Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / CompMod / System / CodeDOM / Compiler / CompilerInfo.cs / 1 / CompilerInfo.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.CodeDom.Compiler { using System; using System.Reflection; using System.Security.Permissions; using System.CodeDom.Compiler; using System.Configuration; using System.Collections.Generic; using System.Diagnostics; [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] public sealed class CompilerInfo { internal String _codeDomProviderTypeName; // This can never by null internal CompilerParameters _compilerParams; // This can never by null internal String[] _compilerLanguages; // This can never by null internal String[] _compilerExtensions; // This can never by null internal String configFileName; internal IDictionary_providerOptions; // This can never be null internal int configFileLineNumber; internal Boolean _mapped; private Type type; private CompilerInfo() {} // Not createable public String[] GetLanguages() { return CloneCompilerLanguages(); } public String[] GetExtensions() { return CloneCompilerExtensions(); } public Type CodeDomProviderType { get { if( type == null) { lock(this) { if( type == null) { type = Type.GetType(_codeDomProviderTypeName); if (type == null) { if( configFileName == null) { throw new ConfigurationErrorsException(SR.GetString(SR.Unable_To_Locate_Type, _codeDomProviderTypeName, string.Empty, 0)); } else { throw new ConfigurationErrorsException(SR.GetString(SR.Unable_To_Locate_Type, _codeDomProviderTypeName), configFileName, configFileLineNumber); } } } } } return type; } } public bool IsCodeDomProviderTypeValid { get { Type type = Type.GetType(_codeDomProviderTypeName); return (type != null); } } public CodeDomProvider CreateProvider() { // if the provider defines an IDictionary ctor and // provider options have been provided then call that and give it the // provider options dictionary. Otherwise call the normal one. Debug.Assert(_providerOptions != null, "Created CompilerInfo w/ null _providerOptions"); if (_providerOptions.Count > 0) { ConstructorInfo ci = CodeDomProviderType.GetConstructor(new Type[] { typeof(IDictionary ) }); if (ci != null) { return (CodeDomProvider)ci.Invoke(new object[] { _providerOptions }); } } return (CodeDomProvider)Activator.CreateInstance(CodeDomProviderType); } public CompilerParameters CreateDefaultCompilerParameters() { return CloneCompilerParameters(); } internal CompilerInfo(CompilerParameters compilerParams, String codeDomProviderTypeName, String [] compilerLanguages, String [] compilerExtensions) { _compilerLanguages = compilerLanguages; _compilerExtensions = compilerExtensions; _codeDomProviderTypeName = codeDomProviderTypeName; if (compilerParams == null) compilerParams = new CompilerParameters(); _compilerParams = compilerParams; } internal CompilerInfo(CompilerParameters compilerParams, String codeDomProviderTypeName) { _codeDomProviderTypeName = codeDomProviderTypeName; if (compilerParams == null) compilerParams = new CompilerParameters(); _compilerParams = compilerParams; } public override int GetHashCode() { return _codeDomProviderTypeName.GetHashCode(); } public override bool Equals(Object o) { CompilerInfo other = o as CompilerInfo; if (o == null) return false; return CodeDomProviderType == other.CodeDomProviderType && CompilerParams.WarningLevel == other.CompilerParams.WarningLevel && CompilerParams.IncludeDebugInformation == other.CompilerParams.IncludeDebugInformation && CompilerParams.CompilerOptions == other.CompilerParams.CompilerOptions; } private CompilerParameters CloneCompilerParameters() { CompilerParameters copy = new CompilerParameters(); copy.IncludeDebugInformation = _compilerParams.IncludeDebugInformation; copy.TreatWarningsAsErrors = _compilerParams.TreatWarningsAsErrors; copy.WarningLevel = _compilerParams.WarningLevel; copy.CompilerOptions = _compilerParams.CompilerOptions; return copy; } private String[] CloneCompilerLanguages() { String[] compilerLanguages = new String[_compilerLanguages.Length]; Array.Copy(_compilerLanguages, compilerLanguages, _compilerLanguages.Length); return compilerLanguages; } private String[] CloneCompilerExtensions() { String[] compilerExtensions = new String[_compilerExtensions.Length]; Array.Copy(_compilerExtensions, compilerExtensions, _compilerExtensions.Length); return compilerExtensions; } internal CompilerParameters CompilerParams { get { return _compilerParams; } } // @ internal IDictionary ProviderOptions { get { return _providerOptions; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.CodeDom.Compiler { using System; using System.Reflection; using System.Security.Permissions; using System.CodeDom.Compiler; using System.Configuration; using System.Collections.Generic; using System.Diagnostics; [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] public sealed class CompilerInfo { internal String _codeDomProviderTypeName; // This can never by null internal CompilerParameters _compilerParams; // This can never by null internal String[] _compilerLanguages; // This can never by null internal String[] _compilerExtensions; // This can never by null internal String configFileName; internal IDictionary_providerOptions; // This can never be null internal int configFileLineNumber; internal Boolean _mapped; private Type type; private CompilerInfo() {} // Not createable public String[] GetLanguages() { return CloneCompilerLanguages(); } public String[] GetExtensions() { return CloneCompilerExtensions(); } public Type CodeDomProviderType { get { if( type == null) { lock(this) { if( type == null) { type = Type.GetType(_codeDomProviderTypeName); if (type == null) { if( configFileName == null) { throw new ConfigurationErrorsException(SR.GetString(SR.Unable_To_Locate_Type, _codeDomProviderTypeName, string.Empty, 0)); } else { throw new ConfigurationErrorsException(SR.GetString(SR.Unable_To_Locate_Type, _codeDomProviderTypeName), configFileName, configFileLineNumber); } } } } } return type; } } public bool IsCodeDomProviderTypeValid { get { Type type = Type.GetType(_codeDomProviderTypeName); return (type != null); } } public CodeDomProvider CreateProvider() { // if the provider defines an IDictionary ctor and // provider options have been provided then call that and give it the // provider options dictionary. Otherwise call the normal one. Debug.Assert(_providerOptions != null, "Created CompilerInfo w/ null _providerOptions"); if (_providerOptions.Count > 0) { ConstructorInfo ci = CodeDomProviderType.GetConstructor(new Type[] { typeof(IDictionary ) }); if (ci != null) { return (CodeDomProvider)ci.Invoke(new object[] { _providerOptions }); } } return (CodeDomProvider)Activator.CreateInstance(CodeDomProviderType); } public CompilerParameters CreateDefaultCompilerParameters() { return CloneCompilerParameters(); } internal CompilerInfo(CompilerParameters compilerParams, String codeDomProviderTypeName, String [] compilerLanguages, String [] compilerExtensions) { _compilerLanguages = compilerLanguages; _compilerExtensions = compilerExtensions; _codeDomProviderTypeName = codeDomProviderTypeName; if (compilerParams == null) compilerParams = new CompilerParameters(); _compilerParams = compilerParams; } internal CompilerInfo(CompilerParameters compilerParams, String codeDomProviderTypeName) { _codeDomProviderTypeName = codeDomProviderTypeName; if (compilerParams == null) compilerParams = new CompilerParameters(); _compilerParams = compilerParams; } public override int GetHashCode() { return _codeDomProviderTypeName.GetHashCode(); } public override bool Equals(Object o) { CompilerInfo other = o as