Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Base / System / Windows / Threading / DispatcherHooks.cs / 1 / DispatcherHooks.cs
using System.Security; using System.Security.Permissions; namespace System.Windows.Threading { ////// Additional information provided about a dispatcher. /// public sealed class DispatcherHooks { ////// An event indicating the the dispatcher has no more operations to process. /// ////// Note that this event will be raised by the dispatcher thread when /// there is no more pending work to do. /// /// Note also that this event could come before the last operation is /// invoked, because that is when we determine that the queue is empty. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _dispatcherInactive /// TreatAsSafe: link-demands /// public event EventHandler DispatcherInactive { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _dispatcherInactive += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _dispatcherInactive -= value; } } } ////// An event indicating that an operation was posted to the dispatcher. /// ////// Typically this is due to the BeginInvoke API, but the Invoke API can /// also cause this if any priority other than DispatcherPriority.Send is /// specified, or if the destination dispatcher is owned by a different /// thread. /// /// Note that any thread can post operations, so this event can be /// raised by any thread. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _operationPosted /// TreatAsSafe: link-demands /// public event DispatcherHookEventHandler OperationPosted { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _operationPosted += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _operationPosted -= value; } } } ////// An event indicating that an operation was completed. /// ////// Note that this event will be raised by the dispatcher thread after /// the operation has completed. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _operationCompleted /// TreatAsSafe: link-demands /// public event DispatcherHookEventHandler OperationCompleted { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _operationCompleted += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _operationCompleted -= value; } } } ////// An event indicating that the priority of an operation was changed. /// ////// Note that any thread can change the priority of operations, /// so this event can be raised by any thread. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _operationPriorityChanged /// TreatAsSafe: link-demands /// public event DispatcherHookEventHandler OperationPriorityChanged { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _operationPriorityChanged += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _operationPriorityChanged -= value; } } } ////// An event indicating that an operation was aborted. /// ////// Note that any thread can abort an operation, so this event /// can be raised by any thread. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _operationAborted /// TreatAsSafe: link-demands /// public event DispatcherHookEventHandler OperationAborted { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _operationAborted += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _operationAborted -= value; } } } // Only we can create these things. internal DispatcherHooks() { } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseDispatcherInactive(Dispatcher dispatcher) { EventHandler dispatcherInactive = _dispatcherInactive; if(dispatcherInactive != null) { dispatcherInactive(dispatcher, EventArgs.Empty); } } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseOperationPosted(Dispatcher dispatcher, DispatcherOperation operation) { DispatcherHookEventHandler operationPosted = _operationPosted; if(operationPosted != null) { operationPosted(dispatcher, new DispatcherHookEventArgs(operation)); } } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseOperationCompleted(Dispatcher dispatcher, DispatcherOperation operation) { DispatcherHookEventHandler operationCompleted = _operationCompleted; if(operationCompleted != null) { operationCompleted(dispatcher, new DispatcherHookEventArgs(operation)); } } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseOperationPriorityChanged(Dispatcher dispatcher, DispatcherOperation operation) { DispatcherHookEventHandler operationPriorityChanged = _operationPriorityChanged; if(operationPriorityChanged != null) { operationPriorityChanged(dispatcher, new