Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / Configuration / ProcessHostConfigUtils.cs / 2 / ProcessHostConfigUtils.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System.Configuration; using System.Collections; using System.Globalization; using System.Runtime.InteropServices; using System.Threading; using System.Xml; using System.Security; using System.Text; using System.Web.Util; using System.Web.UI; using System.IO; using System.Web.Hosting; using System.Runtime.ConstrainedExecution; // // Uses IIS 7 native config // internal static class ProcessHostConfigUtils { internal const uint DEFAULT_SITE_ID_UINT = 1; internal const string DEFAULT_SITE_ID_STRING = "1"; private static string s_defaultSiteName; private static int s_InitedExternalConfig; private static NativeConfigWrapper _configWrapper; // static class ctor static ProcessHostConfigUtils() { HttpRuntime.ForceStaticInit(); } internal static void InitStandaloneConfig() { if (!HostingEnvironment.IsUnderIISProcess) { if (!ServerConfig.UseMetabase) { int inited= Interlocked.Exchange(ref s_InitedExternalConfig, 1); // only do this once if (0 == inited) { _configWrapper = new NativeConfigWrapper(); } } } } internal static string MapPathActual(string siteName, VirtualPath path) { string physicalPath = null; IntPtr pBstr = IntPtr.Zero; int cBstr = 0; try { int result = UnsafeIISMethods.MgdMapPathDirect(siteName, path.VirtualPathString, out pBstr, out cBstr); if (result < 0) { throw new InvalidOperationException(SR.GetString(SR.Cannot_map_path, path.VirtualPathString)); } physicalPath = (pBstr != IntPtr.Zero) ? StringUtil.StringFromWCharPtr(pBstr, cBstr) : null; } finally { if (pBstr != IntPtr.Zero) { Marshal.FreeBSTR(pBstr); } } return physicalPath; } internal static string GetSiteNameFromId(uint siteId) { if ( siteId == DEFAULT_SITE_ID_UINT && s_defaultSiteName != null) { return s_defaultSiteName; } IntPtr pBstr = IntPtr.Zero; int cBstr = 0; string siteName = null; try { int result = UnsafeIISMethods.MgdGetSiteNameFromId(siteId, out pBstr, out cBstr); siteName = (result == 0 && pBstr != IntPtr.Zero) ? StringUtil.StringFromWCharPtr(pBstr, cBstr) : String.Empty; } finally { if (pBstr != IntPtr.Zero) { Marshal.FreeBSTR(pBstr); } } if ( siteId == DEFAULT_SITE_ID_UINT) { s_defaultSiteName = siteName; } return siteName; } private class NativeConfigWrapper : CriticalFinalizerObject { internal NativeConfigWrapper() { int result = UnsafeIISMethods.MgdInitNativeConfig(); if (result < 0) { s_InitedExternalConfig = 0; throw new InvalidOperationException(SR.GetString(SR.Cant_Init_Native_Config, result.ToString("X8", CultureInfo.InvariantCulture))); } } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] ~NativeConfigWrapper() { UnsafeIISMethods.MgdTerminateNativeConfig(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System.Configuration; using System.Collections; using System.Globalization; using System.Runtime.InteropServices; using System.Threading; using System.Xml; using System.Security; using System.Text; using System.Web.Util; using System.Web.UI; using System.IO; using System.Web.Hosting; using System.Runtime.ConstrainedExecution; // // Uses IIS 7 native config // internal static class ProcessHostConfigUtils { internal const uint DEFAULT_SITE_ID_UINT = 1; internal const string DEFAULT_SITE_ID_STRING = "1"; private static string s_defaultSiteName; private static int s_InitedExternalConfig; private static NativeConfigWrapper _configWrapper; // static class ctor static ProcessHostConfigUtils() { HttpRuntime.ForceStaticInit(); } internal static void InitStandaloneConfig() { if (!HostingEnvironment.IsUnderIISProcess) { if (!ServerConfig.UseMetabase) { int inited= Interlocked.Exchange(ref s_InitedExternalConfig, 1); // only do this once if (0 == inited) { _configWrapper = new NativeConfigWrapper(); } } } } internal static string MapPathActual(string siteName, VirtualPath