Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / TrustUi / MS / Internal / documents / Application / DocumentProperties.cs / 1 / DocumentProperties.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: This is a wrapper for DocumentPropertiesDialog, which caches the values which // are displayed in the Dialog, and controls security access. // // History: // 08/31/2005 - [....] created. // //--------------------------------------------------------------------------- using MS.Internal.PresentationUI; // For CriticalDataForSet using System; using System.Drawing; using System.Globalization; using System.IO; using System.IO.Packaging; // For Package using System.Security; // For CriticalData using System.Security.Permissions; // For asserts using System.Windows.TrustUI; // For string resources using System.Windows.Xps.Packaging; // For XpsDocument namespace MS.Internal.Documents.Application { ////// Singleton wrapper class for the DocumentPropertiesDialog. /// [FriendAccessAllowed] internal sealed class DocumentProperties { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructs the DocumentProperties class. /// /// ////// Critical /// 1) Sets the _uri which is critical. /// [SecurityCritical] private DocumentProperties(Uri uri) { if (uri == null) { throw new ArgumentNullException("uri"); } _uri = new SecurityCriticalData(uri); } #endregion Constructors //------------------------------------------------------ // // Internal Properties // //----------------------------------------------------- #region Internal Properties /// /// Current package CoreProperties /// internal PackageProperties CoreProperties { get { // multiple returns are bad, however allocating another local // simply to return is worse // also we can not cache this decision because the values // may change when RM is on / off if (_rmProperties != null) { return _rmProperties; } else { return _xpsProperties; } } } ////// Gets the current singleton instance of DocumentProperties. /// internal static DocumentProperties Current { get { return _current; } } ////// Image representing an XPS Document /// internal Image Image { get { return _image; } set { _image = value; } } ////// Filename of the package. /// ////// Critical /// 1) Determined from the URI which is considered critical. /// internal string Filename { [SecurityCritical] get { if (_filename == null && _uri.Value != null) { _filename = Path.GetFileName(_uri.Value.LocalPath); } return _filename; } } ////// Size of the package. /// ////// Critical /// 1) Requires FileIO to be performed on the package to determine /// this value. /// internal long FileSize { [SecurityCritical] get { long fileSize = 0; if (IsFileInfoValid) { fileSize = _fileInfo.Length; } return fileSize; } } ////// Date package was created. /// ////// Critical /// 1) Requires FileIO to be performed on the package to determine /// this value. /// internal DateTime? FileCreated { [SecurityCritical] get { DateTime? fileCreated = null; if (IsFileInfoValid) { fileCreated = _fileInfo.CreationTime; } return fileCreated; } } ////// Date package was last modified. /// ////// Critical /// 1) Requires FileIO to be performed on the package to determine /// this value. /// internal DateTime? FileModified { [SecurityCritical] get { DateTime? fileModified = null; if (IsFileInfoValid) { fileModified = _fileInfo.LastWriteTime; } return fileModified; } } ////// Date package was last accessed. /// ////// Critical /// 1) Requires FileIO to be performed on the package to determine /// this value. /// internal DateTime? FileAccessed { [SecurityCritical] get { DateTime? fileAccessed = null; if (IsFileInfoValid) { fileAccessed = _fileInfo.LastAccessTime; } return fileAccessed; } } #endregion Internal Properties //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// This