Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / SystemNet / Net / UnsafeMethods.cs / 2 / UnsafeMethods.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System; using System.Collections.Generic; using System.Text; using Microsoft.Win32.SafeHandles; using System.Security; using System.Runtime.InteropServices; using System.Runtime.ConstrainedExecution; using System.Threading; using System.Net.Sockets; [Flags] internal enum FormatMessageFlags : uint { FORMAT_MESSAGE_ALLOCATE_BUFFER = 0x00000100, //FORMAT_MESSAGE_IGNORE_INSERTS = 0x00000200, //FORMAT_MESSAGE_FROM_STRING = 0x00000400, FORMAT_MESSAGE_FROM_HMODULE = 0x00000800, FORMAT_MESSAGE_FROM_SYSTEM = 0x00001000, FORMAT_MESSAGE_ARGUMENT_ARRAY = 0x00002000 } [System.Security.SuppressUnmanagedCodeSecurityAttribute()] internal static class UnsafeSystemNativeMethods { private const string KERNEL32 = "KERNEL32.dll"; [System.Security.SecurityCritical] [DllImport(KERNEL32, ExactSpelling = true, CharSet = CharSet.Unicode, SetLastError = true)] internal static extern unsafe SafeLoadLibrary LoadLibraryExW( string lpwLibFileName, [In] void* hFile, uint dwFlags); [DllImport(KERNEL32, ExactSpelling = true, SetLastError = true)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [return: MarshalAs(UnmanagedType.Bool)] internal static extern unsafe bool FreeLibrary(IntPtr hModule); [System.Security.SecurityCritical] [DllImport(KERNEL32, EntryPoint = "GetProcAddress", SetLastError = true, BestFitMapping = false)] internal extern static IntPtr GetProcAddress(SafeLoadLibrary hModule, string entryPoint); [DllImport(KERNEL32, CharSet = CharSet.Unicode)] internal extern static uint FormatMessage( FormatMessageFlags dwFlags, IntPtr lpSource, UInt32 dwMessageId, UInt32 dwLanguageId, ref IntPtr lpBuffer, UInt32 nSize, IntPtr vaArguments ); [DllImport(KERNEL32, CharSet = CharSet.Unicode)] internal extern static uint LocalFree(IntPtr lpMem); } //// [System.Security.SecurityCritical(System.Security.SecurityCriticalScope.Everything)] [System.Security.SuppressUnmanagedCodeSecurityAttribute()] internal sealed class SafeLoadLibrary : SafeHandleZeroOrMinusOneIsInvalid { private const string KERNEL32 = "kernel32.dll"; private SafeLoadLibrary() : base(true) { } //private SafeLoadLibrary(bool ownsHandle) : base(ownsHandle) { } //internal static readonly SafeLoadLibrary Zero = new SafeLoadLibrary(false); internal unsafe static SafeLoadLibrary LoadLibraryEx(string library) { SafeLoadLibrary result = UnsafeSystemNativeMethods.LoadLibraryExW(library, null, 0); if (result.IsInvalid) { //NOTE: //IsInvalid tests the numeric value of the handle. //SetHandleAsInvalid sets the handle as closed, so that further closing //does not have to take place in the critical finalizer thread. // //You would think that when you assign 0 or -1 to an instance of //SafeHandleZeroOrMinusoneIsInvalid, the handle will not be closed, since after all it is invalid //It turns out that the SafeHandleZetoOrMinusOneIsInvalid overrides only the IsInvalid() method //It does not do anything to automatically close it. //So we