Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / SapiInterop / SpeechEvent.cs / 1 / SpeechEvent.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Speech.AudioFormat; namespace System.Speech.Internal.SapiInterop { // Internal helper class that wraps a SAPI event structure. // A new instance is created by calling SpeechEvent.TryCreateSpeechEvent // Disposing this class will dispose all unmanmanaged memory. internal class SpeechEvent : IDisposable { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors private SpeechEvent(SPEVENTENUM eEventId, SPEVENTLPARAMTYPE elParamType, ulong ullAudioStreamOffset, IntPtr wParam, IntPtr lParam) { // We make a copy of the SPEVENTEX data but that's okay because the lParam will only be deleted once. _eventId = eEventId; _paramType = elParamType; _audioStreamOffset = ullAudioStreamOffset; _wParam = (ulong)wParam.ToInt64(); _lParam = (ulong)lParam; // Let the GC know if we have a unmanaged object with a given size if (_paramType == SPEVENTLPARAMTYPE.SPET_LPARAM_IS_POINTER || _paramType == SPEVENTLPARAMTYPE.SPET_LPARAM_IS_STRING) { GC.AddMemoryPressure(_sizeMemoryPressure = Marshal.SizeOf(_lParam)); } } private SpeechEvent(SPEVENT sapiEvent, SpeechAudioFormatInfo audioFormat) : this(sapiEvent.eEventId, sapiEvent.elParamType, sapiEvent.ullAudioStreamOffset, sapiEvent.wParam, sapiEvent.lParam) { if (audioFormat == null || audioFormat.EncodingFormat == 0) { _audioPosition = TimeSpan.Zero; } else { _audioPosition = audioFormat.AverageBytesPerSecond > 0 ? new TimeSpan ((long) ((sapiEvent.ullAudioStreamOffset * TimeSpan.TicksPerSecond) / (ulong) audioFormat.AverageBytesPerSecond)) : TimeSpan.Zero; } } private SpeechEvent(SPEVENTEX sapiEventEx) : this(sapiEventEx.eEventId, sapiEventEx.elParamType, sapiEventEx.ullAudioStreamOffset, sapiEventEx.wParam, sapiEventEx.lParam) { _audioPosition = new TimeSpan((long)sapiEventEx.ullAudioTimeOffset); } ~SpeechEvent() { Dispose (); } public void Dispose () { // General code to free event data if (_lParam != 0) { if (_paramType == SPEVENTLPARAMTYPE.SPET_LPARAM_IS_TOKEN || _paramType == SPEVENTLPARAMTYPE.SPET_LPARAM_IS_OBJECT) { Marshal.Release ((IntPtr) _lParam); } else { if (_paramType == SPEVENTLPARAMTYPE.SPET_LPARAM_IS_POINTER || _paramType == SPEVENTLPARAMTYPE.SPET_LPARAM_IS_STRING) { Marshal.FreeCoTaskMem ((IntPtr) _lParam); } } // Update the GC if (_sizeMemoryPressure > 0) { GC.RemoveMemoryPressure (_sizeMemoryPressure); _sizeMemoryPressure = 0; } // Mark the object as beeing freed _lParam = 0; } GC.SuppressFinalize (this); } #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region Internal Methods // This tries to get an event from the ISpEventSource. // If there are no events queued then null is returned. // Otherwise a new SpeechEvent is created and returned. static internal SpeechEvent TryCreateSpeechEvent(ISpEventSource sapiEventSource, bool additionalSapiFeatures, SpeechAudioFormatInfo audioFormat) { uint fetched; SpeechEvent speechEvent = null; if (additionalSapiFeatures) { SPEVENTEX sapiEventEx; ((ISpEventSource2)sapiEventSource).GetEventsEx(1, out sapiEventEx, out fetched); if (fetched == 1) { speechEvent = new SpeechEvent(sapiEventEx); } } else { SPEVENT sapiEvent; sapiEventSource.GetEvents(1, out sapiEvent, out fetched); if (fetched == 1) { speechEvent = new SpeechEvent(sapiEvent, audioFormat); } } return speechEvent; } #endregion //******************************************************************** // // Internal Properties // //******************************************************************** #region Internal Properties internal SPEVENTENUM EventId { get { return _eventId; } } internal ulong AudioStreamOffset { get { return _audioStreamOffset; } } // The WParam is returned as a 64-bit value since unmanaged wParam is always 32 or 64 depending on architecture. // This is always some kind of numeric value in SAPI - it is never a