Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / XmlUtils / System / Xml / Xsl / IlGen / XmlIlTypeHelper.cs / 1 / XmlIlTypeHelper.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.IO; using System.Text; using System.Diagnostics; using System.Xml; using System.Xml.XPath; using System.Xml.Schema; using System.Xml.Xsl.Runtime; namespace System.Xml.Xsl.IlGen { ////// Static QilExpression type helper methods. /// internal class XmlILTypeHelper { // Not creatable private XmlILTypeHelper() { } ////// Return the default Clr data type that will be used to store instances of the QilNode's type. /// public static Type GetStorageType(XmlQueryType qyTyp) { Type storageType; if (qyTyp.IsSingleton) { storageType = TypeCodeToStorage[(int) qyTyp.TypeCode]; // Non-strict items must store the type along with the value, so use XPathItem if (!qyTyp.IsStrict && storageType != typeof(XPathNavigator)) return typeof(XPathItem); } else { storageType = TypeCodeToCachedStorage[(int) qyTyp.TypeCode]; // Non-strict items must store the type along with the value, so use XPathItem if (!qyTyp.IsStrict && storageType != typeof(IList)) return typeof(IList ); } return storageType; } private static readonly Type[] TypeCodeToStorage = { typeof(XPathItem), // XmlTypeCode.None typeof(XPathItem), // XmlTypeCode.Item typeof(XPathNavigator), // XmlTypeCode.Node typeof(XPathNavigator), // XmlTypeCode.Document typeof(XPathNavigator), // XmlTypeCode.Element typeof(XPathNavigator), // XmlTypeCode.Attribute typeof(XPathNavigator), // XmlTypeCode.Namespace typeof(XPathNavigator), // XmlTypeCode.ProcessingInstruction typeof(XPathNavigator), // XmlTypeCode.Comment typeof(XPathNavigator), // XmlTypeCode.Text typeof(XPathItem), // XmlTypeCode.AnyAtomicType typeof(string), // XmlTypeCode.UntypedAtomic typeof(string), // XmlTypeCode.String typeof(bool), // XmlTypeCode.Boolean typeof(decimal), // XmlTypeCode.Decimal typeof(float), // XmlTypeCode.Float typeof(double), // XmlTypeCode.Double typeof(string), // XmlTypeCode.Duration typeof(DateTime), // XmlTypeCode.DateTime typeof(DateTime), // XmlTypeCode.Time typeof(DateTime), // XmlTypeCode.Date typeof(DateTime), // XmlTypeCode.GYearMonth typeof(DateTime), // XmlTypeCode.GYear typeof(DateTime), // XmlTypeCode.GMonthDay typeof(DateTime), // XmlTypeCode.GDay typeof(DateTime), // XmlTypeCode.GMonth typeof(byte[]), // XmlTypeCode.HexBinary typeof(byte[]), // XmlTypeCode.Base64Binary typeof(string), // XmlTypeCode.AnyUri typeof(XmlQualifiedName), // XmlTypeCode.QName typeof(XmlQualifiedName), // XmlTypeCode.Notation typeof(string), // XmlTypeCode.NormalizedString typeof(string), // XmlTypeCode.Token typeof(string), // XmlTypeCode.Language typeof(string), // XmlTypeCode.NmToken typeof(string), // XmlTypeCode.Name typeof(string), // XmlTypeCode.NCName typeof(string), // XmlTypeCode.Id typeof(string), // XmlTypeCode.Idref typeof(string), // XmlTypeCode.Entity typeof(long), // XmlTypeCode.Integer typeof(decimal), // XmlTypeCode.NonPositiveInteger typeof(decimal), // XmlTypeCode.NegativeInteger typeof(long), // XmlTypeCode.Long typeof(int), // XmlTypeCode.Int typeof(int), // XmlTypeCode.Short typeof(int), // XmlTypeCode.Byte typeof(decimal), // XmlTypeCode.NonNegativeInteger typeof(decimal), // XmlTypeCode.UnsignedLong typeof(long), // XmlTypeCode.UnsignedInt typeof(int), // XmlTypeCode.UnsignedShort typeof(int), // XmlTypeCode.UnsignedByte typeof(decimal), // XmlTypeCode.PositiveInteger typeof(TimeSpan), // XmlTypeCode.YearMonthDuration typeof(TimeSpan), // XmlTypeCode.DayTimeDuration }; private static readonly Type[] TypeCodeToCachedStorage = { typeof(IList ), // XmlTypeCode.None typeof(IList ), // XmlTypeCode.Item typeof(IList ), // XmlTypeCode.Node typeof(IList ), // XmlTypeCode.Document typeof(IList ), // XmlTypeCode.Element typeof(IList ), // XmlTypeCode.Attribute typeof(IList ), // XmlTypeCode.Namespace typeof(IList ), // XmlTypeCode.ProcessingInstruction typeof(IList ), // XmlTypeCode.Comment typeof(IList ), // XmlTypeCode.Text typeof(IList ), // XmlTypeCode.AnyAtomicType typeof(IList ), // XmlTypeCode.UntypedAtomic typeof(IList ), // XmlTypeCode.String typeof(IList ), // XmlTypeCode.Boolean typeof(IList ), // XmlTypeCode.Decimal typeof(IList ), // XmlTypeCode.Float typeof(IList ), // XmlTypeCode.Double typeof(IList ), // XmlTypeCode.Duration typeof(IList ), // XmlTypeCode.DateTime typeof(IList ), // XmlTypeCode.Time typeof(IList ), // XmlTypeCode.Date typeof(IList ), // XmlTypeCode.GYearMonth typeof(IList ), // XmlTypeCode.GYear typeof(IList ), // XmlTypeCode.GMonthDay typeof(IList ), // XmlTypeCode.GDay typeof(IList ), // XmlTypeCode.GMonth typeof(IList ), // XmlTypeCode.HexBinary typeof(IList ), // XmlTypeCode.Base64Binary typeof(IList ), // XmlTypeCode.AnyUri typeof(IList ), // XmlTypeCode.QName typeof(IList ), // XmlTypeCode.Notation typeof(IList ), // XmlTypeCode.NormalizedString typeof(IList ), // XmlTypeCode.Token typeof(IList ), // XmlTypeCode.Language typeof(IList ), // XmlTypeCode.NmToken typeof(IList ), // XmlTypeCode.Name typeof(IList ), // XmlTypeCode.NCName typeof(IList ), // XmlTypeCode.Id typeof(IList ), // XmlTypeCode.Idref typeof(IList ), // XmlTypeCode.Entity typeof(IList ), // XmlTypeCode.Integer typeof(IList ), // XmlTypeCode.NonPositiveInteger typeof(IList ), // XmlTypeCode.NegativeInteger typeof(IList ), // XmlTypeCode.Long typeof(IList ), // XmlTypeCode.Int typeof(IList ), // XmlTypeCode.Short typeof(IList ), // XmlTypeCode.Byte typeof(IList ), // XmlTypeCode.NonNegativeInteger typeof(IList ), // XmlTypeCode.UnsignedLong typeof(IList ), // XmlTypeCode.UnsignedInt typeof(IList ), // XmlTypeCode.UnsignedShort typeof(IList ), // XmlTypeCode.UnsignedByte typeof(IList ), // XmlTypeCode.PositiveInteger typeof(IList ), // XmlTypeCode.YearMonthDuration typeof(IList ), // XmlTypeCode.DayTimeDuration }; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.IO; using System.Text; using System.Diagnostics; using System.Xml; using System.Xml.XPath; using System.Xml.Schema; using System.Xml.Xsl.Runtime; namespace System.Xml.Xsl.IlGen { ////// Static QilExpression type helper methods. /// internal class XmlILTypeHelper { // Not creatable private XmlILTypeHelper() { } ////// Return the default Clr data type that will be used to store instances of the QilNode's type. /// public static Type GetStorageType(XmlQueryType qyTyp) { Type storageType; if (qyTyp.IsSingleton) { storageType = TypeCodeToStorage[(int) qyTyp.TypeCode]; // Non-strict items must store the type along with the value, so use XPathItem if (!qyTyp.IsStrict && storageType != typeof(XPathNavigator)) return typeof(XPathItem); } else { storageType = TypeCodeToCachedStorage[(int) qyTyp.TypeCode]; // Non-strict items must store the type along with the value, so use XPathItem if (!qyTyp.IsStrict && storageType != typeof(IList)) return typeof(IList ); } return storageType; } private static readonly Type[] TypeCodeToStorage = { typeof(XPathItem), // XmlTypeCode.None typeof(XPathItem), // XmlTypeCode.Item typeof(XPathNavigator), // XmlTypeCode.Node