Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / BuildTasks / MS / Internal / MarkupCompiler / CompilationUnit.cs / 1 / CompilationUnit.cs
using System; using System.Security.Permissions; namespace MS.Internal { ////// The CompilationUnit class /// internal class CompilationUnit { #region Constructors ///constructor public CompilationUnit(string assemblyName, string language, string defaultNamespace, string [] fileList) { _assemblyName = assemblyName; _language = language; _fileList = fileList; _defaultNamespace = defaultNamespace; } #endregion Constructors #region Properties internal bool Pass2 { get { return _pass2; } set { _pass2 = value; } } ///Name of the assembly the package is compiled into public string AssemblyName { get { return _assemblyName; } } ///Name of the CLR language the package is compiled into public string Language { get { return _language; } } ///path to the project root public string SourcePath { get { return _sourcePath; } set { _sourcePath = value; } } ///Default CLR Namespace of the project public string DefaultNamespace { get { return _defaultNamespace; } } ///Application definition file (relative to SourcePath) public string ApplicationFile { get { return _applicationFile; } set { _applicationFile = value; } } ///A list of relative (to SourcePath) file path names comprising the package to be compiled public string [] FileList { get { return _fileList; } } #endregion Properties #region Private Data private bool _pass2 = false; private string _assemblyName = string.Empty; private string _language = string.Empty; private string _sourcePath = string.Empty; private string _defaultNamespace = string.Empty; private string _applicationFile = string.Empty; private string [] _fileList; #endregion Private Data } #region ErrorEvent ////// Delegate for the Error event. /// internal delegate void MarkupErrorEventHandler(Object sender, MarkupErrorEventArgs e); ////// Event args for the Error event /// internal class MarkupErrorEventArgs : EventArgs { #region Constructors ////// constructor /// internal MarkupErrorEventArgs(Exception e, int lineNum, int linePos, string fileName) { _e = e; _lineNum = lineNum; _linePos = linePos; _fileName = fileName; } #endregion Constructors #region Properties ////// The Error Message /// public Exception Exception { get { return _e; } } ////// The line number at which the compile Error occured /// public int LineNumber { get { return _lineNum; } } ////// The character position in the line at which the compile Error occured /// public int LinePosition { get { return _linePos; } } ////// The xaml file in which the compile Error occured /// public string FileName { get { return _fileName; } } #endregion Properties #region Private Data private int _lineNum; private int _linePos; private Exception _e; private string _fileName; #endregion Private Data } #endregion ErrorEvent #region SourceFileResolveEvent ////// Delegate for the SourceFileResolve Event. /// internal delegate void SourceFileResolveEventHandler(Object sender, SourceFileResolveEventArgs e); ////// Event args for the Error event /// internal class SourceFileResolveEventArgs: EventArgs { #region Constructors ////// constructor /// internal SourceFileResolveEventArgs(string filePath) { _sourceFileInfo = new SourceFileInfo(filePath); } #endregion Constructors #region Properties // // FileInfo // internal SourceFileInfo SourceFileInfo { get { return _sourceFileInfo; } } #endregion Properties #region Private Data private SourceFileInfo _sourceFileInfo; #endregion Private Data } #endregion SourceFileResolveEvent #region ReferenceAssembly //// Reference Assembly // Passed by CodeGenertation Task. // Consumed by Parser. // internal class ReferenceAssembly : MarshalByRefObject { #region Constructor //// Constructor // internal ReferenceAssembly() { _path = null; _assemblyName = null; } //// Constructor // // // internal ReferenceAssembly(string path, string assemblyname) { Path = path; AssemblyName = assemblyname; } #endregion Constructor #region Internal Properties //// The path for the assembly. // The path must end with "\", but not include any Assembly Name. // //internal string Path { get { return _path; } set { _path = value; } } // // AssemblyName without any Extension part. // //internal string AssemblyName { get { return _assemblyName; } set { _assemblyName = value;} } #endregion Internal Properties #region private fields private string _path; private string _assemblyName; #endregion private fields } #endregion ReferenceAssembly } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Security.Permissions; namespace MS.Internal { /// /// The CompilationUnit class /// internal class CompilationUnit { #region Constructors ///constructor public CompilationUnit(string assemblyName, string language, string defaultNamespace, string [] fileList) { _assemblyName = assemblyName; _language = language; _fileList = fileList; _defaultNamespace = defaultNamespace; } #endregion Constructors #region Properties internal bool Pass2 { get { return _pass2; } set { _pass2 = value; } } ///Name of the assembly the package is compiled into public string AssemblyName { get { return _assemblyName; } } ///Name of the CLR language the package is compiled into public string Language { get { return _language; } } ///path to the project root public string SourcePath { get { return _sourcePath; } set { _sourcePath = value; } } ///Default CLR Namespace of the project public string DefaultNamespace { get { return _defaultNamespace; } } ///Application definition file (relative to SourcePath) public string ApplicationFile { get { return _applicationFile; } set { _applicationFile = value; } } ///A list of relative (to SourcePath) file path names comprising the package to be compiled public string [] FileList { get { return _fileList; } } #endregion Properties #region Private Data private bool _pass2 = false; private string _assemblyName = string.Empty; private string _language = string.Empty; private string _sourcePath = string.Empty; private string _defaultNamespace = string.Empty; private string _applicationFile = string.Empty; private string [] _fileList; #endregion Private Data } #region ErrorEvent ////// Delegate for the Error event. /// internal delegate void MarkupErrorEventHandler(Object sender, MarkupErrorEventArgs e); ////// Event args for the Error event /// internal class MarkupErrorEventArgs : EventArgs { #region Constructors ////// constructor /// internal MarkupErrorEventArgs(Exception e, int lineNum, int linePos, string fileName) { _e = e; _lineNum = lineNum; _linePos = linePos; _fileName = fileName; } #endregion Constructors #region Properties ////// The Error Message /// public Exception Exception { get { return _e; } } ////// The line number at which the compile Error occured /// public int LineNumber { get { return _lineNum; } } ////// The character position in the line at which the compile Error occured /// public int LinePosition { get { return _linePos; } } ////// The xaml file in which the compile Error occured /// public string FileName { get { return _fileName; } } #endregion Properties #region Private Data private int _lineNum; private int _linePos; private Exception _e; private string _fileName; #endregion Private Data } #endregion ErrorEvent #region SourceFileResolveEvent ////// Delegate for the SourceFileResolve Event. /// internal delegate void SourceFileResolveEventHandler(Object sender, SourceFileResolveEventArgs e); ////// Event args for the Error event /// internal class SourceFileResolveEventArgs: EventArgs { #region Constructors ////// constructor /// internal SourceFileResolveEventArgs(string