute(RefreshProperties.All)] public bool Pooling { get { return _pooling; } set { SetValue(DbConnectionStringKeywords.Pooling, value); _pooling = value; } } [DisplayName(DbConnectionStringKeywords.Replication)] [ResCategoryAttribute(Res.DataCategory_Replication)] [ResDescriptionAttribute(Res.DbConnectionString_Replication )] [RefreshPropertiesAttribute(RefreshProperties.All)] public bool Replication { get { return _replication; } set { SetValue(DbConnectionStringKeywords.Replication, value); _replication = value; } } [DisplayName(DbConnectionStringKeywords.TransactionBinding)] [ResCategoryAttribute(Res.DataCategory_Advanced)] [ResDescriptionAttribute(Res.DbConnectionString_TransactionBinding)] [RefreshPropertiesAttribute(RefreshProperties.All)] public string TransactionBinding { get { return _transactionBinding; } set { SetValue(DbConnectionStringKeywords.TransactionBinding, value); _transactionBinding = value; } } [DisplayName(DbConnectionStringKeywords.TypeSystemVersion)] [ResCategoryAttribute(Res.DataCategory_Advanced)] [ResDescriptionAttribute(Res.DbConnectionString_TypeSystemVersion)] [RefreshPropertiesAttribute(RefreshProperties.All)] public string TypeSystemVersion { get { return _typeSystemVersion; } set { SetValue(DbConnectionStringKeywords.TypeSystemVersion, value); _typeSystemVersion = value; } } [DisplayName(DbConnectionStringKeywords.UserID)] [ResCategoryAttribute(Res.DataCategory_Security)] [ResDescriptionAttribute(Res.DbConnectionString_UserID)] [RefreshPropertiesAttribute(RefreshProperties.All)] public string UserID { get { return _userID; } set { SetValue(DbConnectionStringKeywords.UserID, value); _userID = value; } } [DisplayName(DbConnectionStringKeywords.UserInstance)] [ResCategoryAttribute(Res.DataCategory_Source)] [ResDescriptionAttribute(Res.DbConnectionString_UserInstance)] [RefreshPropertiesAttribute(RefreshProperties.All)] public bool UserInstance { get { return _userInstance; } set { SetValue(DbConnectionStringKeywords.UserInstance, value); _userInstance = value; } } [DisplayName(DbConnectionStringKeywords.WorkstationID)] [ResCategoryAttribute(Res.DataCategory_Context)] [ResDescriptionAttribute(Res.DbConnectionString_WorkstationID)] [RefreshPropertiesAttribute(RefreshProperties.All)] public string WorkstationID { get { return _workstationID; } set { SetValue(DbConnectionStringKeywords.WorkstationID, value); _workstationID = value; } } public override bool IsFixedSize { get { return true; } } public override ICollection Keys { get { return new System.Data.Common.ReadOnlyCollection (_validKeywords); } } public override ICollection Values { get { // written this way so if the ordering of Keywords & _validKeywords changes // this is one less place to maintain object[] values = new object[_validKeywords.Length]; for(int i = 0; i < values.Length; ++i) { values[i] = GetAt((Keywords)i); } return new System.Data.Common.ReadOnlyCollection
Link Menu
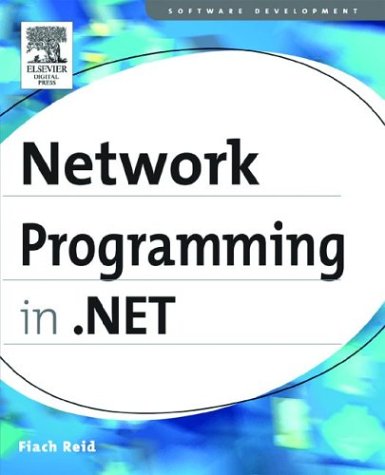
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RowBinding.cs
- SQLDecimalStorage.cs
- MergeLocalizationDirectives.cs
- OpenFileDialog.cs
- AsyncResult.cs
- XmlNodeWriter.cs
- BitmapMetadata.cs
- ReadOnlyObservableCollection.cs
- ToolBarTray.cs
- InputElement.cs
- CoreChannel.cs
- ManifestResourceInfo.cs
- SwitchDesigner.xaml.cs
- TypeBuilderInstantiation.cs
- CheckBox.cs
- ColorAnimation.cs
- InvalidComObjectException.cs
- CompilerError.cs
- Cursor.cs
- SQLMembershipProvider.cs
- SqlBooleanizer.cs
- CustomValidator.cs
- ListControl.cs
- CodeGenerationManager.cs
- HttpCapabilitiesSectionHandler.cs
- SafeNativeMethods.cs
- ProvidersHelper.cs
- LineBreakRecord.cs
- ForeignConstraint.cs
- CompositeScriptReference.cs
- DesignerView.Commands.cs
- GridViewCommandEventArgs.cs
- VirtualDirectoryMapping.cs
- StaticContext.cs
- StatusBar.cs
- ImageListStreamer.cs
- XmlSignificantWhitespace.cs
- SQLCharsStorage.cs
- ComponentGlyph.cs
- DiscoveryInnerClientManaged11.cs
- TriggerBase.cs
- LabelExpression.cs
- RegexInterpreter.cs
- ToolStripDropDown.cs
- BrowsableAttribute.cs
- DataSourceDesigner.cs
- ActivityInfo.cs
- cache.cs
- path.cs
- EventSource.cs
- ApplyHostConfigurationBehavior.cs
- InvalidCardException.cs
- UnknownMessageReceivedEventArgs.cs
- InstanceDataCollection.cs
- ImageBrush.cs
- NodeFunctions.cs
- QueryableFilterRepeater.cs
- ToolStripItemRenderEventArgs.cs
- SafeNativeMethods.cs
- LazyTextWriterCreator.cs
- MarshalDirectiveException.cs
- NetSectionGroup.cs
- CreateUserWizard.cs
- ValueCollectionParameterReader.cs
- TextTreeDeleteContentUndoUnit.cs
- SafeSecurityHandles.cs
- Rijndael.cs
- WebPartDisplayModeCancelEventArgs.cs
- EntityDataSourceContainerNameConverter.cs
- UIPermission.cs
- Storyboard.cs
- XmlReaderSettings.cs
- SiteMapPath.cs
- mediaclock.cs
- FieldTemplateFactory.cs
- DbInsertCommandTree.cs
- PathSegmentCollection.cs
- ExpressionBinding.cs
- ArcSegment.cs
- HttpPostedFileBase.cs
- WebPartZone.cs
- RsaSecurityTokenAuthenticator.cs
- XmlDomTextWriter.cs
- SetterTriggerConditionValueConverter.cs
- ModuleBuilderData.cs
- WsatTransactionHeader.cs
- SmtpSection.cs
- BindingCompleteEventArgs.cs
- ParseHttpDate.cs
- ModelVisual3D.cs
- BitmapImage.cs
- ToolStripPanelRow.cs
- CFStream.cs
- XsltLoader.cs
- ReflectionHelper.cs
- ChannelTokenTypeConverter.cs
- CachedRequestParams.cs
- ListViewGroupItemCollection.cs
- EnumerableCollectionView.cs
- PipelineModuleStepContainer.cs