typeof(XPathNavigator), // XmlTypeCode.Document typeof(XPathNavigator), // XmlTypeCode.Element typeof(XPathNavigator), // XmlTypeCode.Attribute typeof(XPathNavigator), // XmlTypeCode.Namespace typeof(XPathNavigator), // XmlTypeCode.ProcessingInstruction typeof(XPathNavigator), // XmlTypeCode.Comment typeof(XPathNavigator), // XmlTypeCode.Text typeof(XPathItem), // XmlTypeCode.AnyAtomicType typeof(string), // XmlTypeCode.UntypedAtomic typeof(string), // XmlTypeCode.String typeof(bool), // XmlTypeCode.Boolean typeof(decimal), // XmlTypeCode.Decimal typeof(float), // XmlTypeCode.Float typeof(double), // XmlTypeCode.Double typeof(string), // XmlTypeCode.Duration typeof(DateTime), // XmlTypeCode.DateTime typeof(DateTime), // XmlTypeCode.Time typeof(DateTime), // XmlTypeCode.Date typeof(DateTime), // XmlTypeCode.GYearMonth typeof(DateTime), // XmlTypeCode.GYear typeof(DateTime), // XmlTypeCode.GMonthDay typeof(DateTime), // XmlTypeCode.GDay typeof(DateTime), // XmlTypeCode.GMonth typeof(byte[]), // XmlTypeCode.HexBinary typeof(byte[]), // XmlTypeCode.Base64Binary typeof(string), // XmlTypeCode.AnyUri typeof(XmlQualifiedName), // XmlTypeCode.QName typeof(XmlQualifiedName), // XmlTypeCode.Notation typeof(string), // XmlTypeCode.NormalizedString typeof(string), // XmlTypeCode.Token typeof(string), // XmlTypeCode.Language typeof(string), // XmlTypeCode.NmToken typeof(string), // XmlTypeCode.Name typeof(string), // XmlTypeCode.NCName typeof(string), // XmlTypeCode.Id typeof(string), // XmlTypeCode.Idref typeof(string), // XmlTypeCode.Entity typeof(long), // XmlTypeCode.Integer typeof(decimal), // XmlTypeCode.NonPositiveInteger typeof(decimal), // XmlTypeCode.NegativeInteger typeof(long), // XmlTypeCode.Long typeof(int), // XmlTypeCode.Int typeof(int), // XmlTypeCode.Short typeof(int), // XmlTypeCode.Byte typeof(decimal), // XmlTypeCode.NonNegativeInteger typeof(decimal), // XmlTypeCode.UnsignedLong typeof(long), // XmlTypeCode.UnsignedInt typeof(int), // XmlTypeCode.UnsignedShort typeof(int), // XmlTypeCode.UnsignedByte typeof(decimal), // XmlTypeCode.PositiveInteger typeof(TimeSpan), // XmlTypeCode.YearMonthDuration typeof(TimeSpan), // XmlTypeCode.DayTimeDuration }; private static readonly Type[] TypeCodeToCachedStorage = { typeof(IList ), // XmlTypeCode.None typeof(IList ), // XmlTypeCode.Item typeof(IList ), // XmlTypeCode.Node typeof(IList ), // XmlTypeCode.Document typeof(IList ), // XmlTypeCode.Element typeof(IList ), // XmlTypeCode.Attribute typeof(IList ), // XmlTypeCode.Namespace typeof(IList ), // XmlTypeCode.ProcessingInstruction typeof(IList ), // XmlTypeCode.Comment typeof(IList ), // XmlTypeCode.Text typeof(IList ), // XmlTypeCode.AnyAtomicType typeof(IList ), // XmlTypeCode.UntypedAtomic typeof(IList ), // XmlTypeCode.String typeof(IList ), // XmlTypeCode.Boolean typeof(IList ), // XmlTypeCode.Decimal typeof(IList ), // XmlTypeCode.Float typeof(IList ), // XmlTypeCode.Double typeof(IList ), // XmlTypeCode.Duration typeof(IList ), // XmlTypeCode.DateTime typeof(IList ), // XmlTypeCode.Time typeof(IList ), // XmlTypeCode.Date typeof(IList ), // XmlTypeCode.GYearMonth typeof(IList ), // XmlTypeCode.GYear typeof(IList ), // XmlTypeCode.GMonthDay typeof(IList ), // XmlTypeCode.GDay typeof(IList ), // XmlTypeCode.GMonth typeof(IList ), // XmlTypeCode.HexBinary typeof(IList ), // XmlTypeCode.Base64Binary typeof(IList ), // XmlTypeCode.AnyUri typeof(IList ), // XmlTypeCode.QName typeof(IList ), // XmlTypeCode.Notation typeof(IList ), // XmlTypeCode.NormalizedString typeof(IList ), // XmlTypeCode.Token typeof(IList ), // XmlTypeCode.Language typeof(IList ), // XmlTypeCode.NmToken typeof(IList ), // XmlTypeCode.Name typeof(IList ), // XmlTypeCode.NCName typeof(IList ), // XmlTypeCode.Id typeof(IList ), // XmlTypeCode.Idref typeof(IList ), // XmlTypeCode.Entity typeof(IList ), // XmlTypeCode.Integer typeof(IList ), // XmlTypeCode.NonPositiveInteger typeof(IList ), // XmlTypeCode.NegativeInteger typeof(IList ), // XmlTypeCode.Long typeof(IList ), // XmlTypeCode.Int typeof(IList ), // XmlTypeCode.Short typeof(IList ), // XmlTypeCode.Byte typeof(IList ), // XmlTypeCode.NonNegativeInteger typeof(IList ), // XmlTypeCode.UnsignedLong typeof(IList ), // XmlTypeCode.UnsignedInt typeof(IList ), // XmlTypeCode.UnsignedShort typeof(IList ), // XmlTypeCode.UnsignedByte typeof(IList ), // XmlTypeCode.PositiveInteger typeof(IList ), // XmlTypeCode.YearMonthDuration typeof(IList ), // XmlTypeCode.DayTimeDuration }; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
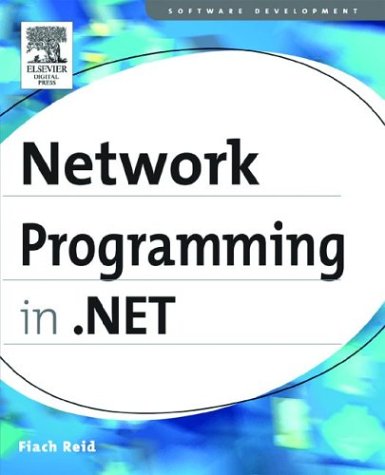
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SamlAuthorizationDecisionStatement.cs
- TextParagraph.cs
- XPathDocumentNavigator.cs
- EnumCodeDomSerializer.cs
- ThrowHelper.cs
- DrawListViewItemEventArgs.cs
- HostUtils.cs
- safemediahandle.cs
- Latin1Encoding.cs
- ColumnWidthChangedEvent.cs
- PeerEndPoint.cs
- SelectionPatternIdentifiers.cs
- WorkflowHostingEndpoint.cs
- WindowsFont.cs
- MemoryRecordBuffer.cs
- PackUriHelper.cs
- GridToolTip.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- StylusSystemGestureEventArgs.cs
- PageAction.cs
- AttachInfo.cs
- VisualTreeUtils.cs
- RightNameExpirationInfoPair.cs
- PageParserFilter.cs
- InternalSafeNativeMethods.cs
- TableLayout.cs
- ConfigurationSettings.cs
- ValidatedControlConverter.cs
- CurrentChangingEventManager.cs
- storepermission.cs
- Column.cs
- AVElementHelper.cs
- OleDbParameterCollection.cs
- DrawingContextDrawingContextWalker.cs
- PhysicalFontFamily.cs
- OdbcEnvironment.cs
- Wizard.cs
- EmptyEnumerator.cs
- Transform.cs
- DashStyle.cs
- FramingChannels.cs
- ContainerActivationHelper.cs
- PlainXmlSerializer.cs
- Content.cs
- DirtyTextRange.cs
- RoleBoolean.cs
- AuthorizationSection.cs
- Int16AnimationUsingKeyFrames.cs
- ScriptDescriptor.cs
- ListManagerBindingsCollection.cs
- UnauthorizedWebPart.cs
- ImplicitInputBrush.cs
- CqlIdentifiers.cs
- ConnectionPointCookie.cs
- BaseUriWithWildcard.cs
- LinkButton.cs
- InputChannelBinder.cs
- SystemParameters.cs
- VScrollProperties.cs
- _NestedMultipleAsyncResult.cs
- ManipulationDeltaEventArgs.cs
- ItemList.cs
- ReferencedCollectionType.cs
- LinkDescriptor.cs
- SelectionItemPattern.cs
- EntityCollection.cs
- EnterpriseServicesHelper.cs
- lengthconverter.cs
- FileInfo.cs
- TemplateBindingExtension.cs
- RowCache.cs
- FilterUserControlBase.cs
- DataGridRow.cs
- Condition.cs
- DropDownButton.cs
- DataGridViewHitTestInfo.cs
- TableDetailsCollection.cs
- StreamWithDictionary.cs
- ImageBrush.cs
- HyperLinkStyle.cs
- NetworkCredential.cs
- RangeValueProviderWrapper.cs
- CharAnimationUsingKeyFrames.cs
- ToolboxBitmapAttribute.cs
- GridViewColumnCollectionChangedEventArgs.cs
- SqlPersonalizationProvider.cs
- X509CertificateStore.cs
- InputMethodStateTypeInfo.cs
- DependencyObject.cs
- RemotingConfiguration.cs
- KeyValuePair.cs
- GeometryValueSerializer.cs
- ArgumentsParser.cs
- TreeNodeBindingCollection.cs
- PasswordBoxAutomationPeer.cs
- Error.cs
- Duration.cs
- ViewDesigner.cs
- NullableDoubleSumAggregationOperator.cs
- ModuleConfigurationInfo.cs