filePath) { _sourceFileInfo = new SourceFileInfo(filePath); } #endregion Constructors #region Properties // // FileInfo // internal SourceFileInfo SourceFileInfo { get { return _sourceFileInfo; } } #endregion Properties #region Private Data private SourceFileInfo _sourceFileInfo; #endregion Private Data } #endregion SourceFileResolveEvent #region ReferenceAssembly //// Reference Assembly // Passed by CodeGenertation Task. // Consumed by Parser. // internal class ReferenceAssembly : MarshalByRefObject { #region Constructor //// Constructor // internal ReferenceAssembly() { _path = null; _assemblyName = null; } //// Constructor // // // internal ReferenceAssembly(string path, string assemblyname) { Path = path; AssemblyName = assemblyname; } #endregion Constructor #region Internal Properties //// The path for the assembly. // The path must end with "\", but not include any Assembly Name. // //internal string Path { get { return _path; } set { _path = value; } } // // AssemblyName without any Extension part. // //internal string AssemblyName { get { return _assemblyName; } set { _assemblyName = value;} } #endregion Internal Properties #region private fields private string _path; private string _assemblyName; #endregion private fields } #endregion ReferenceAssembly } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
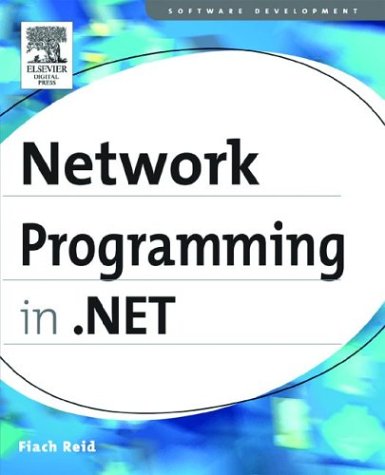
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextRunCache.cs
- GridViewCellAutomationPeer.cs
- NavigationProgressEventArgs.cs
- ScrollData.cs
- AsyncDataRequest.cs
- BindableAttribute.cs
- FocusManager.cs
- ResourceDefaultValueAttribute.cs
- KoreanCalendar.cs
- StorageConditionPropertyMapping.cs
- MaxMessageSizeStream.cs
- NonParentingControl.cs
- SafeNativeMethods.cs
- EventManager.cs
- HttpConfigurationSystem.cs
- StringArrayConverter.cs
- SymbolEqualComparer.cs
- WebPermission.cs
- DataGridRelationshipRow.cs
- StaticFileHandler.cs
- ResourceDefaultValueAttribute.cs
- ContentValidator.cs
- TreeNodeStyleCollection.cs
- TriggerCollection.cs
- TreeSet.cs
- DbConnectionPoolOptions.cs
- FilterQuery.cs
- CachedBitmap.cs
- TypeSystemProvider.cs
- PackagePart.cs
- ConfigurationStrings.cs
- SmiConnection.cs
- ColorAnimationUsingKeyFrames.cs
- ObjectStateEntryDbUpdatableDataRecord.cs
- oledbmetadatacolumnnames.cs
- ParserStack.cs
- _SpnDictionary.cs
- TypeContext.cs
- Model3D.cs
- Funcletizer.cs
- QueryTreeBuilder.cs
- EndpointAddress10.cs
- ColorInterpolationModeValidation.cs
- Error.cs
- OdbcError.cs
- BindUriHelper.cs
- SurrogateEncoder.cs
- WSUtilitySpecificationVersion.cs
- RectKeyFrameCollection.cs
- SystemColors.cs
- BinarySecretKeyIdentifierClause.cs
- BrowserCapabilitiesFactory.cs
- DateTime.cs
- SecurityUtils.cs
- MetaTable.cs
- EntityContainerEntitySet.cs
- StateManagedCollection.cs
- SystemInfo.cs
- CaseCqlBlock.cs
- NTAccount.cs
- CustomWebEventKey.cs
- RectangleGeometry.cs
- GC.cs
- WindowsUserNameSecurityTokenAuthenticator.cs
- StylusDownEventArgs.cs
- CompilerGeneratedAttribute.cs
- smtppermission.cs
- InternalConfigRoot.cs
- LambdaCompiler.Generated.cs
- CodeSnippetStatement.cs
- ProtocolsConfigurationEntry.cs
- X509CertificateCollection.cs
- ZoneMembershipCondition.cs
- ReadWriteSpinLock.cs
- _SslState.cs
- Soap12ProtocolImporter.cs
- ImageBrush.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- HtmlInputText.cs
- Utility.cs
- FrameworkContentElement.cs
- DesignerHost.cs
- TreeNodeStyleCollection.cs
- HtmlInputCheckBox.cs
- SystemParameters.cs
- RectAnimationClockResource.cs
- DbConnectionClosed.cs
- Latin1Encoding.cs
- OleDbParameter.cs
- mactripleDES.cs
- ConstantSlot.cs
- CellParagraph.cs
- EntityDataSourceDataSelectionPanel.designer.cs
- FormClosedEvent.cs
- LineServicesRun.cs
- ExceptionValidationRule.cs
- DbConnectionHelper.cs
- CacheRequest.cs
- DataGridHeaderBorder.cs
- TransformedBitmap.cs