Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Markup / TreeBuilderXamlTranslator.cs / 1 / TreeBuilderXamlTranslator.cs
/****************************************************************************\ * * File: TreeBuilderXamlTranslator.cs * * Purpose: Class that builds a tree from XAML * * History: * 6/06/01: rogerg Created * 5/29/03: peterost Ported to wcp * 11/13/03: peterost Split from XamlTreeBuilder.cs * * Copyright (C) 2003 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Xml; using System.IO; using System.Windows; using System.Text; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Threading; using MS.Internal; using MS.Utility; #if PBTCOMPILER namespace MS.Internal.Markup #else namespace System.Windows.Markup #endif { ////// Handles overrides for case when XAML is being built to a tree /// instead of compiling to a file. /// internal class TreeBuilderXamlTranslator : XamlParser { #region Constructors internal TreeBuilderXamlTranslator( XamlTreeBuilder treeBuilder, ParserContext parserContext, Stream xamlStream, XamlParseMode parseMode) : base(parserContext, null /*bamlWriter*/, xamlStream, false /*multipleRoots*/) { _treeBuilder = treeBuilder; XamlParseMode = parseMode; } internal TreeBuilderXamlTranslator( XamlTreeBuilder treeBuilder, ParserContext parserContext, XmlReader reader, bool wrapWithMarkupCompatReader, XamlParseMode parseMode) : base (parserContext, null /*bamlWriter*/, reader, wrapWithMarkupCompatReader) { _treeBuilder = treeBuilder; XamlParseMode = parseMode; } #endregion Constructors #region Overrides ////// Called when parsing the literal content. /// public override void WriteLiteralContent(XamlLiteralContentNode xamlLiteralContentNode) { if (BamlRecordWriter != null) BamlRecordWriter.WriteLiteralContent(xamlLiteralContentNode); } ////// Write Start of an Element, which is a tag of the form / ////// /// This is overridden to support building object trees directly from Xaml. In order /// to do this we have to pass the reader stack to the serializer, so that the bamlreader /// has all the context information that it needs to continue reading /// public override void WriteElementStart(XamlElementStartNode xamlElementStartNode) { // If we have a serializer for this element's type, then use that // serializer rather than doing default serialization. // NOTE: We currently have faith that the serializer will return when // it is done with the subtree and leave everything in the correct // state. We may want to limit how much the called serializer can // read so that it is forced to return at the end of the subtree. if (xamlElementStartNode.SerializerType != null) { XamlSerializer serializer = XamlTypeMapper.CreateInstance(xamlElementStartNode.SerializerType) as XamlSerializer; if (serializer == null) { ThrowException(SRID.ParserNoSerializer, xamlElementStartNode.TypeFullName, xamlElementStartNode.LineNumber, xamlElementStartNode.LinePosition); } else { serializer.ConvertXamlToObject(TokenReader, StreamManager, BamlRecordWriter.ParserContext, xamlElementStartNode, TreeBuilder.RecordReader); } } else if (BamlRecordWriter != null) { BamlRecordWriter.WriteElementStart(xamlElementStartNode); } } ////// Override of the _Parse method /// If in Async mode then sit in loop and kick of async thread /// else call the base implementation /// internal override void _Parse() { bool moreXamlRecords = true; // to go async we must be in Async mode, have a BamlRecordWriter // and have a TreeBuilder, if these conditions aren't // met we are sychronous if (XamlParseMode == XamlParseMode.Asynchronous) { // since binder requires the tag even in async mode we first read // until we have a root to return to the caller. // check against the TreeBuilderRoot to make sure the TreeBuilder // is setup properly while (moreXamlRecords && (null == TreeBuilder.GetRoot())) { moreXamlRecords = ReadXaml(true); } // don't kick of a thread if read the entire thing if (moreXamlRecords) { // now start the async loop ThreadStart threadStart = new ThreadStart(ReadXamlAsync); Thread thread = new Thread(threadStart); thread.Start(); } } else { base._Parse(); } } ////// Used when an exception is thrown -- does shutdown on the parser and throws the exception. /// /// Exception internal override void ParseError(XamlParseException e) { CloseWriterStream(); TreeBuilder.XamlTreeBuildError(e); throw e; } ////// Called when the parse was cancelled by the designer or the user. /// internal override void ParseCancelled() { CloseWriterStream(); TreeBuilder.XamlTreeBuildCancel(); base.ParseCancelled(); } ////// called when the parse has been completed successfully. /// internal override void ParseCompleted() { CloseWriterStream(); base.ParseCompleted(); } #endregion