Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridViewSelectedColumnCollection.cs / 1305376 / DataGridViewSelectedColumnCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System; using System.Collections; using System.Windows.Forms; using System.ComponentModel; using System.Globalization; using System.Diagnostics.CodeAnalysis; ///[ ListBindable(false), SuppressMessage("Microsoft.Design", "CA1010:CollectionsShouldImplementGenericInterface") // Consider adding an IList implementation ] public class DataGridViewSelectedColumnCollection : BaseCollection, IList { ArrayList items = new ArrayList(); /// /// int IList.Add(object value) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// void IList.Clear() { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// bool IList.Contains(object value) { return this.items.Contains(value); } /// /// int IList.IndexOf(object value) { return this.items.IndexOf(value); } /// /// void IList.Insert(int index, object value) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// void IList.Remove(object value) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// void IList.RemoveAt(int index) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// bool IList.IsFixedSize { get { return true; } } /// /// bool IList.IsReadOnly { get { return true; } } /// /// object IList.this[int index] { get { return this.items[index]; } set { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } } /// /// void ICollection.CopyTo(Array array, int index) { this.items.CopyTo(array, index); } /// /// int ICollection.Count { get { return this.items.Count; } } /// /// bool ICollection.IsSynchronized { get { return false; } } /// /// object ICollection.SyncRoot { get { return this; } } /// /// IEnumerator IEnumerable.GetEnumerator() { return this.items.GetEnumerator(); } internal DataGridViewSelectedColumnCollection() { } /// protected override ArrayList List { get { return this.items; } } /// public DataGridViewColumn this[int index] { get { return (DataGridViewColumn) this.items[index]; } } /// /// /// internal int Add(DataGridViewColumn dataGridViewColumn) { return this.items.Add(dataGridViewColumn); } /* Unused at this point internal void AddRange(DataGridViewColumn[] dataGridViewColumns) { Debug.Assert(dataGridViewColumns != null); foreach(DataGridViewColumn dataGridViewColumn in dataGridViewColumns) { this.items.Add(dataGridViewColumn); } } */ /* Unused at this point internal void AddColumnCollection(DataGridViewColumnCollection dataGridViewColumns) { Debug.Assert(dataGridViewColumns != null); foreach(DataGridViewColumn dataGridViewColumn in dataGridViewColumns) { this.items.Add(dataGridViewColumn); } } */ ///Adds a ///to this collection. [ EditorBrowsable(EditorBrowsableState.Never) ] public void Clear() { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// /// Checks to see if a DataGridViewCell is contained in this collection. /// public bool Contains(DataGridViewColumn dataGridViewColumn) { return this.items.IndexOf(dataGridViewColumn) != -1; } ///public void CopyTo(DataGridViewColumn[] array, int index) { this.items.CopyTo(array, index); } /// [ EditorBrowsable(EditorBrowsableState.Never), SuppressMessage("Microsoft.Performance", "CA1801:AvoidUnusedParameters") ] public void Insert(int index, DataGridViewColumn dataGridViewColumn) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
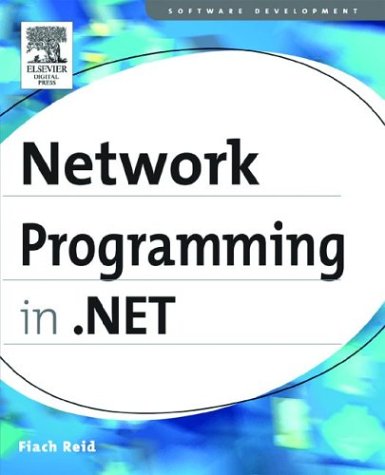
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpEncoder.cs
- GridItemProviderWrapper.cs
- SubMenuStyleCollection.cs
- PointLightBase.cs
- CaseInsensitiveHashCodeProvider.cs
- FrameSecurityDescriptor.cs
- OpenTypeCommon.cs
- OleDbCommandBuilder.cs
- RichTextBoxAutomationPeer.cs
- PrintPreviewGraphics.cs
- EdmToObjectNamespaceMap.cs
- COAUTHIDENTITY.cs
- EventLogPermissionAttribute.cs
- OptimalBreakSession.cs
- PrintDialogException.cs
- XmlCDATASection.cs
- RelationshipSet.cs
- ClientCultureInfo.cs
- BCryptSafeHandles.cs
- XmlIlTypeHelper.cs
- SqlTopReducer.cs
- Timer.cs
- ContentHostHelper.cs
- Vector3DAnimationBase.cs
- EntityDataSourceDesignerHelper.cs
- EntityDataSourceContextCreatedEventArgs.cs
- CreateSequence.cs
- SourceFileInfo.cs
- StrokeCollection2.cs
- QueuePathEditor.cs
- DataRelationPropertyDescriptor.cs
- MediaEntryAttribute.cs
- GeometryConverter.cs
- CheckBox.cs
- CardSpaceShim.cs
- XPathDocument.cs
- DataGridViewComboBoxColumn.cs
- SymLanguageType.cs
- ElementHostPropertyMap.cs
- DataGridViewCellValidatingEventArgs.cs
- SqlBulkCopyColumnMappingCollection.cs
- Style.cs
- HtmlTableCellCollection.cs
- MexHttpBindingCollectionElement.cs
- CssClassPropertyAttribute.cs
- _DomainName.cs
- ArcSegment.cs
- _CacheStreams.cs
- IndexedString.cs
- Zone.cs
- dataobject.cs
- SecurityElement.cs
- DataGridViewColumnConverter.cs
- CssTextWriter.cs
- Trigger.cs
- ListViewGroup.cs
- XmlNavigatorFilter.cs
- Parameter.cs
- WpfGeneratedKnownProperties.cs
- FilterException.cs
- ResourceIDHelper.cs
- ClaimComparer.cs
- FilteredAttributeCollection.cs
- IItemContainerGenerator.cs
- CapabilitiesRule.cs
- IdnElement.cs
- SqlPersistenceProviderFactory.cs
- ADRoleFactory.cs
- DbInsertCommandTree.cs
- Rethrow.cs
- TrustExchangeException.cs
- TableRowGroup.cs
- ServiceContractGenerationContext.cs
- LocalValueEnumerator.cs
- ErrorBehavior.cs
- SqlProcedureAttribute.cs
- HandleExceptionArgs.cs
- DisableDpiAwarenessAttribute.cs
- BufferAllocator.cs
- Floater.cs
- XMLSyntaxException.cs
- UnicastIPAddressInformationCollection.cs
- SafeIUnknown.cs
- HtmlLink.cs
- SortDescription.cs
- EventBookmark.cs
- xmlfixedPageInfo.cs
- WindowsListView.cs
- CheckableControlBaseAdapter.cs
- FunctionQuery.cs
- WebPartManagerInternals.cs
- NonNullItemCollection.cs
- TextEditorDragDrop.cs
- AnnotationResourceCollection.cs
- DocumentProperties.cs
- DataViewManager.cs
- AssemblyAttributesGoHere.cs
- DataTableNameHandler.cs
- Cast.cs
- HtmlForm.cs