Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / UIAutomation / UIAutomationClient / MS / Internal / Automation / BoundingRectTracker.cs / 1305600 / BoundingRectTracker.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Class used to send BoundingRect changes for hwnds // // History: // 06/17/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Automation; using System.Runtime.InteropServices; using System.ComponentModel; using MS.Win32; namespace MS.Internal.Automation { // BoundingRectTracker - Class used to send BoundingRect changes for hwnds internal class BoundingRectTracker : WinEventWrap { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors internal BoundingRectTracker() : base(new int[]{NativeMethods.EVENT_OBJECT_LOCATIONCHANGE, NativeMethods.EVENT_OBJECT_HIDE}) { // Intentionally not setting the callback for the base WinEventWrap since the WinEventProc override // in this class calls RaiseEventInThisClientOnly to actually raise the event to the client. } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal override void WinEventProc(int eventId, IntPtr hwnd, int idObject, int idChild, uint eventTime) { // Filter... send an event for hwnd only if ( hwnd == IntPtr.Zero || idObject != UnsafeNativeMethods.OBJID_WINDOW ) return; switch (eventId) { case NativeMethods.EVENT_OBJECT_HIDE: OnHide(hwnd, idObject, idChild); break; case NativeMethods.EVENT_OBJECT_LOCATIONCHANGE: OnLocationChange(hwnd, idObject, idChild); break; } } #endregion Internal Methods //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ #region Private Methods private void OnHide(IntPtr hwnd, int idObject, int idChild) { // Clear last hwnd/rect variables (stop looking for dups) _lastHwnd = hwnd; _lastRect = _emptyRect; } private void OnLocationChange(IntPtr hwnd, int idObject, int idChild) { // Filter... send events for visible hwnds only if (!SafeNativeMethods.IsWindowVisible(NativeMethods.HWND.Cast( hwnd ))) return; HandleBoundingRectChange(hwnd); } private void HandleBoundingRectChange(IntPtr hwnd) { NativeMethods.HWND nativeHwnd = NativeMethods.HWND.Cast( hwnd ); NativeMethods.RECT rc32 = new NativeMethods.RECT(0,0,0,0); // if GetWindwRect fails, most likely the nativeHwnd is an invalid window, so just return. if (!Misc.GetWindowRect(nativeHwnd, out rc32)) { return; } // Filter... avoid duplicate events if (hwnd == _lastHwnd && Compare( rc32, _lastRect )) { return; } AutomationElement rawEl = AutomationElement.FromHandle(hwnd); // // AutomationPropertyChangedEventArgs e = new AutomationPropertyChangedEventArgs( AutomationElement.BoundingRectangleProperty, Rect.Empty, new Rect (rc32.left, rc32.top, rc32.right - rc32.left, rc32.bottom - rc32.top)); // ClientEventManager.RaiseEventInThisClientOnly(AutomationElement.AutomationPropertyChangedEvent, rawEl, e); // save the last hwnd/rect for filtering out duplicates _lastHwnd = hwnd; _lastRect = rc32; } // private static bool Compare( NativeMethods.RECT rc1, NativeMethods.RECT rc2 ) { return rc1.left == rc2.left && rc1.top == rc2.top && rc1.right == rc2.right && rc1.bottom == rc2.bottom; } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private static NativeMethods.RECT _emptyRect = new NativeMethods.RECT(0,0,0,0); private NativeMethods.RECT _lastRect; // keep track of last location private IntPtr _lastHwnd; // and hwnd for dup checking #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
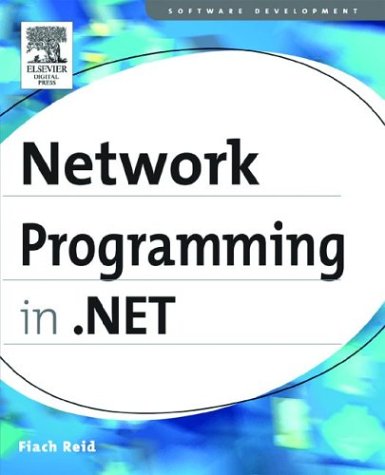
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InsufficientMemoryException.cs
- XmlWhitespace.cs
- NativeMethods.cs
- DCSafeHandle.cs
- TextWriterTraceListener.cs
- WindowsListBox.cs
- SqlCommand.cs
- _DomainName.cs
- DbInsertCommandTree.cs
- Collection.cs
- ServiceThrottle.cs
- GuidelineSet.cs
- ParameterBuilder.cs
- PlanCompilerUtil.cs
- LinkConverter.cs
- SQLString.cs
- FontFamilyValueSerializer.cs
- DurableMessageDispatchInspector.cs
- Internal.cs
- BaseCodePageEncoding.cs
- WebBrowsableAttribute.cs
- VectorKeyFrameCollection.cs
- UpdatePanelControlTrigger.cs
- GridViewDeleteEventArgs.cs
- DeviceSpecificChoice.cs
- Table.cs
- MessageSmuggler.cs
- Aggregates.cs
- SQLInt16.cs
- OpenFileDialog.cs
- DataGridView.cs
- BamlWriter.cs
- TableLayoutSettings.cs
- ContainerFilterService.cs
- PersistChildrenAttribute.cs
- XmlUtil.cs
- TraceData.cs
- SystemNetworkInterface.cs
- DataSourceControlBuilder.cs
- ValidationSummaryDesigner.cs
- TabControlAutomationPeer.cs
- BackgroundWorker.cs
- FilterException.cs
- ControlDesigner.cs
- ModifierKeysValueSerializer.cs
- WindowsGraphics2.cs
- ColumnHeaderConverter.cs
- DesignerTransactionCloseEvent.cs
- HtmlWindow.cs
- SecureConversationVersion.cs
- Vector3DAnimationBase.cs
- SQLGuidStorage.cs
- RuleSettings.cs
- WebPartMenuStyle.cs
- DelegatingHeader.cs
- TypedCompletedAsyncResult.cs
- TrackingCondition.cs
- MetadataArtifactLoaderFile.cs
- StateManagedCollection.cs
- SecuritySessionSecurityTokenProvider.cs
- DbParameterCollectionHelper.cs
- WebPartDisplayModeEventArgs.cs
- ListenerElementsCollection.cs
- StringSorter.cs
- NamespaceExpr.cs
- DecimalAnimationUsingKeyFrames.cs
- HtmlInputRadioButton.cs
- UrlMappingCollection.cs
- HistoryEventArgs.cs
- LocalClientSecuritySettingsElement.cs
- RelatedPropertyManager.cs
- DefaultPrintController.cs
- OracleConnectionStringBuilder.cs
- DefinitionUpdate.cs
- SupportingTokenProviderSpecification.cs
- PartialCachingAttribute.cs
- basenumberconverter.cs
- dtdvalidator.cs
- XmlSchemaComplexContentExtension.cs
- PermissionAttributes.cs
- SqlUDTStorage.cs
- TraceSection.cs
- UnsafeNetInfoNativeMethods.cs
- WindowsListViewGroupSubsetLink.cs
- Popup.cs
- EventHandlersStore.cs
- StateMachineAction.cs
- ThreadAbortException.cs
- FlowDocumentReader.cs
- Internal.cs
- KeyEventArgs.cs
- CodeTypeConstructor.cs
- TableNameAttribute.cs
- LinkedResource.cs
- GPRECT.cs
- DatePicker.cs
- FixedSOMElement.cs
- ActivityStatusChangeEventArgs.cs
- TagPrefixAttribute.cs
- PageAsyncTaskManager.cs