Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Documents / NaturalLanguageHyphenator.cs / 1305600 / NaturalLanguageHyphenator.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: // Implementation of TextLexicalService abstract class used by TextFormatter for // document layout. This implementation is based on the hyphenation service in // NaturalLanguage6.dll - the component owned by the Natural Language Team. // // History: // 06/28/2005 : Worachai Chaoweeraprasit (Wchao) - created // //--------------------------------------------------------------------------- using System.Security; using System.Collections; using System.Globalization; using System.IO; using System.Runtime.InteropServices; using System.Windows.Media.TextFormatting; using MS.Win32; using MS.Internal; namespace System.Windows.Documents { ////// The NLG hyphenation-based implementation of TextLexicalService used by TextFormatter /// for line-breaking purpose. /// internal class NaturalLanguageHyphenator : TextLexicalService, IDisposable { ////// Critical: Holds a COM component instance that has unmanged code elevations /// [SecurityCritical] private IntPtr _hyphenatorResource; private bool _disposed; ////// Construct an NLG-based hyphenator /// ////// Critical: This code calls into NlCreateHyphenator, which elevates unmanaged code permission. /// TreatAsSafe: This function call takes no input parameters /// [SecurityCritical, SecurityTreatAsSafe] internal NaturalLanguageHyphenator() { try { _hyphenatorResource = UnsafeNativeMethods.NlCreateHyphenator(); } catch (DllNotFoundException) { } catch (EntryPointNotFoundException) { } } ////// Finalize hyphenator's unmanaged resource /// ~NaturalLanguageHyphenator() { CleanupInternal(true); } ////// Dispose hyphenator's unmanaged resource /// void IDisposable.Dispose() { GC.SuppressFinalize(this); CleanupInternal(false); } ////// Internal clean-up routine /// ////// Critical: This code calls into NlDestroyHyphenator, which elevates unmanaged code permission. /// TreatAsSafe: This function call takes no input memory block /// [SecurityCritical, SecurityTreatAsSafe] private void CleanupInternal(bool finalizing) { if (!_disposed && _hyphenatorResource != IntPtr.Zero) { UnsafeNativeMethods.NlDestroyHyphenator(ref _hyphenatorResource); _disposed = true; } } ////// TextFormatter to query whether the lexical services component could provides /// analysis for the specified culture. /// /// Culture whose text is to be analyzed ///Boolean value indicates whether the specified culture is supported public override bool IsCultureSupported(CultureInfo culture) { // Accept all cultures for the time being. Ideally NL6 should provide a way for the client // to test supported culture. return true; } ////// TextFormatter to get the lexical breaks of the specified raw text /// /// character array /// number of character in the character array to analyze /// culture of the specified character source ///lexical breaks of the text ////// Critical: This code calls NlHyphenate which is critical. /// TreatAsSafe: This code accepts a buffer that is length checked and returns /// data that is ok to return. /// [SecurityCritical, SecurityTreatAsSafe] public override TextLexicalBreaks AnalyzeText( char[] characterSource, int length, CultureInfo textCulture ) { Invariant.Assert( characterSource != null && characterSource.Length > 0 && length > 0 && length <= characterSource.Length ); if (_hyphenatorResource == IntPtr.Zero) { // No hyphenator available, no service delivered return null; } if (_disposed) { throw new ObjectDisposedException(SR.Get(SRID.HyphenatorDisposed)); } byte[] isHyphenPositions = new byte[(length + 7) / 8]; UnsafeNativeMethods.NlHyphenate( _hyphenatorResource, characterSource, length, ((textCulture != null && textCulture != CultureInfo.InvariantCulture) ? textCulture.LCID : 0), isHyphenPositions, isHyphenPositions.Length ); return new HyphenBreaks(isHyphenPositions, length); } ////// Private implementation of TextLexicalBreaks that encapsulates hyphen opportunities within /// a character string. /// private class HyphenBreaks : TextLexicalBreaks { private byte[] _isHyphenPositions; private int _numPositions; internal HyphenBreaks(byte[] isHyphenPositions, int