Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / UIAutomation / UIAutomationClient / MS / Internal / Automation / input.cs / 1 / input.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Provides mouse and keyboard input functionality // // History: // 05/29/2003 : BrendanM ported to WCP // //--------------------------------------------------------------------------- using System.Windows.Input; using System.ComponentModel; using System.Runtime.InteropServices; using MS.Win32; using System; namespace MS.Internal.Automation { // // Provides internal methods for sending keyboard input // internal sealed class Input { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // Static class - Private to prevent creation Input() { } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods // // Inject keyboard input into the system // internal static void SendKeyboardInput( Key key, bool press ) { UnsafeNativeMethods.INPUT ki = new UnsafeNativeMethods.INPUT(); ki.type = UnsafeNativeMethods.INPUT_KEYBOARD; ki.union.keyboardInput.wVk = (short) KeyInterop.VirtualKeyFromKey( key ); ki.union.keyboardInput.wScan = (short) UnsafeNativeMethods.MapVirtualKey( ki.union.keyboardInput.wVk, 0 ); int dwFlags = 0; if( ki.union.keyboardInput.wScan > 0 ) dwFlags |= UnsafeNativeMethods.KEYEVENTF_SCANCODE; if( !press ) dwFlags |= UnsafeNativeMethods.KEYEVENTF_KEYUP; ki.union.keyboardInput.dwFlags = dwFlags; if( IsExtendedKey( key ) ) { ki.union.keyboardInput.dwFlags |= UnsafeNativeMethods.KEYEVENTF_EXTENDEDKEY; } ki.union.keyboardInput.time = 0; ki.union.keyboardInput.dwExtraInfo = new IntPtr( 0 ); Misc.SendInput(1, ref ki, Marshal.SizeOf(ki)); } // Used internally by the HWND SetFocus code - it sends a hotkey to // itself - because it uses a VK that's not on the keyboard, it needs // to send the VK directly, not the scan code, which regular // SendKeyboardInput does. internal static void SendKeyboardInputVK( byte vk, bool press ) { UnsafeNativeMethods.INPUT ki = new UnsafeNativeMethods.INPUT(); ki.type = UnsafeNativeMethods.INPUT_KEYBOARD; ki.union.keyboardInput.wVk = vk; ki.union.keyboardInput.wScan = 0; ki.union.keyboardInput.dwFlags = press ? 0 : UnsafeNativeMethods.KEYEVENTF_KEYUP; ki.union.keyboardInput.time = 0; ki.union.keyboardInput.dwExtraInfo = new IntPtr( 0 ); Misc.SendInput(1, ref ki, Marshal.SizeOf(ki)); } #endregion Internal Methods //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ #region Private Methods private static bool IsExtendedKey( Key key ) { // From the SDK: // The extended-key flag indicates whether the keystroke message originated from one of // the additional keys on the enhanced keyboard. The extended keys consist of the ALT and // CTRL keys on the right-hand side of the keyboard; the INS, DEL, HOME, END, PAGE UP, // PAGE DOWN, and arrow keys in the clusters to the left of the numeric keypad; the NUM LOCK // key; the BREAK (CTRL+PAUSE) key; the PRINT SCRN key; and the divide (/) and ENTER keys in // the numeric keypad. The extended-key flag is set if the key is an extended key. // // - docs appear to be incorrect. Use of Spy++ indicates that break is not an extended key. // Also, menu key and windows keys also appear to be extended. return key == Key.RightAlt || key == Key.RightCtrl || key == Key.NumLock || key == Key.Insert || key == Key.Delete || key == Key.Home || key == Key.End || key == Key.Prior || key == Key.Next || key == Key.Up || key == Key.Down || key == Key.Left || key == Key.Right || key == Key.Apps || key == Key.RWin || key == Key.LWin; // Note that there are no distinct values for the following keys: // numpad divide // numpad enter } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields // Stateless object, has no private fields #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Provides mouse and keyboard input functionality // // History: // 05/29/2003 : BrendanM ported to WCP // //--------------------------------------------------------------------------- using System.Windows.Input; using System.ComponentModel; using System.Runtime.InteropServices; using MS.Win32; using System; namespace MS.Internal.Automation { // // Provides internal methods for sending keyboard input // internal sealed class Input { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // Static class - Private to prevent creation Input() { } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods // // Inject keyboard input into the system // internal static void SendKeyboardInput( Key key, bool press ) { UnsafeNativeMethods.INPUT ki = new UnsafeNativeMethods.INPUT(); ki.type = UnsafeNativeMethods.INPUT_KEYBOARD; ki.union.keyboardInput.wVk = (short) KeyInterop.VirtualKeyFromKey( key ); ki.union.keyboardInput.wScan = (short) UnsafeNativeMethods.MapVirtualKey( ki.union.keyboardInput.wVk, 0 ); int dwFlags = 0; if( ki.union.keyboardInput.wScan > 0 ) dwFlags |= UnsafeNativeMethods.KEYEVENTF_SCANCODE; if( !press ) dwFlags |= UnsafeNativeMethods.KEYEVENTF_KEYUP; ki.union.keyboardInput.dwFlags = dwFlags; if( IsExtendedKey( key ) ) { ki.union.keyboardInput.dwFlags |= UnsafeNativeMethods.KEYEVENTF_EXTENDEDKEY; } ki.union.keyboardInput.time = 0; ki.union.keyboardInput.dwExtraInfo = new IntPtr( 0 ); Misc.SendInput(1, ref ki, Marshal.SizeOf(ki)); } // Used internally by the HWND SetFocus code - it sends a hotkey to // itself - because it uses a VK that's not on the keyboard, it needs // to send the VK directly, not the scan code, which regular // SendKeyboardInput does. internal static void SendKeyboardInputVK( byte vk, bool press ) { UnsafeNativeMethods.INPUT ki = new UnsafeNativeMethods.INPUT(); ki.type = UnsafeNativeMethods.INPUT_KEYBOARD; ki.union.keyboardInput.wVk = vk; ki.union.keyboardInput.wScan = 0; ki.union.keyboardInput.dwFlags = press ? 0 : UnsafeNativeMethods.KEYEVENTF_KEYUP; ki.union.keyboardInput.time = 0; ki.union.keyboardInput.dwExtraInfo = new IntPtr( 0 ); Misc.SendInput(1, ref ki, Marshal.SizeOf(ki)); } #endregion Internal Methods //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ #region Private Methods private static bool IsExtendedKey( Key key ) { // From the SDK: // The extended-key flag indicates whether the keystroke message originated from one of // the additional keys on the enhanced keyboard. The extended keys consist of the ALT and // CTRL keys on the right-hand side of the keyboard; the INS, DEL, HOME, END, PAGE UP, // PAGE DOWN, and arrow keys in the clusters to the left of the numeric keypad; the NUM LOCK // key; the BREAK (CTRL+PAUSE) key; the PRINT SCRN key; and the divide (/) and ENTER keys in // the numeric keypad. The