Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / InputOutput / Completion.cs / 1 / Completion.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // This file implements completion-related messaging using System; using System.Diagnostics; using System.IdentityModel.Claims; using System.IdentityModel.Policy; using System.ServiceModel; using System.ServiceModel.Channels; using System.Xml; using Microsoft.Transactions.Wsat.InputOutput; using Microsoft.Transactions.Wsat.Messaging; using Microsoft.Transactions.Wsat.Protocol; using Microsoft.Transactions.Wsat.StateMachines; using DiagnosticUtility = Microsoft.Transactions.Bridge.DiagnosticUtility; using Fault = Microsoft.Transactions.Wsat.Messaging.Fault; namespace Microsoft.Transactions.Wsat.InputOutput { class CompletionCoordinator : ICompletionCoordinator { ProtocolState state; AsyncCallback sendComplete; AsyncCallback politeSendComplete; public CompletionCoordinator(ProtocolState state) { this.state = state; this.sendComplete = DiagnosticUtility.ThunkAsyncCallback(new AsyncCallback(SendComplete)); this.politeSendComplete = DiagnosticUtility.ThunkAsyncCallback(new AsyncCallback(PoliteSendComplete)); } // // ICompletionCoordinator // CompletionEnlistment CheckMessage(Message message, bool reply) { Guid enlistmentId; if (!Ports.TryGetEnlistment(message, out enlistmentId)) { DebugTrace.Trace(TraceLevel.Warning, "Could not read enlistment header from message"); if (reply) this.SendFault(message, this.state.Faults.InvalidParameters); return null; } TransactionEnlistment enlistment = state.Lookup.FindEnlistment(enlistmentId); if (enlistment == null) { DebugTrace.Trace(TraceLevel.Warning, "Could not find enlistment {0}", enlistmentId); if (reply) this.SendFault(message, this.state.Faults.InvalidState); return null; } CompletionEnlistment completion = enlistment as CompletionEnlistment; if (completion == null) { DebugTrace.Trace(TraceLevel.Warning, "Completion message received for non-completion enlistment {0}", enlistmentId); if (reply) this.SendFault(message, this.state.Faults.InvalidParameters); return null; } if (!state.Service.Security.CheckIdentity(completion.ParticipantProxy, message)) { if (EnlistmentIdentityCheckFailedRecord.ShouldTrace) { EnlistmentIdentityCheckFailedRecord.Trace(completion.EnlistmentId); } // no fault reply is sent in order to replicate the security // infrastructure behavior - see MB55336 return null; } return completion; } public void Commit(Message message) { CompletionEnlistment completion = CheckMessage(message, true); if (completion != null) { completion.StateMachine.Enqueue(new MsgCompletionCommitEvent(completion)); } } public void Rollback(Message message) { CompletionEnlistment completion = CheckMessage(message, true); if (completion != null) { completion.StateMachine.Enqueue(new MsgCompletionRollbackEvent(completion)); } } public void Fault(Message message, MessageFault fault) { CompletionEnlistment completion = CheckMessage(message, false); if (completion != null) { state.Perf.FaultsReceivedCountPerInterval.Increment(); } if (DebugTrace.Info) { DebugTrace.Trace(TraceLevel.Info, "Ignoring {0} fault from completion participant at {1}: {2}", Library.GetFaultCodeName(fault), Ports.TryGetFromAddress(message), Library.GetFaultCodeReason(fault)); } } // // Sending messages // void SendComplete(IAsyncResult ar) { if (!ar.CompletedSynchronously) { CompletionEnlistment completion = (CompletionEnlistment) ar.AsyncState; OnSendComplete(ar, completion, completion.ParticipantProxy); } } void PoliteSendComplete(IAsyncResult ar) { if (!ar.CompletedSynchronously) { CompletionParticipantProxy proxy = (CompletionParticipantProxy) ar.AsyncState; OnSendComplete(ar, null, proxy); } } void OnSendComplete(IAsyncResult ar, CompletionEnlistment completion, CompletionParticipantProxy proxy) { try { proxy.EndSendMessage(ar); } catch(WsatSendFailureException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); state.Perf.MessageSendFailureCountPerInterval.Increment(); if (completion != null) { // Don't bother to enqueue a send failure. The completion state machine doesn't care DebugTrace.TraceSendFailure(completion.EnlistmentId, e); } else { DebugTrace.TraceSendFailure(e); } } } // // Messages // public void SendCommitted(CompletionEnlistment completion) { if (DebugTrace.Info) { DebugTrace.TxTrace( TraceLevel.Info, completion.EnlistmentId, "Sending Committed to completion participant at {0}", Ports.TryGetAddress(completion.ParticipantProxy)); } IAsyncResult ar = completion.ParticipantProxy.BeginSendCommitted(this.sendComplete, completion); if (ar.CompletedSynchronously) { OnSendComplete(ar, completion, completion.ParticipantProxy); } } public void SendCommitted(EndpointAddress sendTo) { if (sendTo != null) { CompletionParticipantProxy proxy = state.TryCreateCompletionParticipantProxy(sendTo); if (proxy != null) { try { if (DebugTrace.Info) { DebugTrace.Trace(TraceLevel.Info, "Sending Committed to unrecognized completion participant at {0}", Ports.TryGetAddress(proxy)); } IAsyncResult ar = proxy.BeginSendCommitted(this.politeSendComplete, proxy); if (ar.CompletedSynchronously) { OnSendComplete(ar, null, proxy); } } finally { proxy.Release(); } } } } public void SendAborted(CompletionEnlistment completion) { if (DebugTrace.Info) { DebugTrace.TxTrace( TraceLevel.Info, completion.EnlistmentId, "Sending Aborted to completion participant at {0}", Ports.TryGetAddress(completion.ParticipantProxy)); } IAsyncResult ar = completion.ParticipantProxy.BeginSendAborted(this.sendComplete, completion); if (ar.CompletedSynchronously) { OnSendComplete(ar, completion, completion.ParticipantProxy); } } public void SendAborted(EndpointAddress sendTo) { if (sendTo != null) { CompletionParticipantProxy proxy = state.TryCreateCompletionParticipantProxy(sendTo); if (proxy != null) { try { if (DebugTrace.Info) { DebugTrace.Trace(TraceLevel.Info, "Sending Aborted to unrecognized completion participant at {0}", Ports.TryGetAddress(proxy)); } IAsyncResult ar = proxy.BeginSendAborted(this.politeSendComplete, proxy); if (ar.CompletedSynchronously) { OnSendComplete(ar, null, proxy); } } finally { proxy.Release(); } } } } void SendFault(Message message, Fault fault) { SendFault(Library.GetFaultToHeader(message.Headers, this.state.ProtocolVersion), message.Headers.MessageId, fault); } public void SendFault(EndpointAddress faultTo, UniqueId messageID, Fault fault) { if (faultTo != null) { state.FaultSender.TrySendCompletionParticipantFault(faultTo, messageID, fault); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
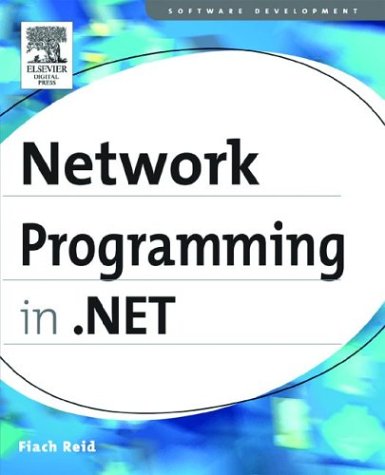
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Activator.cs
- XmlSerializerSection.cs
- SqlUnionizer.cs
- CaseInsensitiveHashCodeProvider.cs
- input.cs
- EventBookmark.cs
- DataListCommandEventArgs.cs
- SmiRequestExecutor.cs
- ScalarType.cs
- DropDownList.cs
- HtmlInputSubmit.cs
- Activity.cs
- UnionExpr.cs
- EmptyEnumerable.cs
- X509CertificateCollection.cs
- PkcsMisc.cs
- altserialization.cs
- RuntimeCompatibilityAttribute.cs
- RichTextBox.cs
- BridgeDataReader.cs
- GB18030Encoding.cs
- ArcSegment.cs
- ThicknessAnimationUsingKeyFrames.cs
- AdCreatedEventArgs.cs
- DialogResultConverter.cs
- CaseCqlBlock.cs
- FormViewUpdatedEventArgs.cs
- DesignBindingEditor.cs
- EmptyArray.cs
- WebPartDisplayMode.cs
- ToolStripSeparator.cs
- InputScope.cs
- QueryCreatedEventArgs.cs
- Application.cs
- FilePrompt.cs
- mediaclock.cs
- ObjectDataSourceMethodEventArgs.cs
- VirtualizedItemPattern.cs
- BamlRecords.cs
- HtmlInputButton.cs
- TableRow.cs
- FunctionNode.cs
- WpfKnownType.cs
- CursorConverter.cs
- TreeNodeBindingCollection.cs
- ProxyManager.cs
- TaskFormBase.cs
- SerializationEventsCache.cs
- SplayTreeNode.cs
- FigureHelper.cs
- LogicalExpr.cs
- NativeWrapper.cs
- EndOfStreamException.cs
- CodeGenerationManager.cs
- XPathAncestorIterator.cs
- NativeMethods.cs
- Enum.cs
- ResourcesGenerator.cs
- DatatypeImplementation.cs
- FormConverter.cs
- FixedBufferAttribute.cs
- CodeTypeMember.cs
- WinInet.cs
- counter.cs
- CellParaClient.cs
- FontNameConverter.cs
- SchemaCollectionPreprocessor.cs
- EntityDataSourceQueryBuilder.cs
- BodyGlyph.cs
- HtmlGenericControl.cs
- XmlEntityReference.cs
- Run.cs
- ComboBoxItem.cs
- GeneralTransform3D.cs
- ServiceEndpointElement.cs
- SafeReversePInvokeHandle.cs
- XmlSchema.cs
- FontCollection.cs
- GeometryGroup.cs
- ADMembershipProvider.cs
- HttpRequest.cs
- EngineSiteSapi.cs
- ChtmlTextWriter.cs
- BitmapEffectDrawing.cs
- CollectionViewProxy.cs
- BitmapCacheBrush.cs
- DesignTimeVisibleAttribute.cs
- EdmMember.cs
- ProfilePropertySettingsCollection.cs
- TaiwanLunisolarCalendar.cs
- BasicExpressionVisitor.cs
- ChildrenQuery.cs
- UITypeEditor.cs
- SqlUtil.cs
- XPathAncestorQuery.cs
- CalculatedColumn.cs
- ExpiredSecurityTokenException.cs
- altserialization.cs
- PackageRelationshipCollection.cs
- FlowPosition.cs