Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Data / System / Data / Filter / ExpressionNode.cs / 1305376 / ExpressionNode.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data { using System; using System.Collections.Generic; using System.Data.Common; using System.Data.SqlTypes; internal abstract class ExpressionNode { private DataTable _table; protected ExpressionNode(DataTable table) { _table = table; } internal IFormatProvider FormatProvider { get { return ((null != _table) ? _table.FormatProvider : System.Globalization.CultureInfo.CurrentCulture); } } internal virtual bool IsSqlColumn{ get{ return false; } } protected DataTable table { get { return _table; } } protected void BindTable(DataTable table) { // when the expression is created, DataColumn may not be associated with a table yet _table = table; } internal abstract void Bind(DataTable table, Listlist); internal abstract object Eval(); internal abstract object Eval(DataRow row, DataRowVersion version); internal abstract object Eval(int[] recordNos); internal abstract bool IsConstant(); internal abstract bool IsTableConstant(); internal abstract bool HasLocalAggregate(); internal abstract bool HasRemoteAggregate(); internal abstract ExpressionNode Optimize(); internal virtual bool DependsOn(DataColumn column) { return false; } internal static bool IsInteger(StorageType type) { return(type == StorageType.Int16 || type == StorageType.Int32 || type == StorageType.Int64 || type == StorageType.UInt16 || type == StorageType.UInt32 || type == StorageType.UInt64 || type == StorageType.SByte || type == StorageType.Byte); } internal static bool IsIntegerSql(StorageType type) { return(type == StorageType.Int16 || type == StorageType.Int32 || type == StorageType.Int64 || type == StorageType.UInt16 || type == StorageType.UInt32 || type == StorageType.UInt64 || type == StorageType.SByte || type == StorageType.Byte || type == StorageType.SqlInt64 || type == StorageType.SqlInt32 || type == StorageType.SqlInt16 || type == StorageType.SqlByte); } internal static bool IsSigned(StorageType type) { return(type == StorageType.Int16 || type == StorageType.Int32 || type == StorageType.Int64 || type == StorageType.SByte || IsFloat(type)); } internal static bool IsSignedSql(StorageType type) { return(type == StorageType.Int16 || // IsSigned(type) type == StorageType.Int32 || type == StorageType.Int64 || type == StorageType.SByte || type == StorageType.SqlInt64 || type == StorageType.SqlInt32 || type == StorageType.SqlInt16 || IsFloatSql(type)); } internal static bool IsUnsigned(StorageType type) { return(type == StorageType.UInt16 || type == StorageType.UInt32 || type == StorageType.UInt64 || type == StorageType.Byte); } internal static bool IsUnsignedSql(StorageType type) { return(type == StorageType.UInt16 || type == StorageType.UInt32 || type == StorageType.UInt64 || type == StorageType.SqlByte ||// SqlByte represents an 8-bit unsigned integer, in the range of 0 through 255, type == StorageType.Byte); } internal static bool IsNumeric(StorageType type) { return(IsFloat(type) || IsInteger(type)); } internal static bool IsNumericSql(StorageType type) { return(IsFloatSql(type) || IsIntegerSql(type)); } internal static bool IsFloat(StorageType type) { return(type == StorageType.Single || type == StorageType.Double || type == StorageType.Decimal); } internal static bool IsFloatSql(StorageType type) { return(type == StorageType.Single || type == StorageType.Double || type == StorageType.Decimal || type == StorageType.SqlDouble || type == StorageType.SqlDecimal || // I expect decimal to be Integer! type == StorageType.SqlMoney || // if decimal is here, this should be definitely here! type == StorageType.SqlSingle); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
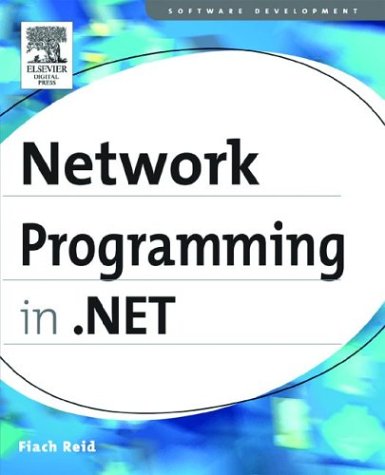
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InkPresenter.cs
- Pair.cs
- CompressionTransform.cs
- CompressionTracing.cs
- DropShadowEffect.cs
- PrintEvent.cs
- NetStream.cs
- AnnotationMap.cs
- Visual3DCollection.cs
- RenderOptions.cs
- TranslateTransform.cs
- XmlWrappingWriter.cs
- WebPartMenu.cs
- Animatable.cs
- AdornedElementPlaceholder.cs
- MulticastDelegate.cs
- TextEvent.cs
- TemplateControl.cs
- Msec.cs
- ShapingEngine.cs
- PageThemeCodeDomTreeGenerator.cs
- CachedFontFace.cs
- FormsAuthentication.cs
- ToolStripPanelSelectionGlyph.cs
- PasswordRecovery.cs
- ProjectionPathSegment.cs
- ClientUrlResolverWrapper.cs
- DBDataPermissionAttribute.cs
- XsdDataContractExporter.cs
- MethodRental.cs
- WindowPatternIdentifiers.cs
- StringCollectionMarkupSerializer.cs
- Substitution.cs
- ActiveDesignSurfaceEvent.cs
- PolyBezierSegmentFigureLogic.cs
- LockCookie.cs
- UrlMappingsSection.cs
- Debugger.cs
- RoutedEvent.cs
- OptimizedTemplateContentHelper.cs
- ColumnResult.cs
- OutputCacheProfileCollection.cs
- WebRequestModuleElement.cs
- ToolBarTray.cs
- MenuItem.cs
- _NtlmClient.cs
- NavigationProgressEventArgs.cs
- OpenTypeCommon.cs
- SurrogateChar.cs
- ObjectViewListener.cs
- ToolStripHighContrastRenderer.cs
- EntityCollection.cs
- SettingsAttributeDictionary.cs
- ApplicationGesture.cs
- SqlTrackingQuery.cs
- CompilationUnit.cs
- User.cs
- NaturalLanguageHyphenator.cs
- XamlInt32CollectionSerializer.cs
- CommonXSendMessage.cs
- EditCommandColumn.cs
- WindowsHyperlink.cs
- FormViewUpdatedEventArgs.cs
- SiteMembershipCondition.cs
- TimeZone.cs
- Deflater.cs
- EventToken.cs
- ActivityBuilderHelper.cs
- TimersDescriptionAttribute.cs
- HideDisabledControlAdapter.cs
- SqlDataSourceView.cs
- Base64Stream.cs
- Camera.cs
- xmlsaver.cs
- MouseGestureValueSerializer.cs
- CodeDefaultValueExpression.cs
- CollectionViewGroupInternal.cs
- TimeSpan.cs
- TextDpi.cs
- EdmMember.cs
- PointAnimationUsingKeyFrames.cs
- XmlSchemaObject.cs
- XPathEmptyIterator.cs
- KnownBoxes.cs
- unsafenativemethodstextservices.cs
- PropertyValidationContext.cs
- SQLInt64Storage.cs
- SecurityTokenResolver.cs
- TreeViewCancelEvent.cs
- ObjectSet.cs
- RC2.cs
- ListMarkerSourceInfo.cs
- ObjectStateManagerMetadata.cs
- ParallelTimeline.cs
- WindowsMenu.cs
- FileDialogCustomPlace.cs
- BaseComponentEditor.cs
- Switch.cs
- ContractListAdapter.cs
- XPathMultyIterator.cs