Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / ManipulationDeltaEventArgs.cs / 1305600 / ManipulationDeltaEventArgs.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Security; using System.Windows; using System.Windows.Media; namespace System.Windows.Input { ////// Provides an update on an ocurring manipulation. /// public sealed class ManipulationDeltaEventArgs : InputEventArgs { ////// Instantiates a new instance of this class. /// internal ManipulationDeltaEventArgs( ManipulationDevice manipulationDevice, int timestamp, IInputElement manipulationContainer, Point origin, ManipulationDelta delta, ManipulationDelta cumulative, ManipulationVelocities velocities, bool isInertial) : base(manipulationDevice, timestamp) { if (delta == null) { throw new ArgumentNullException("delta"); } if (cumulative == null) { throw new ArgumentNullException("cumulative"); } if (velocities == null) { throw new ArgumentNullException("velocities"); } RoutedEvent = Manipulation.ManipulationDeltaEvent; ManipulationContainer = manipulationContainer; ManipulationOrigin = origin; DeltaManipulation = delta; CumulativeManipulation = cumulative; Velocities = velocities; IsInertial = isInertial; } ////// Invokes a handler of this event. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { if (genericHandler == null) { throw new ArgumentNullException("genericHandler"); } if (genericTarget == null) { throw new ArgumentNullException("genericTarget"); } if (RoutedEvent == Manipulation.ManipulationDeltaEvent) { ((EventHandler)genericHandler)(genericTarget, this); } else { base.InvokeEventHandler(genericHandler, genericTarget); } } /// /// Whether the event was generated due to inertia. /// public bool IsInertial { get; private set; } ////// Defines the coordinate space of the other properties. /// public IInputElement ManipulationContainer { get; private set; } ////// Returns the value of the origin. /// public Point ManipulationOrigin { get; private set; } ////// Returns the cumulative transformation associated with the manipulation. /// public ManipulationDelta CumulativeManipulation { get; private set; } ////// Returns the delta transformation associated with the manipulation. /// public ManipulationDelta DeltaManipulation { get; private set; } ////// Returns the current velocities associated with a manipulation. /// public ManipulationVelocities Velocities { get; private set; } ////// Allows a handler to specify that the manipulation has gone beyond certain boundaries. /// By default, this value will then be used to provide panning feedback on the window, but /// it can be change by handling the ManipulationBoundaryFeedback event. /// public void ReportBoundaryFeedback(ManipulationDelta unusedManipulation) { if (unusedManipulation == null) { throw new ArgumentNullException("unusedManipulation"); } UnusedManipulation = unusedManipulation; } ////// The value of the unused manipulation information in global coordinate space. /// internal ManipulationDelta UnusedManipulation { get; private set; } ////// Preempts further processing and completes the manipulation without any inertia. /// public void Complete() { RequestedComplete = true; RequestedInertia = false; RequestedCancel = false; } ////// Preempts further processing and completes the manipulation, allowing inertia to continue. /// public void StartInertia() { RequestedComplete = true; RequestedInertia = true; RequestedCancel = false; } ////// Method to cancel the Manipulation /// ///A bool indicating the success of Cancel public bool Cancel() { if (!IsInertial) { RequestedCancel = true; RequestedComplete = false; RequestedInertia = false; return true; } return false; } ////// A handler requested that the manipulation complete. /// internal bool RequestedComplete { get; private set; } ////// A handler requested that the manipulation complete with inertia. /// internal bool RequestedInertia { get; private set; } ////// A handler Requested to cancel the Manipulation /// internal bool RequestedCancel { get; private set; } ////// The Manipulators for this manipulation. /// public IEnumerableManipulators { get { if (_manipulators == null) { _manipulators = ((ManipulationDevice)Device).GetManipulatorsReadOnly(); } return _manipulators; } } private IEnumerable _manipulators; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Security; using System.Windows; using System.Windows.Media; namespace System.Windows.Input { /// /// Provides an update on an ocurring manipulation. /// public sealed class ManipulationDeltaEventArgs : InputEventArgs { ////// Instantiates a new instance of this class. /// internal ManipulationDeltaEventArgs( ManipulationDevice manipulationDevice, int timestamp, IInputElement manipulationContainer, Point origin, ManipulationDelta delta, ManipulationDelta cumulative, ManipulationVelocities velocities, bool isInertial) : base(manipulationDevice, timestamp) { if (delta == null) { throw new ArgumentNullException("delta"); } if (cumulative == null) { throw new ArgumentNullException("cumulative"); } if (velocities == null) { throw new ArgumentNullException("velocities"); } RoutedEvent = Manipulation.ManipulationDeltaEvent; ManipulationContainer = manipulationContainer; ManipulationOrigin = origin; DeltaManipulation = delta; CumulativeManipulation = cumulative; Velocities = velocities; IsInertial = isInertial; } ////// Invokes a handler of this event. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { if (genericHandler == null) { throw new ArgumentNullException("genericHandler"); } if (genericTarget == null) { throw new ArgumentNullException("genericTarget"); } if (RoutedEvent == Manipulation.ManipulationDeltaEvent) { ((EventHandler)genericHandler)(genericTarget, this); } else { base.InvokeEventHandler(genericHandler, genericTarget); } } /// /// Whether the event was generated due to inertia. /// public bool IsInertial { get; private set; } ////// Defines the coordinate space of the other properties. /// public IInputElement ManipulationContainer { get; private set; } ////// Returns the value of the origin. /// public Point ManipulationOrigin { get; private set; } ////// Returns the cumulative transformation associated with the manipulation. /// public ManipulationDelta CumulativeManipulation { get; private set; } ////// Returns the delta transformation associated with the manipulation. /// public ManipulationDelta DeltaManipulation { get; private set; } ////// Returns the current velocities associated with a manipulation. /// public ManipulationVelocities Velocities { get; private set; } ////// Allows a handler to specify that the manipulation has gone beyond certain boundaries. /// By default, this value will then be used to provide panning feedback on the window, but /// it can be change by handling the ManipulationBoundaryFeedback event. /// public void ReportBoundaryFeedback(ManipulationDelta unusedManipulation) { if (unusedManipulation == null) { throw new ArgumentNullException("unusedManipulation"); } UnusedManipulation = unusedManipulation; } ////// The value of the unused manipulation information in global coordinate space. /// internal ManipulationDelta UnusedManipulation { get; private set; } ////// Preempts further processing and completes the manipulation without any inertia. /// public void Complete() { RequestedComplete = true; RequestedInertia = false; RequestedCancel = false; } ////// Preempts further processing and completes the manipulation, allowing inertia to continue. /// public void StartInertia() { RequestedComplete = true; RequestedInertia = true; RequestedCancel = false; } ////// Method to cancel the Manipulation /// ///A bool indicating the success of Cancel public bool Cancel() { if (!IsInertial) { RequestedCancel = true; RequestedComplete = false; RequestedInertia = false; return true; } return false; } ////// A handler requested that the manipulation complete. /// internal bool RequestedComplete { get; private set; } ////// A handler requested that the manipulation complete with inertia. /// internal bool RequestedInertia { get; private set; } ////// A handler Requested to cancel the Manipulation /// internal bool RequestedCancel { get; private set; } ////// The Manipulators for this manipulation. /// public IEnumerableManipulators { get { if (_manipulators == null) { _manipulators = ((ManipulationDevice)Device).GetManipulatorsReadOnly(); } return _manipulators; } } private IEnumerable _manipulators; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
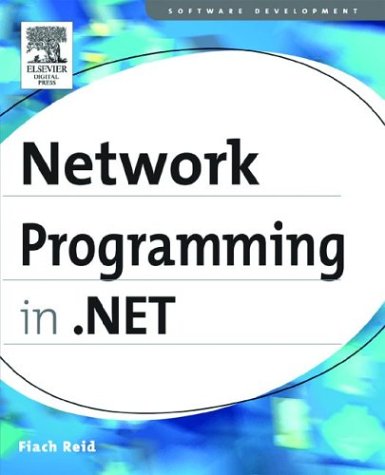
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Int16Animation.cs
- WpfSharedXamlSchemaContext.cs
- ToolBarPanel.cs
- FilterableData.cs
- NativeWindow.cs
- QueryRewriter.cs
- TaiwanLunisolarCalendar.cs
- DbConnectionInternal.cs
- RpcCryptoContext.cs
- SslStream.cs
- Utilities.cs
- AppearanceEditorPart.cs
- X509Utils.cs
- PerfCounterSection.cs
- ContentDisposition.cs
- DesignTimeHTMLTextWriter.cs
- PropertyPathConverter.cs
- ByteConverter.cs
- DBConnectionString.cs
- X509Utils.cs
- MainMenu.cs
- DbParameterHelper.cs
- SQLConvert.cs
- RegisteredArrayDeclaration.cs
- NamespaceInfo.cs
- RichTextBoxAutomationPeer.cs
- DataColumnChangeEvent.cs
- EncryptedType.cs
- Vector3D.cs
- UIAgentMonitorHandle.cs
- CommandEventArgs.cs
- UserControl.cs
- columnmapkeybuilder.cs
- PropertyMetadata.cs
- InternalUserCancelledException.cs
- MobileResource.cs
- XmlExpressionDumper.cs
- HttpCookieCollection.cs
- MSAAWinEventWrap.cs
- COM2IDispatchConverter.cs
- SizeChangedInfo.cs
- XamlBuildTaskServices.cs
- CodeSnippetStatement.cs
- TabletCollection.cs
- DefaultEventAttribute.cs
- BrowserCapabilitiesCodeGenerator.cs
- FlatButtonAppearance.cs
- EventMappingSettingsCollection.cs
- ReliableRequestSessionChannel.cs
- ServiceMetadataExtension.cs
- ObjectDataSourceSelectingEventArgs.cs
- SettingsProviderCollection.cs
- Form.cs
- EventWaitHandleSecurity.cs
- ElementMarkupObject.cs
- AuthenticationService.cs
- DeviceContext.cs
- TypedReference.cs
- TailPinnedEventArgs.cs
- CompensableActivity.cs
- DictionaryEntry.cs
- SqlDataSourceEnumerator.cs
- CacheChildrenQuery.cs
- DoubleLinkList.cs
- SqlCachedBuffer.cs
- ArgumentsParser.cs
- CombinedGeometry.cs
- IgnoreFlushAndCloseStream.cs
- TextEmbeddedObject.cs
- DEREncoding.cs
- OrthographicCamera.cs
- DataException.cs
- XmlDataSource.cs
- ProjectedWrapper.cs
- AutomationPeer.cs
- IdnMapping.cs
- ToolStripControlHost.cs
- SafeFileMappingHandle.cs
- WebControlsSection.cs
- OverrideMode.cs
- FrameworkContextData.cs
- SaveFileDialogDesigner.cs
- InteropExecutor.cs
- SelectionItemPattern.cs
- Scripts.cs
- MsmqAppDomainProtocolHandler.cs
- KeyboardDevice.cs
- Type.cs
- GridItem.cs
- Graphics.cs
- ButtonFieldBase.cs
- Classification.cs
- ParameterModifier.cs
- DataGridViewTextBoxCell.cs
- HtmlInputHidden.cs
- KeyedHashAlgorithm.cs
- ComponentEditorPage.cs
- HybridDictionary.cs
- PropertyTab.cs
- MethodBuilderInstantiation.cs