Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Security / Policy / EvidenceBase.cs / 1305376 / EvidenceBase.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics.Contracts; using System.IO; using System.Runtime.InteropServices; #if FEATURE_SERIALIZATION using System.Runtime.Serialization.Formatters.Binary; #endif // FEATURE_SERIALIZATION using System.Security.Permissions; namespace System.Security.Policy { ////// Base class from which all objects to be used as Evidence must derive /// [ComVisible(true)] [Serializable] public abstract class EvidenceBase { protected EvidenceBase() { #if FEATURE_SERIALIZATION // All objects to be used as evidence must be serializable. Make sure that any derived types // are marked serializable to enforce this, since the attribute does not inherit down to derived // classes. if (!GetType().IsSerializable) { throw new InvalidOperationException(Environment.GetResourceString("Policy_EvidenceMustBeSerializable")); } #endif // FEATURE_SERIALIZATION } ////// Since legacy evidence objects would be cloned by being serialized, the default implementation /// of EvidenceBase will do the same. /// [SecurityPermission(SecurityAction.Assert, SerializationFormatter = true)] [SecuritySafeCritical] public virtual EvidenceBase Clone() { #if FEATURE_SERIALIZATION using (MemoryStream memoryStream = new MemoryStream()) { BinaryFormatter formatter = new BinaryFormatter(); formatter.Serialize(memoryStream, this); memoryStream.Position = 0; return formatter.Deserialize(memoryStream) as EvidenceBase; } #else // !FEATURE_SERIALIZATION throw new NotImplementedException(); #endif // FEATURE_SERIALIZATION } } ////// Interface for types which wrap Whidbey evidence objects for compatibility with v4 evidence rules /// internal interface ILegacyEvidenceAdapter { object EvidenceObject { get; } Type EvidenceType { get; } } ////// Wrapper class to hold legacy evidence objects which do not derive from EvidenceBase, and allow /// them to be held in the Evidence collection which expects to maintain lists of EvidenceBase only /// [Serializable] internal sealed class LegacyEvidenceWrapper : EvidenceBase, ILegacyEvidenceAdapter { private object m_legacyEvidence; internal LegacyEvidenceWrapper(object legacyEvidence) { Contract.Assert(legacyEvidence != null); Contract.Assert(legacyEvidence.GetType() != typeof(EvidenceBase), "Attempt to wrap an EvidenceBase in a LegacyEvidenceWrapper"); Contract.Assert(legacyEvidence.GetType().IsSerializable, "legacyEvidence.GetType().IsSerializable"); m_legacyEvidence = legacyEvidence; } public object EvidenceObject { get { return m_legacyEvidence; } } public Type EvidenceType { get { return m_legacyEvidence.GetType(); } } public override bool Equals(object obj) { return m_legacyEvidence.Equals(obj); } public override int GetHashCode() { return m_legacyEvidence.GetHashCode(); } } ////// Pre-v4 versions of the runtime allow multiple pieces of evidence that all have the same type. /// This type wraps those evidence objects into a single type of list, allowing legacy code to continue /// to work with the Evidence collection that does not expect multiple evidences of the same type. /// /// This may not be limited to LegacyEvidenceWrappers, since it's valid for legacy code to add multiple /// objects of built-in evidence to an Evidence collection. The built-in evidence now derives from /// EvienceObject, so when the legacy code runs on v4, it may end up attempting to add multiple /// Hash evidences for intsance. /// [Serializable] internal sealed class LegacyEvidenceList : EvidenceBase, IEnumerable, ILegacyEvidenceAdapter { private List m_legacyEvidenceList = new List (); public object EvidenceObject { get { // We'll choose the first item in the list to represent us if we're forced to return only // one object. This can occur if multiple pieces of