Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / IdleTimeoutMonitor.cs / 1 / IdleTimeoutMonitor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Request timeout manager -- implements the request timeout mechanism */ namespace System.Web { using System.Threading; using System.Collections; using System.Web.Hosting; using System.Web.Util; internal class IdleTimeoutMonitor { private TimeSpan _idleTimeout; // the timeout value private DateTime _lastEvent; // idle since this time private Timer _timer; private readonly TimeSpan _timerPeriod = new TimeSpan(0, 0, 30); // 30 secs internal IdleTimeoutMonitor(TimeSpan timeout) { _idleTimeout = timeout; _timer = new Timer(new TimerCallback(this.TimerCompletionCallback), null, _timerPeriod, _timerPeriod); _lastEvent = DateTime.UtcNow; } internal void Stop() { // stop the timer if (_timer != null) { lock (this) { if (_timer != null) { ((IDisposable)_timer).Dispose(); _timer = null; } } } } internal DateTime LastEvent { // thread-safe property get { DateTime t; lock (this) { t = _lastEvent; } return t; } set { lock (this) { _lastEvent = value; } } } private void TimerCompletionCallback(Object state) { // user idle timer to trim the free list of app instanced HttpApplicationFactory.TrimApplicationInstances(); // no idle timeout if (_idleTimeout == TimeSpan.MaxValue) return; // don't do idle timeout if already shutting down if (HostingEnvironment.ShutdownInitiated) return; // check if there are active requests if (HostingEnvironment.BusyCount != 0) return; // check if enough time passed if (DateTime.UtcNow <= LastEvent.Add(_idleTimeout)) return; // check if debugger is attached if (System.Diagnostics.Debugger.IsAttached) return; // shutdown HttpRuntime.SetShutdownReason(ApplicationShutdownReason.IdleTimeout, SR.GetString(SR.Hosting_Env_IdleTimeout)); HostingEnvironment.InitiateShutdown(); } } }
Link Menu
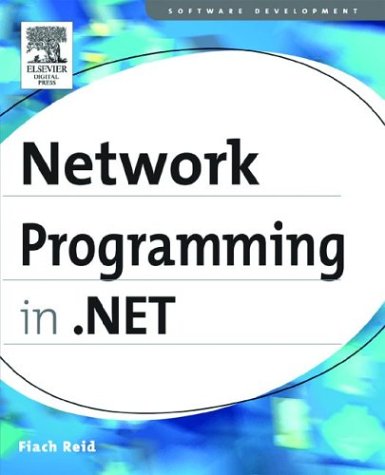
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataServiceQuery.cs
- SchemaAttDef.cs
- OutputCache.cs
- Command.cs
- DataExchangeServiceBinder.cs
- ValidatorAttribute.cs
- Panel.cs
- WebPartDeleteVerb.cs
- SqlInternalConnectionTds.cs
- WCFServiceClientProxyGenerator.cs
- CommandTreeTypeHelper.cs
- CompilationUtil.cs
- OdbcStatementHandle.cs
- AuthStoreRoleProvider.cs
- IntSecurity.cs
- MobileResource.cs
- CriticalExceptions.cs
- GPStream.cs
- KnownTypeDataContractResolver.cs
- CompositeFontInfo.cs
- WebPartTracker.cs
- SmtpClient.cs
- PublisherIdentityPermission.cs
- ParentQuery.cs
- SafeSecurityHandles.cs
- Crc32Helper.cs
- GridViewEditEventArgs.cs
- FlowDocumentReader.cs
- Emitter.cs
- DoubleCollection.cs
- SetterBaseCollection.cs
- WebPartZone.cs
- StringDictionary.cs
- XmlDataImplementation.cs
- DateTimeValueSerializer.cs
- WsdlContractConversionContext.cs
- ResourceContainer.cs
- PropertyGroupDescription.cs
- AssociationProvider.cs
- ParameterToken.cs
- ChannelAcceptor.cs
- CancellationHandlerDesigner.cs
- X509ClientCertificateCredentialsElement.cs
- LinearKeyFrames.cs
- Context.cs
- BitmapMetadataBlob.cs
- MgmtConfigurationRecord.cs
- BitmapData.cs
- SqlUtil.cs
- WebSysDisplayNameAttribute.cs
- DataViewManagerListItemTypeDescriptor.cs
- XmlWrappingWriter.cs
- LinqDataSourceUpdateEventArgs.cs
- ActivityExecutionContextCollection.cs
- GlyphRun.cs
- FixedSOMSemanticBox.cs
- SolidColorBrush.cs
- TextEmbeddedObject.cs
- FontStyle.cs
- SafeFileMapViewHandle.cs
- Transform3DCollection.cs
- ResXFileRef.cs
- TextTabProperties.cs
- XmlHierarchicalDataSourceView.cs
- DBParameter.cs
- TextBox.cs
- XmlComplianceUtil.cs
- ListViewItem.cs
- DataGridViewColumnHeaderCell.cs
- OutputWindow.cs
- RepeatButtonAutomationPeer.cs
- EditorResources.cs
- XmlReader.cs
- EntityDataSourceContextCreatedEventArgs.cs
- SchemaTypeEmitter.cs
- SplashScreenNativeMethods.cs
- TokenBasedSet.cs
- MetadataSource.cs
- DecimalSumAggregationOperator.cs
- MenuItem.cs
- WhileDesigner.xaml.cs
- KeyFrames.cs
- CancellationHandlerDesigner.cs
- SymLanguageType.cs
- StartUpEventArgs.cs
- OdbcInfoMessageEvent.cs
- ModelUIElement3D.cs
- ReceiveContextCollection.cs
- PrePostDescendentsWalker.cs
- GridErrorDlg.cs
- PingOptions.cs
- NameSpaceExtractor.cs
- SchemaTableOptionalColumn.cs
- RemotingConfiguration.cs
- ConfigurationValues.cs
- AssertUtility.cs
- MailDefinition.cs
- HTTPNotFoundHandler.cs
- DesignerTextWriter.cs
- DataGridViewLinkColumn.cs