DispatcherHookEventArgs(operation)); } } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseOperationAborted(Dispatcher dispatcher, DispatcherOperation operation) { DispatcherHookEventHandler operationAborted = _operationAborted; if(operationAborted != null) { operationAborted(dispatcher, new DispatcherHookEventArgs(operation)); } } private object _instanceLock = new object(); ////// Do not expose to partially trusted code. /// [SecurityCritical] private EventHandler _dispatcherInactive; ////// Do not expose to partially trusted code. /// [SecurityCritical] private DispatcherHookEventHandler _operationPosted; ////// Do not expose to partially trusted code. /// [SecurityCritical] private DispatcherHookEventHandler _operationCompleted; ////// Do not expose to partially trusted code. /// [SecurityCritical] private DispatcherHookEventHandler _operationPriorityChanged; ////// Do not expose to partially trusted code. /// [SecurityCritical] private DispatcherHookEventHandler _operationAborted; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System.Security; using System.Security.Permissions; namespace System.Windows.Threading { ////// Additional information provided about a dispatcher. /// public sealed class DispatcherHooks { ////// An event indicating the the dispatcher has no more operations to process. /// ////// Note that this event will be raised by the dispatcher thread when /// there is no more pending work to do. /// /// Note also that this event could come before the last operation is /// invoked, because that is when we determine that the queue is empty. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _dispatcherInactive /// TreatAsSafe: link-demands /// public event EventHandler DispatcherInactive { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _dispatcherInactive += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _dispatcherInactive -= value; } } } ////// An event indicating that an operation was posted to the dispatcher. /// ////// Typically this is due to the BeginInvoke API, but the Invoke API can /// also cause this if any priority other than DispatcherPriority.Send is /// specified, or if the destination dispatcher is owned by a different /// thread. /// /// Note that any thread can post operations, so this event can be /// raised by any thread. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _operationPosted /// TreatAsSafe: link-demands /// public event DispatcherHookEventHandler OperationPosted { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _operationPosted += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _operationPosted -= value; } } } ////// An event indicating that an operation was completed. /// ////// Note that this event will be raised by the dispatcher thread after /// the operation has completed. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _operationCompleted /// TreatAsSafe: link-demands /// public event DispatcherHookEventHandler OperationCompleted { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _operationCompleted += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _operationCompleted -= value; } } } ////// An event indicating that the priority of an operation was changed. /// ////// Note that any thread can change the priority of operations, /// so this event can be raised by any thread. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _operationPriorityChanged /// TreatAsSafe: link-demands /// public event DispatcherHookEventHandler OperationPriorityChanged { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _operationPriorityChanged += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _operationPriorityChanged -= value; } } } ////// An event indicating that an operation was aborted. /// ////// Note that any thread can abort an operation, so this event /// can be raised by any thread. