Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Data / System / Data / Filter / FilterException.cs / 1 / FilterException.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data { using System; using System.Diagnostics; using System.Globalization; using System.Runtime.Serialization; ////// [Serializable] #if WINFSInternalOnly internal #else public #endif class InvalidExpressionException : DataException { protected InvalidExpressionException(SerializationInfo info, StreamingContext context) : base(info, context) { } ///[To be supplied.] ////// public InvalidExpressionException() : base() {} ///[To be supplied.] ////// public InvalidExpressionException(string s) : base(s) {} public InvalidExpressionException(string message, Exception innerException) : base(message, innerException) {} } ///[To be supplied.] ////// [Serializable] #if WINFSInternalOnly internal #else public #endif class EvaluateException : InvalidExpressionException { protected EvaluateException(SerializationInfo info, StreamingContext context) : base(info, context) { } ///[To be supplied.] ////// public EvaluateException() : base() {} ///[To be supplied.] ////// public EvaluateException(string s) : base(s) {} public EvaluateException(string message, Exception innerException) : base(message, innerException) {} } ///[To be supplied.] ////// [Serializable] #if WINFSInternalOnly internal #else public #endif class SyntaxErrorException : InvalidExpressionException { protected SyntaxErrorException(SerializationInfo info, StreamingContext context) : base(info, context) { } ///[To be supplied.] ////// public SyntaxErrorException() : base() {} ///[To be supplied.] ////// public SyntaxErrorException(string s) : base(s) {} public SyntaxErrorException(string message, Exception innerException) : base(message, innerException) {} } internal sealed class ExprException { private ExprException() { /* prevent utility class from being insantiated*/ } static private OverflowException _Overflow(string error) { OverflowException e = new OverflowException(error); ExceptionBuilder.TraceExceptionAsReturnValue(e); return e; } static private InvalidExpressionException _Expr(string error) { InvalidExpressionException e = new InvalidExpressionException(error); ExceptionBuilder.TraceExceptionAsReturnValue(e); return e; } static private SyntaxErrorException _Syntax(string error) { SyntaxErrorException e = new SyntaxErrorException(error); ExceptionBuilder.TraceExceptionAsReturnValue(e); return e; } static private EvaluateException _Eval(string error) { EvaluateException e = new EvaluateException(error); ExceptionBuilder.TraceExceptionAsReturnValue(e); return e; } static private EvaluateException _Eval(string error, Exception innerException) { EvaluateException e = new EvaluateException(error/*, innerException*/); // ExceptionBuilder.TraceExceptionAsReturnValue(e); return e; } static public Exception InvokeArgument() { return ExceptionBuilder._Argument(Res.GetString(Res.Expr_InvokeArgument)); } static public Exception NYI(string moreinfo) { string err = Res.GetString(Res.Expr_NYI, moreinfo); Debug.Fail(err); return _Expr(err); } static public Exception MissingOperand(OperatorInfo before) { return _Syntax(Res.GetString(Res.Expr_MissingOperand, Operators.ToString(before.op))); } static public Exception MissingOperator(string token) { return _Syntax(Res.GetString(Res.Expr_MissingOperand, token)); } static public Exception TypeMismatch(string expr) { return _Eval(Res.GetString(Res.Expr_TypeMismatch, expr)); } static public Exception FunctionArgumentOutOfRange(string arg, string func) { return ExceptionBuilder._ArgumentOutOfRange(arg, Res.GetString(Res.Expr_ArgumentOutofRange, func)); } static public Exception ExpressionTooComplex() { return _Eval(Res.GetString(Res.Expr_ExpressionTooComplex)); } static public Exception UnboundName(string name) { return _Eval(Res.GetString(Res.Expr_UnboundName, name)); } static public Exception InvalidString(string str) { return _Syntax(Res.GetString(Res.Expr_InvalidString, str)); } static public Exception UndefinedFunction(string name) { return _Eval(Res.GetString(Res.Expr_UndefinedFunction, name)); } static public Exception SyntaxError() { return _Syntax(Res.GetString(Res.Expr_Syntax)); } static public Exception FunctionArgumentCount(string name) { return _Eval(Res.GetString(Res.Expr_FunctionArgumentCount, name)); } static public Exception MissingRightParen() { return _Syntax(Res.GetString(Res.Expr_MissingRightParen)); } static public Exception UnknownToken(string token, int position) { return _Syntax(Res.GetString(Res.Expr_UnknownToken, token, position.ToString(CultureInfo.InvariantCulture))); } static public Exception UnknownToken(Tokens tokExpected, Tokens tokCurr, int position) { return _Syntax(Res.GetString(Res.Expr_UnknownToken1, tokExpected.ToString(), tokCurr.ToString(), position.ToString(CultureInfo.InvariantCulture))); } static public Exception DatatypeConvertion(Type type1, Type type2) { return _Eval(Res.GetString(Res.Expr_DatatypeConvertion, type1.ToString(), type2.ToString())); } static public Exception DatavalueConvertion(object value, Type type, Exception innerException) { return _Eval(Res.GetString(Res.Expr_DatavalueConvertion, value.ToString(), type.ToString()), innerException); } static public Exception InvalidName(string name) { return _Syntax(Res.GetString(Res.Expr_InvalidName, name)); } static public Exception InvalidDate(string date) { return _Syntax(Res.GetString(Res.Expr_InvalidDate, date)); } static public Exception NonConstantArgument() { return _Eval(Res.GetString(Res.Expr_NonConstantArgument)); } static public Exception InvalidPattern(string pat) { return _Eval(Res.GetString(Res.Expr_InvalidPattern, pat)); } static public Exception InWithoutParentheses() { return _Syntax(Res.GetString(Res.Expr_InWithoutParentheses)); } static public Exception InWithoutList() { return _Syntax(Res.GetString(Res.Expr_InWithoutList)); } static public Exception InvalidIsSyntax() { return _Syntax(Res.GetString(Res.Expr_IsSyntax)); } static public Exception Overflow(Type type) { return _Overflow(Res.GetString(Res.Expr_Overflow, type.Name)); } static public Exception ArgumentType(string function, int arg, Type type) { return _Eval(Res.GetString(Res.Expr_ArgumentType, function, arg.ToString(CultureInfo.InvariantCulture), type.ToString())); } static public Exception ArgumentTypeInteger(string function, int arg) { return _Eval(Res.GetString(Res.Expr_ArgumentTypeInteger, function, arg.ToString(CultureInfo.InvariantCulture))); } static public Exception TypeMismatchInBinop(int op, Type type1, Type type2) { return _Eval(Res.GetString(Res.Expr_TypeMismatchInBinop, Operators.ToString(op), type1.ToString(), type2.ToString())); } static public Exception AmbiguousBinop(int op, Type type1, Type type2) { return _Eval(Res.GetString(Res.Expr_AmbiguousBinop, Operators.ToString(op), type1.ToString(), type2.ToString())); } static public Exception UnsupportedOperator(int op) { return _Eval(Res.GetString(Res.Expr_UnsupportedOperator, Operators.ToString(op))); } static public Exception InvalidNameBracketing(string name) { return _Syntax(Res.GetString(Res.Expr_InvalidNameBracketing, name)); } static public Exception MissingOperandBefore(string op) { return _Syntax(Res.GetString(Res.Expr_MissingOperandBefore, op)); } static public Exception TooManyRightParentheses() { return _Syntax(Res.GetString(Res.Expr_TooManyRightParentheses)); } static public Exception UnresolvedRelation(string name, string expr) { return _Eval(Res.GetString(Res.Expr_UnresolvedRelation, name, expr)); } static internal EvaluateException BindFailure(string relationName) { return _Eval(Res.GetString(Res.Expr_BindFailure, relationName)); } static public Exception AggregateArgument() { return _Syntax(Res.GetString(Res.Expr_AggregateArgument)); } static public Exception AggregateUnbound(string expr) { return _Eval(Res.GetString(Res.Expr_AggregateUnbound, expr)); } static public Exception EvalNoContext() { return _Eval(Res.GetString(Res.Expr_EvalNoContext)); } static public Exception ExpressionUnbound(string expr) { return _Eval(Res.GetString(Res.Expr_ExpressionUnbound, expr)); } static public Exception ComputeNotAggregate(string expr) { return _Eval(Res.GetString(Res.Expr_ComputeNotAggregate, expr)); } static public Exception FilterConvertion(string expr) { return _Eval(Res.GetString(Res.Expr_FilterConvertion, expr)); } static public Exception LookupArgument() { return _Syntax(Res.GetString(Res.Expr_LookupArgument)); } static public Exception InvalidType(string typeName) { return _Eval(Res.GetString(Res.Expr_InvalidType, typeName)); } static public Exception InvalidHoursArgument() { return _Eval(Res.GetString(Res.Expr_InvalidHoursArgument)); } static public Exception InvalidMinutesArgument() { return _Eval(Res.GetString(Res.Expr_InvalidMinutesArgument)); } static public Exception InvalidTimeZoneRange() { return _Eval(Res.GetString(Res.Expr_InvalidTimeZoneRange)); } static public Exception MismatchKindandTimeSpan() { return _Eval(Res.GetString(Res.Expr_MismatchKindandTimeSpan)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data { using System; using System.Diagnostics; using System.Globalization; using System.Runtime.Serialization; ////// [Serializable] #if WINFSInternalOnly internal #else public #endif class InvalidExpressionException : DataException { protected InvalidExpressionException(SerializationInfo info, StreamingContext context) : base(info, context) { } ///[To be supplied.] ////// public InvalidExpressionException() : base() {} ///[To be supplied.] ////// public InvalidExpressionException(string s) : base(s) {} public InvalidExpressionException(string message, Exception innerException) : base(message, innerException) {} } ///[To be supplied.] ////// [Serializable] #if WINFSInternalOnly internal #else public #endif class EvaluateException : InvalidExpressionException { protected EvaluateException(SerializationInfo info, StreamingContext context) : base(info, context) { } ///[To be supplied.] ////// public EvaluateException() : base() {} ///[To be supplied.] ////// public EvaluateException(string s) : base(s) {} public EvaluateException(string message, Exception innerException) : base(message, innerException) {} } ///[To be supplied.] ////// [Serializable] #if WINFSInternalOnly internal #else public #endif class SyntaxErrorException : InvalidExpressionException { protected SyntaxErrorException(SerializationInfo info, StreamingContext context) : base(info, context) { } ///[To be supplied.] ////// public SyntaxErrorException() : base() {} ///[To be supplied.] ////// public SyntaxErrorException(string s) : base(s) {} public SyntaxErrorException(string message, Exception innerException) : base(message, innerException) {} } internal sealed class ExprException { private ExprException() { /* prevent utility class from being insantiated*/ } static private OverflowException _Overflow(string error) { OverflowException e = new OverflowException(error); ExceptionBuilder.TraceExceptionAsReturnValue(e); return e; } static private InvalidExpressionException _Expr(string error) { InvalidExpressionException e = new InvalidExpressionException(error); ExceptionBuilder.TraceExceptionAsReturnValue(e); return e; } static private SyntaxErrorException _Syntax(string error) { SyntaxErrorException e = new SyntaxErrorException(error); ExceptionBuilder.TraceExceptionAsReturnValue(e); return e; } static private EvaluateException _Eval(string error) { EvaluateException e = new EvaluateException(error); ExceptionBuilder.TraceExceptionAsReturnValue(e); return e; } static private EvaluateException _Eval(string error, Exception innerException) { EvaluateException e = new EvaluateException(error/*, innerException*/); // ExceptionBuilder.TraceExceptionAsReturnValue(e); return e; } static public Exception InvokeArgument() { return ExceptionBuilder._Argument(Res.GetString(Res.Expr_InvokeArgument)); } static public Exception NYI(string moreinfo) { string err = Res.GetString(Res.Expr_NYI, moreinfo); Debug.Fail(err); return _Expr(err); } static public Exception MissingOperand(OperatorInfo before) { return _Syntax(Res.GetString(Res.Expr_MissingOperand, Operators.ToString(before.op))); } static public Exception MissingOperator(string token) { return _Syntax(Res.GetString(Res.Expr_MissingOperand, token)); } static