Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / ToolStripProgressBar.cs / 1305376 / ToolStripProgressBar.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Windows.Forms; using System.ComponentModel; using System.Drawing; using System.Security; using System.Security.Permissions; ///[DefaultProperty("Value")] public class ToolStripProgressBar : ToolStripControlHost { internal static readonly object EventRightToLeftLayoutChanged = new object(); /// public ToolStripProgressBar() : base(CreateControlInstance()) { } public ToolStripProgressBar(string name) : this() { this.Name = name; } /// /// /// Create a strongly typed accessor for the class /// ///[Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public ProgressBar ProgressBar { get { return this.Control as ProgressBar; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public override Image BackgroundImage { get { return base.BackgroundImage; } set { base.BackgroundImage = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override ImageLayout BackgroundImageLayout { get { return base.BackgroundImageLayout; } set { base.BackgroundImageLayout = value; } } /// /// /// Specify what size you want the item to start out at /// ///protected override System.Drawing.Size DefaultSize { get { return new Size(100,15); } } /// /// /// Specify how far from the edges you want to be /// ///protected internal override Padding DefaultMargin { get { if (this.Owner != null && this.Owner is StatusStrip) { return new Padding(1, 3, 1, 3); } else { return new Padding(1, 2, 1, 1); } } } [ DefaultValue(100), SRCategory(SR.CatBehavior), SRDescription(SR.ProgressBarMarqueeAnimationSpeed) ] public int MarqueeAnimationSpeed { get { return ProgressBar.MarqueeAnimationSpeed; } set { ProgressBar.MarqueeAnimationSpeed = value; } } [ DefaultValue(100), SRCategory(SR.CatBehavior), RefreshProperties(RefreshProperties.Repaint), SRDescription(SR.ProgressBarMaximumDescr) ] public int Maximum { get { return ProgressBar.Maximum; } set { ProgressBar.Maximum = value; } } [ DefaultValue(0), SRCategory(SR.CatBehavior), RefreshProperties(RefreshProperties.Repaint), SRDescription(SR.ProgressBarMinimumDescr) ] public int Minimum { get { return ProgressBar.Minimum; } set { ProgressBar.Minimum = value; } } /// /// /// This is used for international applications where the language /// is written from RightToLeft. When this property is true, // and the RightToLeft is true, mirroring will be turned on on the form, and /// control placement and text will be from right to left. /// [ SRCategory(SR.CatAppearance), Localizable(true), DefaultValue(false), SRDescription(SR.ControlRightToLeftLayoutDescr) ] public virtual bool RightToLeftLayout { get { return ProgressBar.RightToLeftLayout; } set { ProgressBar.RightToLeftLayout = value; } } ////// /// Wrap some commonly used properties /// ///[ DefaultValue(10), SRCategory(SR.CatBehavior), SRDescription(SR.ProgressBarStepDescr) ] public int Step { get { return ProgressBar.Step; } set { ProgressBar.Step = value; } } /// /// /// Wrap some commonly used properties /// ///[ DefaultValue(ProgressBarStyle.Blocks), SRCategory(SR.CatBehavior), SRDescription(SR.ProgressBarStyleDescr) ] public ProgressBarStyle Style { get { return ProgressBar.Style; } set { ProgressBar.Style = value; } } /// /// /// Hide the property. /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override string Text { get { return Control.Text; } set { Control.Text = value; } } ////// /// Wrap some commonly used properties /// ///[ DefaultValue(0), SRCategory(SR.CatBehavior), Bindable(true), SRDescription(SR.ProgressBarValueDescr) ] public int Value { get { return ProgressBar.Value; } set { ProgressBar.Value = value; } } private static Control CreateControlInstance() { ProgressBar progressBar = new ProgressBar(); progressBar.Size = new Size(100,15); return progressBar; } private void HandleRightToLeftLayoutChanged(object sender, EventArgs e) { OnRightToLeftLayoutChanged(e); } /// protected virtual void OnRightToLeftLayoutChanged(EventArgs e) { RaiseEvent(EventRightToLeftLayoutChanged, e); } /// protected override void OnSubscribeControlEvents(Control control) { ProgressBar bar = control as ProgressBar; if (bar != null) { // Please keep this alphabetized and in [....] with Unsubscribe // bar.RightToLeftLayoutChanged += new EventHandler(HandleRightToLeftLayoutChanged); } base.OnSubscribeControlEvents(control); } /// protected override void OnUnsubscribeControlEvents(Control control) { ProgressBar bar = control as ProgressBar; if (bar != null) { // Please keep this alphabetized and in [....] with Subscribe // bar.RightToLeftLayoutChanged -= new