Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / MS / Internal / Automation / ScrollProviderWrapper.cs / 1305600 / ScrollProviderWrapper.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Scroll pattern provider wrapper for WCP // // History: // 07/21/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Windows.Automation.Peers; using MS.Internal.Automation; namespace MS.Internal.Automation { // Automation/WCP Wrapper class: Implements that UIAutomation I...Provider // interface, and calls through to a WCP AutomationPeer which implements the corresponding // I...Provider inteface. Marshalls the call from the RPC thread onto the // target AutomationPeer's context. // // Class has two major parts to it: // * Implementation of the I...Provider, which uses Dispatcher.Invoke // to call a private method (lives in second half of the class) via a delegate, // if necessary, packages any params into an object param. Return type of Invoke // must be cast from object to appropriate type. // * private methods - one for each interface entry point - which get called back // on the right context. These call through to the peer that's actually // implenting the I...Provider version of the interface. internal class ScrollProviderWrapper: MarshalByRefObject, IScrollProvider { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private ScrollProviderWrapper( AutomationPeer peer, IScrollProvider iface ) { _peer = peer; _iface = iface; } #endregion Constructors //------------------------------------------------------ // // Interface IScrollProvider // //----------------------------------------------------- #region Interface IScrollProvider public void Scroll( ScrollAmount horizontalAmount, ScrollAmount verticalAmount ) { ElementUtil.Invoke( _peer, new DispatcherOperationCallback( Scroll ), new ScrollAmount [ ] { horizontalAmount, verticalAmount } ); } public void SetScrollPercent( double horizontalPercent, double verticalPercent ) { ElementUtil.Invoke( _peer, new DispatcherOperationCallback( SetScrollPercent ), new double [ ] { horizontalPercent, verticalPercent } ); } public double HorizontalScrollPercent { get { return (double) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetHorizontalScrollPercent ), null ); } } public double VerticalScrollPercent { get { return (double) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetVerticalScrollPercent ), null ); } } public double HorizontalViewSize { get { return (double) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetHorizontalViewSize ), null ); } } public double VerticalViewSize { get { return (double) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetVerticalViewSize ), null ); } } public bool HorizontallyScrollable { get { return (bool) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetHorizontallyScrollable ), null ); } } public bool VerticallyScrollable { get { return (bool) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetVerticallyScrollable ), null ); } } #endregion Interface IScrollProvider //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods internal static object Wrap( AutomationPeer peer, object iface ) { return new ScrollProviderWrapper( peer, (IScrollProvider) iface ); } #endregion Internal Methods //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods private object Scroll( object arg ) { ScrollAmount [ ] args = (ScrollAmount [ ]) arg; _iface.Scroll( args[ 0 ], args[ 1 ] ); return null; } private object SetScrollPercent( object arg ) { double [ ] args = (double [ ]) arg; _iface.SetScrollPercent( args[ 0 ], args[ 1 ] ); return null; } private object GetHorizontalScrollPercent( object unused ) { return _iface.HorizontalScrollPercent; } private object GetVerticalScrollPercent( object unused ) { return _iface.VerticalScrollPercent; } private object GetHorizontalViewSize( object unused ) { return _iface.HorizontalViewSize; } private object GetVerticalViewSize( object unused ) { return _iface.VerticalViewSize; } private object GetHorizontallyScrollable( object unused ) { return _iface.HorizontallyScrollable; } private object GetVerticallyScrollable( object unused ) { return _iface.VerticallyScrollable; } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationPeer _peer; private IScrollProvider _iface; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Scroll pattern provider wrapper for WCP // // History: // 07/21/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Windows.Automation.Peers; using MS.Internal.Automation; namespace MS.Internal.Automation { // Automation/WCP Wrapper class: Implements that UIAutomation I...Provider // interface, and calls through to a WCP AutomationPeer which implements the corresponding // I...Provider inteface. Marshalls the call from the RPC thread onto the // target AutomationPeer's context. // // Class has two major parts to it: // * Implementation of the I...Provider, which uses Dispatcher.Invoke // to call a private method (lives in second half of the class) via a delegate, // if necessary, packages any params into an object param. Return type of Invoke // must be cast from object to appropriate type. // * private methods - one for each interface entry point - which get called back // on the right context. These call through to the peer that's actually // implenting the I...Provider version of the interface. internal class ScrollProviderWrapper: MarshalByRefObject, IScrollProvider { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private ScrollProviderWrapper( AutomationPeer