Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / RequestSecurityToken.cs / 2 / RequestSecurityToken.cs
namespace Microsoft.InfoCards { using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.IO; using System.ServiceModel.Security; using System.ServiceModel; using System.ServiceModel.Channels; using System.Security.Cryptography; using System.Security.Cryptography.Xml; using System.Runtime.Serialization; using System.Globalization; using System.Xml; using System.IdentityModel.Tokens; using System.ServiceModel.Security.Tokens; using System.IdentityModel.Policy; using System.IdentityModel.Selectors; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; using System.Text; internal class RequestSecurityTokenParameters { private AddressingVersion m_addressingVersion = null; private GetBrowserTokenParameters m_browserTokenParams = null; private InfoCardPolicy m_policy = null; private InfoCard m_card = null; private bool m_discloseOptionalClaims; private RSAKeyValue m_rsaKeyValue = null; private byte[] m_clientEntropyForSymmetric = null; private CultureInfo m_cultureInfo = null; private string m_context = null; private Dictionarym_disclosedClaims; private ProtocolProfile m_profile = null; public AddressingVersion Version { get { return m_addressingVersion; } } public GetBrowserTokenParameters BrowserTokenParams { get { return m_browserTokenParams; } } public InfoCardPolicy Policy { get { return m_policy; } } public InfoCard Card { get { return m_card; } } public bool DiscloseOptionalClaims { get { return m_discloseOptionalClaims; } } public RSAKeyValue RSAKey { get { return m_rsaKeyValue; } } public byte[] ClientEntropyForSymmetric { get { return m_clientEntropyForSymmetric; } } public CultureInfo Culture { get { return m_cultureInfo; } } public string Context { get { return m_context; } } public Dictionary DisclosedClaims { get { return m_disclosedClaims; } } public ProtocolProfile Profile { get { return m_profile; } } public RequestSecurityTokenParameters( AddressingVersion version, InfoCard card, InfoCardPolicy policy, bool discloseOptionalClaims, RSAKeyValue rsaKeyValue, Dictionary disclosedClaims, string context, byte[] clientEntropyForSymmetric, CultureInfo displayCulture ) { m_card = card; m_policy = policy; m_discloseOptionalClaims = discloseOptionalClaims; m_rsaKeyValue = rsaKeyValue; m_clientEntropyForSymmetric = clientEntropyForSymmetric; m_profile = policy.ProtocolVersionProfile; IDT.Assert( ( ( null == m_rsaKeyValue && null != m_clientEntropyForSymmetric ) || // symmetric ( null == m_rsaKeyValue && null == m_clientEntropyForSymmetric ) || // no proof key ( null != m_rsaKeyValue && null == m_clientEntropyForSymmetric ) ), // asymmetric "The three allowed cases are symmetric, noProofKey, asymmetric" ); m_disclosedClaims = disclosedClaims; m_addressingVersion = version; m_context = context; m_cultureInfo = displayCulture; } public RequestSecurityTokenParameters( AddressingVersion addressing, GetBrowserTokenParameters parameters, ProtocolProfile profile, InfoCardPolicy policy, bool discloseOptionalClaims ) { m_browserTokenParams = parameters; m_addressingVersion = addressing; m_policy = policy; m_discloseOptionalClaims = discloseOptionalClaims; m_context = Guid.NewGuid().ToString(); m_profile = profile; } } internal abstract class RequestSecurityToken : BodyWriter { protected RequestSecurityTokenParameters m_rstParams; protected RequestSecurityTokenSerializer m_serializer; protected XmlDictionaryWriter