EventHandler(HandleRightToLeftLayoutChanged); } base.OnUnsubscribeControlEvents(control); } /// /// /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never) ] new public event KeyEventHandler KeyDown { add { base.KeyDown += value; } remove { base.KeyDown -= value; } } ///Hide the event. ////// /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never) ] new public event KeyPressEventHandler KeyPress { add { base.KeyPress += value; } remove { base.KeyPress -= value; } } ///Hide the event. ////// /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never) ] new public event KeyEventHandler KeyUp { add { base.KeyUp += value; } remove { base.KeyUp -= value; } } ///Hide the event. ////// /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never) ] new public event EventHandler LocationChanged { add { base.LocationChanged += value; } remove { base.LocationChanged -= value; } } ///Hide the event. ////// /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never) ] new public event EventHandler OwnerChanged { add { base.OwnerChanged += value; } remove { base.OwnerChanged -= value; } } ///Hide the event. ///[SRCategory(SR.CatPropertyChanged), SRDescription(SR.ControlOnRightToLeftLayoutChangedDescr)] public event EventHandler RightToLeftLayoutChanged { add { Events.AddHandler(EventRightToLeftLayoutChanged, value); } remove { Events.RemoveHandler(EventRightToLeftLayoutChanged, value); } } /// /// /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never) ] new public event EventHandler TextChanged { add { base.TextChanged += value; } remove { base.TextChanged -= value; } } ///Hide the event. ////// /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never) ] new public event EventHandler Validated { add { base.Validated += value; } remove { base.Validated -= value; } } ///Hide the event. ////// /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never) ] new public event CancelEventHandler Validating { add { base.Validating += value; } remove { base.Validating -= value; } } public void Increment(int value) { ProgressBar.Increment(value); } public void PerformStep() { ProgressBar.PerformStep(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Hide the event. ///
Link Menu
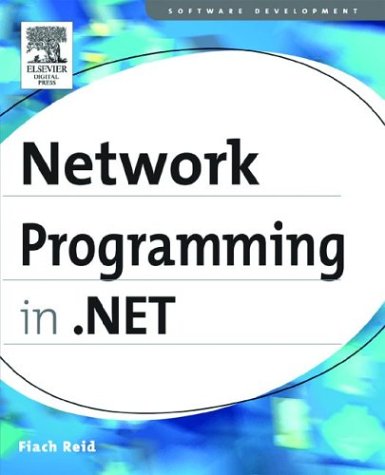
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CommandDevice.cs
- CodeThrowExceptionStatement.cs
- PasswordDeriveBytes.cs
- CachedFontFace.cs
- ImportStoreException.cs
- COM2TypeInfoProcessor.cs
- PropertyValueChangedEvent.cs
- ListBindableAttribute.cs
- ToolTipAutomationPeer.cs
- XmlAnyElementAttributes.cs
- SubordinateTransaction.cs
- GetPageNumberCompletedEventArgs.cs
- Token.cs
- MemoryRecordBuffer.cs
- EntityExpressionVisitor.cs
- CookieParameter.cs
- CodeDOMUtility.cs
- Panel.cs
- GridViewColumnCollectionChangedEventArgs.cs
- SuppressMergeCheckAttribute.cs
- PageRanges.cs
- XmlDocumentViewSchema.cs
- ExpressionNode.cs
- HtmlInputHidden.cs
- TextTreeRootNode.cs
- LinearGradientBrush.cs
- DispatcherEventArgs.cs
- Exceptions.cs
- CompilerCollection.cs
- StatusBarDrawItemEvent.cs
- AlternationConverter.cs
- ConnectionManagementElementCollection.cs
- Slider.cs
- ExceptionValidationRule.cs
- RegexCompilationInfo.cs
- AutomationPeer.cs
- EntityConnectionStringBuilder.cs
- Permission.cs
- PointLight.cs
- ParentUndoUnit.cs
- UIElementParagraph.cs
- KeyGestureValueSerializer.cs
- RoutedEventArgs.cs
- TextBox.cs
- DataRow.cs
- xamlnodes.cs
- COM2TypeInfoProcessor.cs
- BitSet.cs
- ScriptResourceHandler.cs
- PasswordRecovery.cs
- Animatable.cs
- SimpleTypeResolver.cs
- FigureHelper.cs
- CacheVirtualItemsEvent.cs
- TemplateBaseAction.cs
- Label.cs
- ProfessionalColorTable.cs
- EnumerableCollectionView.cs
- SqlServices.cs
- InvalidFilterCriteriaException.cs
- ImageAttributes.cs
- MatrixTransform3D.cs
- EventsTab.cs
- DefaultWorkflowSchedulerService.cs
- MarkerProperties.cs
- counter.cs
- XmlCustomFormatter.cs
- HandleCollector.cs
- DesignerAdapterUtil.cs
- Trace.cs
- SQLDateTime.cs
- SmtpClient.cs
- InternalMappingException.cs
- NativeWindow.cs
- ExcCanonicalXml.cs
- CompilationRelaxations.cs
- ping.cs
- StringArrayConverter.cs
- TemplateComponentConnector.cs
- ReadOnlyHierarchicalDataSource.cs
- RegisteredExpandoAttribute.cs
- FixedTextPointer.cs
- ColumnCollection.cs
- NumericUpDownAccelerationCollection.cs
- RepeatBehaviorConverter.cs
- TableParaClient.cs
- SafeHandles.cs
- CollectionChangedEventManager.cs
- DriveNotFoundException.cs
- Connector.cs
- ClientBuildManagerCallback.cs
- Button.cs
- BitmapMetadata.cs
- HostProtectionPermission.cs
- ExtendedProtectionPolicyTypeConverter.cs
- BrowserDefinitionCollection.cs
- PocoEntityKeyStrategy.cs
- ToolStripSplitStackLayout.cs
- AsyncResult.cs
- ReadOnlyNameValueCollection.cs