CompilerInfo; if (o == null) return false; return CodeDomProviderType == other.CodeDomProviderType && CompilerParams.WarningLevel == other.CompilerParams.WarningLevel && CompilerParams.IncludeDebugInformation == other.CompilerParams.IncludeDebugInformation && CompilerParams.CompilerOptions == other.CompilerParams.CompilerOptions; } private CompilerParameters CloneCompilerParameters() { CompilerParameters copy = new CompilerParameters(); copy.IncludeDebugInformation = _compilerParams.IncludeDebugInformation; copy.TreatWarningsAsErrors = _compilerParams.TreatWarningsAsErrors; copy.WarningLevel = _compilerParams.WarningLevel; copy.CompilerOptions = _compilerParams.CompilerOptions; return copy; } private String[] CloneCompilerLanguages() { String[] compilerLanguages = new String[_compilerLanguages.Length]; Array.Copy(_compilerLanguages, compilerLanguages, _compilerLanguages.Length); return compilerLanguages; } private String[] CloneCompilerExtensions() { String[] compilerExtensions = new String[_compilerExtensions.Length]; Array.Copy(_compilerExtensions, compilerExtensions, _compilerExtensions.Length); return compilerExtensions; } internal CompilerParameters CompilerParams { get { return _compilerParams; } } // @ internal IDictionary ProviderOptions { get { return _providerOptions; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
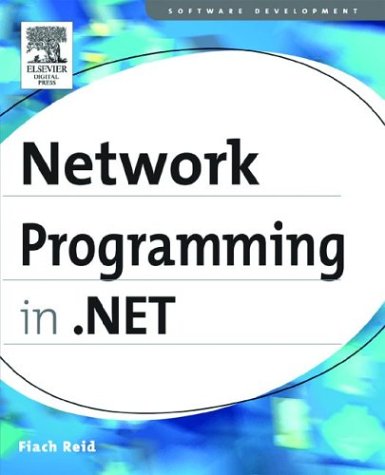
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpCapabilitiesEvaluator.cs
- Annotation.cs
- IpcChannel.cs
- SyntaxCheck.cs
- DynamicILGenerator.cs
- PipelineDeploymentState.cs
- SoundPlayerAction.cs
- MarkerProperties.cs
- WebHttpDispatchOperationSelector.cs
- AlphabeticalEnumConverter.cs
- WebPartManager.cs
- ColorConverter.cs
- HtmlToClrEventProxy.cs
- ExcCanonicalXml.cs
- RSAPKCS1SignatureFormatter.cs
- HashSetDebugView.cs
- XmlSchemaAttribute.cs
- SecurityProtocolCorrelationState.cs
- PointLight.cs
- ScrollBar.cs
- Application.cs
- LinkLabelLinkClickedEvent.cs
- xdrvalidator.cs
- _NetRes.cs
- FunctionImportElement.cs
- LookupTables.cs
- OdbcPermission.cs
- ObjectDataSourceFilteringEventArgs.cs
- SchemaAttDef.cs
- DuplicateWaitObjectException.cs
- DynamicResourceExtension.cs
- XmlResolver.cs
- PixelShader.cs
- ElementsClipboardData.cs
- TextViewBase.cs
- EntityCollection.cs
- ProfileSection.cs
- AmbientLight.cs
- Root.cs
- TabletCollection.cs
- TableHeaderCell.cs
- SimpleExpression.cs
- ObjectDataSourceEventArgs.cs
- DataBoundControl.cs
- DeleteWorkflowOwnerCommand.cs
- WebPartConnectionsCancelEventArgs.cs
- VariableAction.cs
- DbProviderFactory.cs
- Memoizer.cs
- AssociationSetEnd.cs
- Compiler.cs
- PeerUnsafeNativeMethods.cs
- XmlSchemaValidator.cs
- ChangePassword.cs
- BaseHashHelper.cs
- DiscreteKeyFrames.cs
- ExceptionCollection.cs
- MappedMetaModel.cs
- DispatcherObject.cs
- MdiWindowListStrip.cs
- FtpWebRequest.cs
- ZoomPercentageConverter.cs
- StateDesignerConnector.cs
- XmlSchemaIdentityConstraint.cs
- DesignerCategoryAttribute.cs
- AnonymousIdentificationModule.cs
- figurelengthconverter.cs
- _UncName.cs
- NamespaceEmitter.cs
- Int16AnimationBase.cs
- EdmItemError.cs
- DataRelationCollection.cs
- SQLByte.cs
- RSAPKCS1SignatureFormatter.cs
- NullRuntimeConfig.cs
- WSSecurityPolicy.cs
- WindowsStatusBar.cs
- PagePropertiesChangingEventArgs.cs
- DiagnosticTraceSource.cs
- OdbcEnvironmentHandle.cs
- PrivilegedConfigurationManager.cs
- TextEditorTyping.cs
- BaseParaClient.cs
- AutomationPropertyChangedEventArgs.cs
- RC2.cs
- GeometryModel3D.cs
- ListChangedEventArgs.cs
- TextDecorationCollection.cs
- _NegoState.cs
- ExpressionPrefixAttribute.cs
- CommonRemoteMemoryBlock.cs
- ToolStripStatusLabel.cs
- OneOfElement.cs
- UnsafeNativeMethods.cs
- TextAction.cs
- CodeGeneratorOptions.cs
- ComponentCommands.cs
- GridEntry.cs
- ToolStripLabel.cs
- ReferenceEqualityComparer.cs