evidence are added via the legacy APIs, // and then the new APIs are used to retrieve that evidence. return m_legacyEvidenceList.Count > 0 ? m_legacyEvidenceList[0] : null; } } public Type EvidenceType { get { Contract.Assert(m_legacyEvidenceList.Count > 0, "No items in LegacyEvidenceList, cannot tell what type they are"); ILegacyEvidenceAdapter adapter = m_legacyEvidenceList[0] as ILegacyEvidenceAdapter; return adapter == null ? m_legacyEvidenceList[0].GetType() : adapter.EvidenceType; } } public void Add(EvidenceBase evidence) { Contract.Assert(evidence != null); Contract.Assert(m_legacyEvidenceList.Count == 0 || EvidenceType == evidence.GetType() || (evidence is LegacyEvidenceWrapper && (evidence as LegacyEvidenceWrapper).EvidenceType == EvidenceType), "LegacyEvidenceList must be ----geonous"); Contract.Assert(evidence.GetType() != typeof(LegacyEvidenceList), "Attempt to add a legacy evidence list to another legacy evidence list"); m_legacyEvidenceList.Add(evidence); } public IEnumerator GetEnumerator() { return m_legacyEvidenceList.GetEnumerator(); } IEnumerator System.Collections.IEnumerable.GetEnumerator() { return m_legacyEvidenceList.GetEnumerator(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
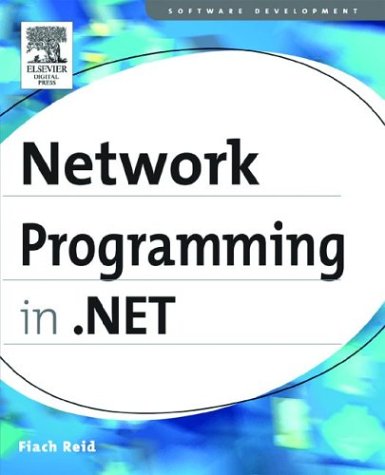
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DbExpressionVisitor_TResultType.cs
- Environment.cs
- MsmqChannelFactoryBase.cs
- CodeCastExpression.cs
- BookmarkCallbackWrapper.cs
- ToolStripHighContrastRenderer.cs
- FloaterParagraph.cs
- ToolStripItemImageRenderEventArgs.cs
- CultureSpecificStringDictionary.cs
- TagPrefixInfo.cs
- MasterPageCodeDomTreeGenerator.cs
- DbConnectionStringCommon.cs
- ResourcePool.cs
- ShellProvider.cs
- WorkflowTransactionService.cs
- InteropBitmapSource.cs
- DescendantBaseQuery.cs
- ASCIIEncoding.cs
- ExtensionDataObject.cs
- BamlRecordHelper.cs
- AttributeEmitter.cs
- TextCollapsingProperties.cs
- ScrollItemProviderWrapper.cs
- SplineQuaternionKeyFrame.cs
- ObjectStateFormatter.cs
- MatrixTransform3D.cs
- Operator.cs
- CorrelationService.cs
- ConnectorSelectionGlyph.cs
- SafeRegistryKey.cs
- AttributeUsageAttribute.cs
- PolicyImporterElement.cs
- ArrayWithOffset.cs
- SymbolMethod.cs
- TransactionState.cs
- NonParentingControl.cs
- HttpProfileGroupBase.cs
- SqlColumnizer.cs
- CqlWriter.cs
- SpeechSynthesizer.cs
- EmptyEnumerable.cs
- XmlDataSourceNodeDescriptor.cs
- CommandLibraryHelper.cs
- mansign.cs
- RegexCharClass.cs
- XmlReflectionImporter.cs
- _DisconnectOverlappedAsyncResult.cs
- BridgeDataRecord.cs
- ValidationEventArgs.cs
- PathNode.cs
- RuntimeCompatibilityAttribute.cs
- CallId.cs
- AutoCompleteStringCollection.cs
- RelationshipConverter.cs
- TextEditorMouse.cs
- _UncName.cs
- _ShellExpression.cs
- EmbossBitmapEffect.cs
- NodeLabelEditEvent.cs
- ServiceNameElementCollection.cs
- DataDocumentXPathNavigator.cs
- FormViewUpdatedEventArgs.cs
- EllipticalNodeOperations.cs
- CodeAttributeDeclaration.cs
- ObjectNavigationPropertyMapping.cs
- XmlSchemaValidator.cs
- BookmarkScopeHandle.cs
- HttpValueCollection.cs
- MappingException.cs
- FormatVersion.cs
- XmlWhitespace.cs
- Send.cs
- ScriptComponentDescriptor.cs
- DataGridViewCellValidatingEventArgs.cs
- InitializingNewItemEventArgs.cs
- DecimalAverageAggregationOperator.cs
- XslNumber.cs
- ScrollProviderWrapper.cs
- RegistrySecurity.cs
- ThaiBuddhistCalendar.cs
- SoapUnknownHeader.cs
- VirtualizedContainerService.cs
- SimpleHandlerBuildProvider.cs
- PersonalizationProvider.cs
- CheckBoxList.cs
- UnmanagedMemoryStream.cs
- Socket.cs
- TableItemProviderWrapper.cs
- LinqToSqlWrapper.cs
- ByteFacetDescriptionElement.cs
- ValueTypeFixupInfo.cs
- MemberCollection.cs
- BindingMAnagerBase.cs
- ObjectStorage.cs
- ToolCreatedEventArgs.cs
- XmlNotation.cs
- HashMembershipCondition.cs
- XPathParser.cs
- OuterGlowBitmapEffect.cs
- TranslateTransform.cs