Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CompMod / System / ComponentModel / DesignerAttribute.cs / 1305376 / DesignerAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel { using System; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Globalization; using System.Security.Permissions; ////// [AttributeUsage(AttributeTargets.Class | AttributeTargets.Interface, AllowMultiple = true, Inherited = true)] public sealed class DesignerAttribute : Attribute { private readonly string designerTypeName; private readonly string designerBaseTypeName; private string typeId; ///Specifies the class to use to implement design-time services. ////// public DesignerAttribute(string designerTypeName) { string temp = designerTypeName.ToUpper(CultureInfo.InvariantCulture); Debug.Assert(temp.IndexOf(".DLL") == -1, "Came across: " + designerTypeName + " . Please remove the .dll extension"); this.designerTypeName = designerTypeName; this.designerBaseTypeName = typeof(IDesigner).FullName; } ////// Initializes a new instance of the ///class using the name of the type that /// provides design-time services. /// /// public DesignerAttribute(Type designerType) { this.designerTypeName = designerType.AssemblyQualifiedName; this.designerBaseTypeName = typeof(IDesigner).FullName; } ////// Initializes a new instance of the ///class using the type that provides /// design-time services. /// /// public DesignerAttribute(string designerTypeName, string designerBaseTypeName) { string temp = designerTypeName.ToUpper(CultureInfo.InvariantCulture); Debug.Assert(temp.IndexOf(".DLL") == -1, "Came across: " + designerTypeName + " . Please remove the .dll extension"); this.designerTypeName = designerTypeName; this.designerBaseTypeName = designerBaseTypeName; } ////// Initializes a new instance of the ///class using the designer type and the /// base class for the designer. /// /// public DesignerAttribute(string designerTypeName, Type designerBaseType) { string temp = designerTypeName.ToUpper(CultureInfo.InvariantCulture); Debug.Assert(temp.IndexOf(".DLL") == -1, "Came across: " + designerTypeName + " . Please remove the .dll extension"); this.designerTypeName = designerTypeName; this.designerBaseTypeName = designerBaseType.AssemblyQualifiedName; } ////// Initializes a new instance of the ///class, using the name of the designer /// class and the base class for the designer. /// /// public DesignerAttribute(Type designerType, Type designerBaseType) { this.designerTypeName = designerType.AssemblyQualifiedName; this.designerBaseTypeName = designerBaseType.AssemblyQualifiedName; } ////// Initializes a new instance of the ///class using the types of the designer and /// designer base class. /// /// public string DesignerBaseTypeName { get { return designerBaseTypeName; } } ////// Gets /// the name of the base type of this designer. /// ////// public string DesignerTypeName { get { return designerTypeName; } } ////// Gets the name of the designer type associated with this designer attribute. /// ////// /// public override object TypeId { get { if (typeId == null) { string baseType = designerBaseTypeName; int comma = baseType.IndexOf(','); if (comma != -1) { baseType = baseType.Substring(0, comma); } typeId = GetType().FullName + baseType; } return typeId; } } public override bool Equals(object obj) { if (obj == this) { return true; } DesignerAttribute other = obj as DesignerAttribute; return (other != null) && other.designerBaseTypeName == designerBaseTypeName && other.designerTypeName == designerTypeName; } public override int GetHashCode() { return designerTypeName.GetHashCode() ^ designerBaseTypeName.GetHashCode(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// This defines a unique ID for this attribute type. It is used /// by filtering algorithms to identify two attributes that are /// the same type. For most attributes, this just returns the /// Type instance for the attribute. DesignerAttribute overrides /// this to include the type of the designer base type. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel { using System; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Globalization; using System.Security.Permissions; ////// [AttributeUsage(AttributeTargets.Class | AttributeTargets.Interface, AllowMultiple = true, Inherited = true)] public sealed class DesignerAttribute : Attribute { private readonly string designerTypeName; private readonly string designerBaseTypeName; private string typeId; ///Specifies the class to use to implement design-time services. ////// public DesignerAttribute(string designerTypeName) { string temp = designerTypeName.ToUpper(CultureInfo.InvariantCulture); Debug.Assert(temp.IndexOf(".DLL") == -1, "Came across: " + designerTypeName + " . Please remove the .dll extension"); this.designerTypeName = designerTypeName; this.designerBaseTypeName = typeof(IDesigner).FullName; } ////// Initializes a new instance of the ///class using the name of the type that /// provides design-time services. /// /// public DesignerAttribute(Type designerType) { this.designerTypeName = designerType.AssemblyQualifiedName; this.designerBaseTypeName = typeof(IDesigner).FullName; } ////// Initializes a new instance of the ///class using the type that provides /// design-time services. /// /// public DesignerAttribute(string designerTypeName, string designerBaseTypeName) { string temp = designerTypeName.ToUpper(CultureInfo.InvariantCulture); Debug.Assert(temp.IndexOf(".DLL") == -1, "Came across: " + designerTypeName + " . Please remove the .dll extension"); this.designerTypeName = designerTypeName; this.designerBaseTypeName = designerBaseTypeName; } ////// Initializes a new instance of the ///class using the designer type and the /// base class for the designer. /// /// public DesignerAttribute(string designerTypeName, Type designerBaseType) { string temp = designerTypeName.ToUpper(CultureInfo.InvariantCulture); Debug.Assert(temp.IndexOf(".DLL") == -1, "Came across: " + designerTypeName + " . Please remove the .dll extension"); this.designerTypeName = designerTypeName; this.designerBaseTypeName = designerBaseType.AssemblyQualifiedName; } ////// Initializes a new instance of the ///class, using the name of the designer /// class and the base class for the designer. /// /// public DesignerAttribute(Type designerType, Type designerBaseType) { this.designerTypeName = designerType.AssemblyQualifiedName; this.designerBaseTypeName = designerBaseType.AssemblyQualifiedName; } ////// Initializes a new instance of the ///class using the types of the designer and /// designer base class. /// /// public string DesignerBaseTypeName { get { return designerBaseTypeName; } } ////// Gets /// the name of the base type of this designer. /// ////// public string DesignerTypeName { get { return designerTypeName; } } ////// Gets the name of the designer type associated with this designer attribute. /// ////// /// public override object TypeId { get { if (typeId == null) { string baseType = designerBaseTypeName; int comma = baseType.IndexOf(','); if (comma != -1) { baseType = baseType.Substring(0, comma); } typeId = GetType().FullName + baseType; } return typeId; } } public override bool Equals(object obj) { if (obj == this) { return true; } DesignerAttribute other = obj as DesignerAttribute; return (other != null) && other.designerBaseTypeName == designerBaseTypeName && other.designerTypeName == designerTypeName; } public override int GetHashCode() { return designerTypeName.GetHashCode() ^ designerBaseTypeName.GetHashCode(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// This defines a unique ID for this attribute type. It is used /// by filtering algorithms to identify two attributes that are /// the same type. For most attributes, this just returns the /// Type instance for the attribute. DesignerAttribute overrides /// this to include the type of the designer base type. /// ///
Link Menu
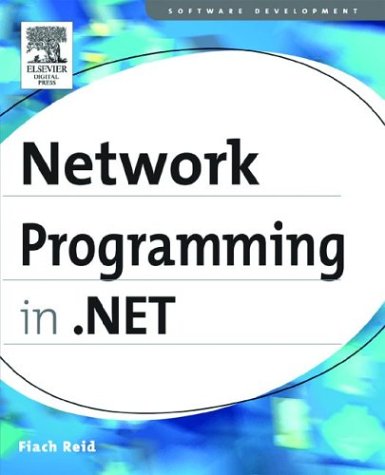
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InputProviderSite.cs
- PageContentAsyncResult.cs
- XmlIlVisitor.cs
- ConstraintEnumerator.cs
- XPathQilFactory.cs
- ParseElement.cs
- ListBindingHelper.cs
- EntitySqlQueryBuilder.cs
- OracleTransaction.cs
- Compensate.cs
- XmlException.cs
- RouteItem.cs
- FlowNode.cs
- TraceInternal.cs
- GeneralTransform3D.cs
- StringWriter.cs
- SiteMap.cs
- ApplicationId.cs
- _AutoWebProxyScriptEngine.cs
- Types.cs
- MatrixCamera.cs
- TreeNodeStyle.cs
- RSAPKCS1SignatureDeformatter.cs
- SystemUnicastIPAddressInformation.cs
- InputScopeAttribute.cs
- Soap12ServerProtocol.cs
- SqlNodeTypeOperators.cs
- DataControlFieldTypeEditor.cs
- RIPEMD160Managed.cs
- BevelBitmapEffect.cs
- CompatibleIComparer.cs
- BindingObserver.cs
- SqlTriggerAttribute.cs
- Encoder.cs
- InfoCardRSACryptoProvider.cs
- ColumnHeaderConverter.cs
- SecurityRuntime.cs
- DataGridItem.cs
- Range.cs
- ForceCopyBuildProvider.cs
- OdbcHandle.cs
- UnitySerializationHolder.cs
- StrongName.cs
- FloatAverageAggregationOperator.cs
- RequestSecurityToken.cs
- ContainerAction.cs
- JsonWriter.cs
- BatchServiceHost.cs
- Membership.cs
- PackageFilter.cs
- SessionParameter.cs
- MD5HashHelper.cs
- AnimationLayer.cs
- ApplicationActivator.cs
- Point3DKeyFrameCollection.cs
- PasswordPropertyTextAttribute.cs
- MouseGesture.cs
- SchemaTableColumn.cs
- ServiceObjectContainer.cs
- EventListener.cs
- SID.cs
- DynamicValueConverter.cs
- DbConnectionPoolIdentity.cs
- Process.cs
- PeerName.cs
- MemberJoinTreeNode.cs
- DbDataSourceEnumerator.cs
- Compiler.cs
- RegistryConfigurationProvider.cs
- ShaderRenderModeValidation.cs
- VerificationAttribute.cs
- GradientStopCollection.cs
- StrokeCollection.cs
- ControlPaint.cs
- SynchronousReceiveBehavior.cs
- BrowserTree.cs
- DecimalConstantAttribute.cs
- XmlSchemaComplexContent.cs
- OdbcConnectionString.cs
- Assert.cs
- DictionarySurrogate.cs
- LoginName.cs
- Track.cs
- PartialTrustVisibleAssembliesSection.cs
- RbTree.cs
- BitmapImage.cs
- Encoding.cs
- BooleanFacetDescriptionElement.cs
- OutputCacheSettingsSection.cs
- GZipDecoder.cs
- SqlReferenceCollection.cs
- DataGridTablesFactory.cs
- MD5CryptoServiceProvider.cs
- ClientProxyGenerator.cs
- RepeaterItemCollection.cs
- CodePrimitiveExpression.cs
- SqlBuffer.cs
- Rule.cs
- ResourceManager.cs
- SplayTreeNode.cs