public Exception TypeMismatch(string expr) { return _Eval(Res.GetString(Res.Expr_TypeMismatch, expr)); } static public Exception FunctionArgumentOutOfRange(string arg, string func) { return ExceptionBuilder._ArgumentOutOfRange(arg, Res.GetString(Res.Expr_ArgumentOutofRange, func)); } static public Exception ExpressionTooComplex() { return _Eval(Res.GetString(Res.Expr_ExpressionTooComplex)); } static public Exception UnboundName(string name) { return _Eval(Res.GetString(Res.Expr_UnboundName, name)); } static public Exception InvalidString(string str) { return _Syntax(Res.GetString(Res.Expr_InvalidString, str)); } static public Exception UndefinedFunction(string name) { return _Eval(Res.GetString(Res.Expr_UndefinedFunction, name)); } static public Exception SyntaxError() { return _Syntax(Res.GetString(Res.Expr_Syntax)); } static public Exception FunctionArgumentCount(string name) { return _Eval(Res.GetString(Res.Expr_FunctionArgumentCount, name)); } static public Exception MissingRightParen() { return _Syntax(Res.GetString(Res.Expr_MissingRightParen)); } static public Exception UnknownToken(string token, int position) { return _Syntax(Res.GetString(Res.Expr_UnknownToken, token, position.ToString(CultureInfo.InvariantCulture))); } static public Exception UnknownToken(Tokens tokExpected, Tokens tokCurr, int position) { return _Syntax(Res.GetString(Res.Expr_UnknownToken1, tokExpected.ToString(), tokCurr.ToString(), position.ToString(CultureInfo.InvariantCulture))); } static public Exception DatatypeConvertion(Type type1, Type type2) { return _Eval(Res.GetString(Res.Expr_DatatypeConvertion, type1.ToString(), type2.ToString())); } static public Exception DatavalueConvertion(object value, Type type, Exception innerException) { return _Eval(Res.GetString(Res.Expr_DatavalueConvertion, value.ToString(), type.ToString()), innerException); } static public Exception InvalidName(string name) { return _Syntax(Res.GetString(Res.Expr_InvalidName, name)); } static public Exception InvalidDate(string date) { return _Syntax(Res.GetString(Res.Expr_InvalidDate, date)); } static public Exception NonConstantArgument() { return _Eval(Res.GetString(Res.Expr_NonConstantArgument)); } static public Exception InvalidPattern(string pat) { return _Eval(Res.GetString(Res.Expr_InvalidPattern, pat)); } static public Exception InWithoutParentheses() { return _Syntax(Res.GetString(Res.Expr_InWithoutParentheses)); } static public Exception InWithoutList() { return _Syntax(Res.GetString(Res.Expr_InWithoutList)); } static public Exception InvalidIsSyntax() { return _Syntax(Res.GetString(Res.Expr_IsSyntax)); } static public Exception Overflow(Type type) { return _Overflow(Res.GetString(Res.Expr_Overflow, type.Name)); } static public Exception ArgumentType(string function, int arg, Type type) { return _Eval(Res.GetString(Res.Expr_ArgumentType, function, arg.ToString(CultureInfo.InvariantCulture), type.ToString())); } static public Exception ArgumentTypeInteger(string function, int arg) { return _Eval(Res.GetString(Res.Expr_ArgumentTypeInteger, function, arg.ToString(CultureInfo.InvariantCulture))); } static public Exception TypeMismatchInBinop(int op, Type type1, Type type2) { return _Eval(Res.GetString(Res.Expr_TypeMismatchInBinop, Operators.ToString(op), type1.ToString(), type2.ToString())); } static public Exception AmbiguousBinop(int op, Type type1, Type type2) { return _Eval(Res.GetString(Res.Expr_AmbiguousBinop, Operators.ToString(op), type1.ToString(), type2.ToString())); } static public Exception UnsupportedOperator(int op) { return _Eval(Res.GetString(Res.Expr_UnsupportedOperator, Operators.ToString(op))); } static public Exception InvalidNameBracketing(string name) { return _Syntax(Res.GetString(Res.Expr_InvalidNameBracketing, name)); } static public Exception MissingOperandBefore(string op) { return _Syntax(Res.GetString(Res.Expr_MissingOperandBefore, op)); } static public Exception TooManyRightParentheses() { return _Syntax(Res.GetString(Res.Expr_TooManyRightParentheses)); } static public Exception UnresolvedRelation(string name, string