Overrides #region Methods ////// Helper function if we are going to a Reader/Writer stream closes the writer /// side. /// internal void CloseWriterStream() { // only close the BamlRecordWriter. (Rename to Root??) if (null != BamlRecordWriter) { if (BamlRecordWriter.BamlStream is WriterStream) { WriterStream writeStream = (WriterStream) BamlRecordWriter.BamlStream; writeStream.Close(); } else { Debug.Assert(false,"stream writing baml to is not a ReaderWriter Stream"); } } } ////// Helper function to ReadXaml async. /// Use this to catch exceptions on the async thread. TreeBuilder will handle /// on the main thread next chance it gets. /// void ReadXamlAsync() { try { ReadXaml(false); } catch (Exception e) { if (CriticalExceptions.IsCriticalException(e)) { throw; } else { // TreeBuilder::HandleAsyncQueueItem() will use this information // to build (& throw) a full parser exception. TreeBuilder.ParseException = e; } } } #endregion Methods #region Properties ////// TreeBuilder associated with this class /// XamlTreeBuilder TreeBuilder { get { return _treeBuilder; } } #endregion Properties #region Data XamlTreeBuilder _treeBuilder; #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /****************************************************************************\ * * File: TreeBuilderXamlTranslator.cs * * Purpose: Class that builds a tree from XAML * * History: * 6/06/01: rogerg Created * 5/29/03: peterost Ported to wcp * 11/13/03: peterost Split from XamlTreeBuilder.cs * * Copyright (C) 2003 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Xml; using System.IO; using System.Windows; using System.Text; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Threading; using MS.Internal; using MS.Utility; #if PBTCOMPILER namespace MS.Internal.Markup #else namespace System.Windows.Markup #endif { ////// Handles overrides for case when XAML is being built to a tree /// instead of compiling to a file. /// internal class TreeBuilderXamlTranslator : XamlParser { #region Constructors internal TreeBuilderXamlTranslator( XamlTreeBuilder treeBuilder, ParserContext parserContext, Stream xamlStream, XamlParseMode parseMode) : base(parserContext, null /*bamlWriter*/, xamlStream, false /*multipleRoots*/) { _treeBuilder = treeBuilder; XamlParseMode = parseMode; } internal TreeBuilderXamlTranslator( XamlTreeBuilder treeBuilder, ParserContext parserContext, XmlReader reader, bool wrapWithMarkupCompatReader, XamlParseMode parseMode) : base (parserContext, null /*bamlWriter*/, reader, wrapWithMarkupCompatReader) { _treeBuilder = treeBuilder; XamlParseMode = parseMode; } #endregion Constructors #region Overrides ////// Called when parsing the literal content. /// public override void WriteLiteralContent(XamlLiteralContentNode xamlLiteralContentNode) { if (BamlRecordWriter != null) BamlRecordWriter.WriteLiteralContent(xamlLiteralContentNode); } ////// Write Start of an Element, which is a tag of the form / ////// /// This is overridden to support building object trees directly from Xaml. In order /// to do this we have to pass the reader stack to the serializer, so that the bamlreader /// has all the context information that it needs to continue reading /// public override void WriteElementStart(XamlElementStartNode xamlElementStartNode) { // If we have a serializer for this element's type, then use that // serializer rather than doing default serialization. // NOTE: We currently have faith that the serializer will return when // it is done with the subtree and leave everything in the correct // state. We may want to limit how much the called serializer can // read so that it is forced to return at the end of the subtree. if (xamlElementStartNode.SerializerType != null) { XamlSerializer serializer = XamlTypeMapper.CreateInstance(xamlElementStartNode.SerializerType) as XamlSerializer; if (serializer == null) { ThrowException(SRID.ParserNoSerializer, xamlElementStartNode.TypeFullName, xamlElementStartNode.LineNumber, xamlElementStartNode.LinePosition); } else { serializer.ConvertXamlToObject(TokenReader, StreamManager, BamlRecordWriter.ParserContext, xamlElementStartNode, TreeBuilder.RecordReader); } } else if (BamlRecordWriter != null) { BamlRecordWriter.WriteElementStart(xamlElementStartNode); } } ////// Override of the _Parse method /// If in Async mode then sit in loop and kick of async thread /// else call the base implementation /// internal override void _Parse() { bool moreXamlRecords = true; // to go async we must be in Async mode, have a BamlRecordWriter // and have a TreeBuilder, if these conditions aren't // met we are sychronous if (XamlParseMode == XamlParseMode.Asynchronous) { // since binder requires the tag even in async mode