m_xmlWriter; // // Retrieves the protocol versions to use when serializing // protected ProtocolProfile ProtocolVersionProfile { get { return m_rstParams.Profile; } } protected InfoCardPolicy Policy { get { return m_rstParams.Policy; } } protected RequestSecurityTokenSerializer Serializer { get { return m_serializer; } set { m_serializer = value; } } protected XmlDictionaryWriter Writer { get { return m_xmlWriter; } set { m_xmlWriter = value; } } protected string WstPrefix { get { return ProtocolVersionProfile.WSTrust.DefaultPrefix; } } public RequestSecurityToken( RequestSecurityTokenParameters rstParams ) : base( false ) { m_rstParams = rstParams; } protected virtual void WriteRSTOpeningElement() { Writer.WriteStartElement( WstPrefix, ProtocolVersionProfile.WSTrust.RequestSecurityToken, ProtocolVersionProfile.WSTrust.Namespace ); Writer.WriteAttributeString( ProtocolVersionProfile.WSTrust.Context, null, m_rstParams.Context ); } protected virtual void WriteInfoCardReferenceElement() { if( m_rstParams.Card != null ) { Serializer.WriteInfoCardReferenceElement( m_rstParams.Card ); } } protected virtual void WriteKeyTypeElement() { IDT.TraceDebug( "IPSTSCLIENT: Writing key type {0} to RST", Policy.GetKeyTypeString() ); Serializer.WriteKeyTypeElement( Policy.GetKeyTypeString() ); } protected virtual void WriteKeySupportingElements() { // // write the (KeySize, Entropy) or (KeySize, UseKey) or nothing // in symmetric, asymmetric, and no proof key cases respectively. // if( SecurityKeyTypeInternal.SymmetricKey == Policy.KeyType ) { // // write the keySize - default is 256 (currently) if not specified // IDT.TraceDebug( "IPSTSCLIENT: Writing key size {0} to RST", Policy.GetIntelligentKeySize( false ) ); Serializer.WriteKeySizeElement( Policy.GetIntelligentKeySize( false ).ToString( CultureInfo.InvariantCulture ) ); IDT.Assert( null != m_rstParams.ClientEntropyForSymmetric, "Should not be null" ); // // Send entropy to IP/STS // BinarySecretSecurityToken bst = new BinarySecretSecurityToken( m_rstParams.ClientEntropyForSymmetric ); Serializer.WriteBinarySecretElement( bst ); // // Write encryptWith // if( !String.IsNullOrEmpty( Policy.OptionalRstParams.EncryptWith ) ) { Serializer.WriteEncryptWithElement( Policy.OptionalRstParams.EncryptWith ); } // // Write signWith // if( !String.IsNullOrEmpty( Policy.OptionalRstParams.SignWith ) ) { Serializer.WriteSignWithElement( Policy.OptionalRstParams.SignWith ); } } else if( SecurityKeyTypeInternal.AsymmetricKey == Policy.KeyType ) { // // The KeyWrapAlgorithm element is specific to WSTrust Oasis 2007 (1.3). It allows the RP to specify (to the STS) // the asymmetric algorithm to use for protecting the key that was used to encrypt the token. // if( XmlNames.WSSpecificationVersion.WSTrustOasis2007 == ProtocolVersionProfile.WSTrust.Version ) { // // Write KeyWrapAlgorithm // if( !String.IsNullOrEmpty( Policy.OptionalRstParams.KeyWrapAlgorithm ) ) { Serializer.WriteKeyWrapAlgorithmElement( Policy.OptionalRstParams.KeyWrapAlgorithm ); } } // // write the keySize - this should be 2048 // IDT.TraceDebug( "IPSTSCLIENT: Writing key size {0} to RST", Policy.GetIntelligentKeySize( false ) ); Serializer.WriteKeySizeElement( Policy.GetIntelligentKeySize( false ).ToString( CultureInfo.InvariantCulture ) ); // // write the UseKey element if Asymmetric keys are to be used // IDT.Assert( null != m_rstParams.RSAKey, "Should have been populated in asymmetric case" ); IDT.TraceDebug( "IPSTSCLIENT: Writing use key {0} to