expr) { return _Eval(Res.GetString(Res.Expr_UnresolvedRelation, name, expr)); } static internal EvaluateException BindFailure(string relationName) { return _Eval(Res.GetString(Res.Expr_BindFailure, relationName)); } static public Exception AggregateArgument() { return _Syntax(Res.GetString(Res.Expr_AggregateArgument)); } static public Exception AggregateUnbound(string expr) { return _Eval(Res.GetString(Res.Expr_AggregateUnbound, expr)); } static public Exception EvalNoContext() { return _Eval(Res.GetString(Res.Expr_EvalNoContext)); } static public Exception ExpressionUnbound(string expr) { return _Eval(Res.GetString(Res.Expr_ExpressionUnbound, expr)); } static public Exception ComputeNotAggregate(string expr) { return _Eval(Res.GetString(Res.Expr_ComputeNotAggregate, expr)); } static public Exception FilterConvertion(string expr) { return _Eval(Res.GetString(Res.Expr_FilterConvertion, expr)); } static public Exception LookupArgument() { return _Syntax(Res.GetString(Res.Expr_LookupArgument)); } static public Exception InvalidType(string typeName) { return _Eval(Res.GetString(Res.Expr_InvalidType, typeName)); } static public Exception InvalidHoursArgument() { return _Eval(Res.GetString(Res.Expr_InvalidHoursArgument)); } static public Exception InvalidMinutesArgument() { return _Eval(Res.GetString(Res.Expr_InvalidMinutesArgument)); } static public Exception InvalidTimeZoneRange() { return _Eval(Res.GetString(Res.Expr_InvalidTimeZoneRange)); } static public Exception MismatchKindandTimeSpan() { return _Eval(Res.GetString(Res.Expr_MismatchKindandTimeSpan)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
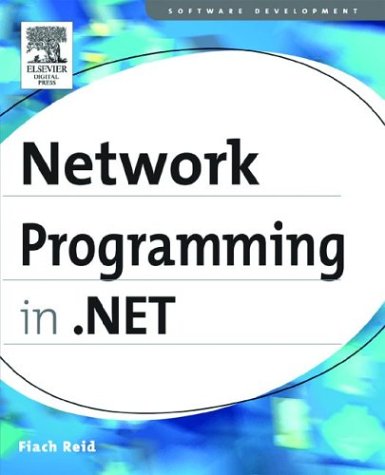
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MultiBindingExpression.cs
- SQLInt64Storage.cs
- XmlDictionaryString.cs
- HttpModuleAction.cs
- TaiwanCalendar.cs
- ExpandButtonVisibilityConverter.cs
- SevenBitStream.cs
- ResourcesChangeInfo.cs
- BinaryWriter.cs
- HtmlMeta.cs
- InvalidFilterCriteriaException.cs
- OptimizerPatterns.cs
- TransformationRules.cs
- WsdlExporter.cs
- SourceInterpreter.cs
- ExtensionSimplifierMarkupObject.cs
- DecoratedNameAttribute.cs
- Types.cs
- AttributeUsageAttribute.cs
- ExpressionParser.cs
- MimeBasePart.cs
- ServiceThrottlingElement.cs
- WorkflowApplicationIdleEventArgs.cs
- mansign.cs
- CompilationSection.cs
- ObjectConverter.cs
- BamlLocalizableResource.cs
- BrowserCapabilitiesCodeGenerator.cs
- HttpProfileGroupBase.cs
- ProtectedConfiguration.cs
- _BasicClient.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- EmbeddedMailObject.cs
- PropertyInformationCollection.cs
- HttpApplication.cs
- EventManager.cs
- Socket.cs
- HelloMessageCD1.cs
- CollectionAdapters.cs
- EntitySqlException.cs
- PassportAuthenticationEventArgs.cs
- PropertyStore.cs
- JsonDeserializer.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- ReliabilityContractAttribute.cs
- mactripleDES.cs
- BuildProvider.cs
- ConstructorNeedsTagAttribute.cs
- SqlClientFactory.cs
- ClientSponsor.cs
- NullableIntAverageAggregationOperator.cs
- HttpRequestBase.cs
- BigIntegerStorage.cs
- KeyValuePair.cs
- TagMapCollection.cs
- DecimalKeyFrameCollection.cs
- AlignmentYValidation.cs
- PrinterUnitConvert.cs
- MemberPath.cs
- BinaryCommonClasses.cs
- _SingleItemRequestCache.cs
- Deserializer.cs
- ObjectStorage.cs
- DataGridTable.cs
- FontNamesConverter.cs
- InstanceKeyCompleteException.cs
- ConfigXmlElement.cs
- UserControlBuildProvider.cs
- WebPartZone.cs
- DataGridViewCellEventArgs.cs
- XmlAttributes.cs
- PeerNode.cs
- ChildChangedEventArgs.cs
- _ListenerAsyncResult.cs
- TTSEngineProxy.cs
- DateTimeFormatInfo.cs
- DynamicUpdateCommand.cs
- SQLUtility.cs
- SqlCaseSimplifier.cs
- SmtpException.cs
- ExpressionPrefixAttribute.cs
- HebrewNumber.cs
- ForeignConstraint.cs
- PrintingPermission.cs
- MultiView.cs
- controlskin.cs
- CounterSetInstance.cs
- Binding.cs
- X509Chain.cs
- XmlDocument.cs
- Int64Converter.cs
- ListCollectionView.cs
- DataList.cs
- AndCondition.cs
- QilVisitor.cs
- RenameRuleObjectDialog.Designer.cs
- CachedPathData.cs
- SByteStorage.cs
- RefType.cs
- DiagnosticsConfigurationHandler.cs