Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / MetadataPropertyCollection.cs / 2 / MetadataPropertyCollection.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....],[....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Diagnostics; using System.Reflection; using System.Data.Common.Utils; namespace System.Data.Metadata.Edm { ////// Metadata collection class supporting delay-loading of system item attributes and /// extended attributes. /// internal sealed class MetadataPropertyCollection : MetadataCollection{ /// /// Constructor taking item. /// /// Item with which the collection is associated. internal MetadataPropertyCollection(MetadataItem item) : base(GetSystemMetadataProperties(item)) { } private readonly static Memoizers_itemTypeMemoizer = new Memoizer (clrType => new ItemTypeInformation(clrType), null); // Given an item, returns all system type attributes for the item. private static IEnumerable GetSystemMetadataProperties(MetadataItem item) { EntityUtil.CheckArgumentNull(item, "item"); Type type = item.GetType(); ItemTypeInformation itemTypeInformation = GetItemTypeInformation(type); return itemTypeInformation.GetItemAttributes(item); } // Retrieves metadata for type. private static ItemTypeInformation GetItemTypeInformation(Type clrType) { return s_itemTypeMemoizer.Evaluate(clrType); } /// /// Encapsulates information about system item attributes for a particular item type. /// private class ItemTypeInformation { ////// Retrieves system attribute information for the given type. /// Requires: type must derive from MetadataItem /// /// Type internal ItemTypeInformation(Type clrType) { Debug.Assert(null != clrType); _itemProperties = GetItemProperties(clrType); } private readonly List_itemProperties; // Returns system item attributes for the given item. internal IEnumerable GetItemAttributes(MetadataItem item) { foreach (ItemPropertyInfo propertyInfo in _itemProperties) { yield return propertyInfo.GetMetadataProperty(item); } } // Gets type information for item with the given type. Uses cached information where // available. private static List GetItemProperties(Type clrType) { List result = new List (); foreach (PropertyInfo propertyInfo in clrType.GetProperties(BindingFlags.Instance | BindingFlags.Public | BindingFlags.NonPublic)) { foreach (MetadataPropertyAttribute attribute in propertyInfo.GetCustomAttributes( typeof(MetadataPropertyAttribute), false)) { result.Add(new ItemPropertyInfo(propertyInfo, attribute)); } } return result; } } /// /// Encapsulates information about a CLR property of an item class. /// private class ItemPropertyInfo { ////// Initialize information. /// Requires: attribute must belong to the given property. /// /// Property referenced. /// Attribute for the property. internal ItemPropertyInfo(PropertyInfo propertyInfo, MetadataPropertyAttribute attribute) { Debug.Assert(null != propertyInfo); Debug.Assert(null != attribute); _propertyInfo = propertyInfo; _attribute = attribute; } private readonly MetadataPropertyAttribute _attribute; private readonly PropertyInfo _propertyInfo; ////// Given an item, returns an instance of the item attribute described by this class. /// /// Item from which to retrieve attribute. ///Item attribute. internal MetadataProperty GetMetadataProperty(MetadataItem item) { return new MetadataProperty(_propertyInfo.Name, _attribute.Type, _attribute.IsCollectionType, new MetadataPropertyValue(_propertyInfo, item)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....],[....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Diagnostics; using System.Reflection; using System.Data.Common.Utils; namespace System.Data.Metadata.Edm { ////// Metadata collection class supporting delay-loading of system item attributes and /// extended attributes. /// internal sealed class MetadataPropertyCollection : MetadataCollection{ /// /// Constructor taking item. /// /// Item with which the collection is associated. internal MetadataPropertyCollection(MetadataItem item) : base(GetSystemMetadataProperties(item)) { } private readonly static Memoizers_itemTypeMemoizer = new Memoizer (clrType => new ItemTypeInformation(clrType), null); // Given an item, returns all system type attributes for the item. private static IEnumerable GetSystemMetadataProperties(MetadataItem item) { EntityUtil.CheckArgumentNull(item, "item"); Type type = item.GetType(); ItemTypeInformation itemTypeInformation = GetItemTypeInformation(type); return itemTypeInformation.GetItemAttributes(item); } // Retrieves metadata for type. private static ItemTypeInformation GetItemTypeInformation(Type clrType) { return s_itemTypeMemoizer.Evaluate(clrType); } /// /// Encapsulates