Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / UI / WebControls / EditCommandColumn.cs / 1 / EditCommandColumn.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Web.UI; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class EditCommandColumn : DataGridColumn { ///Creates a special column with buttons for ///, /// , and commands to edit items /// within the selected row. /// public EditCommandColumn() { } ///Initializes a new instance of an ///class. /// [ DefaultValue(ButtonColumnType.LinkButton) ] public virtual ButtonColumnType ButtonType { get { object o = ViewState["ButtonType"]; if (o != null) return(ButtonColumnType)o; return ButtonColumnType.LinkButton; } set { if (value < ButtonColumnType.LinkButton || value > ButtonColumnType.PushButton) { throw new ArgumentOutOfRangeException("value"); } ViewState["ButtonType"] = value; OnColumnChanged(); } } ///Indicates the button type for the column. ////// [ Localizable(true), DefaultValue("") ] public virtual string CancelText { get { object o = ViewState["CancelText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["CancelText"] = value; OnColumnChanged(); } } [ DefaultValue(true), ] public virtual bool CausesValidation { get { object o = ViewState["CausesValidation"]; if (o != null) { return (bool)o; } return true; } set { ViewState["CausesValidation"] = value; OnColumnChanged(); } } ///Indicates the text to display for the ///command button /// in the column. /// [ Localizable(true), DefaultValue("") ] public virtual string EditText { get { object o = ViewState["EditText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["EditText"] = value; OnColumnChanged(); } } ///Indicates the text to display for the ///command button in /// the column. /// [ Localizable(true), DefaultValue("") ] public virtual string UpdateText { get { object o = ViewState["UpdateText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["UpdateText"] = value; OnColumnChanged(); } } [ DefaultValue(""), ] public virtual string ValidationGroup { get { object o = ViewState["ValidationGroup"]; if (o != null) { return (string)o; } return String.Empty; } set { ViewState["ValidationGroup"] = value; OnColumnChanged(); } } private void AddButtonToCell(TableCell cell, string commandName, string buttonText, bool causesValidation, string validationGroup) { WebControl buttonControl = null; ControlCollection controls = cell.Controls; ButtonColumnType buttonType = ButtonType; if (buttonType == ButtonColumnType.LinkButton) { LinkButton button = new DataGridLinkButton(); buttonControl = button; button.CommandName = commandName; button.Text = buttonText; button.CausesValidation = causesValidation; button.ValidationGroup = validationGroup; } else { Button button = new Button(); buttonControl = button; button.CommandName = commandName; button.Text = buttonText; button.CausesValidation = causesValidation; button.ValidationGroup = validationGroup; } controls.Add(buttonControl); } ///Indicates the text to display for the ///command button /// in the column. /// public override void InitializeCell(TableCell cell, int columnIndex, ListItemType itemType) { base.InitializeCell(cell, columnIndex, itemType); bool causesValidation = CausesValidation; if ((itemType != ListItemType.Header) && (itemType != ListItemType.Footer)) { if (itemType == ListItemType.EditItem) { ControlCollection controls = cell.Controls; AddButtonToCell(cell, DataGrid.UpdateCommandName, UpdateText, causesValidation, ValidationGroup); LiteralControl spaceControl = new LiteralControl(" "); controls.Add(spaceControl); AddButtonToCell(cell, DataGrid.CancelCommandName, CancelText, false, String.Empty); } else { AddButtonToCell(cell, DataGrid.EditCommandName, EditText, false, String.Empty); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Initializes a cell within the column. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Web.UI; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class EditCommandColumn : DataGridColumn { ///Creates a special column with buttons for ///, /// , and commands to edit items /// within the selected row. /// public EditCommandColumn() { } ///Initializes a new instance of an ///class. /// [ DefaultValue(ButtonColumnType.LinkButton) ] public virtual ButtonColumnType ButtonType { get { object o = ViewState["ButtonType"]; if (o != null) return(ButtonColumnType)o; return ButtonColumnType.LinkButton; } set { if (value < ButtonColumnType.LinkButton || value > ButtonColumnType.PushButton) { throw new ArgumentOutOfRangeException("value"); } ViewState["ButtonType"] = value; OnColumnChanged(); } } ///Indicates the button type for the column. ////// [ Localizable(true), DefaultValue("") ] public virtual string CancelText { get { object o = ViewState["CancelText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["CancelText"] = value; OnColumnChanged(); } } [ DefaultValue(true), ] public virtual bool CausesValidation { get { object o = ViewState["CausesValidation"]; if (o != null) { return (bool)o; } return true; } set { ViewState["CausesValidation"] = value; OnColumnChanged(); } } ///Indicates