Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / Log / System / IO / Log / AppendHelper.cs / 1305376 / AppendHelper.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Runtime.InteropServices; namespace System.IO.Log { class AppendHelper : IDisposable { SequenceNumber prev; SequenceNumber next; FileLogRecordHeader header; UnmanagedBlob[] blobs; GCHandle[] handles; public AppendHelper(IList> data, SequenceNumber prev, SequenceNumber next, bool restartArea) { this.prev = prev; this.next = next; this.header = new FileLogRecordHeader(null); this.header.IsRestartArea = restartArea; this.header.PreviousLsn = prev; this.header.NextUndoLsn = next; this.blobs = new UnmanagedBlob[data.Count + 1]; this.handles = new GCHandle[data.Count + 1]; try { this.handles[0] = GCHandle.Alloc(header.Bits, GCHandleType.Pinned); this.blobs[0].cbSize = (uint)FileLogRecordHeader.Size; this.blobs[0].pBlobData = Marshal.UnsafeAddrOfPinnedArrayElement(header.Bits, 0); for (int i = 0; i < data.Count; i++) { handles[i + 1] = GCHandle.Alloc(data[i].Array, GCHandleType.Pinned); blobs[i + 1].cbSize = (uint)data[i].Count; blobs[i + 1].pBlobData = Marshal.UnsafeAddrOfPinnedArrayElement(data[i].Array, data[i].Offset); } } catch { Dispose(); throw; } } public UnmanagedBlob[] Blobs { get { return this.blobs; } } // Caller should always call Dispose. Finalizer not implemented. public void Dispose() { try { lock(this) { for (int i = 0; i < handles.Length; i++) { if (handles[i].IsAllocated) handles[i].Free(); } } } catch(InvalidOperationException exception) { // This indicates something is broken in IO.Log's memory management, // so it's not safe to continue executing DiagnosticUtility.InvokeFinalHandler(exception); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Runtime.InteropServices; namespace System.IO.Log { class AppendHelper : IDisposable { SequenceNumber prev; SequenceNumber next; FileLogRecordHeader header; UnmanagedBlob[] blobs; GCHandle[] handles; public AppendHelper(IList > data, SequenceNumber prev, SequenceNumber next, bool restartArea) { this.prev = prev; this.next = next; this.header = new FileLogRecordHeader(null); this.header.IsRestartArea = restartArea; this.header.PreviousLsn = prev; this.header.NextUndoLsn = next; this.blobs = new UnmanagedBlob[data.Count + 1]; this.handles = new GCHandle[data.Count + 1]; try { this.handles[0] = GCHandle.Alloc(header.Bits, GCHandleType.Pinned); this.blobs[0].cbSize = (uint)FileLogRecordHeader.Size; this.blobs[0].pBlobData = Marshal.UnsafeAddrOfPinnedArrayElement(header.Bits, 0); for (int i = 0; i < data.Count; i++) { handles[i + 1] = GCHandle.Alloc(data[i].Array, GCHandleType.Pinned); blobs[i + 1].cbSize = (uint)data[i].Count; blobs[i + 1].pBlobData = Marshal.UnsafeAddrOfPinnedArrayElement(data[i].Array, data[i].Offset); } } catch { Dispose(); throw; } } public UnmanagedBlob[] Blobs { get { return this.blobs; } } // Caller should always call Dispose. Finalizer not implemented. public void Dispose() { try { lock(this) { for (int i = 0; i < handles.Length; i++) { if (handles[i].IsAllocated) handles[i].Free(); } } } catch(InvalidOperationException exception) { // This indicates something is broken in IO.Log's memory management, // so it's not safe to continue executing DiagnosticUtility.InvokeFinalHandler(exception); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
