Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / System / Windows / NameScope.cs / 1305600 / NameScope.cs
/****************************************************************************\ * * File: NameScope.cs * * Used to store mapping information for names occuring * within the logical tree section. * * Copyright (C) 2005 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Windows; using System.Collections; using System.Collections.Specialized; using System.Globalization; using System.Windows.Markup; using System.ComponentModel; using System.Collections.Generic; using MS.Internal; using MS.Internal.WindowsBase; using System.Runtime.CompilerServices; namespace System.Windows { ////// Used to store mapping information for names occuring /// within the logical tree section. /// [TypeForwardedFrom("PresentationFramework, Version=3.5.0.0, Culture=neutral, PublicKeyToken=31bf3856ad364e35")] public class NameScope : INameScopeDictionary { #region INameScope ////// Register Name-Object Map /// /// name to be registered /// object mapped to name public void RegisterName(string name, object scopedElement) { if (name == null) throw new ArgumentNullException("name"); if (scopedElement == null) throw new ArgumentNullException("scopedElement"); if (name == String.Empty) throw new ArgumentException(SR.Get(SRID.NameScopeNameNotEmptyString)); if (!NameValidationHelper.IsValidIdentifierName(name)) { throw new ArgumentException(SR.Get(SRID.NameScopeInvalidIdentifierName, name)); } if (_nameMap == null) { _nameMap = new HybridDictionary(); _nameMap[name] = scopedElement; } else { object nameContext = _nameMap[name]; // first time adding the Name, set it if (nameContext == null) { _nameMap[name] = scopedElement; } else if (scopedElement != nameContext) { throw new ArgumentException(SR.Get(SRID.NameScopeDuplicateNamesNotAllowed, name)); } } if( TraceNameScope.IsEnabled ) { TraceNameScope.TraceActivityItem( TraceNameScope.RegisterName, this, name, scopedElement ); } } ////// Unregister Name-Object Map /// /// name to be registered public void UnregisterName(string name) { if (name == null) throw new ArgumentNullException("name"); if (name == String.Empty) throw new ArgumentException(SR.Get(SRID.NameScopeNameNotEmptyString)); if (_nameMap != null && _nameMap[name] != null) { _nameMap.Remove(name); } else { throw new ArgumentException(SR.Get(SRID.NameScopeNameNotFound, name)); } if( TraceNameScope.IsEnabled ) { TraceNameScope.TraceActivityItem( TraceNameScope.UnregisterName, this, name ); } } ////// Find - Find the corresponding object given a Name /// /// Name for which context needs to be retrieved ///corresponding Context if found, else null public object FindName(string name) { if (_nameMap == null || name == null || name == String.Empty) return null; return _nameMap[name]; } #endregion INameScope #region InternalMethods internal static INameScope NameScopeFromObject(object obj) { INameScope nameScope = obj as INameScope; if (nameScope == null) { DependencyObject objAsDO = obj as DependencyObject; if (objAsDO != null) { nameScope = GetNameScope(objAsDO); } } return nameScope; } #endregion InternalMethods #region DependencyProperties ////// NameScope property. This is an attached property. /// This property allows the dynamic attachment of NameScopes /// public static readonly DependencyProperty NameScopeProperty = DependencyProperty.RegisterAttached( "NameScope", typeof(INameScope), typeof(NameScope)); ////// Helper for setting NameScope property on a DependencyObject. /// /// Dependency Object to set NameScope property on. /// NameScope property value. public static void SetNameScope(DependencyObject dependencyObject, INameScope value) { if (dependencyObject == null) { throw new ArgumentNullException("dependencyObject"); } dependencyObject.SetValue(NameScopeProperty, value); } ////// Helper for reading NameScope property from a DependencyObject. /// /// DependencyObject to read NameScope property from. ///NameScope property value. [DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public static INameScope GetNameScope(DependencyObject dependencyObject) { if (dependencyObject == null) { throw new ArgumentNullException("dependencyObject"); } return ((INameScope)dependencyObject.GetValue(NameScopeProperty)); } #endregion DependencyProperties #region Data // This is a HybridDictionary of Name-Object maps private HybridDictionary _nameMap; #endregion Data IEnumerator> GetEnumerator() { return new Enumerator(this._nameMap); } #region IEnumerable methods IEnumerator IEnumerable.GetEnumerator() { return this.GetEnumerator(); } #endregion #region IEnumerable methods IEnumerator > IEnumerable >.GetEnumerator() { return this.GetEnumerator(); } #endregion #region ICollection methods public int Count { get { if (_nameMap == null) { return 0; } return _nameMap.Count; } } public bool IsReadOnly { get { return false; } } public void Clear() { _nameMap = null; } public void CopyTo(KeyValuePair [] array, int arrayIndex) { if (_nameMap == null) { array = null; return; } foreach (DictionaryEntry entry in _nameMap) { array[arrayIndex++] = new KeyValuePair ((string)entry.Key, entry.Value); } } public bool Remove(KeyValuePair item) { if (!Contains(item)) { return false; } if (item.Value != this[item.Key]) { return false; } return Remove(item.Key); } public void Add(KeyValuePair item) { if (item.Key == null) { throw new ArgumentException(SR.Get(SRID.ReferenceIsNull, "item.Key"), "item"); } if (item.Value == null) { throw new ArgumentException(SR.Get(SRID.ReferenceIsNull, "item.Value"), "item"); } Add(item.Key, item.Value); } public bool Contains(KeyValuePair item) { if (item.Key == null) { throw new ArgumentException(SR.Get(SRID.ReferenceIsNull, "item.Key"), "item"); } return ContainsKey(item.Key); } #endregion #region IDictionary methods public object this[string key] { get { if (key == null) { throw new ArgumentNullException("key"); } return FindName(key); } set { if (key == null) { throw new ArgumentNullException("key"); } if (value == null) { throw new ArgumentNullException("value"); } RegisterName(key, value); } } public void Add(string key, object value) { if (key == null) { throw new ArgumentNullException("key"); } RegisterName(key, value); } public bool ContainsKey(string key) { if (key == null) { throw new ArgumentNullException("key"); } object value = FindName(key); return (value != null); } public bool Remove(string key) { if (!ContainsKey(key)) { return false; } UnregisterName(key); return true; } public bool TryGetValue(string key, out object value) { if (!ContainsKey(key)) { value = null; return false; } value = FindName(key); return true; } public ICollection Keys { get { if (_nameMap == null) { return null; } var list = new List (); foreach (string key in _nameMap.Keys) { list.Add(key); } return list; } } public ICollection
Link Menu
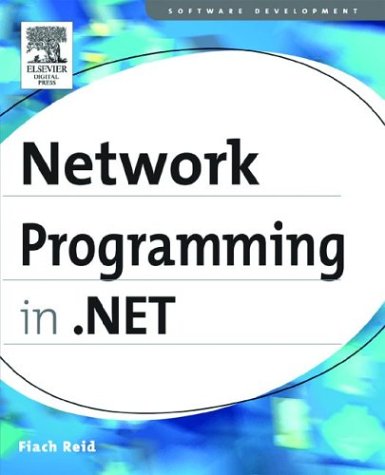
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WindowsPrincipal.cs
- UnitControl.cs
- TableAdapterManagerHelper.cs
- HwndSource.cs
- TextMarkerSource.cs
- CommandEventArgs.cs
- CodeDelegateCreateExpression.cs
- BitmapVisualManager.cs
- XmlSchemaAll.cs
- PrintController.cs
- EntityParameterCollection.cs
- TraceListener.cs
- WebPartZoneBase.cs
- AdornedElementPlaceholder.cs
- CachedBitmap.cs
- QilName.cs
- Int32Storage.cs
- QuaternionAnimation.cs
- LayoutDump.cs
- QilTernary.cs
- PointValueSerializer.cs
- BridgeDataRecord.cs
- MruCache.cs
- ReferentialConstraintRoleElement.cs
- XmlTypeAttribute.cs
- ObjectCloneHelper.cs
- GridViewColumnHeaderAutomationPeer.cs
- FunctionDescription.cs
- GridViewRowPresenterBase.cs
- Rethrow.cs
- PeerNameRegistration.cs
- SubtreeProcessor.cs
- SQLBoolean.cs
- TextParaClient.cs
- ListViewDataItem.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- XmlTextWriter.cs
- GACIdentityPermission.cs
- UdpDiscoveryEndpoint.cs
- SafeLibraryHandle.cs
- RegexReplacement.cs
- UnionQueryOperator.cs
- TraceHwndHost.cs
- PrintPreviewControl.cs
- Label.cs
- OdbcReferenceCollection.cs
- TextBoxAutomationPeer.cs
- Accessible.cs
- UnsafeNativeMethods.cs
- EnumerableWrapperWeakToStrong.cs
- XmlAtomicValue.cs
- NamespaceExpr.cs
- IFlowDocumentViewer.cs
- ZipIOExtraFieldElement.cs
- ConfigurationManagerInternal.cs
- Rule.cs
- AddingNewEventArgs.cs
- Pointer.cs
- ConnectionPointConverter.cs
- DataTableMapping.cs
- WebCategoryAttribute.cs
- Vector3DValueSerializer.cs
- HostingEnvironment.cs
- FileDialogPermission.cs
- XslNumber.cs
- TargetControlTypeAttribute.cs
- Matrix3DStack.cs
- InOutArgument.cs
- PackagePart.cs
- FormattedText.cs
- GenericUriParser.cs
- AttributedMetaModel.cs
- WebReferencesBuildProvider.cs
- FormsAuthentication.cs
- NotSupportedException.cs
- InputScopeNameConverter.cs
- ImportContext.cs
- DurableInstanceProvider.cs
- EventLogEntry.cs
- UserInitiatedNavigationPermission.cs
- ViewgenGatekeeper.cs
- InvokePatternIdentifiers.cs
- TableStyle.cs
- DBPropSet.cs
- basenumberconverter.cs
- TemplateLookupAction.cs
- EmbeddedMailObjectsCollection.cs
- AllMembershipCondition.cs
- _LazyAsyncResult.cs
- EnvelopedPkcs7.cs
- BitmapFrameDecode.cs
- SqlDataSourceCommandEventArgs.cs
- RTLAwareMessageBox.cs
- SoapElementAttribute.cs
- ProcessModule.cs
- BitStream.cs
- Propagator.JoinPropagator.JoinPredicateVisitor.cs
- KeysConverter.cs
- TextEditorCopyPaste.cs
- DbgCompiler.cs