Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / MS / Internal / AppModel / PresentationAppDomainManager.cs / 1 / PresentationAppDomainManager.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Implements a custom AppDomainManager. // //--------------------------------------------------------------------------- using System; using System.Net; using System.Reflection; using System.Runtime.Remoting; using System.Security; using System.Security.Permissions; using System.Security.Policy; using System.Runtime.Hosting; using System.Text; using MS.Win32; using MS.Internal; using MS.Internal.AppModel; using MS.Internal.Utility; using MS.Utility; namespace System.Windows.Interop { internal class PresentationHostSecurityManager : HostSecurityManager { ////// Critical - HostSecurityManager..ctor LinkDemand's. This class should not be called directly by PT'ed callers, since it can interfere with /// the privileges an AppDomain gets. /// [SecurityCritical] internal PresentationHostSecurityManager() { } ////// Critical: /// 1) accesses critical data AppDomain.CurrentDomain.SetupInformation.ActivationArguments /// and sets BrowserInteropHelper.IsBrowserHosted. /// 2) calls the critical AddPermissionForUri(). /// Safe: /// 1) does not expose critical data; BrowserInteropHelper.IsBrowserHosted is set to true, as it /// has to be. /// 2) The additional permission added is to the "fake" site-of-origin used for debugging. /// [SecurityCritical, SecurityTreatAsSafe] public override ApplicationTrust DetermineApplicationTrust(Evidence applicationEvidence, Evidence activatorEvidence, TrustManagerContext context) { if (EventTrace.IsEnabled(EventTrace.Flags.performance, EventTrace.Level.verbose)) { EventTrace.EventProvider.TraceEvent( EventTrace.Level.verbose, EventTrace.GuidFromId(EventTraceGuidId.HOSTINGGUID), (byte)EventTrace.HostingEvent.DetermineApplicationTrustStart); } ApplicationTrust trust; Uri activationUri = GetUriFromActivationData(0); bool isDebug = PresentationAppDomainManager.IsDebug ? true : GetBoolFromActivationData(1); BrowserInteropHelper.SetBrowserHosted(true); if (isDebug) { context.IgnorePersistedDecision = true; context.Persist = false; context.KeepAlive = false; context.NoPrompt = true; trust = base.DetermineApplicationTrust(applicationEvidence, activatorEvidence, context); Uri debugSecurityZoneURL = GetUriFromActivationData(2); if (debugSecurityZoneURL != null) { PermissionSet permissions = trust.DefaultGrantSet.PermissionSet; trust.DefaultGrantSet.PermissionSet = AddPermissionForUri(permissions, debugSecurityZoneURL); } } else { // Defense in depth. Should never get to this point and require this to be // set, but just in case, we do this so that we will never get a trust // prompt even if something goes wrong somewhere else. context.NoPrompt = true; trust = base.DetermineApplicationTrust(applicationEvidence, activatorEvidence, context); } if (EventTrace.IsEnabled(EventTrace.Flags.performance, EventTrace.Level.verbose)) { EventTrace.EventProvider.TraceEvent( EventTrace.Level.verbose, EventTrace.GuidFromId(EventTraceGuidId.HOSTINGGUID), (byte)EventTrace.HostingEvent.DetermineApplicationTrustEnd); } return trust; } ////// Critical - because adds permission to default permissionset granted by trustmanager. /// CAUTION: If srcUri denotes a directory path, it must end with '/'. Otherwise permission will /// be granted to the parent directory. /// [SecurityCritical] internal static PermissionSet AddPermissionForUri(PermissionSet originalPermSet, Uri srcUri) { PermissionSet newPermSet = originalPermSet; if (srcUri != null) { Evidence evidence = new Evidence(); evidence.AddHost(new Url(BindUriHelper.UriToString(srcUri))); // important: the parameter must be a UrL object not a UrI object IMembershipCondition membership = new UrlMembershipCondition(BindUriHelper.UriToString(srcUri)); CodeGroup group = (srcUri.IsFile) ? (CodeGroup)new FileCodeGroup(membership, FileIOPermissionAccess.Read | FileIOPermissionAccess.PathDiscovery) :(CodeGroup)new