Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / UI / FilteredAttributeCollection.cs / 1 / FilteredAttributeCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections; using System.Collections.Specialized; using System.Globalization; ////// Contains a filtered (by device filter) view of the attributes parsed from a tag /// internal sealed class FilteredAttributeDictionary : IDictionary { private string _filter; private IDictionary _data; private ParsedAttributeCollection _owner; internal FilteredAttributeDictionary(ParsedAttributeCollection owner, string filter) { _filter = filter; _owner = owner; _data = new ListDictionary(StringComparer.OrdinalIgnoreCase); } ////// The actual dictionary used for storing the data /// internal IDictionary Data { get { return _data; } } ////// The filter that this collection is filtering on /// public string Filter { get { return _filter; } } ////// Returns the value of a particular attribute for this filter /// public string this[string key] { get { return (string)_data[key]; } set { _owner.ReplaceFilteredAttribute(_filter, key, value); } } ////// Adds a new attribute for this filter /// public void Add(string key, string value) { _owner.AddFilteredAttribute(_filter, key, value); } ////// Clears all attributes for this filter /// public void Clear() { _owner.ClearFilter(_filter); } ////// Returns true if this filtered view contains the specified attribute /// public bool Contains(string key) { return _data.Contains(key); } ////// Removes the specified attribute for this filter /// public void Remove(string key) { _owner.RemoveFilteredAttribute(_filter, key); } #region IDictionary implementation ///bool IDictionary.IsFixedSize { get { return false; } } /// bool IDictionary.IsReadOnly { get { return false; } } /// object IDictionary.this[object key] { get { if (!(key is string)) { throw new ArgumentException(SR.GetString(SR.FilteredAttributeDictionary_ArgumentMustBeString), "key"); } return this[key.ToString()]; } set { if (!(key is string)) { throw new ArgumentException(SR.GetString(SR.FilteredAttributeDictionary_ArgumentMustBeString), "key"); } if (!(value is string)) { throw new ArgumentException(SR.GetString(SR.FilteredAttributeDictionary_ArgumentMustBeString), "value"); } this[key.ToString()] = value.ToString(); } } /// ICollection IDictionary.Keys { get { return _data.Keys; } } /// ICollection IDictionary.Values { get { return _data.Values; } } /// void IDictionary.Add(object key, object value) { if (key == null) { throw new ArgumentNullException("key"); } if (!(key is string)) { throw new ArgumentException(SR.GetString(SR.FilteredAttributeDictionary_ArgumentMustBeString), "key"); } if (!(value is string)) { throw new ArgumentException(SR.GetString(SR.FilteredAttributeDictionary_ArgumentMustBeString), "value"); } if (value == null) { value = String.Empty; } Add(key.ToString(), value.ToString()); } /// bool IDictionary.Contains(object key) { if (!(key is string)) { throw new ArgumentException(SR.GetString(SR.FilteredAttributeDictionary_ArgumentMustBeString), "key"); } return Contains(key.ToString()); } /// void IDictionary.Clear() { Clear(); } /// IDictionaryEnumerator IDictionary.GetEnumerator() { return _data.GetEnumerator(); } /// void IDictionary.Remove(object key) { Remove(key.ToString()); } #endregion IDictionary implementation #region ICollection implementation /// int ICollection.Count { get { return _data.Count; } } /// bool ICollection.IsSynchronized { get { return ((ICollection)_data).IsSynchronized; } } /// object ICollection.SyncRoot { get { return _data.SyncRoot; } } /// void ICollection.CopyTo(Array array, int index) { _data.CopyTo(array, index); } /// IEnumerator IEnumerable.GetEnumerator() { return _data.GetEnumerator(); } #endregion ICollection implementation } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections; using System.Collections.Specialized; using System.Globalization; ////// Contains a filtered (by device filter) view of the attributes parsed from a tag /// internal sealed class FilteredAttributeDictionary : IDictionary { private string _filter; private IDictionary _data; private ParsedAttributeCollection _owner; internal FilteredAttributeDictionary(ParsedAttributeCollection owner, string filter) { _filter = filter; _owner = owner; _data = new ListDictionary(StringComparer.OrdinalIgnoreCase); } ////// The actual dictionary used for storing the data /// internal IDictionary Data { get { return _data; } } ////// The filter that this collection is filtering on /// public string Filter { get { return _filter; } } ////// Returns the value of a particular attribute for this filter /// public string this[string key] { get { return (string)_data[key]; } set { _owner.ReplaceFilteredAttribute(_filter, key, value); } } ////// Adds a new attribute for this filter /// public void Add(string key, string value) { _owner.AddFilteredAttribute(_filter, key, value); } ////// Clears all attributes for this filter /// public void Clear() { _owner.ClearFilter(_filter); } ////// Returns true if this filtered view contains the specified attribute /// public bool Contains(string key) { return _data.Contains(key); } ////// Removes the specified attribute for this filter /// public void Remove(string key) { _owner.RemoveFilteredAttribute(_filter, key); } #region IDictionary implementation ///bool IDictionary.IsFixedSize { get { return false; } } /// bool IDictionary.IsReadOnly { get { return false; } } /// object IDictionary.this[object key] { get { if (!