Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / MS / Internal / PtsHost / ParagraphVisual.cs / 1 / ParagraphVisual.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: ParagraphVisaul.cs // // Description: Visual representing a paragraph. // // History: // 05/20/2003 : grzegorz - created. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; namespace MS.Internal.PtsHost { ////// Visual representing a paragraph. /// internal class ParagraphVisual : DrawingVisual { ////// Constructor. /// internal ParagraphVisual() { _renderBounds = Rect.Empty; } ////// Draw background and border. /// /// The brush used for background. /// The brush used for border. /// Border thickness. /// Render bounds of the visual. /// Whether this is paragraph's first chunk. /// Whether this is paragraph's last chunk. internal void DrawBackgroundAndBorder(Brush backgroundBrush, Brush borderBrush, Thickness borderThickness, Rect renderBounds, bool isFirstChunk, bool isLastChunk) { if (_backgroundBrush != backgroundBrush || _renderBounds != renderBounds || _borderBrush != borderBrush || !Thickness.AreClose(_borderThickness, borderThickness)) { // Open DrawingContext and draw background. using (DrawingContext dc = RenderOpen()) { DrawBackgroundAndBorderIntoContext(dc, backgroundBrush, borderBrush, borderThickness, renderBounds, isFirstChunk, isLastChunk); } } } ////// Draw background and border. /// /// Drawing context. /// The brush used for background. /// The brush used for border. /// Border thickness. /// Render bounds of the visual. /// Whether this is paragraph's first chunk. /// Whether this is paragraph's last chunk. internal void DrawBackgroundAndBorderIntoContext(DrawingContext dc, Brush backgroundBrush, Brush borderBrush, Thickness borderThickness, Rect renderBounds, bool isFirstChunk, bool isLastChunk) { // We do not want to cause the user's Brushes to become frozen when we // freeze the Pen in OnRender, therefore we make our own copy of the // Brushes if they are not already frozen. _backgroundBrush = (Brush)FreezableOperations.GetAsFrozenIfPossible(backgroundBrush); _renderBounds = renderBounds; _borderBrush = (Brush)FreezableOperations.GetAsFrozenIfPossible(borderBrush); _borderThickness = borderThickness; // Exclude top/bottom border if this is not the first/last chunk of the paragraph. if (!isFirstChunk) { _borderThickness.Top = 0.0; } if (!isLastChunk) { _borderThickness.Bottom = 0.0; } // If we have a brush with which to draw the border, do so. // NB: We double draw corners right now. Corner handling is tricky (bevelling, &c...) and // we need a firm spec before doing "the right thing." (greglett, ffortes) if (_borderBrush != null) { // Initialize the first pen. Note that each pen is created via new() // and frozen if possible. Doing this avoids the overhead of // maintaining changed handlers. Pen pen = new Pen(); pen.Brush = _borderBrush; pen.Thickness = _borderThickness.Left; if (pen.CanFreeze) { pen.Freeze(); } if (_borderThickness.IsUniform) { // Uniform border; stroke a rectangle. dc.DrawRectangle(null, pen, new Rect( new Point(_renderBounds.Left + pen.Thickness * 0.5, _renderBounds.Bottom - pen.Thickness * 0.5), new Point(_renderBounds.Right - pen.Thickness * 0.5, _renderBounds.Top + pen.Thickness * 0.5))); } else { if (DoubleUtil.GreaterThan(_borderThickness.Left, 0)) { dc.DrawLine(pen, new Point(_renderBounds.Left + pen.Thickness / 2, _renderBounds.Top), new Point(_renderBounds.Left + pen.Thickness / 2, _renderBounds.Bottom)); } if (DoubleUtil.GreaterThan(_borderThickness.Right, 0)) { pen = new Pen(); pen.Brush = _borderBrush; pen.Thickness = _borderThickness.Right; if (pen.CanFreeze) { pen.Freeze(); } dc.DrawLine(pen, new Point(_renderBounds.Right - pen.Thickness / 2, _renderBounds.Top), new Point(_renderBounds.Right - pen.Thickness / 2, _renderBounds.Bottom)); } if (DoubleUtil.GreaterThan(_borderThickness.Top, 0)) { pen = new Pen(); pen.Brush = _borderBrush; pen.Thickness = _borderThickness.Top; if (pen.CanFreeze) { pen.Freeze(); } dc.DrawLine(pen, new Point(_renderBounds.Left, _renderBounds.Top + pen.Thickness / 2), new Point(_renderBounds.Right, _renderBounds.Top + pen.Thickness / 2)); } if (DoubleUtil.GreaterThan(_borderThickness.Bottom, 0)) { pen = new Pen(); pen.Brush = _borderBrush; pen.Thickness = _borderThickness.Bottom; if (pen.CanFreeze) { pen.Freeze(); } dc.DrawLine(pen, new Point(_renderBounds.Left, _renderBounds.Bottom - pen.Thickness / 2), new Point(_renderBounds.Right, _renderBounds.Bottom - pen.Thickness / 2)); } } } // Draw background in rectangle inside border. if (_backgroundBrush != null) { dc.DrawRectangle(_backgroundBrush, null, new Rect( new Point(_renderBounds.Left + _borderThickness.Left, _renderBounds.Top + _borderThickness.Top), new Point(_renderBounds.Right - _borderThickness.Right, _renderBounds.Bottom - _borderThickness.Bottom))); } } private Brush _backgroundBrush; // Background brush private Brush _borderBrush; // Border brush private Thickness _borderThickness; // Border thickness private Rect _renderBounds; // Render bounds of the visual } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: ParagraphVisaul.cs // // Description: Visual representing a paragraph. // // History: // 05/20/2003 : grzegorz - created. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; namespace MS.Internal.PtsHost { ////// Visual representing a paragraph. /// internal class ParagraphVisual : DrawingVisual { ////// Constructor. /// internal ParagraphVisual() { _renderBounds = Rect.Empty; } ////// Draw background and border. /// /// The brush used for background. /// The brush used for border. /// Border thickness. /// Render bounds of the visual. /// Whether this is paragraph's first chunk. /// Whether this is paragraph's last chunk. internal void DrawBackgroundAndBorder(Brush backgroundBrush, Brush borderBrush, Thickness borderThickness, Rect renderBounds, bool isFirstChunk, bool isLastChunk) { if (_backgroundBrush != backgroundBrush || _renderBounds != renderBounds || _borderBrush != borderBrush || !Thickness.AreClose(_borderThickness, borderThickness)) { // Open DrawingContext and draw background. using (DrawingContext dc = RenderOpen()) { DrawBackgroundAndBorderIntoContext(dc, backgroundBrush, borderBrush, borderThickness, renderBounds, isFirstChunk, isLastChunk); } } } ////// Draw background and border. /// /// Drawing context. /// The brush used for background. /// The brush used for border. /// Border thickness. /// Render bounds of the visual. /// Whether this is paragraph's first chunk. /// Whether this is paragraph's last chunk. internal void DrawBackgroundAndBorderIntoContext(DrawingContext dc, Brush backgroundBrush, Brush borderBrush, Thickness borderThickness, Rect renderBounds, bool isFirstChunk, bool isLastChunk) { // We do not want to cause the user's Brushes to become frozen when we // freeze the Pen in OnRender, therefore we make our own copy of the // Brushes if they are not already frozen. _backgroundBrush = (Brush)FreezableOperations.GetAsFrozenIfPossible(backgroundBrush); _renderBounds = renderBounds; _borderBrush = (Brush)FreezableOperations.GetAsFrozenIfPossible(borderBrush); _borderThickness = borderThickness; // Exclude top/bottom border if this is not the first/last chunk of the paragraph. if (!isFirstChunk) { _borderThickness.Top = 0.0; } if (!isLastChunk) { _borderThickness.Bottom = 0.0; } // If we have a brush with which to draw the border, do so. // NB: We double draw corners right now. Corner handling is tricky (bevelling, &c...) and // we need a firm spec before doing "the right thing." (greglett, ffortes) if (_borderBrush != null) { // Initialize the first pen. Note that each pen is created via new() // and frozen if possible. Doing this avoids the overhead of // maintaining changed handlers. Pen pen = new Pen(); pen.Brush = _borderBrush; pen.Thickness = _borderThickness.Left; if (pen.CanFreeze) { pen.Freeze(); } if (_borderThickness.IsUniform) { // Uniform border; stroke a rectangle. dc.DrawRectangle(null, pen, new Rect( new Point(_renderBounds.Left + pen.Thickness * 0.5, _renderBounds.Bottom - pen.Thickness * 0.5), new Point(_renderBounds.Right - pen.Thickness * 0.5, _renderBounds.Top + pen.Thickness * 0.5))); } else { if (DoubleUtil.GreaterThan(_borderThickness.Left, 0)) { dc.DrawLine(pen, new Point(_renderBounds.Left + pen.Thickness / 2, _renderBounds.Top), new Point(_renderBounds.Left + pen.Thickness / 2, _renderBounds.Bottom)); } if (DoubleUtil.GreaterThan(_borderThickness.Right, 0)) { pen = new Pen(); pen.Brush = _borderBrush; pen.Thickness = _borderThickness.Right; if (pen.CanFreeze) { pen.Freeze(); } dc.DrawLine(pen, new Point(_renderBounds.Right - pen.Thickness / 2, _renderBounds.Top), new Point(_renderBounds.Right - pen.Thickness / 2, _renderBounds.Bottom)); } if (DoubleUtil.GreaterThan(_borderThickness.Top, 0)) { pen = new Pen(); pen.Brush = _borderBrush; pen.Thickness = _borderThickness.Top; if (pen.CanFreeze) { pen.Freeze(); } dc.DrawLine(pen, new Point(_renderBounds.Left, _renderBounds.Top + pen.Thickness / 2), new Point(_renderBounds.Right, _renderBounds.Top + pen.Thickness / 2)); } if (DoubleUtil.GreaterThan(_borderThickness.Bottom, 0)) { pen = new Pen(); pen.Brush = _borderBrush; pen.Thickness = _borderThickness.Bottom; if (pen.CanFreeze) { pen.Freeze(); } dc.DrawLine(pen, new Point(_renderBounds.Left, _renderBounds.Bottom - pen.Thickness / 2), new Point(_renderBounds.Right, _renderBounds.Bottom - pen.Thickness / 2)); } } } // Draw background in rectangle inside border. if (_backgroundBrush != null) { dc.DrawRectangle(_backgroundBrush, null, new Rect( new Point(_renderBounds.Left + _borderThickness.Left, _renderBounds.Top + _borderThickness.Top), new Point(_renderBounds.Right - _borderThickness.Right, _renderBounds.Bottom - _borderThickness.Bottom))); } } private Brush _backgroundBrush; // Background brush private Brush _borderBrush; // Border brush private Thickness _borderThickness; // Border thickness private Rect _renderBounds; // Render bounds of the visual } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
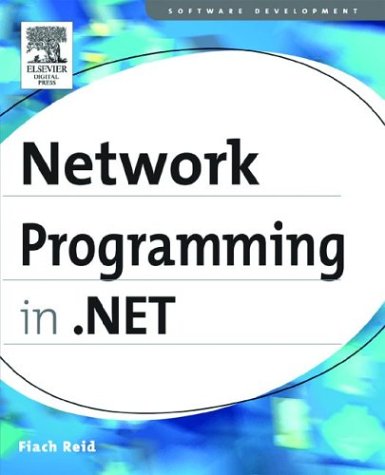
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProviderUtil.cs
- RectAnimationBase.cs
- GridViewSortEventArgs.cs
- SafeLocalMemHandle.cs
- DrawingContextDrawingContextWalker.cs
- BaseComponentEditor.cs
- TextViewBase.cs
- StylusCaptureWithinProperty.cs
- SizeAnimationClockResource.cs
- ResourcesBuildProvider.cs
- AuthenticationConfig.cs
- CallbackValidator.cs
- SendKeys.cs
- ColumnWidthChangedEvent.cs
- HostedHttpRequestAsyncResult.cs
- FontCollection.cs
- CoTaskMemHandle.cs
- TableLayoutRowStyleCollection.cs
- ListViewUpdatedEventArgs.cs
- GenerateTemporaryTargetAssembly.cs
- IODescriptionAttribute.cs
- DataGridItemEventArgs.cs
- OutputCacheSection.cs
- ChangeConflicts.cs
- DataTableReader.cs
- Quad.cs
- DelegateSerializationHolder.cs
- EmptyQuery.cs
- Comparer.cs
- UpdateProgress.cs
- MergablePropertyAttribute.cs
- Oid.cs
- ObjectTag.cs
- SocketAddress.cs
- MessageSecurityVersionConverter.cs
- CodeComment.cs
- Pens.cs
- LiteralControl.cs
- PluralizationServiceUtil.cs
- HttpHandlerAction.cs
- DynamicObjectAccessor.cs
- DependentList.cs
- ItemAutomationPeer.cs
- TransactionBridgeSection.cs
- PointAnimationBase.cs
- DataContractSerializerFaultFormatter.cs
- SqlCommandBuilder.cs
- ProfileServiceManager.cs
- PngBitmapEncoder.cs
- HostingPreferredMapPath.cs
- NetworkCredential.cs
- thaishape.cs
- RegexWriter.cs
- MonikerSyntaxException.cs
- HttpCacheVary.cs
- Stream.cs
- TrackingStringDictionary.cs
- SynchronizationHandlesCodeDomSerializer.cs
- Guid.cs
- TcpClientSocketManager.cs
- DBNull.cs
- XPathSelectionIterator.cs
- BatchStream.cs
- FaultContext.cs
- TextEmbeddedObject.cs
- WasHostedComPlusFactory.cs
- wmiprovider.cs
- AnimationStorage.cs
- EntityDesignerDataSourceView.cs
- MulticastOption.cs
- Permission.cs
- Vector3DCollectionValueSerializer.cs
- FileUpload.cs
- FixedDSBuilder.cs
- HelpProvider.cs
- SafeLocalMemHandle.cs
- Int16Storage.cs
- TypeUtil.cs
- ThemeDirectoryCompiler.cs
- ScriptResourceHandler.cs
- JumpPath.cs
- HtmlHead.cs
- SvcMapFileLoader.cs
- QilLoop.cs
- XsltSettings.cs
- UniformGrid.cs
- InternalConfigSettingsFactory.cs
- XPathEmptyIterator.cs
- XmlSchemaType.cs
- EntityRecordInfo.cs
- SafeProcessHandle.cs
- OracleTransaction.cs
- EventRouteFactory.cs
- UdpDiscoveryMessageFilter.cs
- StringFormat.cs
- ThicknessKeyFrameCollection.cs
- DataServiceQuery.cs
- HttpEncoderUtility.cs
- SystemResources.cs
- ResourceDisplayNameAttribute.cs