method copies members from one PackageProperties object to another. /// ////// This design is very fragile as if new properties are added by PackageProperties /// we will quietly lose data. An improved implementation would be to have /// PackageProperties implement this behavior or have a KeyValue pair exposed /// that we would iterate through and copy. /// ////// Critical /// 1) source or target may be UmcSuppressedProperties with all /// critical members /// [SecurityCritical] internal static void Copy(PackageProperties source, PackageProperties target) { target.Category = source.Category; target.ContentStatus = source.ContentStatus; target.ContentType = source.ContentType; target.Created = source.Created; target.Creator = source.Creator; target.Description = source.Description; target.Identifier = source.Identifier; target.Keywords = source.Keywords; target.Language = source.Language; target.LastModifiedBy = source.LastModifiedBy; target.LastPrinted = source.LastPrinted; target.Modified = source.Modified; target.Revision = source.Revision; target.Subject = source.Subject; target.Title = source.Title; target.Version = source.Version; } ////// Constructs and Initializes the static 'Current' DocumentProperties object which /// is the singleton instance. /// /// The URI for the package. ////// Critical /// 1) Constructs a new DocumentProperties object using the URI passed in. /// [SecurityCritical] internal static void InitializeCurrentDocumentProperties(Uri uri) { // Ensure that DocumentProperties has not been constructed yet, and initialize a // new instance. System.Diagnostics.Debug.Assert( Current == null, "DocumentProperties initialized twice."); if (Current == null) { _current = new DocumentProperties(uri); } } internal void SetXpsProperties(PackageProperties properties) { _xpsProperties = properties; } internal void SetRightsManagedProperties(PackageProperties properties) { _rmProperties = properties; } ////// Confirms whether the XPS properties exactly match the RM properties /// for purposes of determining whether the OPC properties have been changed /// in violation of signing policy. /// ////// This will return true if the properties match /// or there are no RM properties in the package, /// otherwise it will return false. /// [SecurityCritical, SecurityTreatAsSafe] internal bool VerifyPropertiesUnchanged() { if (_xpsProperties == null ) { return false; } if (_rmProperties == null) { return true; } return (String.Equals(_xpsProperties.Category, _rmProperties.Category, StringComparison.Ordinal) && String.Equals(_xpsProperties.ContentStatus, _rmProperties.ContentStatus, StringComparison.Ordinal) && String.Equals(_xpsProperties.ContentType, _rmProperties.ContentType, StringComparison.Ordinal) && String.Equals(_xpsProperties.Creator, _rmProperties.Creator, StringComparison.Ordinal) && String.Equals(_xpsProperties.Description, _rmProperties.Description, StringComparison.Ordinal) && String.Equals(_xpsProperties.Identifier, _rmProperties.Identifier, StringComparison.Ordinal) && String.Equals(_xpsProperties.Keywords, _rmProperties.Keywords, StringComparison.Ordinal) && String.Equals(_xpsProperties.Language, _rmProperties.Language, StringComparison.Ordinal) && String.Equals(_xpsProperties.LastModifiedBy, _rmProperties.LastModifiedBy, StringComparison.Ordinal) && String.Equals(_xpsProperties.Revision, _rmProperties.Revision, StringComparison.Ordinal) && String.Equals(_xpsProperties.Subject, _rmProperties.Subject, StringComparison.Ordinal) && String.Equals(_xpsProperties.Title, _rmProperties.Title, StringComparison.Ordinal) && String.Equals(_xpsProperties.Version, _rmProperties.Version, StringComparison.Ordinal) && _xpsProperties.Created == _rmProperties.Created && _xpsProperties.LastPrinted == _rmProperties.LastPrinted && _xpsProperties.Modified == _rmProperties.Modified); } ////// Critical /// 1) This method is critical for change tracking purposes (per PT review) /// since it is used to determine validity of signatures, which is a /// critical operation. /// TreatAsSafe /// 1) We are marking this critical only for change tracking purposes. /// ////// ShowDialog: Displays the DocumentProperties dialog. /// ////// Critical /// 1) Checks critical member _fileInfo for null and calls the Refresh /// method on it. /// 2) Elevation to show a top level window. /// TreatAsSafe /// 1) None of the data from the _fileInfo is read or leaked. /// 2) The window is a blessed internal window that uses purely Windows Forms rather than Avalon. /// [SecurityCritical, SecurityTreatAsSafe] internal void ShowDialog() { // Setup the dialog data once and cache it for future calls. if (!_isDataAcquired) { AcquireData(); _isDataAcquired = true; } // Refresh file information before showing the dialog. if (_fileInfo != null) { // We intentionally `swallow exceptions here, since any failure // will make _fileInfo invalid as determined by the property // IsFileInfoValid. We also don't set _fileInfo to null so that // we can call Refresh again. This is useful since the file may // be inaccessible now but come back later. try { _fileInfo.Refresh(); } catch (ArgumentException) { } catch (IOException) { } } DocumentPropertiesDialog dialog = null; // Assert for UIPermission to spawn dialog (new UIPermission(UIPermissionWindow.AllWindows)).Assert(); //BlessedAssert try { dialog = new DocumentPropertiesDialog(); dialog.ShowDialog(); } finally { UIPermission.RevertAssert(); if (dialog != null) { dialog.Dispose(); } } } #endregion Internal Methods //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods ////// AcquireData: Setup the URI related data for the dialog. /// ////// Critical /// 1) Elevation to acquire local file information (FileInfo). /// 2) Accesses _uri. /// 3) Sets critical _fileInfo member. /// TreatAsSafe /// 1) The assert is only done for local files, using the URI which is /// marked critical. /// 2) Does not leak _uri. /// 3) Data for critical members is derived from critical data using /// trusted classes. /// [SecurityCritical, SecurityTreatAsSafe] private void AcquireData() { // Ensure URI exists. if (_uri.Value == null) { return; } // Determine if the URI represents a file if (_uri.Value.IsFile) { // Determine the full path and assert for file permission string filePath = _uri.Value.LocalPath; FileInfo fileInfo = null; (new FileIOPermission( FileIOPermissionAccess.Read, filePath)).Assert(); //BlessedAssert try { // Get the FileInfo for the current file fileInfo = new FileInfo(filePath); } finally { FileIOPermission.RevertAssert(); } // Check that FileInfo is valid, and save it. if (fileInfo.Exists) { _fileInfo = fileInfo; } } } #endregion Private Methods //----------------------------------------------------- // // Private Properties // //----------------------------------------------------- #region Private Properties ////// True if the stored FileInfo is valid and can be used to determine /// file properties. /// ////// If the file has become completely inaccessible (i.e. the drive has /// been disconnected, the UNC path is unreachable, etc.), according to /// MSDN the Refresh call should throw an ArgumentException or /// IOException. In reality it doesn't appear to ever throw. Instead, /// if Refresh fails, any future calls to _fileInfo.Exists return /// false. Accessing any of the file information properties at that /// point throws an exception. As a result, if _fileInfo.Refresh() /// fails, we can leave _fileInfo as is and simply check /// _fileInfo.Exists before accessing it. /// ////// Critical /// 1) Accesses critical member _fileInfo /// TreatAsSafe /// 1) No critical