information about system item attributes for a particular item type. /// private class ItemTypeInformation { ////// Retrieves system attribute information for the given type. /// Requires: type must derive from MetadataItem /// /// Type internal ItemTypeInformation(Type clrType) { Debug.Assert(null != clrType); _itemProperties = GetItemProperties(clrType); } private readonly List_itemProperties; // Returns system item attributes for the given item. internal IEnumerable GetItemAttributes(MetadataItem item) { foreach (ItemPropertyInfo propertyInfo in _itemProperties) { yield return propertyInfo.GetMetadataProperty(item); } } // Gets type information for item with the given type. Uses cached information where // available. private static List GetItemProperties(Type clrType) { List result = new List (); foreach (PropertyInfo propertyInfo in clrType.GetProperties(BindingFlags.Instance | BindingFlags.Public | BindingFlags.NonPublic)) { foreach (MetadataPropertyAttribute attribute in propertyInfo.GetCustomAttributes( typeof(MetadataPropertyAttribute), false)) { result.Add(new ItemPropertyInfo(propertyInfo, attribute)); } } return result; } } /// /// Encapsulates information about a CLR property of an item class. /// private class ItemPropertyInfo { ////// Initialize information. /// Requires: attribute must belong to the given property. /// /// Property referenced. /// Attribute for the property. internal ItemPropertyInfo(PropertyInfo propertyInfo, MetadataPropertyAttribute attribute) { Debug.Assert(null != propertyInfo); Debug.Assert(null != attribute); _propertyInfo = propertyInfo; _attribute = attribute; } private readonly MetadataPropertyAttribute _attribute; private readonly PropertyInfo _propertyInfo; ////// Given an item, returns an instance of the item attribute described by this class. /// /// Item from which to retrieve attribute. ///Item attribute. internal MetadataProperty GetMetadataProperty(MetadataItem item) { return new MetadataProperty(_propertyInfo.Name, _attribute.Type, _attribute.IsCollectionType, new MetadataPropertyValue(_propertyInfo, item)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
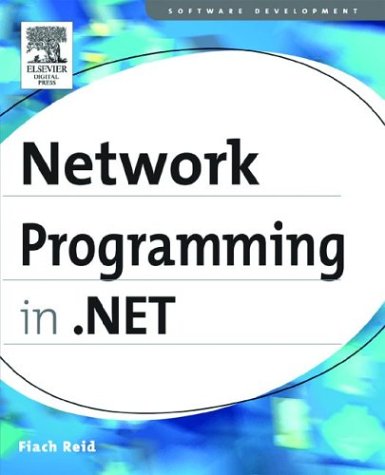
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextRangeEditTables.cs
- EventItfInfo.cs
- ImageInfo.cs
- CapabilitiesUse.cs
- IsolatedStorageException.cs
- HttpRawResponse.cs
- ServiceDeploymentInfo.cs
- DoubleSumAggregationOperator.cs
- ListManagerBindingsCollection.cs
- ListViewGroupConverter.cs
- DbProviderConfigurationHandler.cs
- XmlSchemaGroup.cs
- MenuItemCollection.cs
- WebServiceBindingAttribute.cs
- Validator.cs
- contentDescriptor.cs
- SystemIPGlobalProperties.cs
- SpellerHighlightLayer.cs
- Stream.cs
- MultiPageTextView.cs
- LineVisual.cs
- UserValidatedEventArgs.cs
- FilterException.cs
- CallTemplateAction.cs
- Baml2006KnownTypes.cs
- SqlVisitor.cs
- Size3DValueSerializer.cs
- GenericTypeParameterBuilder.cs
- DataRowChangeEvent.cs
- cookieexception.cs
- MexServiceChannelBuilder.cs
- AuthorizationRule.cs
- CustomExpression.cs
- HMACSHA256.cs
- _CacheStreams.cs
- DesignerActionKeyboardBehavior.cs
- TimeoutException.cs
- ToolStripItemClickedEventArgs.cs
- ServiceDurableInstance.cs
- DataStreamFromComStream.cs
- FileAuthorizationModule.cs
- CodeTypeReferenceCollection.cs
- ApplicationHost.cs
- InputEventArgs.cs
- AssemblyAttributes.cs
- TraceData.cs
- CheckBoxField.cs
- SessionStateSection.cs
- TextAction.cs
- CodeDirectoryCompiler.cs
- SqlTypesSchemaImporter.cs
- CloudCollection.cs
- AggregateNode.cs
- HtmlForm.cs
- SmtpNtlmAuthenticationModule.cs
- ProviderUtil.cs
- Stylesheet.cs
- CounterSetInstance.cs
- MaskedTextProvider.cs
- TemplatedAdorner.cs
- MasterPageCodeDomTreeGenerator.cs
- XmlDomTextWriter.cs
- ValidationError.cs
- Polyline.cs
- MenuBindingsEditor.cs
- CookielessHelper.cs
- DoubleIndependentAnimationStorage.cs
- X509DefaultServiceCertificateElement.cs
- objectquery_tresulttype.cs
- AppDomain.cs
- NativeWindow.cs
- Transform3DGroup.cs
- HtmlTextBoxAdapter.cs
- sqlser.cs
- DefaultAssemblyResolver.cs
- COM2AboutBoxPropertyDescriptor.cs
- OleDbFactory.cs
- MissingManifestResourceException.cs
- IntranetCredentialPolicy.cs
- OleDbFactory.cs
- String.cs
- XpsS0ValidatingLoader.cs
- EntityRecordInfo.cs
- selecteditemcollection.cs
- WorkflowServiceHostFactory.cs
- MailBnfHelper.cs
- GridViewColumnHeader.cs
- ImageFormat.cs
- EntityDataSourceDesigner.cs
- EntityException.cs
- DependencyProperty.cs
- ProgressBarHighlightConverter.cs
- XPathNodeInfoAtom.cs
- StreamGeometryContext.cs
- Frame.cs
- SqlRewriteScalarSubqueries.cs
- CqlWriter.cs
- Soap.cs
- OAVariantLib.cs
- ColorTranslator.cs