path) { string physicalPath = null; IntPtr pBstr = IntPtr.Zero; int cBstr = 0; try { int result = UnsafeIISMethods.MgdMapPathDirect(siteName, path.VirtualPathString, out pBstr, out cBstr); if (result < 0) { throw new InvalidOperationException(SR.GetString(SR.Cannot_map_path, path.VirtualPathString)); } physicalPath = (pBstr != IntPtr.Zero) ? StringUtil.StringFromWCharPtr(pBstr, cBstr) : null; } finally { if (pBstr != IntPtr.Zero) { Marshal.FreeBSTR(pBstr); } } return physicalPath; } internal static string GetSiteNameFromId(uint siteId) { if ( siteId == DEFAULT_SITE_ID_UINT && s_defaultSiteName != null) { return s_defaultSiteName; } IntPtr pBstr = IntPtr.Zero; int cBstr = 0; string siteName = null; try { int result = UnsafeIISMethods.MgdGetSiteNameFromId(siteId, out pBstr, out cBstr); siteName = (result == 0 && pBstr != IntPtr.Zero) ? StringUtil.StringFromWCharPtr(pBstr, cBstr) : String.Empty; } finally { if (pBstr != IntPtr.Zero) { Marshal.FreeBSTR(pBstr); } } if ( siteId == DEFAULT_SITE_ID_UINT) { s_defaultSiteName = siteName; } return siteName; } private class NativeConfigWrapper : CriticalFinalizerObject { internal NativeConfigWrapper() { int result = UnsafeIISMethods.MgdInitNativeConfig(); if (result < 0) { s_InitedExternalConfig = 0; throw new InvalidOperationException(SR.GetString(SR.Cant_Init_Native_Config, result.ToString("X8", CultureInfo.InvariantCulture))); } } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] ~NativeConfigWrapper() { UnsafeIISMethods.MgdTerminateNativeConfig(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
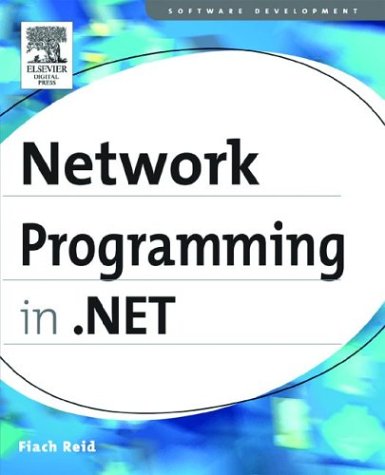
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BinaryFormatter.cs
- Brushes.cs
- DisableDpiAwarenessAttribute.cs
- SystemFonts.cs
- grammarelement.cs
- Transform3D.cs
- InvokeDelegate.cs
- OdbcInfoMessageEvent.cs
- coordinatorfactory.cs
- DesignerForm.cs
- SerialReceived.cs
- __Error.cs
- URLBuilder.cs
- WindowsTokenRoleProvider.cs
- basenumberconverter.cs
- GeneralTransform3D.cs
- CharStorage.cs
- MexTcpBindingCollectionElement.cs
- XmlQueryContext.cs
- SectionVisual.cs
- Rect3DValueSerializer.cs
- ObjectStorage.cs
- SqlUtil.cs
- DiagnosticsConfiguration.cs
- NavigationPropertyEmitter.cs
- ApplicationActivator.cs
- IndicCharClassifier.cs
- WmfPlaceableFileHeader.cs
- ApplicationServiceHelper.cs
- DataContractJsonSerializer.cs
- RectConverter.cs
- PingOptions.cs
- TemplateBindingExpressionConverter.cs
- COM2EnumConverter.cs
- RelationshipSet.cs
- Int32RectValueSerializer.cs
- Expr.cs
- Literal.cs
- ArgumentException.cs
- ResourceDefaultValueAttribute.cs
- RelationshipEnd.cs
- MimeTypeMapper.cs
- HttpRequest.cs
- ListViewDataItem.cs
- CellParaClient.cs
- ServiceCredentials.cs
- ParserHooks.cs
- xsdvalidator.cs
- ObjectHelper.cs
- PageCache.cs
- AutomationPattern.cs
- ExtendedProtectionPolicy.cs
- InkPresenter.cs
- PieceNameHelper.cs
- VirtualPath.cs
- HttpWebRequestElement.cs
- ReachNamespaceInfo.cs
- JsonServiceDocumentSerializer.cs
- Shape.cs
- UserPersonalizationStateInfo.cs
- ScriptComponentDescriptor.cs
- TypeInfo.cs
- ProviderConnectionPointCollection.cs
- Parallel.cs
- ImageFormatConverter.cs
- WmiEventSink.cs
- PropertyItemInternal.cs
- HitTestDrawingContextWalker.cs
- UIElement.cs
- CryptoApi.cs
- XmlRootAttribute.cs
- SignatureDescription.cs
- TableItemPattern.cs
- ContentFileHelper.cs
- XmlWellformedWriter.cs
- ProvidersHelper.cs
- CodeChecksumPragma.cs
- METAHEADER.cs
- WebServiceEnumData.cs
- TransformerInfo.cs
- ThicknessAnimation.cs
- RemotingSurrogateSelector.cs
- GcSettings.cs
- RuntimeArgumentHandle.cs
- TraceHwndHost.cs
- BasePattern.cs
- XmlJsonReader.cs
- SiteMapHierarchicalDataSourceView.cs
- FtpWebRequest.cs
- StylusShape.cs
- FaultException.cs
- NativeMethods.cs
- SpellerError.cs
- IdnMapping.cs
- Enum.cs
- DetailsViewRow.cs
- RangeValidator.cs
- EpmCustomContentWriterNodeData.cs
- ValuePattern.cs
- KeyNotFoundException.cs