RST", m_rstParams.RSAKey.GetXml().InnerXml ); Serializer.WriteUseKeyElement( m_rstParams.RSAKey.GetXml() ); // // Write the encryptWith // if( !String.IsNullOrEmpty( Policy.OptionalRstParams.EncryptWith ) ) { Serializer.WriteEncryptWithElement( Policy.OptionalRstParams.EncryptWith ); } // // Write signWith // if( !String.IsNullOrEmpty( Policy.OptionalRstParams.SignWith ) ) { Serializer.WriteSignWithElement( Policy.OptionalRstParams.SignWith ); } } else { IDT.Assert( SecurityKeyTypeInternal.NoKey == Policy.KeyType, "Should be no proof key" ); } } protected virtual void WriteRequestTypeElement() { // // Write RequestType element. If none found in policy write the WSTrust version specific Issue action uri. // For browser case, we only support the Issue action. // if( !String.IsNullOrEmpty( Policy.RequestType ) ) { IDT.TraceDebug( "IPSTSCLIENT: Writing RequestType {0} to RST", Policy.RequestType ); Serializer.WriteRequestTypeElement( Policy.RequestType ); } else { IDT.TraceDebug( "IPSTSCLIENT: Writing RequestType {0} to RST", ProtocolVersionProfile.WSTrust.IssueRequestType ); Serializer.WriteRequestTypeElement( ProtocolVersionProfile.WSTrust.IssueRequestType ); } } protected virtual void WritePPIDElement() { // // Write the /ClientPseudonym/PPID element if PPID claim is present in card // PPID = base64 string version of hash( card key, recipient org id, card salt ) // if( ( null != m_rstParams.Card ) && ( m_rstParams.Card.GetClaims().ContainsKey( InfoCardConstants.PPIDClaimsUri ) ) ) { byte[] ppid = Utility.CreateHash( m_rstParams.Card.Key, Convert.FromBase64String( Policy.ImmediateTokenRecipient.GetOrganizationPPIDIdentifier() ), m_rstParams.Card.HashSalt ); Serializer.WritePPIDElement( ppid ); } } protected virtual void WriteEncryptionAlgorithmElement() { // // Write the EncryptionAlgorithm element // if( !string.IsNullOrEmpty( Policy.OptionalRstParams.EncryptionAlgorithm ) ) { IDT.TraceDebug( "IPSTSCLIENT: Writing encrypting algorithm {0} to RST", Policy.OptionalRstParams.EncryptionAlgorithm ); Serializer.WriteEncryptionAlgorithmElement( Policy.OptionalRstParams.EncryptionAlgorithm ); } } protected virtual void WriteClaimsElement() { Serializer.WriteClaimsElement( m_rstParams.DisclosedClaims, Policy.RequiredClaims, Policy.OptionalClaims ); } protected virtual void WriteDisplayTokenElement() { IDT.TraceDebug( "IPSTSCLIENT: Writing display token elemnet to RST" ); if( null != m_rstParams.Culture ) { Serializer.WriteDisplayTokenElement( m_rstParams.Culture ); } } protected virtual void WriteTokenTypeElement() { if( !String.IsNullOrEmpty( Policy.OptionalRstParams.TokenType ) ) { Serializer.WriteTokenTypeElement( Policy.OptionalRstParams.TokenType ); } } protected virtual void WritePassOnElements() { // // Write the pass on parameters from Recipient policy's RequestSecurityTokenTemplate. // Optional RST Parameters include: // // wst:TokenType // wst:SignatureAlgorithm // wst:EncryptionAlgorithm // wst:CanonicalizationAlgorithm // wst:SignWith // wst:EncryptWith // wst:KeyWrapAlgorithm // Policy.OptionalRstParams.WritePassOnElements( Writer, ProtocolVersionProfile ); } protected virtual void WriteUnprocessedPolicyElements() { // // Write all the other policy elements // if( ( null != Policy.UnprocessedPolicyElements ) && ( Policy.UnprocessedPolicyElements.Length > 0 ) ) { Serializer.WriteUnprocessedPolicyElements( Policy.UnprocessedPolicyElements ); } } protected abstract void WriteSecondaryParametersElement(); protected virtual void