Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / MS / Internal / PtsHost / CellParagraph.cs / 1 / CellParagraph.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: CellParagraph.cs // // Description: CellParagraph represents a single list item. // // History: // 06/01/2004 : grzegorz - moving from Avalon branch. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Security; using System.Windows; using System.Windows.Documents; using MS.Internal.PtsHost; using MS.Internal.Text; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace MS.Internal.PtsHost { // --------------------------------------------------------------------- // CellParagraph represents a single cell. // --------------------------------------------------------------------- internal sealed class CellParagraph : SubpageParagraph { // ------------------------------------------------------------------ // Constructor. // // element - Element associated with paragraph. // structuralCache - Content's structural cache // ----------------------------------------------------------------- internal CellParagraph(DependencyObject element, StructuralCache structuralCache) : base(element, structuralCache) { _isInterruptible = false; } ////// Table owner accessor /// internal TableCell Cell { get { return (TableCell)Element; } } ////// FormatCellFinite /// /// Table para client /// Subpage break record - not NULL if cell broken from previous page/column /// Footnote rejector /// Allow empty cell /// Table direction /// Available vertical space /// Formatting result /// Cell para client /// Break record for the current cell /// Ised vertical space ////// Critical - as this calls Critical function CellParaClient.FormatCellFinite. /// [SecurityCritical] internal void FormatCellFinite( TableParaClient tableParaClient, // IN: IntPtr pfsbrkcellIn, // IN: not NULL if cell broken from previous page/column IntPtr pfsFtnRejector, // IN: int fEmptyOK, // IN: uint fswdirTable, // IN: int dvrAvailable, // IN: out PTS.FSFMTR pfmtr, // OUT: out IntPtr ppfscell, // OUT: cell object out IntPtr pfsbrkcellOut, // OUT: break if cell does not fit in dvrAvailable out int dvrUsed) // OUT: height -- min height required { Debug.Assert(Cell.Index != -1 && Cell.ColumnIndex != -1, "Cell is not in a table"); CellParaClient cellParaClient; Size subpageSize; Debug.Assert(Cell.Table != null); cellParaClient = new CellParaClient(this, tableParaClient); subpageSize = new Size( cellParaClient.CalculateCellWidth(tableParaClient), Math.Max(TextDpi.FromTextDpi(dvrAvailable), 0)); cellParaClient.FormatCellFinite(subpageSize, pfsbrkcellIn, PTS.ToBoolean(fEmptyOK), fswdirTable, PTS.FSKSUPPRESSHARDBREAKBEFOREFIRSTPARA.fsksuppresshardbreakbeforefirstparaNone, out pfmtr, out dvrUsed, out pfsbrkcellOut); // initialize output parameters ppfscell = cellParaClient.Handle; if(pfmtr.kstop == PTS.FSFMTRKSTOP.fmtrNoProgressOutOfSpace) { cellParaClient.Dispose(); ppfscell = IntPtr.Zero; dvrUsed = 0; } if (dvrAvailable < dvrUsed) { if (PTS.ToBoolean(fEmptyOK)) { if (cellParaClient != null) { cellParaClient.Dispose(); } if (pfsbrkcellOut != IntPtr.Zero) { PTS.Validate(PTS.FsDestroySubpageBreakRecord(cellParaClient.PtsContext.Context, pfsbrkcellOut), cellParaClient.PtsContext); pfsbrkcellOut = IntPtr.Zero; } ppfscell = IntPtr.Zero; pfmtr.kstop = PTS.FSFMTRKSTOP.fmtrNoProgressOutOfSpace; dvrUsed = 0; } else { pfmtr.fForcedProgress = PTS.True; } } } ////// FormatCellBottomless /// /// Table para client /// Flow direction /// Formatting result /// Cell para client /// Height consumed internal void FormatCellBottomless( TableParaClient tableParaClient, // IN: uint fswdirTable, // IN: out PTS.FSFMTRBL fmtrbl, // OUT: out IntPtr ppfscell, // OUT: cell object out int dvrUsed) // OUT: height -- min height // required { Debug.Assert(Cell.Index != -1 && Cell.ColumnIndex != -1, "Cell is not in a table"); Debug.Assert(Cell.Table != null); CellParaClient cellParaClient = new CellParaClient(this, tableParaClient); cellParaClient.FormatCellBottomless(fswdirTable, cellParaClient.CalculateCellWidth(tableParaClient), out fmtrbl, out dvrUsed); // initialize output parameters ppfscell = cellParaClient.Handle; } ////// UpdateBottomlessCell /// /// Current cell para client /// Table para cleint /// Flow direction /// Formatting result /// Height consumed internal void UpdateBottomlessCell( CellParaClient cellParaClient, // IN: TableParaClient tableParaClient, // IN: uint fswdirTable, // IN: out PTS.FSFMTRBL fmtrbl, // OUT: out int dvrUsed) // OUT: height -- min height required { Debug.Assert(Cell.Index != -1 && Cell.ColumnIndex != -1, "Cell is not in a table"); Debug.Assert(Cell.Table != null); cellParaClient.UpdateBottomlessCell(fswdirTable, cellParaClient.CalculateCellWidth(tableParaClient), out fmtrbl, out dvrUsed); } ////// SetCellHeight /// /// Cell para client /// Table para client /// Break record if cell is broken /// Cell broken on this page/column /// Flow direction /// Actual height internal void SetCellHeight( CellParaClient cellParaClient, // IN: cell object TableParaClient tableParaClient, // table's para client IntPtr subpageBreakRecord, // not NULL if cell broken from previous page/column int fBrokenHere, // TRUE if cell broken on this page/column: no reformatting uint fswdirTable, int dvrActual) { cellParaClient.ArrangeHeight = TextDpi.FromTextDpi(dvrActual); } ////// UpdGetCellChange /// /// Indication that cell's width changed /// /// Change kind. One of: None, New, Inside /// internal void UpdGetCellChange( out int fWidthChanged, // OUT: out PTS.FSKCHANGE fskchCell) // OUT: { // calculate state change fWidthChanged = PTS.True; fskchCell = PTS.FSKCHANGE.fskchNew; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: CellParagraph.cs // // Description: CellParagraph represents a single list item. // // History: // 06/01/2004 : grzegorz - moving from Avalon branch. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Security; using System.Windows; using System.Windows.Documents; using MS.Internal.PtsHost; using MS.Internal.Text; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace MS.Internal.PtsHost { // --------------------------------------------------------------------- // CellParagraph represents a single cell. // --------------------------------------------------------------------- internal sealed class CellParagraph : SubpageParagraph { // ------------------------------------------------------------------ // Constructor. // // element - Element associated with paragraph. // structuralCache - Content's structural cache // ----------------------------------------------------------------- internal CellParagraph(DependencyObject element, StructuralCache structuralCache) : base(element, structuralCache) { _isInterruptible = false; } ////// Table owner accessor /// internal TableCell Cell { get { return (TableCell)Element; } } ////// FormatCellFinite /// /// Table para client /// Subpage break record - not NULL if cell broken from previous page/column /// Footnote rejector /// Allow empty cell /// Table direction /// Available vertical space /// Formatting result /// Cell para client /// Break record for the current cell /// Ised vertical space ////// Critical - as this calls Critical function CellParaClient.FormatCellFinite. /// [SecurityCritical] internal void FormatCellFinite( TableParaClient tableParaClient, // IN: IntPtr pfsbrkcellIn, // IN: not NULL if cell broken from previous page/column IntPtr pfsFtnRejector, // IN: int fEmptyOK, // IN: uint fswdirTable, // IN: int dvrAvailable, // IN: out PTS.FSFMTR pfmtr, // OUT: out IntPtr ppfscell, // OUT: cell object out IntPtr pfsbrkcellOut, // OUT: break if cell does not fit in dvrAvailable out int dvrUsed) // OUT: height -- min height required { Debug.Assert(Cell.Index != -1 && Cell.ColumnIndex != -1, "Cell is not in a table"); CellParaClient cellParaClient; Size subpageSize; Debug.Assert(Cell.Table != null); cellParaClient = new CellParaClient(this, tableParaClient); subpageSize = new Size( cellParaClient.CalculateCellWidth(tableParaClient), Math.Max(TextDpi.FromTextDpi(dvrAvailable), 0)); cellParaClient.FormatCellFinite(subpageSize, pfsbrkcellIn, PTS.ToBoolean(fEmptyOK), fswdirTable, PTS.FSKSUPPRESSHARDBREAKBEFOREFIRSTPARA.fsksuppresshardbreakbeforefirstparaNone, out pfmtr, out dvrUsed, out pfsbrkcellOut); // initialize output parameters ppfscell = cellParaClient.Handle; if(pfmtr.kstop == PTS.FSFMTRKSTOP.fmtrNoProgressOutOfSpace) { cellParaClient.Dispose(); ppfscell = IntPtr.Zero; dvrUsed = 0; } if (dvrAvailable < dvrUsed) { if (PTS.ToBoolean(fEmptyOK)) { if (cellParaClient != null) { cellParaClient.Dispose(); } if (pfsbrkcellOut != IntPtr.Zero) { PTS.Validate(PTS.FsDestroySubpageBreakRecord(cellParaClient.PtsContext.Context, pfsbrkcellOut), cellParaClient.PtsContext); pfsbrkcellOut = IntPtr.Zero; } ppfscell = IntPtr.Zero; pfmtr.kstop = PTS.FSFMTRKSTOP.fmtrNoProgressOutOfSpace; dvrUsed = 0; } else { pfmtr.fForcedProgress = PTS.True; } } } ////// FormatCellBottomless /// /// Table para client /// Flow direction /// Formatting result /// Cell para client /// Height consumed internal void FormatCellBottomless( TableParaClient tableParaClient, // IN: uint fswdirTable, // IN: out PTS.FSFMTRBL fmtrbl, // OUT: out IntPtr ppfscell, // OUT: cell object out int dvrUsed) // OUT: height -- min height // required { Debug.Assert(Cell.Index != -1 && Cell.ColumnIndex != -1, "Cell is not in a table"); Debug.Assert(Cell.Table != null); CellParaClient cellParaClient = new CellParaClient(this, tableParaClient); cellParaClient.FormatCellBottomless(fswdirTable, cellParaClient.CalculateCellWidth(tableParaClient), out fmtrbl, out dvrUsed); // initialize output parameters ppfscell = cellParaClient.Handle; } ////// UpdateBottomlessCell /// /// Current cell para client /// Table para cleint /// Flow direction /// Formatting result /// Height consumed internal void UpdateBottomlessCell( CellParaClient cellParaClient, // IN: TableParaClient tableParaClient, // IN: uint fswdirTable, // IN: out PTS.FSFMTRBL fmtrbl, // OUT: out int dvrUsed) // OUT: height -- min height required { Debug.Assert(Cell.Index != -1 && Cell.ColumnIndex != -1, "Cell is not in a table"); Debug.Assert(Cell.Table != null); cellParaClient.UpdateBottomlessCell(fswdirTable, cellParaClient.CalculateCellWidth(tableParaClient), out fmtrbl, out dvrUsed); } ////// SetCellHeight /// /// Cell para client /// Table para client /// Break record if cell is broken /// Cell broken on this page/column /// Flow direction /// Actual height internal void SetCellHeight( CellParaClient cellParaClient, // IN: cell object TableParaClient tableParaClient, // table's para client IntPtr subpageBreakRecord, // not NULL if cell broken from previous page/column int fBrokenHere, // TRUE if cell broken on this page/column: no reformatting uint fswdirTable, int dvrActual) { cellParaClient.ArrangeHeight = TextDpi.FromTextDpi(dvrActual); } ////// UpdGetCellChange /// /// Indication that cell's width changed /// /// Change kind. One of: None, New, Inside /// internal void UpdGetCellChange( out int fWidthChanged, // OUT: out PTS.FSKCHANGE fskchCell) // OUT: { // calculate state change fWidthChanged = PTS.True; fskchCell = PTS.FSKCHANGE.fskchNew; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
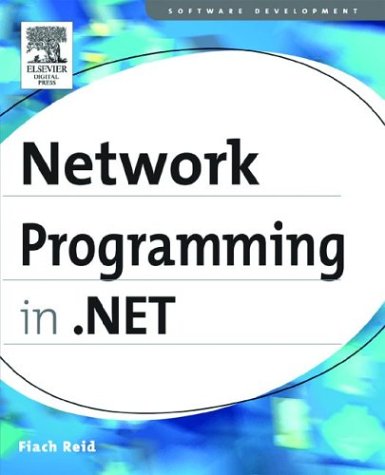
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- unsafenativemethodstextservices.cs
- Msmq.cs
- LineMetrics.cs
- Trace.cs
- AttributeExtensions.cs
- Page.cs
- BuildProvider.cs
- TagMapCollection.cs
- ExpressionBuilderContext.cs
- TogglePattern.cs
- EncoderParameters.cs
- FileInfo.cs
- WmpBitmapEncoder.cs
- PeerService.cs
- DotExpr.cs
- InvalidPropValue.cs
- AsyncCompletedEventArgs.cs
- RadialGradientBrush.cs
- CalendarTable.cs
- CodeStatement.cs
- StylusButton.cs
- VideoDrawing.cs
- ControlCommandSet.cs
- SelectionEditingBehavior.cs
- HijriCalendar.cs
- SqlCachedBuffer.cs
- Substitution.cs
- SpotLight.cs
- HttpInputStream.cs
- HttpModulesSection.cs
- ProxyGenerationError.cs
- SerializerDescriptor.cs
- ImmComposition.cs
- EncoderReplacementFallback.cs
- ButtonPopupAdapter.cs
- KeyboardNavigation.cs
- UserInitiatedRoutedEventPermission.cs
- XmlEnumAttribute.cs
- ToolStripItemTextRenderEventArgs.cs
- Popup.cs
- DragDropManager.cs
- XmlSerializer.cs
- UnionCodeGroup.cs
- XmlHierarchicalDataSourceView.cs
- DurableEnlistmentState.cs
- StoragePropertyMapping.cs
- XamlTypeMapperSchemaContext.cs
- SqlConnectionManager.cs
- ScriptModule.cs
- ArrangedElementCollection.cs
- SimpleColumnProvider.cs
- BaseValidator.cs
- IProvider.cs
- WaitHandle.cs
- DataGridViewUtilities.cs
- _NestedMultipleAsyncResult.cs
- BaseCollection.cs
- QuestionEventArgs.cs
- ObjectKeyFrameCollection.cs
- CopyAction.cs
- TextSchema.cs
- WebEventCodes.cs
- CompilerState.cs
- SizeAnimationUsingKeyFrames.cs
- _ShellExpression.cs
- QueryPageSettingsEventArgs.cs
- IndicShape.cs
- HScrollBar.cs
- GroupedContextMenuStrip.cs
- ScrollPattern.cs
- AttachedAnnotation.cs
- CellPartitioner.cs
- MessageEventSubscriptionService.cs
- Pair.cs
- HuffCodec.cs
- XomlCompilerParameters.cs
- processwaithandle.cs
- ConfigurationSettings.cs
- IERequestCache.cs
- TextEffect.cs
- InOutArgument.cs
- ConfigurationValues.cs
- PersonalizationStateInfoCollection.cs
- GifBitmapEncoder.cs
- DataGridColumnEventArgs.cs
- DbExpressionBuilder.cs
- HitTestFilterBehavior.cs
- ChangesetResponse.cs
- ObjectMemberMapping.cs
- MetadataHelper.cs
- Positioning.cs
- ServiceDesigner.xaml.cs
- XmlSchemaRedefine.cs
- CodeDirectionExpression.cs
- AttributeEmitter.cs
- ConfigXmlDocument.cs
- ScriptIgnoreAttribute.cs
- EntityDataSourceViewSchema.cs
- RuleInfoComparer.cs
- ProgressBar.cs