numPositions) { _isHyphenPositions = isHyphenPositions; _numPositions = numPositions; } ////// Indexer for the value at the nth break index (bit nth of the logical bit array) /// private bool this[int index] { get { return (_isHyphenPositions[index / 8] & (1 << index % 8)) != 0; } } public override int Length { get { return _numPositions; } } public override int GetNextBreak(int currentIndex) { if (_isHyphenPositions != null && currentIndex >= 0) { int ich = currentIndex + 1; while (ich < _numPositions && !this[ich]) ich++; if (ich < _numPositions) return ich; } // return negative value when break is not found. return -1; } public override int GetPreviousBreak(int currentIndex) { if (_isHyphenPositions != null && currentIndex < _numPositions) { int ich = currentIndex; while (ich > 0 && !this[ich]) ich--; if (ich > 0) return ich; } // return negative value when break is not found. return -1; } } private static class UnsafeNativeMethods { ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(Microsoft.Internal.DllImport.PresentationNative, PreserveSig = false)] internal static extern IntPtr NlCreateHyphenator(); ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(Microsoft.Internal.DllImport.PresentationNative, PreserveSig = false)] internal static extern void NlDestroyHyphenator(ref IntPtr hyphenator); ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(Microsoft.Internal.DllImport.PresentationNative, PreserveSig = false)] internal static extern void NlHyphenate( IntPtr hyphenator, [In, MarshalAs(UnmanagedType.LPArray, ArraySubType = UnmanagedType.U2, SizeParamIndex = 2)] char[] inputText, int textLength, int localeID, [In, MarshalAs(UnmanagedType.LPArray, SizeParamIndex = 5)] byte[] hyphenBreaks, int numPositions ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
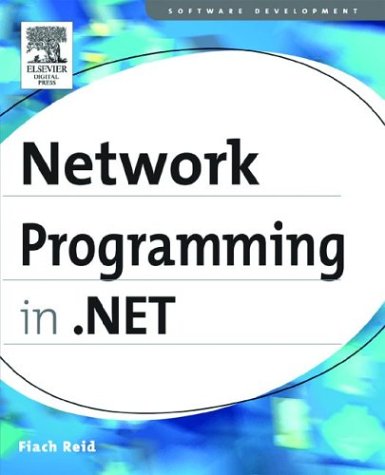
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlError.cs
- ProcessHostFactoryHelper.cs
- ListViewUpdateEventArgs.cs
- WebControlsSection.cs
- NotifyInputEventArgs.cs
- ProxyWebPartManager.cs
- DbDataSourceEnumerator.cs
- AssemblyName.cs
- DataGridComponentEditor.cs
- MobileCapabilities.cs
- SafeNativeMethodsMilCoreApi.cs
- KnownTypes.cs
- PageTheme.cs
- DefaultClaimSet.cs
- QilLiteral.cs
- TimersDescriptionAttribute.cs
- CustomLineCap.cs
- FormsIdentity.cs
- OdbcUtils.cs
- embossbitmapeffect.cs
- ContractListAdapter.cs
- FactoryGenerator.cs
- IQueryable.cs
- PermissionSet.cs
- SmtpMail.cs
- TextFormatterContext.cs
- XmlNamespaceMapping.cs
- PieceNameHelper.cs
- ListItemCollection.cs
- UnauthorizedAccessException.cs
- PrivateFontCollection.cs
- SQLResource.cs
- ListViewDeletedEventArgs.cs
- PowerModeChangedEventArgs.cs
- TemplateBaseAction.cs
- ServiceReference.cs
- HtmlControl.cs
- SqlNodeAnnotation.cs
- PropertyGridEditorPart.cs
- WindowsFormsLinkLabel.cs
- Point3DCollectionValueSerializer.cs
- ListViewInsertionMark.cs
- LogReserveAndAppendState.cs
- ThreadPool.cs
- ParentUndoUnit.cs
- PackageFilter.cs
- ComponentCollection.cs
- XmlMembersMapping.cs
- ApplicationContext.cs
- UnhandledExceptionEventArgs.cs
- GridViewCommandEventArgs.cs
- ProcessRequestArgs.cs
- HtmlInputReset.cs
- DataRowCollection.cs
- TokenizerHelper.cs
- SessionPageStatePersister.cs
- Pts.cs
- Style.cs
- SessionPageStateSection.cs
- HtmlTable.cs
- Content.cs
- Hash.cs
- RoleService.cs
- SafeNativeMethodsMilCoreApi.cs
- AvTraceFormat.cs
- AddInProcess.cs
- Listener.cs
- ConfigurationManagerInternalFactory.cs
- UnknownWrapper.cs
- X509Chain.cs
- WebBrowserUriTypeConverter.cs
- SystemIPv6InterfaceProperties.cs
- ObjectConverter.cs
- DataGridViewIntLinkedList.cs
- ProfileSettingsCollection.cs
- CuspData.cs
- BaseComponentEditor.cs
- WhitespaceRuleLookup.cs
- BridgeDataRecord.cs
- StrongNameKeyPair.cs
- ConnectionManagementElementCollection.cs
- ToolboxComponentsCreatingEventArgs.cs
- RoleGroup.cs
- StringCollectionMarkupSerializer.cs
- RedBlackList.cs
- RenderTargetBitmap.cs
- MonitorWrapper.cs
- EventBuilder.cs
- DataObjectAttribute.cs
- Misc.cs
- SectionInformation.cs
- _NetRes.cs
- HostedElements.cs
- InfoCardX509Validator.cs
- LogLogRecordEnumerator.cs
- InvalidComObjectException.cs
- DataTableTypeConverter.cs
- SortedList.cs
- UICuesEvent.cs
- AsyncStreamReader.cs