the text to display for the ///command button /// in the column. /// [ Localizable(true), DefaultValue("") ] public virtual string EditText { get { object o = ViewState["EditText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["EditText"] = value; OnColumnChanged(); } } ///Indicates the text to display for the ///command button in /// the column. /// [ Localizable(true), DefaultValue("") ] public virtual string UpdateText { get { object o = ViewState["UpdateText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["UpdateText"] = value; OnColumnChanged(); } } [ DefaultValue(""), ] public virtual string ValidationGroup { get { object o = ViewState["ValidationGroup"]; if (o != null) { return (string)o; } return String.Empty; } set { ViewState["ValidationGroup"] = value; OnColumnChanged(); } } private void AddButtonToCell(TableCell cell, string commandName, string buttonText, bool causesValidation, string validationGroup) { WebControl buttonControl = null; ControlCollection controls = cell.Controls; ButtonColumnType buttonType = ButtonType; if (buttonType == ButtonColumnType.LinkButton) { LinkButton button = new DataGridLinkButton(); buttonControl = button; button.CommandName = commandName; button.Text = buttonText; button.CausesValidation = causesValidation; button.ValidationGroup = validationGroup; } else { Button button = new Button(); buttonControl = button; button.CommandName = commandName; button.Text = buttonText; button.CausesValidation = causesValidation; button.ValidationGroup = validationGroup; } controls.Add(buttonControl); } ///Indicates the text to display for the ///command button /// in the column. /// public override void InitializeCell(TableCell cell, int columnIndex, ListItemType itemType) { base.InitializeCell(cell, columnIndex, itemType); bool causesValidation = CausesValidation; if ((itemType != ListItemType.Header) && (itemType != ListItemType.Footer)) { if (itemType == ListItemType.EditItem) { ControlCollection controls = cell.Controls; AddButtonToCell(cell, DataGrid.UpdateCommandName, UpdateText, causesValidation, ValidationGroup); LiteralControl spaceControl = new LiteralControl(" "); controls.Add(spaceControl); AddButtonToCell(cell, DataGrid.CancelCommandName, CancelText, false, String.Empty); } else { AddButtonToCell(cell, DataGrid.EditCommandName, EditText, false, String.Empty); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Initializes a cell within the column. ///
Link Menu
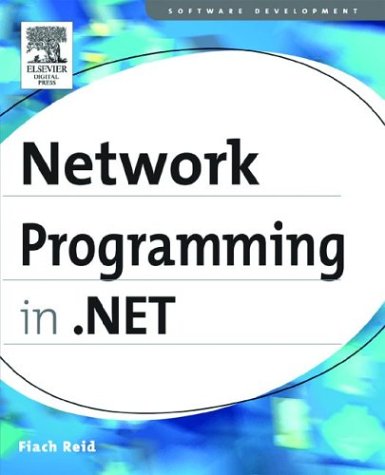
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- X509ServiceCertificateAuthenticationElement.cs
- WebPartEditVerb.cs
- ViewStateAttachedPropertyFeature.cs
- PrinterResolution.cs
- GridEntryCollection.cs
- TimeSpanMinutesOrInfiniteConverter.cs
- TypeInfo.cs
- MonthCalendar.cs
- ModuleConfigurationInfo.cs
- CookieProtection.cs
- SecurityHelper.cs
- QueryContinueDragEventArgs.cs
- Condition.cs
- QueryOperationResponseOfT.cs
- Constants.cs
- ThemeDirectoryCompiler.cs
- Int32CollectionConverter.cs
- AspCompat.cs
- LOSFormatter.cs
- ConstNode.cs
- XPathParser.cs
- DataReceivedEventArgs.cs
- SqlStatistics.cs
- DataControlCommands.cs
- WrapPanel.cs
- LogicalExpr.cs
- ColorAnimationUsingKeyFrames.cs
- SqlPersistenceWorkflowInstanceDescription.cs
- Contracts.cs
- ByteAnimationBase.cs
- HtmlLink.cs
- SqlDataSourceFilteringEventArgs.cs
- TouchEventArgs.cs
- XmlMemberMapping.cs
- WebMessageFormatHelper.cs
- WebPageTraceListener.cs
- AxisAngleRotation3D.cs
- EntitySet.cs
- MethodExpression.cs
- AssemblyBuilderData.cs
- XmlChildNodes.cs
- QilTypeChecker.cs
- EventlogProvider.cs
- DataTableMappingCollection.cs
- NamespaceExpr.cs
- WriterOutput.cs
- HotCommands.cs
- TextEditorMouse.cs
- DataGridViewComboBoxColumn.cs
- PathFigure.cs
- XmlnsDefinitionAttribute.cs
- StaticSiteMapProvider.cs
- AttributeProviderAttribute.cs
- XsltContext.cs
- StaticSiteMapProvider.cs
- SettingsPropertyIsReadOnlyException.cs
- SynthesizerStateChangedEventArgs.cs
- ModelItemDictionaryImpl.cs
- DispatchChannelSink.cs
- WebPartConnectionsCancelVerb.cs
- ToolStripLabel.cs
- ReturnEventArgs.cs
- Line.cs
- HtmlLink.cs
- Matrix3D.cs
- ClassDataContract.cs
- Attributes.cs
- ColorIndependentAnimationStorage.cs
- FontFamilyIdentifier.cs
- SqlBulkCopyColumnMappingCollection.cs
- SqlCacheDependencyDatabaseCollection.cs
- DocumentApplicationJournalEntry.cs
- PromptBuilder.cs
- COM2ColorConverter.cs
- ToolStripTextBox.cs
- CodeGenerator.cs
- NullableDecimalSumAggregationOperator.cs
- MeshGeometry3D.cs
- FontCollection.cs
- FieldReference.cs
- LoadRetryConstantStrategy.cs
- CheckBoxRenderer.cs
- MapPathBasedVirtualPathProvider.cs
- AbandonedMutexException.cs
- FixedPage.cs
- CustomValidator.cs
- ActivationArguments.cs
- MediaScriptCommandRoutedEventArgs.cs
- HttpServerUtilityBase.cs
- AggregateNode.cs
- TableSectionStyle.cs
- cookiecontainer.cs
- UserPreferenceChangingEventArgs.cs
- SafeUserTokenHandle.cs
- ResourceExpression.cs
- KeyMatchBuilder.cs
- NameTable.cs
- XmlHierarchicalEnumerable.cs
- HostedTcpTransportManager.cs
- ContentFileHelper.cs