pointer that needs to freed. internal ulong WParam { get { return _wParam; } } internal ulong LParam { get { return _lParam; } } internal TimeSpan AudioPosition { get { return _audioPosition; } } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields private SPEVENTENUM _eventId; private SPEVENTLPARAMTYPE _paramType; private ulong _audioStreamOffset; private ulong _wParam; private ulong _lParam; private TimeSpan _audioPosition; private int _sizeMemoryPressure; #endregion } internal enum SPEVENTLPARAMTYPE : ushort { SPET_LPARAM_IS_UNDEFINED = 0x0000, SPET_LPARAM_IS_TOKEN = 0x0001, SPET_LPARAM_IS_OBJECT = 0x0002, SPET_LPARAM_IS_POINTER = 0x0003, SPET_LPARAM_IS_STRING = 0x0004 } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Speech.AudioFormat; namespace System.Speech.Internal.SapiInterop { // Internal helper class that wraps a SAPI event structure. // A new instance is created by calling SpeechEvent.TryCreateSpeechEvent // Disposing this class will dispose all unmanmanaged memory. internal class SpeechEvent : IDisposable { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors private SpeechEvent(SPEVENTENUM eEventId, SPEVENTLPARAMTYPE elParamType, ulong ullAudioStreamOffset, IntPtr wParam, IntPtr lParam) { // We make a copy of the SPEVENTEX data but that's okay because the lParam will only be deleted once. _eventId = eEventId; _paramType = elParamType; _audioStreamOffset = ullAudioStreamOffset; _wParam = (ulong)wParam.ToInt64(); _lParam = (ulong)lParam; // Let the GC know if we have a unmanaged object with a given size if (_paramType == SPEVENTLPARAMTYPE.SPET_LPARAM_IS_POINTER || _paramType == SPEVENTLPARAMTYPE.SPET_LPARAM_IS_STRING) { GC.AddMemoryPressure(_sizeMemoryPressure = Marshal.SizeOf(_lParam)); } } private SpeechEvent(SPEVENT sapiEvent, SpeechAudioFormatInfo audioFormat) : this(sapiEvent.eEventId, sapiEvent.elParamType, sapiEvent.ullAudioStreamOffset, sapiEvent.wParam, sapiEvent.lParam) { if (audioFormat == null || audioFormat.EncodingFormat == 0) { _audioPosition = TimeSpan.Zero; } else { _audioPosition = audioFormat.AverageBytesPerSecond > 0 ? new TimeSpan ((long) ((sapiEvent.ullAudioStreamOffset * TimeSpan.TicksPerSecond) / (ulong) audioFormat.AverageBytesPerSecond)) : TimeSpan.Zero; } } private SpeechEvent(SPEVENTEX sapiEventEx) : this(sapiEventEx.eEventId, sapiEventEx.elParamType, sapiEventEx.ullAudioStreamOffset, sapiEventEx.wParam, sapiEventEx.lParam) { _audioPosition = new TimeSpan((long)sapiEventEx.ullAudioTimeOffset); } ~SpeechEvent() { Dispose (); } public void Dispose () { // General code to free event data if (_lParam != 0) { if (_paramType == SPEVENTLPARAMTYPE.SPET_LPARAM_IS_TOKEN || _paramType == SPEVENTLPARAMTYPE.SPET_LPARAM_IS_OBJECT) { Marshal.Release ((IntPtr) _lParam); } else { if (_paramType == SPEVENTLPARAMTYPE.SPET_LPARAM_IS_POINTER || _paramType == SPEVENTLPARAMTYPE.SPET_LPARAM_IS_STRING) { Marshal.FreeCoTaskMem ((IntPtr) _lParam); } } // Update the GC if (_sizeMemoryPressure > 0) { GC.RemoveMemoryPressure (_sizeMemoryPressure); _sizeMemoryPressure = 0; } // Mark the object as beeing freed _lParam = 0; } GC.SuppressFinalize (this); } #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region Internal Methods // This tries to get an event from the ISpEventSource. // If there are no events queued then null is returned. // Otherwise a new SpeechEvent is created and returned. static internal SpeechEvent TryCreateSpeechEvent(ISpEventSource sapiEventSource, bool additionalSapiFeatures, SpeechAudioFormatInfo audioFormat) { uint fetched; SpeechEvent speechEvent = null; if (additionalSapiFeatures) { SPEVENTEX sapiEventEx; ((ISpEventSource2)sapiEventSource).GetEventsEx(1, out sapiEventEx, out fetched); if (fetched == 1) { speechEvent = new SpeechEvent(sapiEventEx); } } else { SPEVENT sapiEvent; sapiEventSource.GetEvents(1, out sapiEvent, out fetched); if (fetched == 1) { speechEvent = new