data is leaked. /// private bool IsFileInfoValid { [SecurityCritical, SecurityTreatAsSafe] get { return ((_fileInfo != null) && (_fileInfo.Exists)); } } #endregion Private Properties //----------------------------------------------------- // // Private Fields // // These must be checked for null prior to use. If available, use the CLR properties // above before these. // //------------------------------------------------------ #region Private Fields private static DocumentProperties _current = null; ////// Critical /// 1) This is critical for set since it is used to determine the filename /// which is opened to acquire the FileInfo object. /// 2) This is critical for get since it can leak file system info. /// private SecurityCriticalData_uri; /// /// The properties in the XpsPackage (OPC). /// private PackageProperties _xpsProperties = null; ////// The properties for RightsManaged encrypted packages (OLE). /// private PackageProperties _rmProperties = null; private bool _isDataAcquired; private Image _image = null; ////// Critical /// 1) Determined from the critical URI. /// [SecurityCritical] private string _filename = null; ////// Critical /// 1) Requires FileIO to be performed on the package to determine /// this value. /// [SecurityCritical] private FileInfo _fileInfo; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
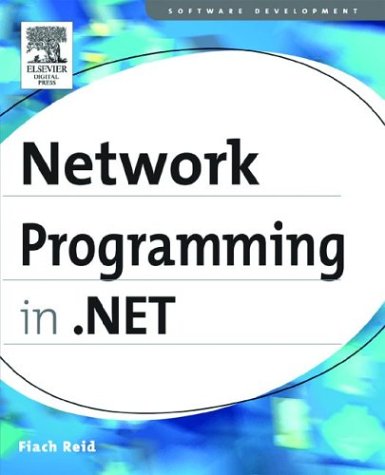
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- JavascriptXmlWriterWrapper.cs
- SingleObjectCollection.cs
- SafeProcessHandle.cs
- WaitHandle.cs
- CompiledQueryCacheKey.cs
- ProcessModelSection.cs
- SuppressMergeCheckAttribute.cs
- CombinedTcpChannel.cs
- AccessDataSourceView.cs
- DataGridAddNewRow.cs
- SqlCaseSimplifier.cs
- CultureInfo.cs
- Deserializer.cs
- GlyphingCache.cs
- SqlCachedBuffer.cs
- StylusPoint.cs
- SplitterPanel.cs
- ValidatedControlConverter.cs
- HierarchicalDataBoundControlAdapter.cs
- UserPreferenceChangingEventArgs.cs
- DefaultAuthorizationContext.cs
- DiagnosticEventProvider.cs
- XmlWrappingReader.cs
- EventLogEntryCollection.cs
- NameScope.cs
- FileSystemInfo.cs
- WindowsTab.cs
- PageClientProxyGenerator.cs
- GridProviderWrapper.cs
- SystemWebExtensionsSectionGroup.cs
- StaticFileHandler.cs
- RequestValidator.cs
- InkCanvasAutomationPeer.cs
- AppDomainInstanceProvider.cs
- FileSystemWatcher.cs
- EmissiveMaterial.cs
- TreeViewImageKeyConverter.cs
- FlowDocumentPage.cs
- TypedElement.cs
- RichTextBoxConstants.cs
- MsmqTransportSecurityElement.cs
- ChtmlTextWriter.cs
- XmlHierarchicalDataSourceView.cs
- GetPageCompletedEventArgs.cs
- DispatchRuntime.cs
- TextTreeTextBlock.cs
- Attributes.cs
- SafeHandles.cs
- NamespaceTable.cs
- FacetDescriptionElement.cs
- Stack.cs
- XPathEmptyIterator.cs
- ConnectionPointCookie.cs
- StaticTextPointer.cs
- QilSortKey.cs
- QilGenerator.cs
- OptimalTextSource.cs
- ForeignKeyConstraint.cs
- NotifyInputEventArgs.cs
- InstanceNameConverter.cs
- CommandLibraryHelper.cs
- LoginView.cs
- HierarchicalDataSourceConverter.cs
- XmlSchemaSimpleTypeList.cs
- CompareInfo.cs
- AdjustableArrowCap.cs
- Scripts.cs
- AliasExpr.cs
- WizardPanel.cs
- Int16Storage.cs
- CompositeFontFamily.cs
- ButtonDesigner.cs
- DataContractAttribute.cs
- ObjectManager.cs
- AlignmentXValidation.cs
- FlagsAttribute.cs
- HMACSHA256.cs
- RegexCompiler.cs
- ProjectedSlot.cs
- _PooledStream.cs
- ProcessHost.cs
- SessionIDManager.cs
- LockCookie.cs
- TemplateInstanceAttribute.cs
- SHA256CryptoServiceProvider.cs
- ClientType.cs
- XmlAtomicValue.cs
- ListBindingHelper.cs
- Rfc2898DeriveBytes.cs
- infer.cs
- ObjectQuery_EntitySqlExtensions.cs
- ValueHandle.cs
- ExpandedWrapper.cs
- InteropAutomationProvider.cs
- AdornedElementPlaceholder.cs
- HttpInputStream.cs
- ConnectorSelectionGlyph.cs
- MouseBinding.cs
- SystemIcmpV4Statistics.cs
- MemberMemberBinding.cs