have to SetHandleAsInvalid --> Which means mark it closed -- so that //we will not eventually call CloseHandle on 0 or -1 result.SetHandleAsInvalid(); } return result; } protected override bool ReleaseHandle() { return UnsafeSystemNativeMethods.FreeLibrary(handle); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System; using System.Collections.Generic; using System.Text; using Microsoft.Win32.SafeHandles; using System.Security; using System.Runtime.InteropServices; using System.Runtime.ConstrainedExecution; using System.Threading; using System.Net.Sockets; [Flags] internal enum FormatMessageFlags : uint { FORMAT_MESSAGE_ALLOCATE_BUFFER = 0x00000100, //FORMAT_MESSAGE_IGNORE_INSERTS = 0x00000200, //FORMAT_MESSAGE_FROM_STRING = 0x00000400, FORMAT_MESSAGE_FROM_HMODULE = 0x00000800, FORMAT_MESSAGE_FROM_SYSTEM = 0x00001000, FORMAT_MESSAGE_ARGUMENT_ARRAY = 0x00002000 } [System.Security.SuppressUnmanagedCodeSecurityAttribute()] internal static class UnsafeSystemNativeMethods { private const string KERNEL32 = "KERNEL32.dll"; [System.Security.SecurityCritical] [DllImport(KERNEL32, ExactSpelling = true, CharSet = CharSet.Unicode, SetLastError = true)] internal static extern unsafe SafeLoadLibrary LoadLibraryExW( string lpwLibFileName, [In] void* hFile, uint dwFlags); [DllImport(KERNEL32, ExactSpelling = true, SetLastError = true)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [return: MarshalAs(UnmanagedType.Bool)] internal static extern unsafe bool FreeLibrary(IntPtr hModule); [System.Security.SecurityCritical] [DllImport(KERNEL32, EntryPoint = "GetProcAddress", SetLastError = true, BestFitMapping = false)] internal extern static IntPtr GetProcAddress(SafeLoadLibrary hModule, string entryPoint); [DllImport(KERNEL32, CharSet = CharSet.Unicode)] internal extern static uint FormatMessage( FormatMessageFlags dwFlags, IntPtr lpSource, UInt32 dwMessageId, UInt32 dwLanguageId, ref IntPtr lpBuffer, UInt32 nSize, IntPtr vaArguments ); [DllImport(KERNEL32, CharSet = CharSet.Unicode)] internal extern static uint LocalFree(IntPtr lpMem); } //// [System.Security.SecurityCritical(System.Security.SecurityCriticalScope.Everything)] [System.Security.SuppressUnmanagedCodeSecurityAttribute()] internal sealed class SafeLoadLibrary : SafeHandleZeroOrMinusOneIsInvalid { private const string KERNEL32 = "kernel32.dll"; private SafeLoadLibrary() : base(true) { } //private SafeLoadLibrary(bool ownsHandle) : base(ownsHandle) { } //internal static readonly SafeLoadLibrary Zero = new SafeLoadLibrary(false); internal unsafe static SafeLoadLibrary LoadLibraryEx(string library) { SafeLoadLibrary result = UnsafeSystemNativeMethods.LoadLibraryExW(library, null, 0); if (result.IsInvalid) { //NOTE: //IsInvalid tests the numeric value of the handle. //SetHandleAsInvalid sets the handle as closed, so that further closing //does not have to take place in the critical finalizer thread. // //You would think that when you assign 0 or -1 to an instance of //SafeHandleZeroOrMinusoneIsInvalid, the handle will not be closed, since after all it is invalid //It