NetCodeGroup(membership); PolicyStatement policy = group.Resolve(evidence); if (!policy.PermissionSet.IsEmpty()) { newPermSet = originalPermSet.Union(policy.PermissionSet); } } return newPermSet; } ////// Critical - because this accesses critical data AppDomain.CurrentDomain.SetupInformation.ActivationArguments. /// Safe - because this does not expose that string. /// [SecurityCritical, SecurityTreatAsSafe] private bool GetBoolFromActivationData(int index) { bool flag = false; // default if (AppDomain.CurrentDomain.SetupInformation.ActivationArguments != null && AppDomain.CurrentDomain.SetupInformation.ActivationArguments.ActivationData.Length > index) { if (AppDomain.CurrentDomain.SetupInformation.ActivationArguments.ActivationData[index] == true.ToString()) { flag = true; } } return flag; } ////// Critical - because this accesses critical data AppDomain.CurrentDomain.SetupInformation.ActivationArguments. /// Safe - because this does not expose that string. /// [SecurityCritical, SecurityTreatAsSafe] private Uri GetUriFromActivationData(int index) { Uri uri = null; if (AppDomain.CurrentDomain.SetupInformation.ActivationArguments != null && AppDomain.CurrentDomain.SetupInformation.ActivationArguments.ActivationData.Length > index) { if (!string.IsNullOrEmpty(AppDomain.CurrentDomain.SetupInformation.ActivationArguments.ActivationData[index])) { uri = new UriBuilder(AppDomain.CurrentDomain.SetupInformation.ActivationArguments.ActivationData[index]).Uri; } } return uri; } } // This is the custom ApplicationActivator that will be returned by // the PresentationAppDomainManager.ApplicationActivator property. // CreateInstance will be called twice: the first time to create // the new AppDomain, and the second time to create the app inside // of the new AppDomain. internal class PresentationApplicationActivator : System.Runtime.Hosting.ApplicationActivator { ////// Critical - because this does an elevation to get the ID string. /// Safe - because this does not expose that string. /// [SecurityCritical, SecurityTreatAsSafe] public override ObjectHandle CreateInstance(ActivationContext actCtx) { if (EventTrace.IsEnabled(EventTrace.Flags.performance, EventTrace.Level.verbose)) { EventTrace.EventProvider.TraceEvent( EventTrace.Level.verbose, EventTrace.GuidFromId(EventTraceGuidId.HOSTINGGUID), (byte)EventTrace.HostingEvent.ApplicationActivatorCreateInstanceStart, PresentationAppDomainManager.ActivationUri != null ? PresentationAppDomainManager.ActivationUri.ToString() : string.Empty); } ObjectHandle oh; if (PresentationAppDomainManager.ActivationUri != null) { oh = base.CreateInstance( actCtx, new string[] { BindUriHelper.UriToString(PresentationAppDomainManager.ActivationUri), PresentationAppDomainManager.IsDebug.ToString(), (PresentationAppDomainManager.DebugSecurityZoneURL == null? string.Empty : PresentationAppDomainManager.DebugSecurityZoneURL.ToString())}); } else { oh = base.CreateInstance(actCtx); } bool returnAppDomain = false; try { new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Assert(); // BlessedAssert: if (AppDomain.CurrentDomain.ActivationContext != null && AppDomain.CurrentDomain.ActivationContext.Identity.ToString().Equals(actCtx.Identity.ToString())) { returnAppDomain = true; } } finally { CodeAccessPermission.RevertAssert(); } if (EventTrace.IsEnabled(EventTrace.Flags.performance, EventTrace.Level.verbose)) { EventTrace.EventProvider.TraceEvent(EventTrace.Level.verbose, EventTrace.GuidFromId(EventTraceGuidId.HOSTINGGUID), (byte)EventTrace.HostingEvent.ApplicationActivatorCreateInstanceEnd); } if (returnAppDomain) { // this is the server domain return new ObjectHandle(AppDomain.CurrentDomain); } else { return oh; } } } // This is a custom AppDomainManager class we're using. We need to set the // assembly name and class name in the environment for CLR to use it. We // need to use this to detect new AppDomain creation. internal class PresentationAppDomainManager : AppDomainManager { ////// Critical: Initializes Critical data /// [SecurityCritical, SecurityTreatAsSafe] static