WriteEndElement() { // // Write closing element // Writer.WriteEndElement(); } protected abstract void WriteAppliesToElement(); protected virtual void InitializeWriters( XmlDictionaryWriter writer ) { Writer = writer; // // Initialize the serializer does the actual work of writing the RST elements. // This property will be used by all the write functions called below. // Serializer = new RequestSecurityTokenSerializer( writer, ProtocolVersionProfile ); } // // We are called back here when the rst is being serialized into the message body, and write the custom // element in as an rst customToken tag. // protected override void OnWriteBodyContents( XmlDictionaryWriter writer ) { // // Call the pure virtual version of the function so we can ensure the method has been implemented // by any derived instances. // CustomWriteBodyContents( writer ); } protected abstract void CustomWriteBodyContents( XmlDictionaryWriter writer ); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
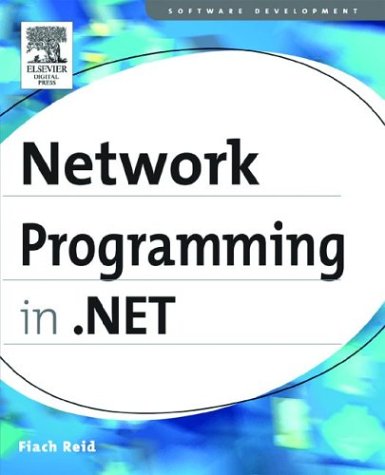
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UnicastIPAddressInformationCollection.cs
- MultiPageTextView.cs
- DataMisalignedException.cs
- TailCallAnalyzer.cs
- WindowsHyperlink.cs
- XmlElementAttributes.cs
- GridSplitterAutomationPeer.cs
- CryptoStream.cs
- EUCJPEncoding.cs
- CodeTypeConstructor.cs
- Events.cs
- SecurityElement.cs
- AddInAttribute.cs
- cache.cs
- MenuItemCollectionEditor.cs
- ZipIOLocalFileHeader.cs
- GraphicsContext.cs
- TrackBarRenderer.cs
- UnsafeMethods.cs
- BasicExpandProvider.cs
- TrackingProvider.cs
- ExpandableObjectConverter.cs
- SiteMapNodeItem.cs
- XmlTextReaderImpl.cs
- Perspective.cs
- SqlTopReducer.cs
- ButtonField.cs
- GeneralTransform2DTo3DTo2D.cs
- HtmlControlPersistable.cs
- OdbcCommand.cs
- Convert.cs
- EntityDataSourceEntityTypeFilterItem.cs
- ArrayList.cs
- ScalarOps.cs
- PersianCalendar.cs
- TrustLevelCollection.cs
- XmlAttributeCollection.cs
- WebZone.cs
- DotExpr.cs
- TextBreakpoint.cs
- InternalBase.cs
- TextStore.cs
- XPathException.cs
- WmlValidatorAdapter.cs
- ExpressionNormalizer.cs
- PrintPreviewControl.cs
- WebEventTraceProvider.cs
- WindowsSspiNegotiation.cs
- TimeStampChecker.cs
- InfoCardMasterKey.cs
- XmlDataSourceNodeDescriptor.cs
- TextCharacters.cs
- MenuItem.cs
- AsymmetricSignatureDeformatter.cs
- SystemTcpConnection.cs
- BindingEditor.xaml.cs
- XmlWriter.cs
- TableLayoutStyleCollection.cs
- TypeInformation.cs
- SimpleHandlerFactory.cs
- GlyphsSerializer.cs
- StreamResourceInfo.cs
- InvalidDocumentContentsException.cs
- MultipartContentParser.cs
- DynamicControl.cs
- EntityDataSourceQueryBuilder.cs
- TriggerCollection.cs
- DataGridViewRowsRemovedEventArgs.cs
- SqlDataRecord.cs
- ConsumerConnectionPointCollection.cs
- ClaimTypes.cs
- FormsAuthenticationCredentials.cs
- ShaderEffect.cs
- XmlCharCheckingWriter.cs
- HostingEnvironmentWrapper.cs
- SmuggledIUnknown.cs
- ImageCodecInfoPrivate.cs
- FillErrorEventArgs.cs
- PingOptions.cs
- SafeNativeMemoryHandle.cs
- Brushes.cs
- AuthenticationModulesSection.cs
- BindingManagerDataErrorEventArgs.cs
- ResXBuildProvider.cs
- ReleaseInstanceMode.cs
- SemanticResultValue.cs
- UnsafeNativeMethodsMilCoreApi.cs
- NativeMethods.cs
- NativeCompoundFileAPIs.cs
- AutomationPropertyInfo.cs
- XmlSchemaValidator.cs
- SafeNativeMethods.cs
- ActivityExecutorSurrogate.cs
- SyntaxCheck.cs
- DataGridViewSelectedColumnCollection.cs
- ReachFixedPageSerializer.cs
- XomlCompiler.cs
- FormViewRow.cs
- HttpWriter.cs
- PageCatalogPart.cs