turns out that the SafeHandleZetoOrMinusOneIsInvalid overrides only the IsInvalid() method //It does not do anything to automatically close it. //So we have to SetHandleAsInvalid --> Which means mark it closed -- so that //we will not eventually call CloseHandle on 0 or -1 result.SetHandleAsInvalid(); } return result; } protected override bool ReleaseHandle() { return UnsafeSystemNativeMethods.FreeLibrary(handle); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.//
Link Menu
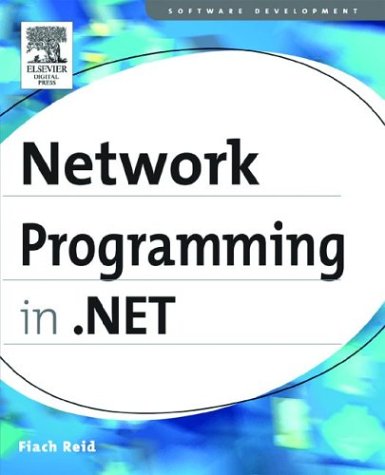
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MouseActionValueSerializer.cs
- BeginStoryboard.cs
- ParameterCollectionEditor.cs
- SubclassTypeValidatorAttribute.cs
- StateWorkerRequest.cs
- MemberPath.cs
- ConfigurationElement.cs
- MappedMetaModel.cs
- ConfigurationValues.cs
- Margins.cs
- AutomationPatternInfo.cs
- DomNameTable.cs
- KnownTypes.cs
- ObjectDataSourceEventArgs.cs
- EncryptedData.cs
- DefaultExpressionVisitor.cs
- BuildProvider.cs
- HttpRuntimeSection.cs
- ApplicationServiceHelper.cs
- PropertyManager.cs
- UnmanagedMarshal.cs
- PresentationAppDomainManager.cs
- DispatcherSynchronizationContext.cs
- EdgeModeValidation.cs
- UnsafeNativeMethods.cs
- XhtmlBasicFormAdapter.cs
- Serializer.cs
- HostingEnvironmentSection.cs
- WebPartCancelEventArgs.cs
- SiteMapSection.cs
- WSDualHttpSecurity.cs
- BitmapMetadataEnumerator.cs
- CompModSwitches.cs
- WindowInteropHelper.cs
- TracedNativeMethods.cs
- Serializer.cs
- UrlMappingsSection.cs
- NameGenerator.cs
- ReflectionServiceProvider.cs
- PrintDialogException.cs
- BaseDataBoundControl.cs
- StackOverflowException.cs
- FixedBufferAttribute.cs
- XmlUTF8TextReader.cs
- XPathMultyIterator.cs
- UInt64.cs
- EntityDataSourceSelectedEventArgs.cs
- Transform3D.cs
- InfoCardClaim.cs
- ParsedAttributeCollection.cs
- HtmlTableRow.cs
- DispatcherHookEventArgs.cs
- CachedRequestParams.cs
- TreeNode.cs
- ConsumerConnectionPointCollection.cs
- SqlCacheDependencySection.cs
- HttpRuntime.cs
- OutputCacheProfile.cs
- MetadataExporter.cs
- WebPageTraceListener.cs
- SessionPageStatePersister.cs
- ExpressionBuilderCollection.cs
- FloaterParaClient.cs
- ReferenceTypeElement.cs
- BuildResultCache.cs
- XmlNamedNodeMap.cs
- MediaContext.cs
- EditBehavior.cs
- WebControlsSection.cs
- DataServiceConfiguration.cs
- COMException.cs
- WmlValidatorAdapter.cs
- BasicCellRelation.cs
- DataGridCaption.cs
- NumericUpDownAcceleration.cs
- KeyedCollection.cs
- ViewGenerator.cs
- DefaultParameterValueAttribute.cs
- _CommandStream.cs
- _AutoWebProxyScriptHelper.cs
- M3DUtil.cs
- ConfigurationManagerInternal.cs
- DatatypeImplementation.cs
- ReadOnlyHierarchicalDataSource.cs
- streamingZipPartStream.cs
- LeftCellWrapper.cs
- SignatureDescription.cs
- RepeatInfo.cs
- WorkflowItemPresenter.cs
- PropertyStore.cs
- SafeSecurityHandles.cs
- ThousandthOfEmRealPoints.cs
- ChannelEndpointElementCollection.cs
- QuaternionAnimation.cs
- AppDomainShutdownMonitor.cs
- RpcAsyncResult.cs
- VariableElement.cs
- SoapRpcServiceAttribute.cs
- LinkUtilities.cs
- X509ThumbprintKeyIdentifierClause.cs