we first read // until we have a root to return to the caller. // check against the TreeBuilderRoot to make sure the TreeBuilder // is setup properly while (moreXamlRecords && (null == TreeBuilder.GetRoot())) { moreXamlRecords = ReadXaml(true); } // don't kick of a thread if read the entire thing if (moreXamlRecords) { // now start the async loop ThreadStart threadStart = new ThreadStart(ReadXamlAsync); Thread thread = new Thread(threadStart); thread.Start(); } } else { base._Parse(); } } ////// Used when an exception is thrown -- does shutdown on the parser and throws the exception. /// /// Exception internal override void ParseError(XamlParseException e) { CloseWriterStream(); TreeBuilder.XamlTreeBuildError(e); throw e; } ////// Called when the parse was cancelled by the designer or the user. /// internal override void ParseCancelled() { CloseWriterStream(); TreeBuilder.XamlTreeBuildCancel(); base.ParseCancelled(); } ////// called when the parse has been completed successfully. /// internal override void ParseCompleted() { CloseWriterStream(); base.ParseCompleted(); } #endregion Overrides #region Methods ////// Helper function if we are going to a Reader/Writer stream closes the writer /// side. /// internal void CloseWriterStream() { // only close the BamlRecordWriter. (Rename to Root??) if (null != BamlRecordWriter) { if (BamlRecordWriter.BamlStream is WriterStream) { WriterStream writeStream = (WriterStream) BamlRecordWriter.BamlStream; writeStream.Close(); } else { Debug.Assert(false,"stream writing baml to is not a ReaderWriter Stream"); } } } ////// Helper function to ReadXaml async. /// Use this to catch exceptions on the async thread. TreeBuilder will handle /// on the main thread next chance it gets. /// void ReadXamlAsync() { try { ReadXaml(false); } catch (Exception e) { if (CriticalExceptions.IsCriticalException(e)) { throw; } else { // TreeBuilder::HandleAsyncQueueItem() will use this information // to build (& throw) a full parser exception. TreeBuilder.ParseException = e; } } } #endregion Methods #region Properties ////// TreeBuilder associated with this class /// XamlTreeBuilder TreeBuilder { get { return _treeBuilder; } } #endregion Properties #region Data XamlTreeBuilder _treeBuilder; #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
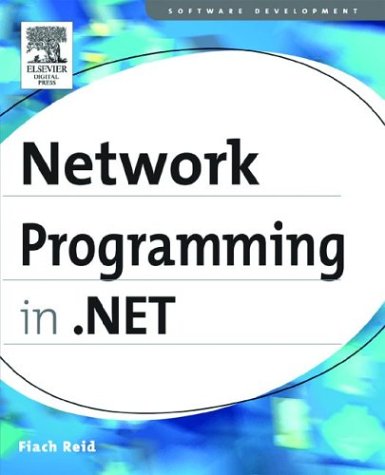
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- filewebrequest.cs
- MediaTimeline.cs
- CacheMemory.cs
- RectAnimationUsingKeyFrames.cs
- Win32.cs
- KerberosTicketHashIdentifierClause.cs
- PlaceHolder.cs
- Table.cs
- TripleDES.cs
- WebConfigurationFileMap.cs
- PolicyValidationException.cs
- MenuCommand.cs
- ValueChangedEventManager.cs
- SecurityDescriptor.cs
- SqlParameterizer.cs
- UpdatePanelControlTrigger.cs
- RIPEMD160.cs
- ADConnectionHelper.cs
- CompilerCollection.cs
- KeyInterop.cs
- FileDialogCustomPlaces.cs
- ConditionCollection.cs
- GatewayIPAddressInformationCollection.cs
- WindowsAuthenticationModule.cs
- AttributeProviderAttribute.cs
- IRCollection.cs
- datacache.cs
- ScriptReferenceEventArgs.cs
- SystemTcpStatistics.cs
- HierarchicalDataBoundControl.cs
- BufferBuilder.cs
- ProvideValueServiceProvider.cs
- OperatingSystem.cs
- ExceptionUtil.cs
- Validator.cs
- FormatPage.cs
- Schema.cs
- DataGridTable.cs
- NavigatingCancelEventArgs.cs
- CodeComment.cs
- UrlPath.cs
- XmlConverter.cs
- RadioButtonStandardAdapter.cs
- BinaryExpressionHelper.cs
- CallTemplateAction.cs
- RelationshipManager.cs
- StringArrayConverter.cs
- TableLayoutStyle.cs
- BaseCollection.cs
- DetailsViewPageEventArgs.cs
- EventlogProvider.cs
- SessionEndedEventArgs.cs
- Pens.cs
- ChannelBinding.cs
- IndexExpression.cs
- IOThreadScheduler.cs
- WindowsToolbarAsMenu.cs
- CodeValidator.cs
- DataView.cs
- DocumentGridPage.cs
- RolePrincipal.cs
- MissingFieldException.cs
- SafeProcessHandle.cs
- StrongNameKeyPair.cs
- DataSourceListEditor.cs
- RowSpanVector.cs
- TransactionFlowBindingElementImporter.cs
- autovalidator.cs
- TemplatePropertyEntry.cs
- Point3DKeyFrameCollection.cs
- ReferentialConstraint.cs
- translator.cs
- ToolStripSystemRenderer.cs
- Hashtable.cs
- StrongNameMembershipCondition.cs
- DataRowCollection.cs
- dbenumerator.cs
- SqlParameter.cs
- XXXOnTypeBuilderInstantiation.cs
- AddInServer.cs
- TriState.cs
- ObjectNotFoundException.cs
- arclist.cs
- _BufferOffsetSize.cs
- ByteRangeDownloader.cs
- SecurityPolicySection.cs
- SubordinateTransaction.cs
- remotingproxy.cs
- XsltCompileContext.cs
- HostedTransportConfigurationManager.cs
- TransactionBridge.cs
- DataShape.cs
- PathNode.cs
- Range.cs
- ToolStripItemClickedEventArgs.cs
- MethodBody.cs
- KeyNotFoundException.cs
- DrawingContextWalker.cs
- ControlBindingsConverter.cs
- SchemaDeclBase.cs