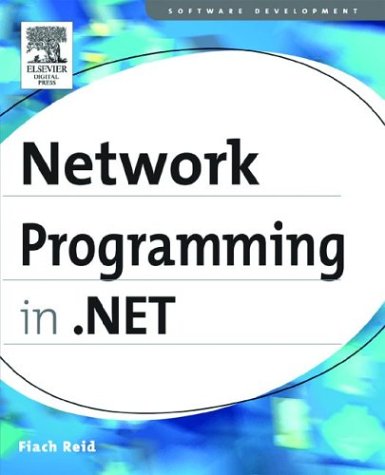
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ContainerSelectorGlyph.cs
- SqlNotificationEventArgs.cs
- ExecutedRoutedEventArgs.cs
- DbProviderConfigurationHandler.cs
- BooleanStorage.cs
- EntryWrittenEventArgs.cs
- ListDataBindEventArgs.cs
- ActivityWithResultValueSerializer.cs
- WindowsGraphics.cs
- EntityContainerRelationshipSet.cs
- CheckBox.cs
- CharAnimationBase.cs
- DiscoveryCallbackBehavior.cs
- ObjectItemCollectionAssemblyCacheEntry.cs
- PenThread.cs
- MasterPageCodeDomTreeGenerator.cs
- CopyCodeAction.cs
- ProcessHostFactoryHelper.cs
- FontStyle.cs
- RNGCryptoServiceProvider.cs
- Pointer.cs
- DrawingContextWalker.cs
- COM2IProvidePropertyBuilderHandler.cs
- OdbcConnectionHandle.cs
- MenuItemBinding.cs
- WizardPanel.cs
- MenuItemCollectionEditorDialog.cs
- Maps.cs
- UseAttributeSetsAction.cs
- MultiBinding.cs
- StylusPlugin.cs
- TdsParserHelperClasses.cs
- StructuralObject.cs
- DataTemplateSelector.cs
- _SslStream.cs
- Grid.cs
- TempFiles.cs
- AutoGeneratedFieldProperties.cs
- SamlAuthorizationDecisionStatement.cs
- QuaternionConverter.cs
- DataRowChangeEvent.cs
- ConnectionConsumerAttribute.cs
- InheritanceContextHelper.cs
- OperationCanceledException.cs
- FormsAuthenticationEventArgs.cs
- WebPartCatalogCloseVerb.cs
- TcpDuplicateContext.cs
- Quad.cs
- MarginsConverter.cs
- RoleManagerModule.cs
- ISAPIRuntime.cs
- PropertyValue.cs
- BuilderPropertyEntry.cs
- RangeValuePattern.cs
- FlowPosition.cs
- FacetValues.cs
- followingsibling.cs
- EnlistmentState.cs
- SizeKeyFrameCollection.cs
- EntityDataSourceWrapperPropertyDescriptor.cs
- DateTimeSerializationSection.cs
- EventLog.cs
- CodeVariableDeclarationStatement.cs
- ManagedFilter.cs
- WinFormsSpinner.cs
- Subtree.cs
- TextElementEnumerator.cs
- DefaultBinder.cs
- XmlSiteMapProvider.cs
- WeakReferenceKey.cs
- StsCommunicationException.cs
- ButtonStandardAdapter.cs
- GreenMethods.cs
- httpserverutility.cs
- WebPartActionVerb.cs
- BoundsDrawingContextWalker.cs
- PeerFlooder.cs
- ScaleTransform3D.cs
- ComponentCollection.cs
- HwndSourceParameters.cs
- Memoizer.cs
- XmlIlGenerator.cs
- ImpersonateTokenRef.cs
- ServicesUtilities.cs
- LineSegment.cs
- ValueQuery.cs
- RunWorkerCompletedEventArgs.cs
- MetricEntry.cs
- SecurityPermission.cs
- OpenFileDialog.cs
- HyperLink.cs
- TypedTableBase.cs
- StructureChangedEventArgs.cs
- CommandField.cs
- SchemaObjectWriter.cs
- TimeIntervalCollection.cs
- PropertyIDSet.cs
- UInt32Storage.cs
- DateRangeEvent.cs
- DrawingContextDrawingContextWalker.cs