extended-key flag is set if the key is an extended key. // // - docs appear to be incorrect. Use of Spy++ indicates that break is not an extended key. // Also, menu key and windows keys also appear to be extended. return key == Key.RightAlt || key == Key.RightCtrl || key == Key.NumLock || key == Key.Insert || key == Key.Delete || key == Key.Home || key == Key.End || key == Key.Prior || key == Key.Next || key == Key.Up || key == Key.Down || key == Key.Left || key == Key.Right || key == Key.Apps || key == Key.RWin || key == Key.LWin; // Note that there are no distinct values for the following keys: // numpad divide // numpad enter } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields // Stateless object, has no private fields #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
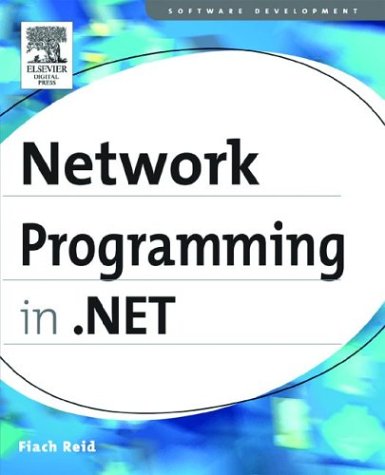
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CoTaskMemUnicodeSafeHandle.cs
- Assert.cs
- ObjectDataSourceSelectingEventArgs.cs
- TableCell.cs
- GlobalItem.cs
- ApplicationServiceHelper.cs
- XmlDataImplementation.cs
- DataGridViewComponentPropertyGridSite.cs
- EntityParameter.cs
- Parameter.cs
- SizeConverter.cs
- WindowsFormsSynchronizationContext.cs
- OperationFormatStyle.cs
- CompareValidator.cs
- OpenFileDialog.cs
- ColorTransform.cs
- ContentFilePart.cs
- ContentType.cs
- HebrewNumber.cs
- EventMappingSettingsCollection.cs
- XmlElementAttributes.cs
- PowerStatus.cs
- Baml2006SchemaContext.cs
- ContextMenu.cs
- EncodingDataItem.cs
- FormViewInsertedEventArgs.cs
- DataSourceControlBuilder.cs
- GroupBox.cs
- DataTableReaderListener.cs
- TypeProvider.cs
- DefaultValueMapping.cs
- EnumMember.cs
- ProcessHost.cs
- SQLMembershipProvider.cs
- HwndTarget.cs
- ScrollProviderWrapper.cs
- ScriptModule.cs
- DependencyPropertyDescriptor.cs
- RelationshipType.cs
- MonthCalendar.cs
- CollectionViewSource.cs
- ResourceReferenceExpressionConverter.cs
- ComboBox.cs
- UnmanagedBitmapWrapper.cs
- UiaCoreApi.cs
- MouseActionConverter.cs
- TextProperties.cs
- TextTreeDeleteContentUndoUnit.cs
- CodeCatchClause.cs
- ProfessionalColors.cs
- FormViewCommandEventArgs.cs
- BorderGapMaskConverter.cs
- BoundingRectTracker.cs
- ChildTable.cs
- SuppressMergeCheckAttribute.cs
- XmlAttributes.cs
- DependencyObjectType.cs
- SwitchAttribute.cs
- SiblingIterators.cs
- DataServiceCollectionOfT.cs
- XamlFilter.cs
- DesignerTransaction.cs
- TemplateParser.cs
- PersonalizationStateInfo.cs
- ToolStripDropDownClosingEventArgs.cs
- AxisAngleRotation3D.cs
- SiteMapProvider.cs
- RefExpr.cs
- DataGridViewRowErrorTextNeededEventArgs.cs
- ComponentCollection.cs
- InternalsVisibleToAttribute.cs
- DeviceContext2.cs
- TypeUnloadedException.cs
- Double.cs
- ResizeGrip.cs
- LogicalMethodInfo.cs
- ColorMatrix.cs
- CryptoApi.cs
- DependencyObjectPropertyDescriptor.cs
- Compiler.cs
- RecognizedAudio.cs
- OleDbParameter.cs
- SoapExtensionStream.cs
- MatrixValueSerializer.cs
- AnnouncementDispatcherAsyncResult.cs
- DataColumnPropertyDescriptor.cs
- OracleTransaction.cs
- GridViewSortEventArgs.cs
- AsyncOperationContext.cs
- SecurityRuntime.cs
- UriTemplateMatchException.cs
- DataGridRowsPresenter.cs
- WebPartUtil.cs
- HostVisual.cs
- RealProxy.cs
- UpDownEvent.cs
- UIElement3DAutomationPeer.cs
- RelatedPropertyManager.cs
- TextStore.cs
- XmlReflectionImporter.cs