PresentationAppDomainManager() { if (EventTrace.IsEnabled(EventTrace.Flags.performance, EventTrace.Level.verbose)) { EventTrace.EventProvider.TraceEvent(EventTrace.Level.verbose, EventTrace.GuidFromId(EventTraceGuidId.HOSTINGGUID), (byte)EventTrace.HostingEvent.AppDomainManagerCctor); } } ////// Critical: Base class has link demnd and inheritance demand /// [SecurityCritical] public PresentationAppDomainManager() { } public override ApplicationActivator ApplicationActivator { get { if (_appActivator == null) _appActivator = new PresentationApplicationActivator(); return _appActivator; } } ////// Critical: This hooks up the assembly filter which we want to prevent arbitrary code from doing. /// [SecurityCritical] public override void InitializeNewDomain(AppDomainSetup appDomainInfo) { //Hookup the assembly load event _assemblyFilter = new AssemblyFilter(); AppDomain.CurrentDomain.AssemblyLoad += new AssemblyLoadEventHandler(_assemblyFilter.FilterCallback); } ////// Critical - get: Discloses a HostSecurityManager object /// public override HostSecurityManager HostSecurityManager { [SecurityCritical] get { if (_hostsecuritymanager == null) { _hostsecuritymanager = new PresentationHostSecurityManager(); } return _hostsecuritymanager; } } // Creates ApplicationProxyInternal. Creating it from Default domain will // cause a stack walk for ReflectionPermission which will fail for partial // trust apps. ////// Critical: This calls the critical ApplicationProxyInternal ctor /// [SecurityCritical] internal ApplicationProxyInternal CreateApplicationProxyInternal() { return new ApplicationProxyInternal(); } internal static AppDomain NewAppDomain { get { return _newAppDomain; } set { _newAppDomain = value; } } internal static bool SaveAppDomain { get { return _saveAppDomain; } set { _saveAppDomain = value; // Allow garbage collection to happen. _newAppDomain = null; } } internal static Uri ActivationUri { get { return _activationUri; } set { _activationUri = value; } } internal static Uri DebugSecurityZoneURL { get { return _debugSecurityZoneURL; } set { _debugSecurityZoneURL = value; } } internal static bool IsDebug { get { return _isdebug; } set { _isdebug = value; } } private static bool _isdebug = false; private ApplicationActivator _appActivator = null; ////// It holds a HostSecurityManager object, which is critical and can interfere with the permissions that are assigned to an AppDomain. /// [SecurityCritical] private HostSecurityManager _hostsecuritymanager = null; private static AppDomain _newAppDomain; private static bool _saveAppDomain; private static Uri _activationUri; private static Uri _debugSecurityZoneURL; ////// Critical: This should not be exposed since it can be used to bring down process /// [SecurityCritical] private AssemblyFilter _assemblyFilter; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Implements a custom AppDomainManager. // //--------------------------------------------------------------------------- using System; using System.Net; using System.Reflection; using System.Runtime.Remoting; using System.Security; using System.Security.Permissions; using System.Security.Policy; using System.Runtime.Hosting; using System.Text; using MS.Win32; using MS.Internal; using MS.Internal.AppModel; using MS.Internal.Utility; using MS.Utility; namespace System.Windows.Interop { internal class PresentationHostSecurityManager : HostSecurityManager { ////// Critical - HostSecurityManager..ctor LinkDemand's. This class should not be called directly by PT'ed callers, since it can interfere with /// the privileges an AppDomain gets. /// [SecurityCritical] internal PresentationHostSecurityManager() { } ////// Critical: /// 1) accesses critical data AppDomain.CurrentDomain.SetupInformation.ActivationArguments /// and sets BrowserInteropHelper.IsBrowserHosted. /// 2) calls the critical AddPermissionForUri(). /// Safe: /// 1) does not expose critical data; BrowserInteropHelper.IsBrowserHosted is set to true, as it /// has to be. /// 2) The