peer, IScrollProvider iface ) { _peer = peer; _iface = iface; } #endregion Constructors //------------------------------------------------------ // // Interface IScrollProvider // //----------------------------------------------------- #region Interface IScrollProvider public void Scroll( ScrollAmount horizontalAmount, ScrollAmount verticalAmount ) { ElementUtil.Invoke( _peer, new DispatcherOperationCallback( Scroll ), new ScrollAmount [ ] { horizontalAmount, verticalAmount } ); } public void SetScrollPercent( double horizontalPercent, double verticalPercent ) { ElementUtil.Invoke( _peer, new DispatcherOperationCallback( SetScrollPercent ), new double [ ] { horizontalPercent, verticalPercent } ); } public double HorizontalScrollPercent { get { return (double) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetHorizontalScrollPercent ), null ); } } public double VerticalScrollPercent { get { return (double) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetVerticalScrollPercent ), null ); } } public double HorizontalViewSize { get { return (double) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetHorizontalViewSize ), null ); } } public double VerticalViewSize { get { return (double) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetVerticalViewSize ), null ); } } public bool HorizontallyScrollable { get { return (bool) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetHorizontallyScrollable ), null ); } } public bool VerticallyScrollable { get { return (bool) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetVerticallyScrollable ), null ); } } #endregion Interface IScrollProvider //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods internal static object Wrap( AutomationPeer peer, object iface ) { return new ScrollProviderWrapper( peer, (IScrollProvider) iface ); } #endregion Internal Methods //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods private object Scroll( object arg ) { ScrollAmount [ ] args = (ScrollAmount [ ]) arg; _iface.Scroll( args[ 0 ], args[ 1 ] ); return null; } private object SetScrollPercent( object arg ) { double [ ] args = (double [ ]) arg; _iface.SetScrollPercent( args[ 0 ], args[ 1 ] ); return null; } private object GetHorizontalScrollPercent( object unused ) { return _iface.HorizontalScrollPercent; } private object GetVerticalScrollPercent( object unused ) { return _iface.VerticalScrollPercent; } private object GetHorizontalViewSize( object unused ) { return _iface.HorizontalViewSize; } private object GetVerticalViewSize( object unused ) { return _iface.VerticalViewSize; } private object GetHorizontallyScrollable( object unused ) { return _iface.HorizontallyScrollable; } private object GetVerticallyScrollable( object unused ) { return _iface.VerticallyScrollable; } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationPeer _peer; private IScrollProvider _iface; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
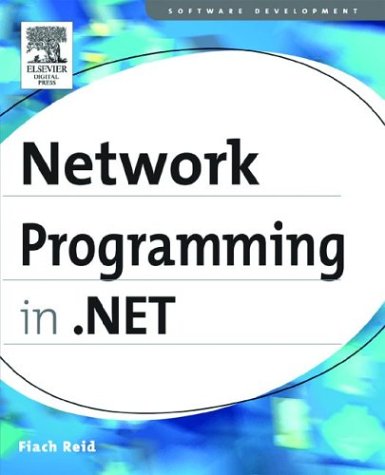
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TargetControlTypeCache.cs
- SpeechDetectedEventArgs.cs
- LinqDataSourceInsertEventArgs.cs
- ScriptManagerProxy.cs
- DbConnectionPoolGroupProviderInfo.cs
- DesignBinding.cs
- LineGeometry.cs
- EntitySqlQueryBuilder.cs
- Function.cs
- OleDbCommandBuilder.cs
- CrossSiteScriptingValidation.cs
- ToolStripEditorManager.cs
- SMSvcHost.cs
- SecurityPolicySection.cs
- EditorPartChrome.cs
- ToolboxComponentsCreatingEventArgs.cs
- DoWhileDesigner.xaml.cs
- DoubleCollectionConverter.cs
- BookmarkOptionsHelper.cs
- HighContrastHelper.cs
- OutputScope.cs
- SqlDependencyListener.cs
- EventSinkHelperWriter.cs
- NameSpaceExtractor.cs
- Listbox.cs
- X509UI.cs
- IncrementalHitTester.cs
- DomainUpDown.cs
- FixedSOMPageConstructor.cs
- ActionFrame.cs
- QilValidationVisitor.cs
- DelimitedListTraceListener.cs
- ComPlusSynchronizationContext.cs
- PropertyEntry.cs
- HtmlInputText.cs
- DEREncoding.cs
- SqlDataSourceSelectingEventArgs.cs
- transactioncontext.cs
- SQLChars.cs
- TextEditorSelection.cs
- StatusBarItem.cs
- designeractionbehavior.cs
- WebPartVerbCollection.cs
- sqlser.cs
- TextTreeInsertElementUndoUnit.cs
- CompiledRegexRunner.cs
- AutomationElement.cs
- SafeEventLogReadHandle.cs
- TextBoxAutomationPeer.cs
- ProfileSection.cs
- CookielessHelper.cs
- WebPartTransformer.cs
- CriticalExceptions.cs
- FilePrompt.cs
- RowUpdatingEventArgs.cs
- SafeRegistryHandle.cs
- RayMeshGeometry3DHitTestResult.cs
- EnumBuilder.cs
- SQLDoubleStorage.cs
- FormViewPagerRow.cs
- LinqDataSourceContextEventArgs.cs
- ObjectSet.cs
- OrderedHashRepartitionStream.cs
- CatalogPart.cs
- GetPageNumberCompletedEventArgs.cs
- EmptyCollection.cs
- TraceEventCache.cs
- EndpointDiscoveryElement.cs
- listitem.cs
- DetailsViewModeEventArgs.cs
- SoapObjectReader.cs
- UserNameSecurityToken.cs
- SimpleExpression.cs
- ConnectionDemuxer.cs
- ServerIdentity.cs
- DialogResultConverter.cs
- DynamicDiscoveryDocument.cs
- XmlSchemaDatatype.cs
- CharStorage.cs
- CodeDirectionExpression.cs
- LiteralControl.cs
- MetadataArtifactLoaderResource.cs
- PathSegment.cs
- OAVariantLib.cs
- TrustLevel.cs
- IntSecurity.cs
- BaseParagraph.cs
- FloatAverageAggregationOperator.cs
- BufferedWebEventProvider.cs
- DictionaryTraceRecord.cs
- SqlMethodCallConverter.cs
- Encoding.cs
- WindowsFormsSynchronizationContext.cs
- TreeView.cs
- SinglePhaseEnlistment.cs
- PriorityBindingExpression.cs
- RequiredFieldValidator.cs
- DuplicateWaitObjectException.cs
- CmsUtils.cs
- SharedConnectionInfo.cs