Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / IO / StringWriter.cs / 1305376 / StringWriter.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: StringWriter ** **[....] ** ** Purpose: For writing text to a string ** ** ===========================================================*/ using System; using System.Runtime; using System.Text; using System.Globalization; using System.Diagnostics.Contracts; namespace System.IO { // This class implements a text writer that writes to a string buffer and allows // the resulting sequence of characters to be presented as a string. // [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public class StringWriter : TextWriter { private static UnicodeEncoding m_encoding=null; private StringBuilder _sb; private bool _isOpen; // Constructs a new StringWriter. A new StringBuilder is automatically // created and associated with the new StringWriter. #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public StringWriter() : this(new StringBuilder(), CultureInfo.CurrentCulture) { } #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public StringWriter(IFormatProvider formatProvider) : this(new StringBuilder(), formatProvider) { } // Constructs a new StringWriter that writes to the given StringBuilder. // #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public StringWriter(StringBuilder sb) : this(sb, CultureInfo.CurrentCulture) { } public StringWriter(StringBuilder sb, IFormatProvider formatProvider) : base(formatProvider) { if (sb==null) throw new ArgumentNullException("sb", Environment.GetResourceString("ArgumentNull_Buffer")); Contract.EndContractBlock(); _sb = sb; _isOpen = true; } #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public override void Close() { Dispose(true); } #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif protected override void Dispose(bool disposing) { // Do not destroy _sb, so that we can extract this after we are // done writing (similar to MemoryStream's GetBuffer & ToArray methods) _isOpen = false; base.Dispose(disposing); } public override Encoding Encoding { #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif get { if (m_encoding==null) { m_encoding = new UnicodeEncoding(false, false); } return m_encoding; } } // Returns the underlying StringBuilder. This is either the StringBuilder // that was passed to the constructor, or the StringBuilder that was // automatically created. // public virtual StringBuilder GetStringBuilder() { return _sb; } // Writes a character to the underlying string buffer. // #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public override void Write(char value) { if (!_isOpen) __Error.WriterClosed(); _sb.Append(value); } // Writes a range of a character array to the underlying string buffer. // This method will write count characters of data into this // StringWriter from the buffer character array starting at position // index. // public override void Write(char[] buffer, int index, int count) { if (buffer==null) throw new ArgumentNullException("buffer", Environment.GetResourceString("ArgumentNull_Buffer")); if (index < 0) throw new ArgumentOutOfRangeException("index", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); if (count < 0) throw new ArgumentOutOfRangeException("count", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); if (buffer.Length - index < count) throw new ArgumentException(Environment.GetResourceString("Argument_InvalidOffLen")); Contract.EndContractBlock(); if (!_isOpen) __Error.WriterClosed(); _sb.Append(buffer, index, count); } // Writes a string to the underlying string buffer. If the given string is // null, nothing is written. // #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public override void Write(String value) { if (!_isOpen) __Error.WriterClosed(); if (value != null) _sb.Append(value); } // Returns a string containing the characters written to this TextWriter // so far. // #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public override String ToString() { return _sb.ToString(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
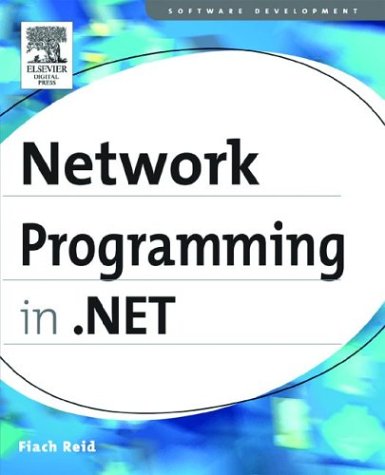
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WmpBitmapDecoder.cs
- StringFormat.cs
- XmlWrappingReader.cs
- AsymmetricSignatureFormatter.cs
- ValidationErrorCollection.cs
- XmlDictionaryWriter.cs
- ModifierKeysConverter.cs
- SHA384Managed.cs
- DayRenderEvent.cs
- XmlQueryRuntime.cs
- cryptoapiTransform.cs
- MonthChangedEventArgs.cs
- DynamicControlParameter.cs
- ObjectDataSourceDisposingEventArgs.cs
- FactoryRecord.cs
- HttpConfigurationSystem.cs
- ConfigXmlElement.cs
- HtmlProps.cs
- PropertyPath.cs
- AnnotationResource.cs
- X509CertificateValidator.cs
- XmlSchemaInferenceException.cs
- PrintController.cs
- Tablet.cs
- DataKeyArray.cs
- TimeSpanMinutesOrInfiniteConverter.cs
- ObjectSet.cs
- XNodeNavigator.cs
- AppearanceEditorPart.cs
- XmlCodeExporter.cs
- DataGridViewTextBoxCell.cs
- XmlDataLoader.cs
- XPathNodeList.cs
- TemplateComponentConnector.cs
- InkCanvasSelectionAdorner.cs
- Point.cs
- PolicyManager.cs
- XmlLinkedNode.cs
- tibetanshape.cs
- FusionWrap.cs
- WindowsFormsLinkLabel.cs
- WorkflowServiceBehavior.cs
- RichTextBox.cs
- PropertyEntry.cs
- SecurityBindingElement.cs
- XComponentModel.cs
- RawAppCommandInputReport.cs
- FacetDescription.cs
- ClockGroup.cs
- XPathNodeHelper.cs
- MSAAEventDispatcher.cs
- ToolStripPanelCell.cs
- CompilerParameters.cs
- InputGestureCollection.cs
- MimeXmlReflector.cs
- NativeMethodsCLR.cs
- ParagraphVisual.cs
- OdbcStatementHandle.cs
- PaperSource.cs
- ClientUtils.cs
- HelpHtmlBuilder.cs
- TypeUsage.cs
- Int32Converter.cs
- XmlAggregates.cs
- HttpsChannelListener.cs
- XmlRawWriterWrapper.cs
- TransformPattern.cs
- SqlFlattener.cs
- AutomationElement.cs
- Label.cs
- CompensationDesigner.cs
- SqlConnectionManager.cs
- PropertyPathWorker.cs
- ObjectDataSourceStatusEventArgs.cs
- ReadWriteObjectLock.cs
- ProcessHostConfigUtils.cs
- DataGridTablesFactory.cs
- CompositionTarget.cs
- HyperLinkField.cs
- ProgressBarBrushConverter.cs
- Geometry.cs
- HtmlMeta.cs
- RemoteWebConfigurationHostServer.cs
- ManagementNamedValueCollection.cs
- ReferencedAssembly.cs
- __ConsoleStream.cs
- EventManager.cs
- DataGridTable.cs
- HijriCalendar.cs
- XmlWrappingReader.cs
- XmlExceptionHelper.cs
- PrtCap_Public.cs
- WsatTransactionHeader.cs
- PasswordBox.cs
- XmlProcessingInstruction.cs
- elementinformation.cs
- XmlSchemaValidationException.cs
- ArrayEditor.cs
- DocumentSchemaValidator.cs
- BooleanProjectedSlot.cs