SpeechEvent(sapiEvent, audioFormat); } } return speechEvent; } #endregion //******************************************************************** // // Internal Properties // //******************************************************************** #region Internal Properties internal SPEVENTENUM EventId { get { return _eventId; } } internal ulong AudioStreamOffset { get { return _audioStreamOffset; } } // The WParam is returned as a 64-bit value since unmanaged wParam is always 32 or 64 depending on architecture. // This is always some kind of numeric value in SAPI - it is never a pointer that needs to freed. internal ulong WParam { get { return _wParam; } } internal ulong LParam { get { return _lParam; } } internal TimeSpan AudioPosition { get { return _audioPosition; } } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields private SPEVENTENUM _eventId; private SPEVENTLPARAMTYPE _paramType; private ulong _audioStreamOffset; private ulong _wParam; private ulong _lParam; private TimeSpan _audioPosition; private int _sizeMemoryPressure; #endregion } internal enum SPEVENTLPARAMTYPE : ushort { SPET_LPARAM_IS_UNDEFINED = 0x0000, SPET_LPARAM_IS_TOKEN = 0x0001, SPET_LPARAM_IS_OBJECT = 0x0002, SPET_LPARAM_IS_POINTER = 0x0003, SPET_LPARAM_IS_STRING = 0x0004 } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
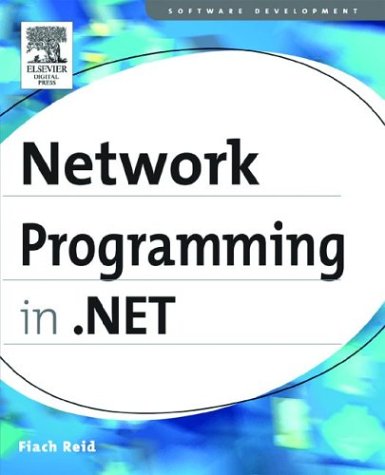
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OleDbDataAdapter.cs
- FeatureSupport.cs
- TraceHwndHost.cs
- documentsequencetextpointer.cs
- XNodeNavigator.cs
- TextParagraphView.cs
- CodeStatement.cs
- BindingMAnagerBase.cs
- SymLanguageVendor.cs
- RelationalExpressions.cs
- DataGridViewImageCell.cs
- RegexParser.cs
- ParenthesizePropertyNameAttribute.cs
- _ListenerResponseStream.cs
- GenericXmlSecurityToken.cs
- OdbcUtils.cs
- DelegatingConfigHost.cs
- ExitEventArgs.cs
- CompensationExtension.cs
- BitmapFrameEncode.cs
- CheckBoxAutomationPeer.cs
- InvokePattern.cs
- FunctionUpdateCommand.cs
- HMAC.cs
- EditorZoneBase.cs
- XPathConvert.cs
- MenuTracker.cs
- TogglePatternIdentifiers.cs
- WhitespaceRule.cs
- ExplicitDiscriminatorMap.cs
- Int16AnimationBase.cs
- HtmlControl.cs
- CustomCredentialPolicy.cs
- LogSwitch.cs
- ParameterBuilder.cs
- SHA512.cs
- ListControlConvertEventArgs.cs
- ConsumerConnectionPointCollection.cs
- TraceUtility.cs
- DataListItemEventArgs.cs
- _SSPIWrapper.cs
- CustomError.cs
- GraphicsPath.cs
- SoapTypeAttribute.cs
- XmlChildEnumerator.cs
- BrowserCapabilitiesFactoryBase.cs
- DataGridViewRowCancelEventArgs.cs
- StoreItemCollection.Loader.cs
- Rijndael.cs
- WebEncodingValidator.cs
- GuidelineSet.cs
- DynamicRouteExpression.cs
- UpdateProgress.cs
- JsonObjectDataContract.cs
- BaseDataList.cs
- CodeDomSerializationProvider.cs
- DataPagerField.cs
- AlphabeticalEnumConverter.cs
- TemplateManager.cs
- TreeWalkHelper.cs
- ActivitySurrogateSelector.cs
- MessageFormatterConverter.cs
- Boolean.cs
- PropertySegmentSerializationProvider.cs
- WasEndpointConfigContainer.cs
- ProtocolsSection.cs
- CollectionBuilder.cs
- ReliableSessionBindingElementImporter.cs
- Soap12ServerProtocol.cs
- WebPartMovingEventArgs.cs
- EntityDataSourceViewSchema.cs
- ToolTipAutomationPeer.cs
- DataGridViewTopRowAccessibleObject.cs
- WebPartCatalogAddVerb.cs
- _SSPISessionCache.cs
- WindowsIPAddress.cs
- PngBitmapEncoder.cs
- MultipleViewProviderWrapper.cs
- SystemIPv4InterfaceProperties.cs
- XmlIgnoreAttribute.cs
- CustomCategoryAttribute.cs
- DBDataPermissionAttribute.cs
- Variant.cs
- OptimizerPatterns.cs
- BinHexDecoder.cs
- DataBindingHandlerAttribute.cs
- SelectionPattern.cs
- ResourcesGenerator.cs
- ConfigPathUtility.cs
- CombinedTcpChannel.cs
- GlobalizationSection.cs
- TableCellCollection.cs
- RegularExpressionValidator.cs
- IOException.cs
- EntityDataSourceState.cs
- RoutedEventConverter.cs
- PictureBox.cs
- ZipIOBlockManager.cs
- RowBinding.cs
- WebPartsPersonalizationAuthorization.cs