(key is string)) { throw new ArgumentException(SR.GetString(SR.FilteredAttributeDictionary_ArgumentMustBeString), "key"); } return this[key.ToString()]; } set { if (!(key is string)) { throw new ArgumentException(SR.GetString(SR.FilteredAttributeDictionary_ArgumentMustBeString), "key"); } if (!(value is string)) { throw new ArgumentException(SR.GetString(SR.FilteredAttributeDictionary_ArgumentMustBeString), "value"); } this[key.ToString()] = value.ToString(); } } /// ICollection IDictionary.Keys { get { return _data.Keys; } } /// ICollection IDictionary.Values { get { return _data.Values; } } /// void IDictionary.Add(object key, object value) { if (key == null) { throw new ArgumentNullException("key"); } if (!(key is string)) { throw new ArgumentException(SR.GetString(SR.FilteredAttributeDictionary_ArgumentMustBeString), "key"); } if (!(value is string)) { throw new ArgumentException(SR.GetString(SR.FilteredAttributeDictionary_ArgumentMustBeString), "value"); } if (value == null) { value = String.Empty; } Add(key.ToString(), value.ToString()); } /// bool IDictionary.Contains(object key) { if (!(key is string)) { throw new ArgumentException(SR.GetString(SR.FilteredAttributeDictionary_ArgumentMustBeString), "key"); } return Contains(key.ToString()); } /// void IDictionary.Clear() { Clear(); } /// IDictionaryEnumerator IDictionary.GetEnumerator() { return _data.GetEnumerator(); } /// void IDictionary.Remove(object key) { Remove(key.ToString()); } #endregion IDictionary implementation #region ICollection implementation /// int ICollection.Count { get { return _data.Count; } } /// bool ICollection.IsSynchronized { get { return ((ICollection)_data).IsSynchronized; } } /// object ICollection.SyncRoot { get { return _data.SyncRoot; } } /// void ICollection.CopyTo(Array array, int index) { _data.CopyTo(array, index); } /// IEnumerator IEnumerable.GetEnumerator() { return _data.GetEnumerator(); } #endregion ICollection implementation } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
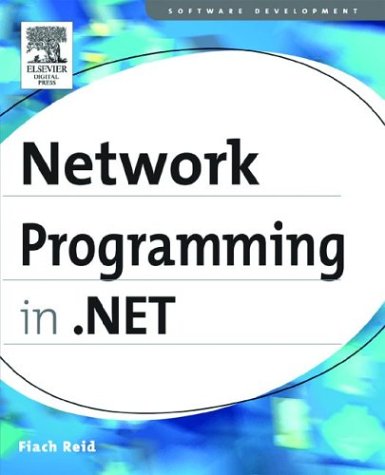
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CreateSequenceResponse.cs
- ISFTagAndGuidCache.cs
- SubpageParaClient.cs
- dataSvcMapFileLoader.cs
- Exceptions.cs
- DefaultPropertiesToSend.cs
- TreeSet.cs
- TextSelectionHelper.cs
- Debug.cs
- GifBitmapEncoder.cs
- ContextStack.cs
- WebEvents.cs
- ThemeDirectoryCompiler.cs
- PolicyException.cs
- ConfigXmlWhitespace.cs
- InternalException.cs
- User.cs
- SimpleExpression.cs
- FileEnumerator.cs
- RecordManager.cs
- MsmqProcessProtocolHandler.cs
- Currency.cs
- ProfileSettingsCollection.cs
- InlineCollection.cs
- TreeViewTemplateSelector.cs
- SecurityCriticalDataForSet.cs
- ListBase.cs
- BoundField.cs
- SectionVisual.cs
- Brush.cs
- PtsPage.cs
- XsdCachingReader.cs
- SequentialWorkflowRootDesigner.cs
- InvalidPropValue.cs
- Automation.cs
- Object.cs
- DispatcherHooks.cs
- StandardCommands.cs
- OneOf.cs
- UnsafeNativeMethods.cs
- TableStyle.cs
- DataGridItemCollection.cs
- ResourceDisplayNameAttribute.cs
- SqlLiftWhereClauses.cs
- TreeNodeConverter.cs
- FrameworkElementFactoryMarkupObject.cs
- CrossSiteScriptingValidation.cs
- SymbolMethod.cs
- VisualStyleTypesAndProperties.cs
- PathSegmentCollection.cs
- UniformGrid.cs
- SmiRequestExecutor.cs
- DataGridViewCellValueEventArgs.cs
- DesignColumn.cs
- RecognizedAudio.cs
- WebPartHelpVerb.cs
- QilIterator.cs
- PersianCalendar.cs
- SqlNodeAnnotation.cs
- TreeNodeStyle.cs
- EnumerableRowCollection.cs
- X509ChainElement.cs
- RijndaelManaged.cs
- XmlDataDocument.cs
- NonVisualControlAttribute.cs
- ControlAdapter.cs
- ISO2022Encoding.cs
- UnitControl.cs
- Baml2006KeyRecord.cs
- Timer.cs
- FileVersion.cs
- BStrWrapper.cs
- BitmapEffectGeneralTransform.cs
- TableRowCollection.cs
- CodeDomComponentSerializationService.cs
- HelpKeywordAttribute.cs
- ErrorFormatterPage.cs
- MetadataCollection.cs
- BaseValidator.cs
- WebPartConnectionsCancelEventArgs.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- EmptyEnumerator.cs
- _emptywebproxy.cs
- TextSegment.cs
- ThrowHelper.cs
- StrongTypingException.cs
- DetailsViewInsertedEventArgs.cs
- SqlStatistics.cs
- CompilerInfo.cs
- DataObjectFieldAttribute.cs
- ProcessHostMapPath.cs
- HttpListenerPrefixCollection.cs
- AccessorTable.cs
- XhtmlBasicValidatorAdapter.cs
- OperationInfo.cs
- WindowsToolbar.cs
- Matrix3D.cs
- ControlPropertyNameConverter.cs
- SocketAddress.cs
- PixelShader.cs