additional permission added is to the "fake" site-of-origin used for debugging. /// [SecurityCritical, SecurityTreatAsSafe] public override ApplicationTrust DetermineApplicationTrust(Evidence applicationEvidence, Evidence activatorEvidence, TrustManagerContext context) { if (EventTrace.IsEnabled(EventTrace.Flags.performance, EventTrace.Level.verbose)) { EventTrace.EventProvider.TraceEvent( EventTrace.Level.verbose, EventTrace.GuidFromId(EventTraceGuidId.HOSTINGGUID), (byte)EventTrace.HostingEvent.DetermineApplicationTrustStart); } ApplicationTrust trust; Uri activationUri = GetUriFromActivationData(0); bool isDebug = PresentationAppDomainManager.IsDebug ? true : GetBoolFromActivationData(1); BrowserInteropHelper.SetBrowserHosted(true); if (isDebug) { context.IgnorePersistedDecision = true; context.Persist = false; context.KeepAlive = false; context.NoPrompt = true; trust = base.DetermineApplicationTrust(applicationEvidence, activatorEvidence, context); Uri debugSecurityZoneURL = GetUriFromActivationData(2); if (debugSecurityZoneURL != null) { PermissionSet permissions = trust.DefaultGrantSet.PermissionSet; trust.DefaultGrantSet.PermissionSet = AddPermissionForUri(permissions, debugSecurityZoneURL); } } else { // Defense in depth. Should never get to this point and require this to be // set, but just in case, we do this so that we will never get a trust // prompt even if something goes wrong somewhere else. context.NoPrompt = true; trust = base.DetermineApplicationTrust(applicationEvidence, activatorEvidence, context); } if (EventTrace.IsEnabled(EventTrace.Flags.performance, EventTrace.Level.verbose)) { EventTrace.EventProvider.TraceEvent( EventTrace.Level.verbose, EventTrace.GuidFromId(EventTraceGuidId.HOSTINGGUID), (byte)EventTrace.HostingEvent.DetermineApplicationTrustEnd); } return trust; } ////// Critical - because adds permission to default permissionset granted by trustmanager. /// CAUTION: If srcUri denotes a directory path, it must end with '/'. Otherwise permission will /// be granted to the parent directory. /// [SecurityCritical] internal static PermissionSet AddPermissionForUri(PermissionSet originalPermSet, Uri srcUri) { PermissionSet newPermSet = originalPermSet; if (srcUri != null) { Evidence evidence = new Evidence(); evidence.AddHost(new Url(BindUriHelper.UriToString(srcUri))); // important: the parameter must be a UrL object not a UrI object IMembershipCondition membership = new UrlMembershipCondition(BindUriHelper.UriToString(srcUri)); CodeGroup group = (srcUri.IsFile) ? (CodeGroup)new FileCodeGroup(membership, FileIOPermissionAccess.Read | FileIOPermissionAccess.PathDiscovery) :(CodeGroup)new NetCodeGroup(membership); PolicyStatement policy = group.Resolve(evidence); if (!policy.PermissionSet.IsEmpty()) { newPermSet = originalPermSet.Union(policy.PermissionSet); } } return newPermSet; } ////// Critical - because this accesses critical data AppDomain.CurrentDomain.SetupInformation.ActivationArguments. /// Safe - because this does not expose that string. /// [SecurityCritical, SecurityTreatAsSafe] private bool GetBoolFromActivationData(int index) { bool flag = false; // default if (AppDomain.CurrentDomain.SetupInformation.ActivationArguments != null && AppDomain.CurrentDomain.SetupInformation.ActivationArguments.ActivationData.Length > index) { if (AppDomain.CurrentDomain.SetupInformation.ActivationArguments.ActivationData[index] == true.ToString()) { flag = true; } } return flag; } ////// Critical - because this accesses critical data AppDomain.CurrentDomain.SetupInformation.ActivationArguments. /// Safe - because this does not expose that string. /// [SecurityCritical, SecurityTreatAsSafe] private Uri GetUriFromActivationData(int index) { Uri uri = null; if (AppDomain.CurrentDomain.SetupInformation.ActivationArguments != null && AppDomain.CurrentDomain.SetupInformation.ActivationArguments.ActivationData.Length > index) { if (!string.IsNullOrEmpty(AppDomain.CurrentDomain.SetupInformation.ActivationArguments.ActivationData[index])) { uri = new