Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / XPathBinder.cs / 1305376 / XPathBinder.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Globalization; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Data; using System.Xml; using System.Xml.XPath; ////// public sealed class XPathBinder { ///private XPathBinder() { } /// /// public static object Eval(object container, string xPath) { IXmlNamespaceResolver resolver = null; return Eval(container, xPath, resolver); } public static object Eval(object container, string xPath, IXmlNamespaceResolver resolver) { if (container == null) { throw new ArgumentNullException("container"); } if (String.IsNullOrEmpty(xPath)) { throw new ArgumentNullException("xPath"); } IXPathNavigable node = container as IXPathNavigable; if (node == null) { throw new ArgumentException(SR.GetString(SR.XPathBinder_MustBeIXPathNavigable, container.GetType().FullName)); } XPathNavigator navigator = node.CreateNavigator(); object retValue = navigator.Evaluate(xPath, resolver); // If we get back an XPathNodeIterator instead of a simple object, advance // the iterator to the first node and return the value. XPathNodeIterator iterator = retValue as XPathNodeIterator; if (iterator != null) { if (iterator.MoveNext()) { retValue = iterator.Current.Value; } else { retValue = null; } } return retValue; } ////// public static string Eval(object container, string xPath, string format) { return Eval(container, xPath, format, null); } public static string Eval(object container, string xPath, string format, IXmlNamespaceResolver resolver) { object value = XPathBinder.Eval(container, xPath, resolver); if (value == null) { return String.Empty; } else { if (String.IsNullOrEmpty(format)) { return value.ToString(); } else { return String.Format(format, value); } } } ////// Evaluates an XPath query with respect to a context IXPathNavigable object that returns a NodeSet. /// public static IEnumerable Select(object container, string xPath) { return Select(container, xPath, null); } public static IEnumerable Select(object container, string xPath, IXmlNamespaceResolver resolver) { if (container == null) { throw new ArgumentNullException("container"); } if (String.IsNullOrEmpty(xPath)) { throw new ArgumentNullException("xPath"); } ArrayList results = new ArrayList(); IXPathNavigable node = container as IXPathNavigable; if (node == null) { throw new ArgumentException(SR.GetString(SR.XPathBinder_MustBeIXPathNavigable, container.GetType().FullName)); } XPathNavigator navigator = node.CreateNavigator(); XPathNodeIterator iterator = navigator.Select(xPath, resolver); while (iterator.MoveNext()) { IHasXmlNode hasXmlNode = iterator.Current as IHasXmlNode; if (hasXmlNode == null) { throw new InvalidOperationException(SR.GetString(SR.XPathBinder_MustHaveXmlNodes)); } results.Add(hasXmlNode.GetNode()); } return results; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Globalization; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Data; using System.Xml; using System.Xml.XPath; ////// public sealed class XPathBinder { ///private XPathBinder() { } /// /// public static object Eval(object container, string xPath) { IXmlNamespaceResolver resolver = null; return Eval(container, xPath, resolver); } public static object Eval(object container, string xPath, IXmlNamespaceResolver resolver) { if (container == null) { throw new ArgumentNullException("container"); } if (String.IsNullOrEmpty(xPath)) { throw new ArgumentNullException("xPath"); } IXPathNavigable node = container as IXPathNavigable; if (node == null) { throw new ArgumentException(SR.GetString(SR.XPathBinder_MustBeIXPathNavigable, container.GetType().FullName)); } XPathNavigator navigator = node.CreateNavigator(); object retValue = navigator.Evaluate(xPath, resolver); // If we get back an XPathNodeIterator instead of a simple object, advance // the iterator to the first node and return the value. XPathNodeIterator iterator = retValue as XPathNodeIterator; if (iterator != null) { if (iterator.MoveNext()) { retValue = iterator.Current.Value; } else { retValue = null; } } return retValue; } ////// public static string Eval(object container, string xPath, string format) { return Eval(container, xPath, format, null); } public static string Eval(object container, string xPath, string format, IXmlNamespaceResolver resolver) { object value = XPathBinder.Eval(container, xPath, resolver); if (value == null) { return String.Empty; } else { if (String.IsNullOrEmpty(format)) { return value.ToString(); } else { return String.Format(format, value); } } } ////// Evaluates an XPath query with respect to a context IXPathNavigable object that returns a NodeSet. /// public static IEnumerable Select(object container, string xPath) { return Select(container, xPath, null); } public static IEnumerable Select(object container, string xPath, IXmlNamespaceResolver resolver) { if (container == null) { throw new ArgumentNullException("container"); } if (String.IsNullOrEmpty(xPath)) { throw new ArgumentNullException("xPath"); } ArrayList results = new ArrayList(); IXPathNavigable node = container as IXPathNavigable; if (node == null) { throw new ArgumentException(SR.GetString(SR.XPathBinder_MustBeIXPathNavigable, container.GetType().FullName)); } XPathNavigator navigator = node.CreateNavigator(); XPathNodeIterator iterator = navigator.Select(xPath, resolver); while (iterator.MoveNext()) { IHasXmlNode hasXmlNode = iterator.Current as IHasXmlNode; if (hasXmlNode == null) { throw new InvalidOperationException(SR.GetString(SR.XPathBinder_MustHaveXmlNodes)); } results.Add(hasXmlNode.GetNode()); } return results; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
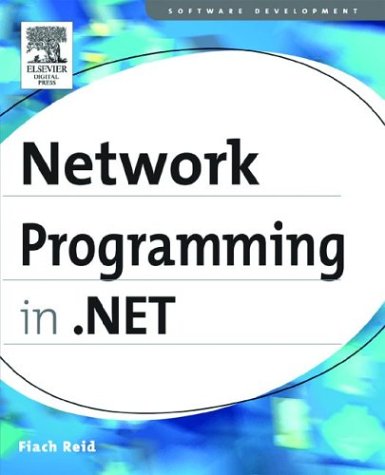
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FastEncoderWindow.cs
- ReaderOutput.cs
- ToolStripContainerDesigner.cs
- FixedPageAutomationPeer.cs
- XsltQilFactory.cs
- BitmapEffectDrawing.cs
- AccessText.cs
- Odbc32.cs
- BitmapDownload.cs
- Completion.cs
- PrinterUnitConvert.cs
- TagMapInfo.cs
- TimeoutHelper.cs
- SafeIUnknown.cs
- Vector.cs
- Sql8ConformanceChecker.cs
- VisualStates.cs
- designeractionlistschangedeventargs.cs
- KnownBoxes.cs
- TimeStampChecker.cs
- LinearKeyFrames.cs
- CachedTypeface.cs
- ElementProxy.cs
- Column.cs
- SchemaCollectionCompiler.cs
- ProvidersHelper.cs
- Size3DValueSerializer.cs
- BinaryCommonClasses.cs
- SelectingProviderEventArgs.cs
- NullRuntimeConfig.cs
- ProfileModule.cs
- SqlGenericUtil.cs
- DocComment.cs
- DataColumnMappingCollection.cs
- ToolStripPanelSelectionGlyph.cs
- GenericXmlSecurityToken.cs
- ChannelOptions.cs
- ArraySubsetEnumerator.cs
- CatalogPart.cs
- HandlerWithFactory.cs
- StateInitialization.cs
- BehaviorEditorPart.cs
- TypeDefinition.cs
- ToolStripPanelRenderEventArgs.cs
- Geometry.cs
- Decorator.cs
- _FtpDataStream.cs
- DependencyPropertyAttribute.cs
- HandlerMappingMemo.cs
- VBCodeProvider.cs
- _IPv4Address.cs
- JavaScriptString.cs
- JournalNavigationScope.cs
- MarginsConverter.cs
- ProfileParameter.cs
- StandardToolWindows.cs
- FormParameter.cs
- DataGridViewCellValidatingEventArgs.cs
- TransformConverter.cs
- GridViewColumn.cs
- FormViewPagerRow.cs
- LoginUtil.cs
- ColorConverter.cs
- Simplifier.cs
- InputLanguageEventArgs.cs
- InvalidWMPVersionException.cs
- BinaryMessageEncoder.cs
- ConstraintConverter.cs
- HelpKeywordAttribute.cs
- StreamWithDictionary.cs
- SeekStoryboard.cs
- DecoratedNameAttribute.cs
- Panel.cs
- DispatcherHookEventArgs.cs
- Automation.cs
- QilUnary.cs
- TextTreeUndoUnit.cs
- MeshGeometry3D.cs
- XmlTextWriter.cs
- ImageConverter.cs
- GrammarBuilderWildcard.cs
- BufferAllocator.cs
- AuthorizationRule.cs
- SessionEndedEventArgs.cs
- UIntPtr.cs
- DataGridViewTopRowAccessibleObject.cs
- Code.cs
- IdentitySection.cs
- PeerApplicationLaunchInfo.cs
- Rect.cs
- SynchronizedDispatch.cs
- WhileDesigner.cs
- GetWinFXPath.cs
- ReadOnlyObservableCollection.cs
- WaitHandleCannotBeOpenedException.cs
- SubstitutionList.cs
- StreamingContext.cs
- RotateTransform3D.cs
- ELinqQueryState.cs
- PerformanceCounter.cs