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _operationAborted /// TreatAsSafe: link-demands /// public event DispatcherHookEventHandler OperationAborted { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _operationAborted += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _operationAborted -= value; } } } // Only we can create these things. internal DispatcherHooks() { } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseDispatcherInactive(Dispatcher dispatcher) { EventHandler dispatcherInactive = _dispatcherInactive; if(dispatcherInactive != null) { dispatcherInactive(dispatcher, EventArgs.Empty); } } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseOperationPosted(Dispatcher dispatcher, DispatcherOperation operation) { DispatcherHookEventHandler operationPosted = _operationPosted; if(operationPosted != null) { operationPosted(dispatcher, new DispatcherHookEventArgs(operation)); } } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseOperationCompleted(Dispatcher dispatcher, DispatcherOperation operation) { DispatcherHookEventHandler operationCompleted = _operationCompleted; if(operationCompleted != null) { operationCompleted(dispatcher, new DispatcherHookEventArgs(operation)); } } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseOperationPriorityChanged(Dispatcher dispatcher, DispatcherOperation operation) { DispatcherHookEventHandler operationPriorityChanged = _operationPriorityChanged; if(operationPriorityChanged != null) { operationPriorityChanged(dispatcher, new DispatcherHookEventArgs(operation)); } } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseOperationAborted(Dispatcher dispatcher, DispatcherOperation operation) { DispatcherHookEventHandler operationAborted = _operationAborted; if(operationAborted != null) { operationAborted(dispatcher, new DispatcherHookEventArgs(operation)); } } private object _instanceLock = new object(); ////// Do not expose to partially trusted code. /// [SecurityCritical] private EventHandler _dispatcherInactive; ////// Do not expose to partially trusted code. /// [SecurityCritical] private DispatcherHookEventHandler _operationPosted; ////// Do not expose to partially trusted code. /// [SecurityCritical] private DispatcherHookEventHandler _operationCompleted; ////// Do not expose to partially trusted code. /// [SecurityCritical] private DispatcherHookEventHandler _operationPriorityChanged; ////// Do not expose to partially trusted code. /// [SecurityCritical] private DispatcherHookEventHandler _operationAborted; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
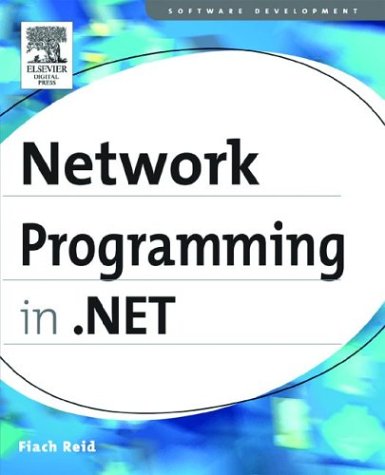
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AssociationSetEnd.cs
- DragEventArgs.cs
- InnerItemCollectionView.cs
- XmlSubtreeReader.cs
- DeviceSpecificChoice.cs
- wmiprovider.cs
- DesignTimeTemplateParser.cs
- JpegBitmapDecoder.cs
- figurelengthconverter.cs
- IndentedWriter.cs
- ColumnMap.cs
- SqlUtils.cs
- DataGridViewElement.cs
- ObjectContext.cs
- HashCryptoHandle.cs
- PolyLineSegmentFigureLogic.cs
- StrokeCollection.cs
- TableDetailsRow.cs
- SendKeys.cs
- BrushMappingModeValidation.cs
- BindingCollection.cs
- DetailsViewDeleteEventArgs.cs
- CodeBinaryOperatorExpression.cs
- HyperlinkAutomationPeer.cs
- SafeNativeMethods.cs
- EDesignUtil.cs
- RequestQueue.cs
- smtpconnection.cs
- HtmlElement.cs
- SqlCommandBuilder.cs
- XmlNavigatorStack.cs
- WhitespaceRule.cs
- Overlapped.cs
- GPRECT.cs
- SchemaLookupTable.cs
- EventDescriptor.cs
- AnnouncementService.cs
- FileDialog_Vista.cs
- DelegateInArgument.cs
- UrlParameterReader.cs
- CallContext.cs
- WorkflowView.cs
- Mouse.cs
- UserNamePasswordValidator.cs
- DesignBindingEditor.cs
- DataGridViewTextBoxColumn.cs
- PopupRootAutomationPeer.cs
- SqlXmlStorage.cs
- TreeChangeInfo.cs
- DataTableNewRowEvent.cs
- Brush.cs
- ToolStripGripRenderEventArgs.cs
- ScriptIgnoreAttribute.cs
- TextStore.cs
- RewritingProcessor.cs
- PersianCalendar.cs
- WhiteSpaceTrimStringConverter.cs
- ConditionCollection.cs
- FastPropertyAccessor.cs
- TypeElement.cs
- NameNode.cs
- RSAPKCS1SignatureDeformatter.cs
- EntityDataSourceState.cs
- FontStretches.cs
- StandardCommands.cs
- FixedSOMSemanticBox.cs
- DrawingAttributesDefaultValueFactory.cs
- CodeExpressionRuleDeclaration.cs
- OleAutBinder.cs
- DataObjectCopyingEventArgs.cs
- FormsAuthentication.cs
- AlphabetConverter.cs
- TextTreeTextNode.cs
- ScrollChangedEventArgs.cs
- LoginUtil.cs
- CompiledIdentityConstraint.cs
- TextEditorLists.cs
- ReaderWriterLock.cs
- ArrayConverter.cs
- MediaContext.cs
- UdpDiscoveryEndpointProvider.cs
- InternalResources.cs
- PropertyFilter.cs
- HMACRIPEMD160.cs
- TreeNodeStyle.cs
- _BasicClient.cs
- TaskSchedulerException.cs
- TypeUsageBuilder.cs
- AsymmetricSignatureFormatter.cs
- ImageMap.cs
- EllipseGeometry.cs
- ConnectionPointCookie.cs
- SourceSwitch.cs
- DocumentOrderComparer.cs
- SqlException.cs
- WindowsAuthenticationModule.cs
- CodeAttributeArgument.cs
- NullNotAllowedCollection.cs
- Misc.cs
- MetabaseSettingsIis7.cs