UriBuilder(AppDomain.CurrentDomain.SetupInformation.ActivationArguments.ActivationData[index]).Uri; } } return uri; } } // This is the custom ApplicationActivator that will be returned by // the PresentationAppDomainManager.ApplicationActivator property. // CreateInstance will be called twice: the first time to create // the new AppDomain, and the second time to create the app inside // of the new AppDomain. internal class PresentationApplicationActivator : System.Runtime.Hosting.ApplicationActivator { ////// Critical - because this does an elevation to get the ID string. /// Safe - because this does not expose that string. /// [SecurityCritical, SecurityTreatAsSafe] public override ObjectHandle CreateInstance(ActivationContext actCtx) { if (EventTrace.IsEnabled(EventTrace.Flags.performance, EventTrace.Level.verbose)) { EventTrace.EventProvider.TraceEvent( EventTrace.Level.verbose, EventTrace.GuidFromId(EventTraceGuidId.HOSTINGGUID), (byte)EventTrace.HostingEvent.ApplicationActivatorCreateInstanceStart, PresentationAppDomainManager.ActivationUri != null ? PresentationAppDomainManager.ActivationUri.ToString() : string.Empty); } ObjectHandle oh; if (PresentationAppDomainManager.ActivationUri != null) { oh = base.CreateInstance( actCtx, new string[] { BindUriHelper.UriToString(PresentationAppDomainManager.ActivationUri), PresentationAppDomainManager.IsDebug.ToString(), (PresentationAppDomainManager.DebugSecurityZoneURL == null? string.Empty : PresentationAppDomainManager.DebugSecurityZoneURL.ToString())}); } else { oh = base.CreateInstance(actCtx); } bool returnAppDomain = false; try { new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Assert(); // BlessedAssert: if (AppDomain.CurrentDomain.ActivationContext != null && AppDomain.CurrentDomain.ActivationContext.Identity.ToString().Equals(actCtx.Identity.ToString())) { returnAppDomain = true; } } finally { CodeAccessPermission.RevertAssert(); } if (EventTrace.IsEnabled(EventTrace.Flags.performance, EventTrace.Level.verbose)) { EventTrace.EventProvider.TraceEvent(EventTrace.Level.verbose, EventTrace.GuidFromId(EventTraceGuidId.HOSTINGGUID), (byte)EventTrace.HostingEvent.ApplicationActivatorCreateInstanceEnd); } if (returnAppDomain) { // this is the server domain return new ObjectHandle(AppDomain.CurrentDomain); } else { return oh; } } } // This is a custom AppDomainManager class we're using. We need to set the // assembly name and class name in the environment for CLR to use it. We // need to use this to detect new AppDomain creation. internal class PresentationAppDomainManager : AppDomainManager { ////// Critical: Initializes Critical data /// [SecurityCritical, SecurityTreatAsSafe] static PresentationAppDomainManager() { if (EventTrace.IsEnabled(EventTrace.Flags.performance, EventTrace.Level.verbose)) { EventTrace.EventProvider.TraceEvent(EventTrace.Level.verbose, EventTrace.GuidFromId(EventTraceGuidId.HOSTINGGUID), (byte)EventTrace.HostingEvent.AppDomainManagerCctor); } } ////// Critical: Base class has link demnd and inheritance demand /// [SecurityCritical] public PresentationAppDomainManager() { } public override ApplicationActivator ApplicationActivator { get { if (_appActivator == null) _appActivator = new PresentationApplicationActivator(); return _appActivator; } } ////// Critical: This hooks up the assembly filter which we want to prevent arbitrary code from doing. /// [SecurityCritical] public override void InitializeNewDomain(AppDomainSetup appDomainInfo) { //Hookup the assembly load event _assemblyFilter = new AssemblyFilter(); AppDomain.CurrentDomain.AssemblyLoad += new AssemblyLoadEventHandler(_assemblyFilter.FilterCallback); } ////// Critical - get: Discloses a HostSecurityManager object /// public override HostSecurityManager HostSecurityManager { [SecurityCritical] get { if (_hostsecuritymanager == null) { _hostsecuritymanager = new PresentationHostSecurityManager(); } return _hostsecuritymanager; } } // Creates ApplicationProxyInternal. Creating it from Default domain will // cause a stack walk for ReflectionPermission which will fail for partial // trust apps. ////// Critical: This calls the critical ApplicationProxyInternal ctor /// [SecurityCritical] internal ApplicationProxyInternal CreateApplicationProxyInternal() { return new ApplicationProxyInternal(); } internal static AppDomain NewAppDomain { get { return _newAppDomain; } set { _newAppDomain = value; } } internal static bool SaveAppDomain { get { return _saveAppDomain; } set { _saveAppDomain = value; // Allow garbage collection to happen. _newAppDomain = null; } } internal static Uri ActivationUri { get { return _activationUri; } set { _activationUri = value; } } internal static Uri DebugSecurityZoneURL { get { return _debugSecurityZoneURL; } set { _debugSecurityZoneURL = value; } } internal static bool IsDebug { get { return _isdebug; } set { _isdebug = value; } } private static bool _isdebug = false; private ApplicationActivator _appActivator = null; ////// It holds a HostSecurityManager object, which is critical and can interfere with the permissions that are assigned to an AppDomain. /// [SecurityCritical] private HostSecurityManager _hostsecuritymanager = null; private static AppDomain _newAppDomain; private static bool _saveAppDomain; private static Uri _activationUri; private static Uri _debugSecurityZoneURL; ////// Critical: This should not be exposed since it can be used to bring down process /// [SecurityCritical] private AssemblyFilter _assemblyFilter; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
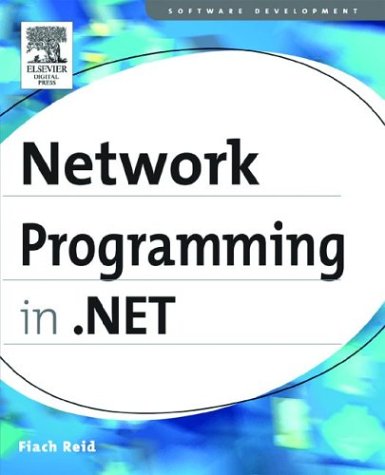
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Point3D.cs
- PolyQuadraticBezierSegment.cs
- XmlCharCheckingWriter.cs
- ComplexPropertyEntry.cs
- StrongNamePublicKeyBlob.cs
- MessageSmuggler.cs
- DocComment.cs
- StorageMappingFragment.cs
- CodeMemberEvent.cs
- MetafileHeader.cs
- DataSourceProvider.cs
- GetUserPreferenceRequest.cs
- StringToken.cs
- SamlSecurityTokenAuthenticator.cs
- MetaForeignKeyColumn.cs
- WebPartCatalogAddVerb.cs
- GradientStop.cs
- FixedPage.cs
- Tile.cs
- RelationshipEnd.cs
- SpeechDetectedEventArgs.cs
- ControlAdapter.cs
- AssertFilter.cs
- WeakReferenceEnumerator.cs
- DataObjectCopyingEventArgs.cs
- EntityStoreSchemaGenerator.cs
- BitmapEffectState.cs
- AppDomainUnloadedException.cs
- BaseCodeDomTreeGenerator.cs
- SqlSupersetValidator.cs
- DbFunctionCommandTree.cs
- HtmlAnchor.cs
- PhonemeConverter.cs
- HtmlElementErrorEventArgs.cs
- ObjectItemAttributeAssemblyLoader.cs
- TabControlAutomationPeer.cs
- DecoderNLS.cs
- RemotingConfiguration.cs
- RSAOAEPKeyExchangeDeformatter.cs
- PageAsyncTaskManager.cs
- Point4DConverter.cs
- RtType.cs
- ResourceDescriptionAttribute.cs
- HTMLTextWriter.cs
- ResolveMatchesApril2005.cs
- DocumentXPathNavigator.cs
- QilPatternFactory.cs
- ScrollItemProviderWrapper.cs
- ClientSettingsSection.cs
- Rotation3D.cs
- PopOutPanel.cs
- StringReader.cs
- Span.cs
- RetriableClipboard.cs
- ProfilePropertySettings.cs
- PolyLineSegment.cs
- DesignerSerializationManager.cs
- ProxyWebPartManager.cs
- ProjectionRewriter.cs
- MetadataPropertyCollection.cs
- Atom10FormatterFactory.cs
- ListItem.cs
- SchemaCollectionCompiler.cs
- CounterSample.cs
- UnitySerializationHolder.cs
- RelatedEnd.cs
- VisualTransition.cs
- KeyToListMap.cs
- RijndaelManagedTransform.cs
- AutoResizedEvent.cs
- ExpressionCopier.cs
- DataReceivedEventArgs.cs
- StyleReferenceConverter.cs
- DictionaryContent.cs
- RayMeshGeometry3DHitTestResult.cs
- PEFileEvidenceFactory.cs
- Vector3DAnimation.cs
- EventProviderWriter.cs
- XamlSerializationHelper.cs
- CreateUserWizardStep.cs
- ProcessInputEventArgs.cs
- ConfigUtil.cs
- Stacktrace.cs
- InterleavedZipPartStream.cs
- QuaternionRotation3D.cs
- EventToken.cs
- X500Name.cs
- _NegoStream.cs
- AssociatedControlConverter.cs
- HttpContext.cs
- ProviderCollection.cs
- basecomparevalidator.cs
- OracleTransaction.cs
- CultureInfo.cs
- HtmlSelectionListAdapter.cs
- MenuItemBindingCollection.cs
- EndOfStreamException.cs
- KnownTypes.cs
- WebSysDisplayNameAttribute.cs
- ParameterToken.cs